JSON Serialization
6 mins
6 mins

Ashutosh
Published on Oct 16, 2024
JSON and Serialization in Flutter: Complete Guide for Flutter App
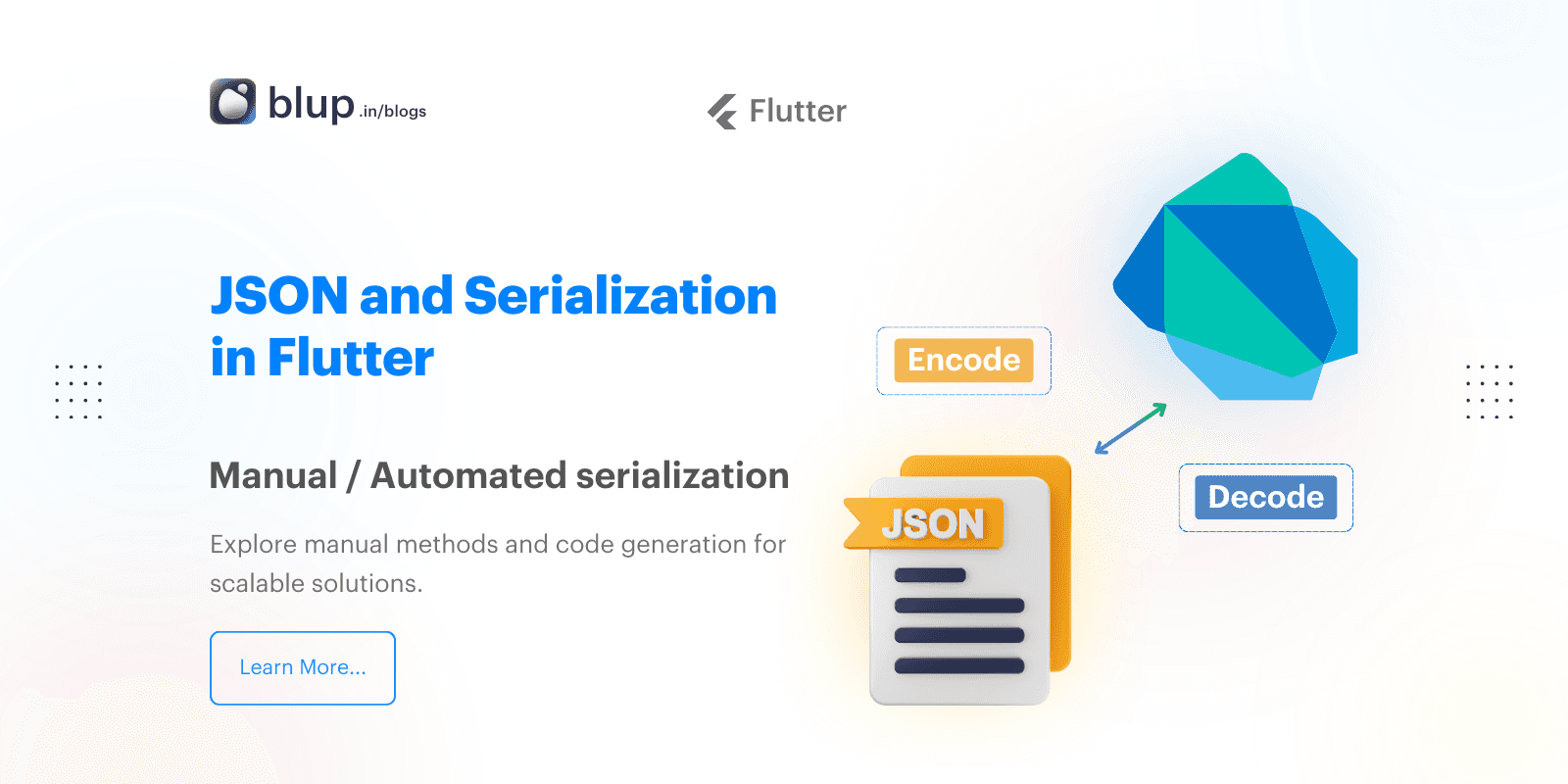
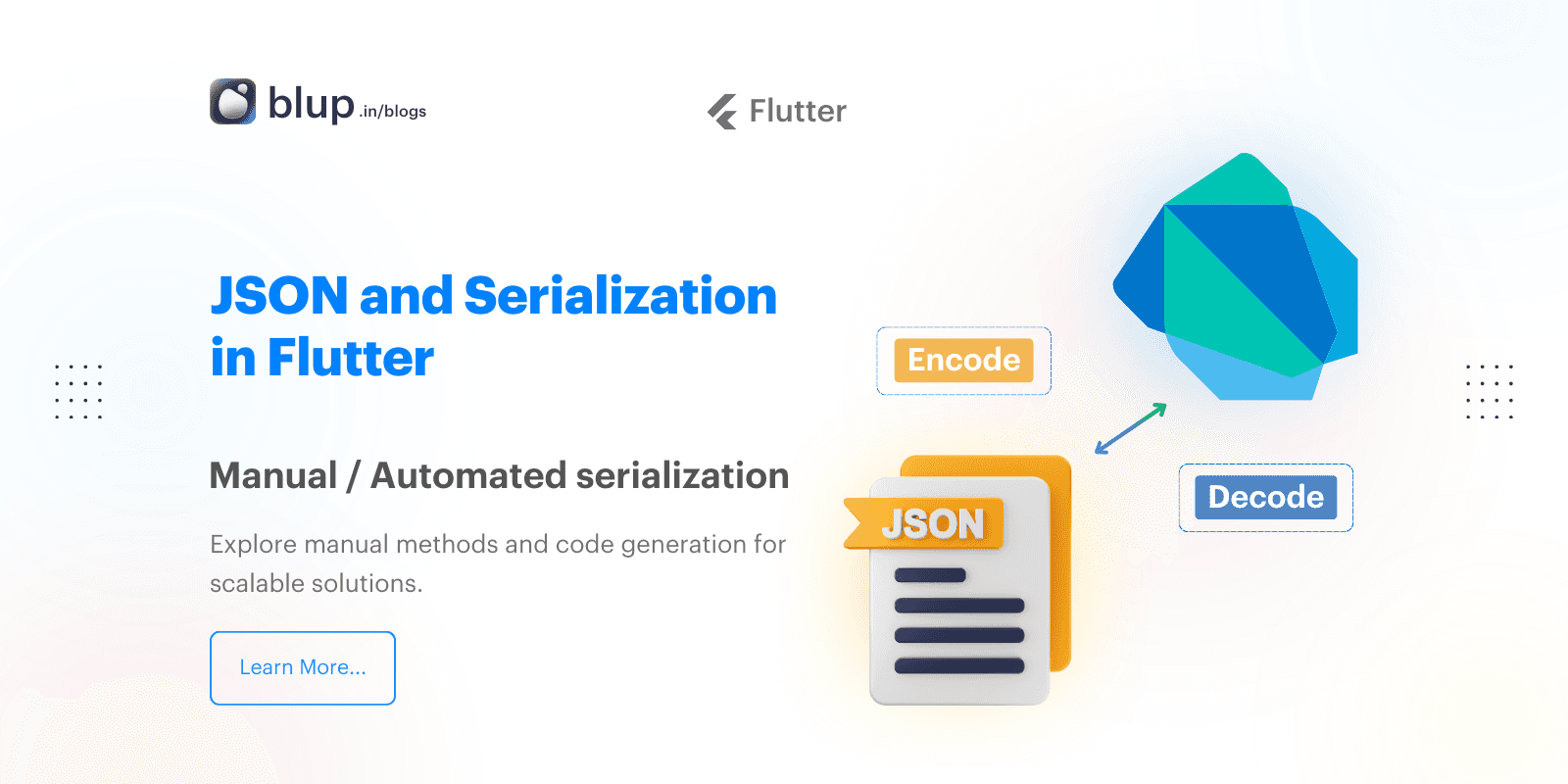
Introduction
Introduction
Introduction
Introduction
When building Flutter apps, especially when working with external APIs or communicating with servers, handling JSON data and serializing it into Dart objects is essential. This process, JSON serialization, is integral to building efficient, scalable Flutter applications. In this blog, we’ll explore different approaches to JSON serialization, from manual methods to code generation and help you decide which method is right for your Flutter app development process.
Contents
Which JSON serialization method is right for me?
Use manual serialization for smaller projects
Use code generation for medium to large projects
Is there a GSON/Jackson/Moshi equivalent in Flutter?
Serializing JSON manually using
dart:convert
Serializing JSON inline
Serializing JSON inside model classes
Serializing JSON using code generation libraries
Setting up
json_serializable
in a projectCreating model classes the
json_serializable
wayRunning the code generation utility
Consuming
json_serializable
modelsGenerating code for nested classes
Further references
When building Flutter apps, especially when working with external APIs or communicating with servers, handling JSON data and serializing it into Dart objects is essential. This process, JSON serialization, is integral to building efficient, scalable Flutter applications. In this blog, we’ll explore different approaches to JSON serialization, from manual methods to code generation and help you decide which method is right for your Flutter app development process.
Contents
Which JSON serialization method is right for me?
Use manual serialization for smaller projects
Use code generation for medium to large projects
Is there a GSON/Jackson/Moshi equivalent in Flutter?
Serializing JSON manually using
dart:convert
