Firebase Flutter Tutorial
5 mins
5 mins

Ashutosh
Published on Nov 9, 2024
Firestore Real-Time Data and Offline Capabilities
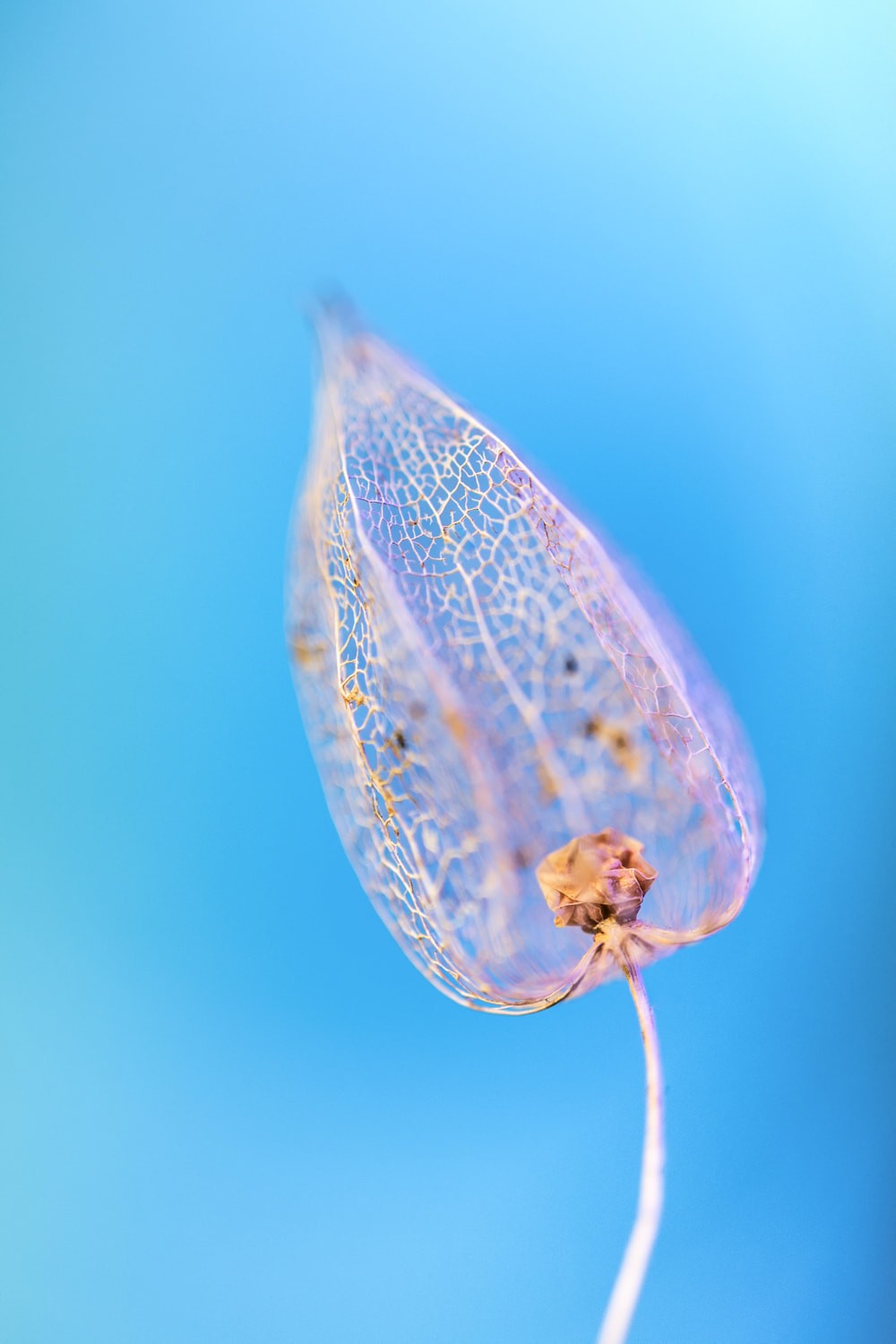
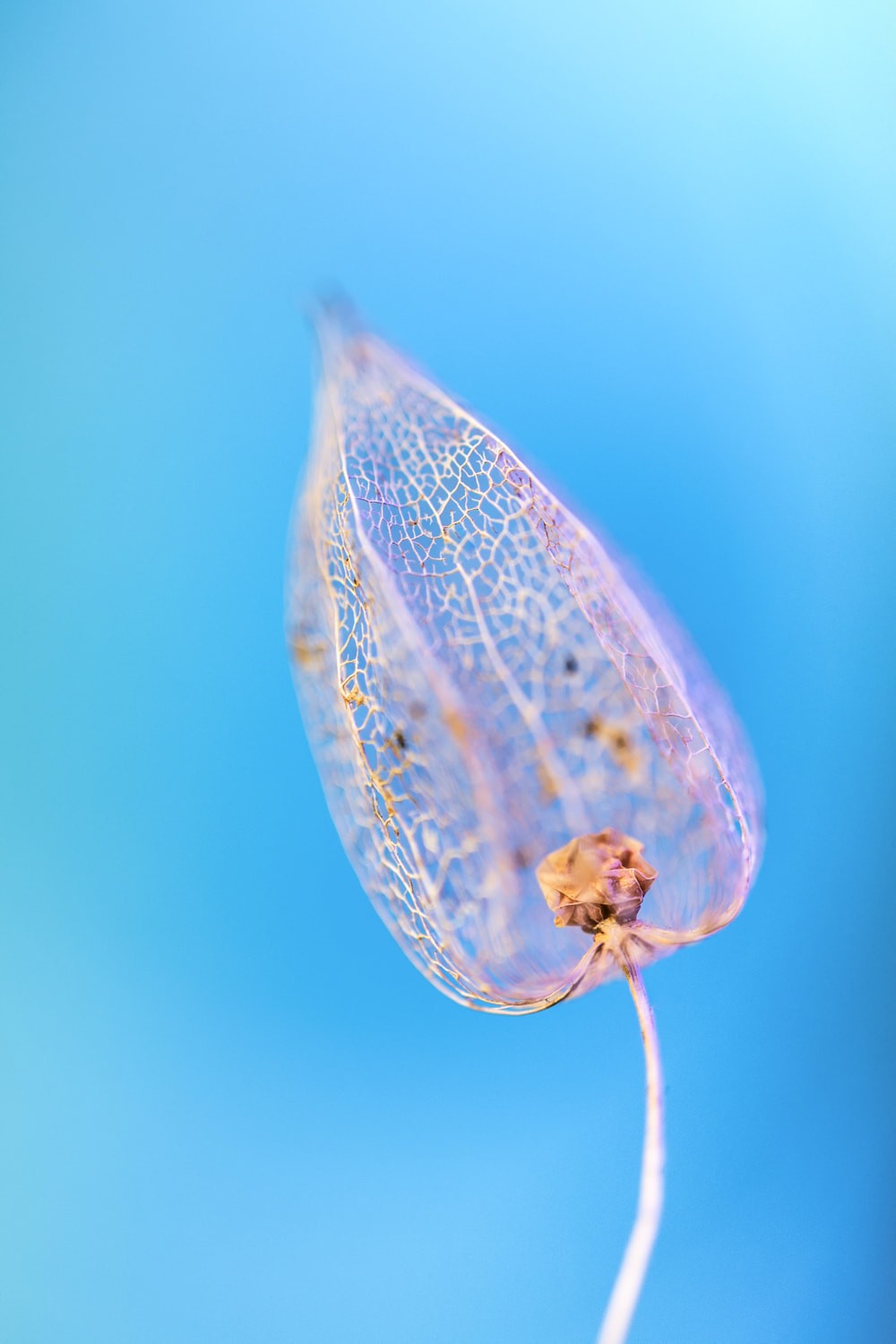
Introduction
Introduction
Introduction
Introduction
In the previous blog posts, we covered the essentials of Firebase and Firestore integration with Flutter. If you missed them, check out our introductory guide on Getting Started with Firebase for Flutter Development and our in-depth tutorial on Firestore Basics: Storing and Retrieving Data in Flutter. Today, we're diving deeper into Firestore’s real-time data and offline capabilities, crucial for creating dynamic, resilient Flutter apps that offer a seamless user experience.
Firestore’s real-time data updates and offline capabilities can be transformative. Real-time listeners keep your app data current without user intervention, and offline support ensures uninterrupted access to data, even without an internet connection. Let’s break down how these features work in Firestore and explore how to implement them in your Flutter app.
In the previous blog posts, we covered the essentials of Firebase and Firestore integration with Flutter. If you missed them, check out our introductory guide on Getting Started with Firebase for Flutter Development and our in-depth tutorial on Firestore Basics: Storing and Retrieving Data in Flutter. Today, we're diving deeper into Firestore’s real-time data and offline capabilities, crucial for creating dynamic, resilient Flutter apps that offer a seamless user experience.
Firestore’s real-time data updates and offline capabilities can be transformative. Real-time listeners keep your app data current without user intervention, and offline support ensures uninterrupted access to data, even without an internet connection. Let’s break down how these features work in Firestore and explore how to implement them in your Flutter app.
In the previous blog posts, we covered the essentials of Firebase and Firestore integration with Flutter. If you missed them, check out our introductory guide on Getting Started with Firebase for Flutter Development and our in-depth tutorial on Firestore Basics: Storing and Retrieving Data in Flutter. Today, we're diving deeper into Firestore’s real-time data and offline capabilities, crucial for creating dynamic, resilient Flutter apps that offer a seamless user experience.
Firestore’s real-time data updates and offline capabilities can be transformative. Real-time listeners keep your app data current without user intervention, and offline support ensures uninterrupted access to data, even without an internet connection. Let’s break down how these features work in Firestore and explore how to implement them in your Flutter app.
In the previous blog posts, we covered the essentials of Firebase and Firestore integration with Flutter. If you missed them, check out our introductory guide on Getting Started with Firebase for Flutter Development and our in-depth tutorial on Firestore Basics: Storing and Retrieving Data in Flutter. Today, we're diving deeper into Firestore’s real-time data and offline capabilities, crucial for creating dynamic, resilient Flutter apps that offer a seamless user experience.
Firestore’s real-time data updates and offline capabilities can be transformative. Real-time listeners keep your app data current without user intervention, and offline support ensures uninterrupted access to data, even without an internet connection. Let’s break down how these features work in Firestore and explore how to implement them in your Flutter app.
Real-Time Data and Offline Capabilities
Real-Time Data and Offline Capabilities
Real-Time Data and Offline Capabilities
Real-Time Data and Offline Capabilities
Why Real-Time Data and Offline Capabilities Matter in Mobile Apps
In modern mobile applications, users expect data to be always available and instantly updated. Real-time updates and offline functionality are particularly important for apps where:
Timeliness of data is critical (e.g., messaging, social media).
Offline usage is necessary due to inconsistent network availability.
User retention depends on seamless experience regardless of connectivity.
Firestore enables these features by providing real-time listeners and automatic offline data synchronization. By using Firestore’s SDK with Flutter, you can develop apps that sync and update data efficiently, offering a user-friendly experience regardless of network availability.
Why Real-Time Data and Offline Capabilities Matter in Mobile Apps
In modern mobile applications, users expect data to be always available and instantly updated. Real-time updates and offline functionality are particularly important for apps where:
Timeliness of data is critical (e.g., messaging, social media).
Offline usage is necessary due to inconsistent network availability.
User retention depends on seamless experience regardless of connectivity.
Firestore enables these features by providing real-time listeners and automatic offline data synchronization. By using Firestore’s SDK with Flutter, you can develop apps that sync and update data efficiently, offering a user-friendly experience regardless of network availability.