Serializing JSON inline
Serializing JSON inside model classes
Serializing JSON using code generation libraries
Setting up
json_serializable
in a projectCreating model classes the
json_serializable
wayRunning the code generation utility
Consuming
json_serializable
modelsGenerating code for nested classes
Further references
When building Flutter apps, especially when working with external APIs or communicating with servers, handling JSON data and serializing it into Dart objects is essential. This process, JSON serialization, is integral to building efficient, scalable Flutter applications. In this blog, we’ll explore different approaches to JSON serialization, from manual methods to code generation and help you decide which method is right for your Flutter app development process.
Contents
Which JSON serialization method is right for me?
Use manual serialization for smaller projects
Use code generation for medium to large projects
Is there a GSON/Jackson/Moshi equivalent in Flutter?
Serializing JSON manually using
dart:convert
Serializing JSON inline
Serializing JSON inside model classes
Serializing JSON using code generation libraries
Setting up
json_serializable
in a projectCreating model classes the
json_serializable
wayRunning the code generation utility
Consuming
json_serializable
modelsGenerating code for nested classes
Further references
When building Flutter apps, especially when working with external APIs or communicating with servers, handling JSON data and serializing it into Dart objects is essential. This process, JSON serialization, is integral to building efficient, scalable Flutter applications. In this blog, we’ll explore different approaches to JSON serialization, from manual methods to code generation and help you decide which method is right for your Flutter app development process.
Contents
Which JSON serialization method is right for me?
Use manual serialization for smaller projects
Use code generation for medium to large projects
Is there a GSON/Jackson/Moshi equivalent in Flutter?