Why Real-Time Data and Offline Capabilities Matter in Mobile Apps
In modern mobile applications, users expect data to be always available and instantly updated. Real-time updates and offline functionality are particularly important for apps where:
Timeliness of data is critical (e.g., messaging, social media).
Offline usage is necessary due to inconsistent network availability.
User retention depends on seamless experience regardless of connectivity.
Firestore enables these features by providing real-time listeners and automatic offline data synchronization. By using Firestore’s SDK with Flutter, you can develop apps that sync and update data efficiently, offering a user-friendly experience regardless of network availability.
Why Real-Time Data and Offline Capabilities Matter in Mobile Apps
In modern mobile applications, users expect data to be always available and instantly updated. Real-time updates and offline functionality are particularly important for apps where:
Timeliness of data is critical (e.g., messaging, social media).
Offline usage is necessary due to inconsistent network availability.
User retention depends on seamless experience regardless of connectivity.
Firestore enables these features by providing real-time listeners and automatic offline data synchronization. By using Firestore’s SDK with Flutter, you can develop apps that sync and update data efficiently, offering a user-friendly experience regardless of network availability.
Implementing Real-Time Data with Firestore in Flutter
Implementing Real-Time Data with Firestore in Flutter
Implementing Real-Time Data with Firestore in Flutter
Implementing Real-Time Data with Firestore in Flutter
Firestore’s real-time data feature allows you to listen to changes in documents and collections. Instead of manually checking for updates, you can set up listeners that automatically trigger whenever data changes, allowing you to update your UI instantly.
1. Setting Up a Real-Time Listener
To implement a real-time listener, you need to create a snapshot listener on a document or collection. Here's a quick example:
In this example, any changes to the document with ID user_id
in the users
collection will trigger the listener, giving you the latest data instantly.
2. Real-Time Listeners for Collections
If you need real-time updates for multiple documents, such as all documents in a collection, set up a listener for the entire collection:
This approach ensures you have updated data for all users in the collection whenever any document changes, new documents are added, or documents are deleted.
Optimizing Real-Time Data for Performance
While real-time listeners are powerful, they can increase network usage and battery consumption. Firestore optimizes this by only fetching incremental updates, but here are a few tips to manage performance:
Use Listeners Only When Necessary: Only use listeners for critical data that requires real-time updates.
Limit Query Scope: Query only the data you need to minimize the data Firestore has to retrieve and manage.
Unsubscribe When Not Needed: Make sure to unsubscribe from listeners when they’re no longer necessary, like when leaving a screen.
Firestore’s real-time data feature allows you to listen to changes in documents and collections. Instead of manually checking for updates, you can set up listeners that automatically trigger whenever data changes, allowing you to update your UI instantly.
1. Setting Up a Real-Time Listener
To implement a real-time listener, you need to create a snapshot listener on a document or collection. Here's a quick example:
In this example, any changes to the document with ID user_id
in the users
collection will trigger the listener, giving you the latest data instantly.
2. Real-Time Listeners for Collections
If you need real-time updates for multiple documents, such as all documents in a collection, set up a listener for the entire collection:
This approach ensures you have updated data for all users in the collection whenever any document changes, new documents are added, or documents are deleted.
Optimizing Real-Time Data for Performance
While real-time listeners are powerful, they can increase network usage and battery consumption. Firestore optimizes this by only fetching incremental updates, but here are a few tips to manage performance:
Use Listeners Only When Necessary: Only use listeners for critical data that requires real-time updates.
Limit Query Scope: Query only the data you need to minimize the data Firestore has to retrieve and manage.
Unsubscribe When Not Needed: Make sure to unsubscribe from listeners when they’re no longer necessary, like when leaving a screen.
Firestore’s real-time data feature allows you to listen to changes in documents and collections. Instead of manually checking for updates, you can set up listeners that automatically trigger whenever data changes, allowing you to update your UI instantly.