Serializing JSON manually using
dart:convert
Serializing JSON inline
Serializing JSON inside model classes
Serializing JSON using code generation libraries
Setting up
json_serializable
in a projectCreating model classes the
json_serializable
wayRunning the code generation utility
Consuming
json_serializable
modelsGenerating code for nested classes
Further references
JSON Serialization
JSON Serialization
JSON Serialization
JSON Serialization
Which JSON Serialization Method is Right for Me?
In Flutter development, there are multiple ways to handle JSON serialization, but the method you choose depends largely on the size and complexity of your project. We’ll cover both manual and automated approaches, so you can choose the method that best suits your Flutter app development needs.
Use Manual Serialization for Smaller Projects
Manual serialization involves writing code to explicitly convert JSON data to Dart objects and vice versa. This is ideal for smaller projects or simple Flutter apps where you don’t have many models to work with, as it keeps your codebase lightweight.
Pros:
Simple and easy to implement
No additional dependencies required
Full control over serialization
Cons:
Can become repetitive and error-prone as your project scales
Difficult to manage when working with nested or complex objects
Use Code Generation for Medium to Large Projects
For larger, more complex Flutter apps, code generation is a more efficient way to handle JSON serialization. By using libraries like json_serializable
, you can automate the process of generating serialization code, reducing human error and saving time.
Pros:
Automatically generates serialization code
Reduces repetitive code
Easier to maintain as the project scales
Cons:
Additional dependencies required
Requires an initial setup for code generation
Which JSON Serialization Method is Right for Me?
In Flutter development, there are multiple ways to handle JSON serialization, but the method you choose depends largely on the size and complexity of your project. We’ll cover both manual and automated approaches, so you can choose the method that best suits your Flutter app development needs.
Use Manual Serialization for Smaller Projects
Manual serialization involves writing code to explicitly convert JSON data to Dart objects and vice versa. This is ideal for smaller projects or simple Flutter apps where you don’t have many models to work with, as it keeps your codebase lightweight.
Pros:
Simple and easy to implement
No additional dependencies required
Full control over serialization
Cons:
Can become repetitive and error-prone as your project scales
Difficult to manage when working with nested or complex objects
Use Code Generation for Medium to Large Projects
For larger, more complex Flutter apps, code generation is a more efficient way to handle JSON serialization. By using libraries like json_serializable
, you can automate the process of generating serialization code, reducing human error and saving time.
Pros:
Automatically generates serialization code
Reduces repetitive code
Easier to maintain as the project scales
Cons:
Additional dependencies required
Requires an initial setup for code generation
Which JSON Serialization Method is Right for Me?
In Flutter development, there are multiple ways to handle JSON serialization, but the method you choose depends largely on the size and complexity of your project. We’ll cover both manual and automated approaches, so you can choose the method that best suits your Flutter app development needs.
Use Manual Serialization for Smaller Projects
Manual serialization involves writing code to explicitly convert JSON data to Dart objects and vice versa. This is ideal for smaller projects or simple Flutter apps where you don’t have many models to work with, as it keeps your codebase lightweight.
Pros:
Simple and easy to implement
No additional dependencies required
Full control over serialization
Cons:
Can become repetitive and error-prone as your project scales
Difficult to manage when working with nested or complex objects
Use Code Generation for Medium to Large Projects
For larger, more complex Flutter apps, code generation is a more efficient way to handle JSON serialization. By using libraries like json_serializable
, you can automate the process of generating serialization code, reducing human error and saving time.
Pros:
Automatically generates serialization code
Reduces repetitive code
Easier to maintain as the project scales
Cons:
Additional dependencies required
Requires an initial setup for code generation
Which JSON Serialization Method is Right for Me?
In Flutter development, there are multiple ways to handle JSON serialization, but the method you choose depends largely on the size and complexity of your project. We’ll cover both manual and automated approaches, so you can choose the method that best suits your Flutter app development needs.
Use Manual Serialization for Smaller Projects
Manual serialization involves writing code to explicitly convert JSON data to Dart objects and vice versa. This is ideal for smaller projects or simple Flutter apps where you don’t have many models to work with, as it keeps your codebase lightweight.
Pros:
Simple and easy to implement
No additional dependencies required
Full control over serialization
Cons:
Can become repetitive and error-prone as your project scales
Difficult to manage when working with nested or complex objects
Use Code Generation for Medium to Large Projects
For larger, more complex Flutter apps, code generation is a more efficient way to handle JSON serialization. By using libraries like json_serializable
, you can automate the process of generating serialization code, reducing human error and saving time.
Pros:
Automatically generates serialization code
Reduces repetitive code
Easier to maintain as the project scales
Cons:
Additional dependencies required
Requires an initial setup for code generation
Is There a GSON/Jackson/Moshi Equivalent in Flutter?
Is There a GSON/Jackson/Moshi Equivalent in Flutter?
Is There a GSON/Jackson/Moshi Equivalent in Flutter?
Is There a GSON/Jackson/Moshi Equivalent in Flutter?
If you're familiar with JSON serialization libraries like GSON, Jackson, or Moshi from Android development, you'll be pleased to know that Flutter has similar solutions. While there's no direct equivalent, the json_serializable
. The package comes close, offering code generation for converting Dart objects to and from JSON and making Flutter app development more seamless.
If you're familiar with JSON serialization libraries like GSON, Jackson, or Moshi from Android development, you'll be pleased to know that Flutter has similar solutions. While there's no direct equivalent, the json_serializable
. The package comes close, offering code generation for converting Dart objects to and from JSON and making Flutter app development more seamless.
If you're familiar with JSON serialization libraries like GSON, Jackson, or Moshi from Android development, you'll be pleased to know that Flutter has similar solutions. While there's no direct equivalent, the json_serializable
. The package comes close, offering code generation for converting Dart objects to and from JSON and making Flutter app development more seamless.
If you're familiar with JSON serialization libraries like GSON, Jackson, or Moshi from Android development, you'll be pleased to know that Flutter has similar solutions. While there's no direct equivalent, the json_serializable
. The package comes close, offering code generation for converting Dart objects to and from JSON and making Flutter app development more seamless.
Serializing JSON Manually Using dart:convert
Serializing JSON Manually Using dart:convert
Serializing JSON Manually Using dart:convert
Serializing JSON Manually Using dart:convert
Manual JSON serialization involves the dart:convert
library, which provides utilities for encoding and decoding JSON. Here’s how you can manually serialize JSON in a Flutter app:
import 'dart:convert';
class User {
final String name;
final int age;
User({required this.name, required this.age});
// Deserialize from JSON
factory User.fromJson(Map<String, dynamic> json) {
return User(
name: json['name'],
age: json['age'],
);
}
// Serialize to JSON
Map<String, dynamic> toJson() {
return {
'name': name,
'age': age,
};
}
}
void main() {
// Example JSON string
String jsonString = '{"name": "John", "age": 30}';
// Convert JSON to User object
Map<String, dynamic> userMap = jsonDecode(jsonString);
var user = User.fromJson(userMap);
// Convert User object to JSON
String json = jsonEncode(user.toJson());
print(json);
}
Serializing JSON Inline
You can even serialize and deserialize JSON directly without creating model classes for smaller Flutter apps or simpler data structures.
void main() {
String jsonString = '{"name": "John", "age": 30}';
// Parse JSON directly
Map<String, dynamic> user = jsonDecode(jsonString);
print('User Name: ${user['name']}');
}
Serializing JSON Inside Model Classes
For more structured code, it's a best practice to create model classes to handle JSON data. This keeps your Flutter UI and business logic cleaner, particularly when handling complex objects in Flutter development.
Manual JSON serialization involves the dart:convert
library, which provides utilities for encoding and decoding JSON. Here’s how you can manually serialize JSON in a Flutter app:
import 'dart:convert';
class User {
final String name;
final int age;
User({required this.name, required this.age});
// Deserialize from JSON
factory User.fromJson(Map<String, dynamic> json) {
return User(
name: json['name'],
age: json['age'],
);
}
// Serialize to JSON
Map<String, dynamic> toJson() {
return {
'name': name,
'age': age,
};
}
}
void main() {
// Example JSON string
String jsonString = '{"name": "John", "age": 30}';
// Convert JSON to User object
Map<String, dynamic> userMap = jsonDecode(jsonString);
var user = User.fromJson(userMap);
// Convert User object to JSON
String json = jsonEncode(user.toJson());
print(json);
}
Serializing JSON Inline
You can even serialize and deserialize JSON directly without creating model classes for smaller Flutter apps or simpler data structures.
void main() {
String jsonString = '{"name": "John", "age": 30}';
// Parse JSON directly
Map<String, dynamic> user = jsonDecode(jsonString);
print('User Name: ${user['name']}');
}
Serializing JSON Inside Model Classes
For more structured code, it's a best practice to create model classes to handle JSON data. This keeps your Flutter UI and business logic cleaner, particularly when handling complex objects in Flutter development.
Manual JSON serialization involves the dart:convert
library, which provides utilities for encoding and decoding JSON. Here’s how you can manually serialize JSON in a Flutter app:
import 'dart:convert';
class User {
final String name;
final int age;
User({required this.name, required this.age});
// Deserialize from JSON
factory User.fromJson(Map<String, dynamic> json) {
return User(
name: json['name'],
age: json['age'],
);
}
// Serialize to JSON
Map<String, dynamic> toJson() {
return {
'name': name,
'age': age,
};
}
}
void main() {
// Example JSON string
String jsonString = '{"name": "John", "age": 30}';
// Convert JSON to User object
Map<String, dynamic> userMap = jsonDecode(jsonString);
var user = User.fromJson(userMap);
// Convert User object to JSON
String json = jsonEncode(user.toJson());
print(json);
}
Serializing JSON Inline
You can even serialize and deserialize JSON directly without creating model classes for smaller Flutter apps or simpler data structures.
void main() {
String jsonString = '{"name": "John", "age": 30}';
// Parse JSON directly
Map<String, dynamic> user = jsonDecode(jsonString);
print('User Name: ${user['name']}');
}
Serializing JSON Inside Model Classes
For more structured code, it's a best practice to create model classes to handle JSON data. This keeps your Flutter UI and business logic cleaner, particularly when handling complex objects in Flutter development.
Manual JSON serialization involves the dart:convert
library, which provides utilities for encoding and decoding JSON. Here’s how you can manually serialize JSON in a Flutter app:
import 'dart:convert';
class User {
final String name;
final int age;
User({required this.name, required this.age});
// Deserialize from JSON
factory User.fromJson(Map<String, dynamic> json) {
return User(
name: json['name'],
age: json['age'],
);
}
// Serialize to JSON
Map<String, dynamic> toJson() {
return {
'name': name,
'age': age,
};
}
}
void main() {
// Example JSON string
String jsonString = '{"name": "John", "age": 30}';
// Convert JSON to User object
Map<String, dynamic> userMap = jsonDecode(jsonString);
var user = User.fromJson(userMap);
// Convert User object to JSON
String json = jsonEncode(user.toJson());
print(json);
}
Serializing JSON Inline
You can even serialize and deserialize JSON directly without creating model classes for smaller Flutter apps or simpler data structures.
void main() {
String jsonString = '{"name": "John", "age": 30}';
// Parse JSON directly
Map<String, dynamic> user = jsonDecode(jsonString);
print('User Name: ${user['name']}');
}
Serializing JSON Inside Model Classes
For more structured code, it's a best practice to create model classes to handle JSON data. This keeps your Flutter UI and business logic cleaner, particularly when handling complex objects in Flutter development.