1. Setting Up a Real-Time Listener
To implement a real-time listener, you need to create a snapshot listener on a document or collection. Here's a quick example:
In this example, any changes to the document with ID user_id
in the users
collection will trigger the listener, giving you the latest data instantly.
2. Real-Time Listeners for Collections
If you need real-time updates for multiple documents, such as all documents in a collection, set up a listener for the entire collection:
This approach ensures you have updated data for all users in the collection whenever any document changes, new documents are added, or documents are deleted.
Optimizing Real-Time Data for Performance
While real-time listeners are powerful, they can increase network usage and battery consumption. Firestore optimizes this by only fetching incremental updates, but here are a few tips to manage performance:
Use Listeners Only When Necessary: Only use listeners for critical data that requires real-time updates.
Limit Query Scope: Query only the data you need to minimize the data Firestore has to retrieve and manage.
Unsubscribe When Not Needed: Make sure to unsubscribe from listeners when they’re no longer necessary, like when leaving a screen.
Firestore’s real-time data feature allows you to listen to changes in documents and collections. Instead of manually checking for updates, you can set up listeners that automatically trigger whenever data changes, allowing you to update your UI instantly.
1. Setting Up a Real-Time Listener
To implement a real-time listener, you need to create a snapshot listener on a document or collection. Here's a quick example:
In this example, any changes to the document with ID user_id
in the users
collection will trigger the listener, giving you the latest data instantly.
2. Real-Time Listeners for Collections
If you need real-time updates for multiple documents, such as all documents in a collection, set up a listener for the entire collection:
This approach ensures you have updated data for all users in the collection whenever any document changes, new documents are added, or documents are deleted.
Optimizing Real-Time Data for Performance
While real-time listeners are powerful, they can increase network usage and battery consumption. Firestore optimizes this by only fetching incremental updates, but here are a few tips to manage performance:
Use Listeners Only When Necessary: Only use listeners for critical data that requires real-time updates.
Limit Query Scope: Query only the data you need to minimize the data Firestore has to retrieve and manage.
Unsubscribe When Not Needed: Make sure to unsubscribe from listeners when they’re no longer necessary, like when leaving a screen.
Firestore Offline Capabilities
Firestore Offline Capabilities
Firestore Offline Capabilities
Firestore Offline Capabilities
Firestore Offline Capabilities: Seamless Data Access Without Internet
Firestore’s offline capabilities allow you to cache data locally, so users can access it even without an internet connection. This caching not only enables offline functionality but also speeds up the app by reducing redundant network requests.
1. How Offline Persistence Works
Firestore automatically caches data that your app reads or writes. When the app goes offline, Firestore continues to serve data from this cache, making it available to the user. Once the app is back online, Firestore syncs any pending changes to the server.
By default, offline persistence is enabled on mobile devices but disabled on web. To ensure offline persistence is enabled, add this line in your initialization:
2. Handling Offline Scenarios with Firestore
Firestore provides the ability to differentiate between online and offline data states using isFromCache
in snapshots. Here’s how you can implement it:
The isFromCache
flag helps you detect when data is coming from the cache, so you can notify users about connectivity status if necessary.
Firestore Offline Capabilities: Seamless Data Access Without Internet
Firestore’s offline capabilities allow you to cache data locally, so users can access it even without an internet connection. This caching not only enables offline functionality but also speeds up the app by reducing redundant network requests.
1. How Offline Persistence Works
Firestore automatically caches data that your app reads or writes. When the app goes offline, Firestore continues to serve data from this cache, making it available to the user. Once the app is back online, Firestore syncs any pending changes to the server.
By default, offline persistence is enabled on mobile devices but disabled on web. To ensure offline persistence is enabled, add this line in your initialization:
2. Handling Offline Scenarios with Firestore
Firestore provides the ability to differentiate between online and offline data states using isFromCache
in snapshots. Here’s how you can implement it:
The isFromCache
flag helps you detect when data is coming from the cache, so you can notify users about connectivity status if necessary.