Serializing JSON Using Code Generation Libraries
Serializing JSON Using Code Generation Libraries
Serializing JSON Using Code Generation Libraries
Serializing JSON Using Code Generation Libraries
Using code generation libraries json_serializable
streamlines the process of serializing JSON in larger Flutter apps. You don’t have to manually write boilerplate code for every model; instead, code generation handles it for you.
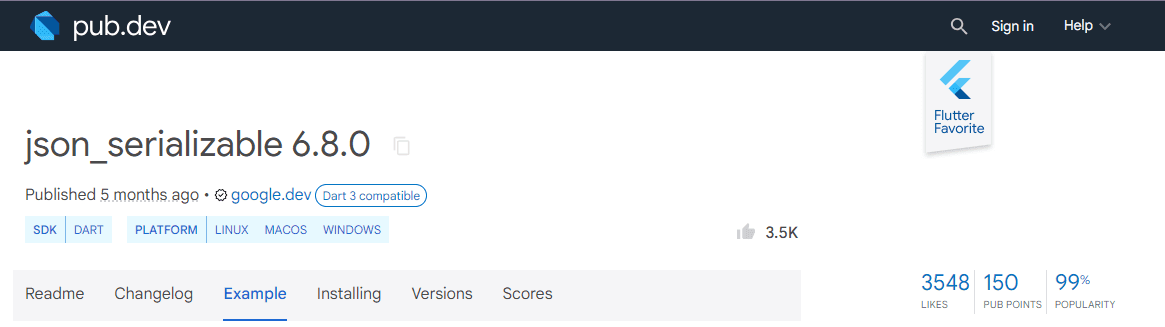
Setting Up json_serializable
in a Project
To set up json_serializable
, add the following dependencies to your pubspec.yaml
file:
dependencies:
json_annotation: ^4.0.1
dev_dependencies:
build_runner: ^2.0.0
json_serializable: ^4.0.0
Run flutter pub get
to install the dependencies.
Creating Model Classes the json_serializable
Annotate your model class with @JsonSerializable()
, and the library will generate a serialization code for you.
import 'package:json_annotation/json_annotation.dart';
part 'user.g.dart';
@JsonSerializable()
class User {
final String name;
final int age;
User({required this.name, required this.age});
factory User.fromJson(Map<String, dynamic> json) => _$UserFromJson(json);
Map<String, dynamic> toJson() => _$UserToJson(this);
}
Running the Code Generation Utility
Run the following command to generate the necessary serialization code:
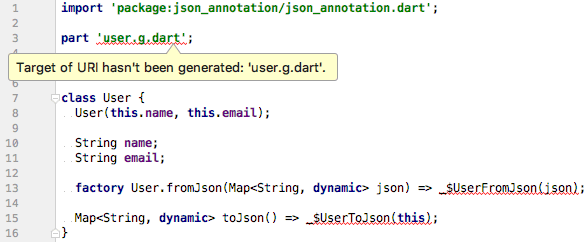
flutter pub run build_runner build
This generates the user.g.dart
file with the User.fromJson
and User.toJson
methods implemented automatically.
Consuming json_serializable
Models
Once the code is generated, you can use your model classes just as you would with manual serialization. Here’s how you can consume the User
class:
void main() {
String jsonString = '{"name": "John", "age": 30}';
Map<String, dynamic> userMap = jsonDecode(jsonString);
// Use the generated User class
User user = User.fromJson(userMap);
print('User Name: ${user.name}');
}
Using code generation libraries json_serializable
streamlines the process of serializing JSON in larger Flutter apps. You don’t have to manually write boilerplate code for every model; instead, code generation handles it for you.
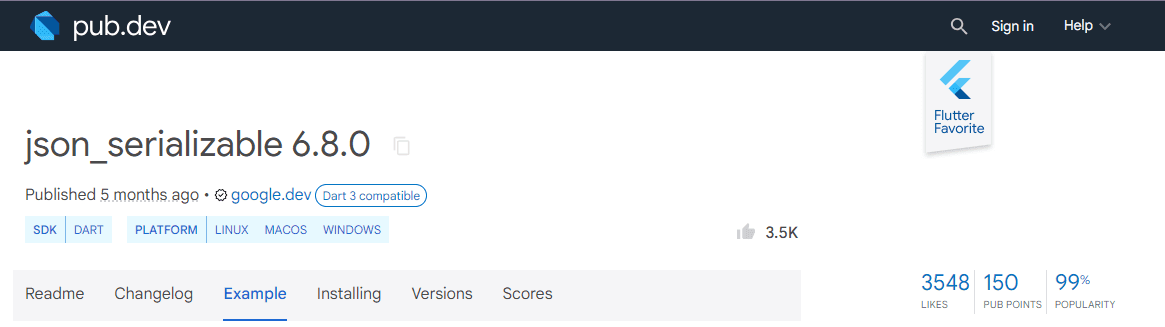
Setting Up json_serializable
in a Project
To set up json_serializable
, add the following dependencies to your pubspec.yaml
file:
dependencies:
json_annotation: ^4.0.1
dev_dependencies:
build_runner: ^2.0.0
json_serializable: ^4.0.0
Run flutter pub get
to install the dependencies.
Creating Model Classes the json_serializable
Annotate your model class with @JsonSerializable()
, and the library will generate a serialization code for you.
import 'package:json_annotation/json_annotation.dart';
part 'user.g.dart';
@JsonSerializable()
class User {
final String name;
final int age;
User({required this.name, required this.age});
factory User.fromJson(Map<String, dynamic> json) => _$UserFromJson(json);
Map<String, dynamic> toJson() => _$UserToJson(this);
}
Running the Code Generation Utility
Run the following command to generate the necessary serialization code:
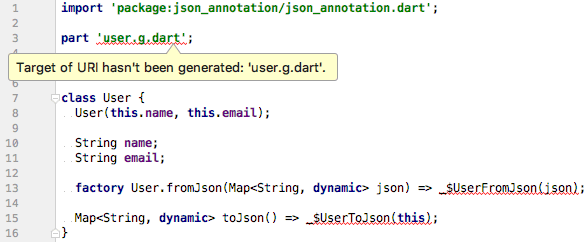
flutter pub run build_runner build
This generates the user.g.dart
file with the User.fromJson
and User.toJson
methods implemented automatically.
Consuming json_serializable
Models
Once the code is generated, you can use your model classes just as you would with manual serialization. Here’s how you can consume the User
class:
void main() {
String jsonString = '{"name": "John", "age": 30}';
Map<String, dynamic> userMap = jsonDecode(jsonString);
// Use the generated User class
User user = User.fromJson(userMap);
print('User Name: ${user.name}');
}
Using code generation libraries json_serializable
streamlines the process of serializing JSON in larger Flutter apps. You don’t have to manually write boilerplate code for every model; instead, code generation handles it for you.
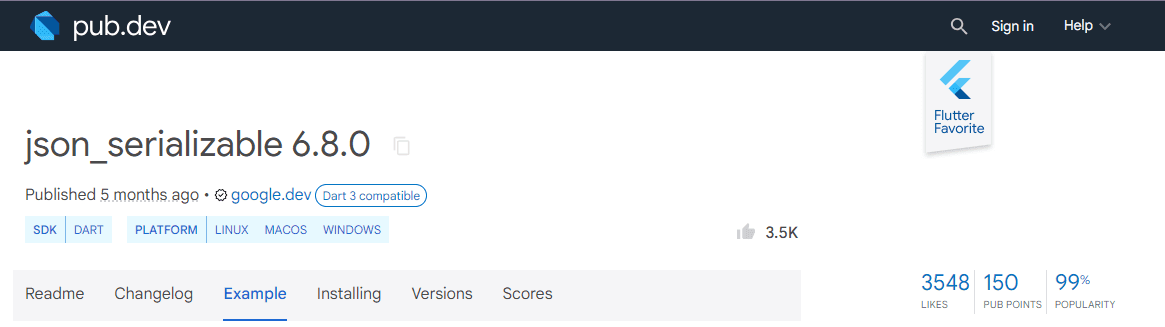
Setting Up json_serializable
in a Project
To set up json_serializable
, add the following dependencies to your pubspec.yaml
file:
dependencies:
json_annotation: ^4.0.1
dev_dependencies:
build_runner: ^2.0.0
json_serializable: ^4.0.0
Run flutter pub get
to install the dependencies.
Creating Model Classes the json_serializable
Annotate your model class with @JsonSerializable()
, and the library will generate a serialization code for you.
import 'package:json_annotation/json_annotation.dart';
part 'user.g.dart';
@JsonSerializable()
class User {
final String name;
final int age;
User({required this.name, required this.age});
factory User.fromJson(Map<String, dynamic> json) => _$UserFromJson(json);
Map<String, dynamic> toJson() => _$UserToJson(this);
}
Running the Code Generation Utility
Run the following command to generate the necessary serialization code:
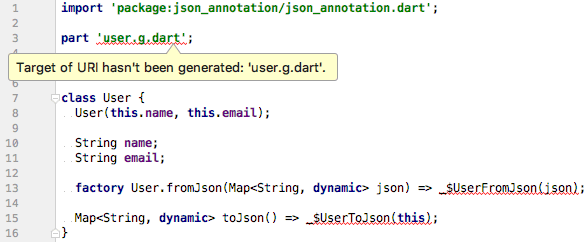
flutter pub run build_runner build
This generates the user.g.dart
file with the User.fromJson
and User.toJson
methods implemented automatically.
Consuming json_serializable
Models
Once the code is generated, you can use your model classes just as you would with manual serialization. Here’s how you can consume the User
class:
void main() {
String jsonString = '{"name": "John", "age": 30}';
Map<String, dynamic> userMap = jsonDecode(jsonString);
// Use the generated User class
User user = User.fromJson(userMap);
print('User Name: ${user.name}');
}
Using code generation libraries json_serializable
streamlines the process of serializing JSON in larger Flutter apps. You don’t have to manually write boilerplate code for every model; instead, code generation handles it for you.