Firestore Offline Capabilities: Seamless Data Access Without Internet
Firestore’s offline capabilities allow you to cache data locally, so users can access it even without an internet connection. This caching not only enables offline functionality but also speeds up the app by reducing redundant network requests.
1. How Offline Persistence Works
Firestore automatically caches data that your app reads or writes. When the app goes offline, Firestore continues to serve data from this cache, making it available to the user. Once the app is back online, Firestore syncs any pending changes to the server.
By default, offline persistence is enabled on mobile devices but disabled on web. To ensure offline persistence is enabled, add this line in your initialization:
2. Handling Offline Scenarios with Firestore
Firestore provides the ability to differentiate between online and offline data states using isFromCache
in snapshots. Here’s how you can implement it:
The isFromCache
flag helps you detect when data is coming from the cache, so you can notify users about connectivity status if necessary.
Firestore Offline Capabilities: Seamless Data Access Without Internet
Firestore’s offline capabilities allow you to cache data locally, so users can access it even without an internet connection. This caching not only enables offline functionality but also speeds up the app by reducing redundant network requests.
1. How Offline Persistence Works
Firestore automatically caches data that your app reads or writes. When the app goes offline, Firestore continues to serve data from this cache, making it available to the user. Once the app is back online, Firestore syncs any pending changes to the server.
By default, offline persistence is enabled on mobile devices but disabled on web. To ensure offline persistence is enabled, add this line in your initialization:
2. Handling Offline Scenarios with Firestore
Firestore provides the ability to differentiate between online and offline data states using isFromCache
in snapshots. Here’s how you can implement it:
The isFromCache
flag helps you detect when data is coming from the cache, so you can notify users about connectivity status if necessary.
Firestore Data Sync Options in Flutter
Firestore Data Sync Options in Flutter
Firestore Data Sync Options in Flutter
Firestore Data Sync Options in Flutter
Firestore offers robust data sync options to handle different app scenarios, such as conflict resolution, data merging, and priority sync.
1. Conflict Resolution
In offline scenarios, conflicts may arise if multiple users modify the same data simultaneously. Firestore uses last-write-wins logic for resolving conflicts, ensuring the most recent change is applied when syncing.
2. Data Merging Options
For structured data updates, Firestore supports merging updates instead of overwriting documents. For example, if you want to update specific fields in a document without overwriting other fields, you can use the merge option:
By merging fields, you preserve existing data while updating only the necessary fields, which is ideal for an offline-first design where data syncs sporadically.
Firestore offers robust data sync options to handle different app scenarios, such as conflict resolution, data merging, and priority sync.
1. Conflict Resolution
In offline scenarios, conflicts may arise if multiple users modify the same data simultaneously. Firestore uses last-write-wins logic for resolving conflicts, ensuring the most recent change is applied when syncing.
2. Data Merging Options
For structured data updates, Firestore supports merging updates instead of overwriting documents. For example, if you want to update specific fields in a document without overwriting other fields, you can use the merge option:
By merging fields, you preserve existing data while updating only the necessary fields, which is ideal for an offline-first design where data syncs sporadically.
Firestore offers robust data sync options to handle different app scenarios, such as conflict resolution, data merging, and priority sync.
1. Conflict Resolution
In offline scenarios, conflicts may arise if multiple users modify the same data simultaneously. Firestore uses last-write-wins logic for resolving conflicts, ensuring the most recent change is applied when syncing.
2. Data Merging Options
For structured data updates, Firestore supports merging updates instead of overwriting documents. For example, if you want to update specific fields in a document without overwriting other fields, you can use the merge option:
By merging fields, you preserve existing data while updating only the necessary fields, which is ideal for an offline-first design where data syncs sporadically.
Firestore offers robust data sync options to handle different app scenarios, such as conflict resolution, data merging, and priority sync.
1. Conflict Resolution
In offline scenarios, conflicts may arise if multiple users modify the same data simultaneously. Firestore uses last-write-wins logic for resolving conflicts, ensuring the most recent change is applied when syncing.
2. Data Merging Options
For structured data updates, Firestore supports merging updates instead of overwriting documents. For example, if you want to update specific fields in a document without overwriting other fields, you can use the merge option:
By merging fields, you preserve existing data while updating only the necessary fields, which is ideal for an offline-first design where data syncs sporadically.
Practical Use Case
Practical Use Case
Practical Use Case
Practical Use Case
Practical Use Case: Building a Chat App with Firestore
A common application of Firestore’s real-time and offline features is in chat apps. Here’s a simplified approach to using Firestore for a chat app:
Real-Time Chat Updates: Set up real-time listeners on chat messages. As users send messages, listeners update the chat interface instantly.
Offline Messaging: When offline, users can still send messages. These messages are saved locally and sync with Firestore once the app reconnects.
Handling Conflicts: If two users edit the same message offline, Firestore’s last-write-wins rule ensures data integrity on reconnection.
By implementing Firestore’s features, you create a chat app that’s responsive and functional, whether users are online or offline.
FAQs on Firestore Real-Time Data and Offline Capabilities
Q1: How can I disable offline persistence in Firestore?
A: You can disable offline persistence by setting the persistence-enabled property to false:
Q2: Does Firestore guarantee real-time data consistency?
A: Firestore is designed for strong data consistency, but there may be slight delays in data propagation across regions in rare cases. For most apps, Firestore's real-time data handling is reliable enough.
Q3: Can I limit the size of offline data stored by Firestore?
A: Yes, Firestore provides an option to set cache size limits to manage storage:
Q4: What happens to unsynced data when a user uninstalls the app?
A: If the app is uninstalled, any data not yet synced to the server will be lost. Ensuring regular syncs for critical data minimizes this risk.
Q5: Is offline persistence available for Firestore on the web?
A: Firestore’s offline persistence is available on mobile platforms by default. For the web, offline persistence must be enabled explicitly, and there may be limitations compared to mobile.
Q6: How does Firestore handle offline data conflicts?
A: Firestore uses the last-write-wins method for resolving conflicts. When a conflict arises, the most recent write will overwrite previous data upon synchronization.
Practical Use Case: Building a Chat App with Firestore
A common application of Firestore’s real-time and offline features is in chat apps. Here’s a simplified approach to using Firestore for a chat app:
Real-Time Chat Updates: Set up real-time listeners on chat messages. As users send messages, listeners update the chat interface instantly.
Offline Messaging: When offline, users can still send messages. These messages are saved locally and sync with Firestore once the app reconnects.
Handling Conflicts: If two users edit the same message offline, Firestore’s last-write-wins rule ensures data integrity on reconnection.
By implementing Firestore’s features, you create a chat app that’s responsive and functional, whether users are online or offline.
FAQs on Firestore Real-Time Data and Offline Capabilities
Q1: How can I disable offline persistence in Firestore?
A: You can disable offline persistence by setting the persistence-enabled property to false:
Q2: Does Firestore guarantee real-time data consistency?
A: Firestore is designed for strong data consistency, but there may be slight delays in data propagation across regions in rare cases. For most apps, Firestore's real-time data handling is reliable enough.
Q3: Can I limit the size of offline data stored by Firestore?
A: Yes, Firestore provides an option to set cache size limits to manage storage:
Q4: What happens to unsynced data when a user uninstalls the app?
A: If the app is uninstalled, any data not yet synced to the server will be lost. Ensuring regular syncs for critical data minimizes this risk.
Q5: Is offline persistence available for Firestore on the web?
A: Firestore’s offline persistence is available on mobile platforms by default. For the web, offline persistence must be enabled explicitly, and there may be limitations compared to mobile.
Q6: How does Firestore handle offline data conflicts?
A: Firestore uses the last-write-wins method for resolving conflicts. When a conflict arises, the most recent write will overwrite previous data upon synchronization.