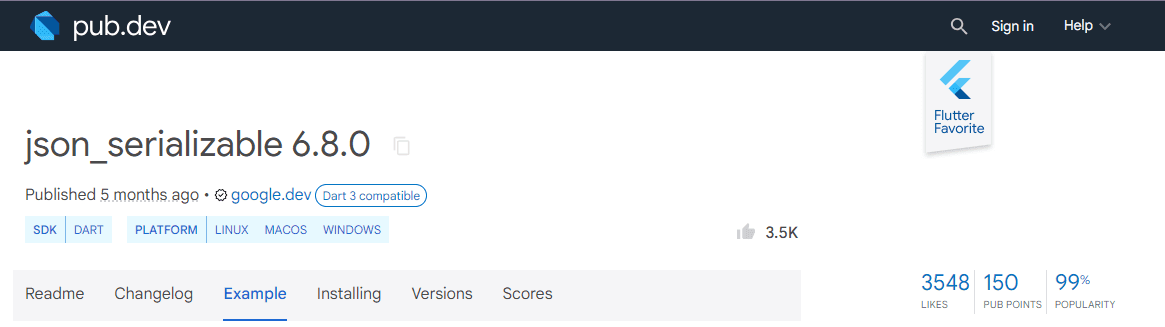
Setting Up json_serializable
in a Project
To set up json_serializable
, add the following dependencies to your pubspec.yaml
file:
dependencies:
json_annotation: ^4.0.1
dev_dependencies:
build_runner: ^2.0.0
json_serializable: ^4.0.0
Run flutter pub get
to install the dependencies.
Creating Model Classes the json_serializable
Annotate your model class with @JsonSerializable()
, and the library will generate a serialization code for you.
import 'package:json_annotation/json_annotation.dart';
part 'user.g.dart';
@JsonSerializable()
class User {
final String name;
final int age;
User({required this.name, required this.age});
factory User.fromJson(Map<String, dynamic> json) => _$UserFromJson(json);
Map<String, dynamic> toJson() => _$UserToJson(this);
}
Running the Code Generation Utility
Run the following command to generate the necessary serialization code:
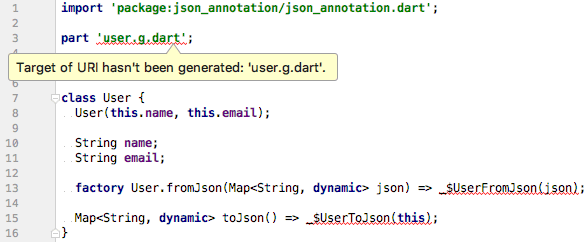
flutter pub run build_runner build
This generates the user.g.dart
file with the User.fromJson
and User.toJson
methods implemented automatically.
Consuming json_serializable
Models
Once the code is generated, you can use your model classes just as you would with manual serialization. Here’s how you can consume the User
class:
void main() {
String jsonString = '{"name": "John", "age": 30}';
Map<String, dynamic> userMap = jsonDecode(jsonString);
// Use the generated User class
User user = User.fromJson(userMap);
print('User Name: ${user.name}');
}
Generating Code for Nested Classes
Generating Code for Nested Classes
Generating Code for Nested Classes
Generating Code for Nested Classes
json_serializable
also supports nested classes. For instance, if you have a User
class that contains a Address
class, json_serializable
will generate the necessary serialization code for both, making Flutter development easier.
@JsonSerializable()
class Address {
final String city;
final String country;
Address({required this.city, required this.country});
factory Address.fromJson(Map<String, dynamic> json) => _$AddressFromJson(json);
Map<String, dynamic> toJson() => _$AddressToJson(this);
}
@JsonSerializable()
class User {
final String name;
final Address address;
User({required this.name, required this.address});
factory User.fromJson(Map<String, dynamic> json) => _$UserFromJson(json);
Map<String, dynamic> toJson() => _$UserToJson(this);
}
After running the code generation tool, both the User
and Address
classes will have their serialization code generated. This is particularly useful when dealing with complex models in Flutter backend communication.
For more information, see explicitToJson
in the JsonSerializable
class for the json_annotation
package.
json_serializable
also supports nested classes. For instance, if you have a User
class that contains a Address
class, json_serializable
will generate the necessary serialization code for both, making Flutter development easier.
@JsonSerializable()
class Address {
final String city;
final String country;
Address({required this.city, required this.country});
factory Address.fromJson(Map<String, dynamic> json) => _$AddressFromJson(json);
Map<String, dynamic> toJson() => _$AddressToJson(this);
}
@JsonSerializable()
class User {
final String name;
final Address address;
User({required this.name, required this.address});
factory User.fromJson(Map<String, dynamic> json) => _$UserFromJson(json);
Map<String, dynamic> toJson() => _$UserToJson(this);
}
After running the code generation tool, both the User
and Address
classes will have their serialization code generated. This is particularly useful when dealing with complex models in Flutter backend communication.
For more information, see explicitToJson
in the JsonSerializable
class for the json_annotation
package.
json_serializable
also supports nested classes. For instance, if you have a User
class that contains a Address
class, json_serializable
will generate the necessary serialization code for both, making Flutter development easier.
@JsonSerializable()
class Address {
final String city;
final String country;
Address({required this.city, required this.country});
factory Address.fromJson(Map<String, dynamic> json) => _$AddressFromJson(json);
Map<String, dynamic> toJson() => _$AddressToJson(this);
}
@JsonSerializable()
class User {
final String name;
final Address address;
User({required this.name, required this.address});
factory User.fromJson(Map<String, dynamic> json) => _$UserFromJson(json);
Map<String, dynamic> toJson() => _$UserToJson(this);
}
After running the code generation tool, both the User
and Address
classes will have their serialization code generated. This is particularly useful when dealing with complex models in Flutter backend communication.
For more information, see explicitToJson
in the JsonSerializable
class for the json_annotation
package.
json_serializable
also supports nested classes. For instance, if you have a User
class that contains a Address
class, json_serializable
will generate the necessary serialization code for both, making Flutter development easier.
@JsonSerializable()
class Address {
final String city;
final String country;
Address({required this.city, required this.country});
factory Address.fromJson(Map<String, dynamic> json) => _$AddressFromJson(json);
Map<String, dynamic> toJson() => _$AddressToJson(this);
}
@JsonSerializable()
class User {
final String name;
final Address address;
User({required this.name, required this.address});
factory User.fromJson(Map<String, dynamic> json) => _$UserFromJson(json);
Map<String, dynamic> toJson() => _$UserToJson(this);
}
After running the code generation tool, both the User
and Address
classes will have their serialization code generated. This is particularly useful when dealing with complex models in Flutter backend communication.
For more information, see explicitToJson
in the JsonSerializable
class for the json_annotation
package.
Further References
Further References
Further References
Further References
For more details on JSON serialization in Flutter, visit the official documentation here: Flutter JSON and Serialization.
The
dart:convert
andJsonCodec
documentationThe
json_serializable
package on pub.devThe
json_serializable
examples on GitHubThe Dive into Dart's patterns and records code lab
This ultimate guide about how to parse JSON in Dart/Flutter
For more details on JSON serialization in Flutter, visit the official documentation here: Flutter JSON and Serialization.
The
dart:convert
andJsonCodec
documentationThe
json_serializable
package on pub.devThe
json_serializable
examples on GitHubThe Dive into Dart's patterns and records code lab
This ultimate guide about how to parse JSON in Dart/Flutter
For more details on JSON serialization in Flutter, visit the official documentation here: Flutter JSON and Serialization.
The
dart:convert
andJsonCodec
documentationThe
json_serializable
package on pub.devThe
json_serializable
examples on GitHubThe Dive into Dart's patterns and records code lab
This ultimate guide about how to parse JSON in Dart/Flutter
For more details on JSON serialization in Flutter, visit the official documentation here: Flutter JSON and Serialization.
The
dart:convert
andJsonCodec
documentationThe
json_serializable
package on pub.devThe
json_serializable
examples on GitHubThe Dive into Dart's patterns and records code lab
This ultimate guide about how to parse JSON in Dart/Flutter
conclusion
conclusion
conclusion
conclusion
In conclusion, whether you're working on a small Flutter app or a complex mobile app development project, choosing the right JSON serialization method is critical. For simpler projects, manual serialization using dart:convert
is a viable option. However, for medium to large-scale projects, leveraging code generation tools like json_serializable
can save time and reduce boilerplate code, allowing you to focus more on Flutter UI and Flutter widgets.
By efficiently handling JSON serialization, you can build dynamic, data-driven Flutter apps that deliver seamless user experiences. To learn more about Flutter app development, Flutter widgets, and Flutter backend integration, continue exploring our Flutter development resources!
In conclusion, whether you're working on a small Flutter app or a complex mobile app development project, choosing the right JSON serialization method is critical. For simpler projects, manual serialization using dart:convert
is a viable option. However, for medium to large-scale projects, leveraging code generation tools like json_serializable
can save time and reduce boilerplate code, allowing you to focus more on Flutter UI and Flutter widgets.
By efficiently handling JSON serialization, you can build dynamic, data-driven Flutter apps that deliver seamless user experiences. To learn more about Flutter app development, Flutter widgets, and Flutter backend integration, continue exploring our Flutter development resources!
In conclusion, whether you're working on a small Flutter app or a complex mobile app development project, choosing the right JSON serialization method is critical. For simpler projects, manual serialization using dart:convert
is a viable option. However, for medium to large-scale projects, leveraging code generation tools like json_serializable
can save time and reduce boilerplate code, allowing you to focus more on Flutter UI and Flutter widgets.
By efficiently handling JSON serialization, you can build dynamic, data-driven Flutter apps that deliver seamless user experiences. To learn more about Flutter app development, Flutter widgets, and Flutter backend integration, continue exploring our Flutter development resources!
In conclusion, whether you're working on a small Flutter app or a complex mobile app development project, choosing the right JSON serialization method is critical. For simpler projects, manual serialization using dart:convert
is a viable option. However, for medium to large-scale projects, leveraging code generation tools like json_serializable
can save time and reduce boilerplate code, allowing you to focus more on Flutter UI and Flutter widgets.
By efficiently handling JSON serialization, you can build dynamic, data-driven Flutter apps that deliver seamless user experiences. To learn more about Flutter app development, Flutter widgets, and Flutter backend integration, continue exploring our Flutter development resources!
Table of content
India(HQ)
9th Floor, Tower C, Logix Cyber Park,
C Block, Phase 2, Industrial Area, Sector 62, Noida, Uttar Pradesh, 201309
USA
2081 Center Street Berkeley,
CA 94704
© 2021-23 Blupx Private Limited.
All rights reserved.
India(HQ)
9th Floor, Tower C, Logix Cyber Park,
C Block, Phase 2, Industrial Area, Sector 62, Noida, Uttar Pradesh, 201309
USA
2081 Center Street Berkeley,
CA 94704
© 2021-23 Blupx Private Limited.
All rights reserved.
India(HQ)
9th Floor, Tower C, Logix Cyber Park,
C Block, Phase 2, Industrial Area, Sector 62, Noida, Uttar Pradesh, 201309
USA
2081 Center Street Berkeley,
CA 94704
© 2021-23 Blupx Private Limited.
All rights reserved.