Practical Use Case: Building a Chat App with Firestore
A common application of Firestore’s real-time and offline features is in chat apps. Here’s a simplified approach to using Firestore for a chat app:
Real-Time Chat Updates: Set up real-time listeners on chat messages. As users send messages, listeners update the chat interface instantly.
Offline Messaging: When offline, users can still send messages. These messages are saved locally and sync with Firestore once the app reconnects.
Handling Conflicts: If two users edit the same message offline, Firestore’s last-write-wins rule ensures data integrity on reconnection.
By implementing Firestore’s features, you create a chat app that’s responsive and functional, whether users are online or offline.
FAQs on Firestore Real-Time Data and Offline Capabilities
Q1: How can I disable offline persistence in Firestore?
A: You can disable offline persistence by setting the persistence-enabled property to false:
Q2: Does Firestore guarantee real-time data consistency?
A: Firestore is designed for strong data consistency, but there may be slight delays in data propagation across regions in rare cases. For most apps, Firestore's real-time data handling is reliable enough.
Q3: Can I limit the size of offline data stored by Firestore?
A: Yes, Firestore provides an option to set cache size limits to manage storage:
Q4: What happens to unsynced data when a user uninstalls the app?
A: If the app is uninstalled, any data not yet synced to the server will be lost. Ensuring regular syncs for critical data minimizes this risk.
Q5: Is offline persistence available for Firestore on the web?
A: Firestore’s offline persistence is available on mobile platforms by default. For the web, offline persistence must be enabled explicitly, and there may be limitations compared to mobile.
Q6: How does Firestore handle offline data conflicts?
A: Firestore uses the last-write-wins method for resolving conflicts. When a conflict arises, the most recent write will overwrite previous data upon synchronization.
Practical Use Case: Building a Chat App with Firestore
A common application of Firestore’s real-time and offline features is in chat apps. Here’s a simplified approach to using Firestore for a chat app:
Real-Time Chat Updates: Set up real-time listeners on chat messages. As users send messages, listeners update the chat interface instantly.
Offline Messaging: When offline, users can still send messages. These messages are saved locally and sync with Firestore once the app reconnects.
Handling Conflicts: If two users edit the same message offline, Firestore’s last-write-wins rule ensures data integrity on reconnection.
By implementing Firestore’s features, you create a chat app that’s responsive and functional, whether users are online or offline.
FAQs on Firestore Real-Time Data and Offline Capabilities
Q1: How can I disable offline persistence in Firestore?
A: You can disable offline persistence by setting the persistence-enabled property to false:
Q2: Does Firestore guarantee real-time data consistency?
A: Firestore is designed for strong data consistency, but there may be slight delays in data propagation across regions in rare cases. For most apps, Firestore's real-time data handling is reliable enough.
Q3: Can I limit the size of offline data stored by Firestore?
A: Yes, Firestore provides an option to set cache size limits to manage storage:
Q4: What happens to unsynced data when a user uninstalls the app?
A: If the app is uninstalled, any data not yet synced to the server will be lost. Ensuring regular syncs for critical data minimizes this risk.
Q5: Is offline persistence available for Firestore on the web?
A: Firestore’s offline persistence is available on mobile platforms by default. For the web, offline persistence must be enabled explicitly, and there may be limitations compared to mobile.
Q6: How does Firestore handle offline data conflicts?
A: Firestore uses the last-write-wins method for resolving conflicts. When a conflict arises, the most recent write will overwrite previous data upon synchronization.
Conclusion
Conclusion
Conclusion
Conclusion
Firestore’s real-time and offline capabilities bring exceptional value to Flutter apps, enhancing user experience with instant updates and reliable offline access. By implementing real-time listeners and offline data handling in your app, you can build a resilient, user-friendly experience that keeps users engaged.
Whether you’re building a chat app, social feed, or any dynamic application, these features provide the foundation for an interactive and responsive app. To further explore Firestore in Flutter, revisit our guides on Getting Started with Firebase for Flutter Development and Firestore Basics: Storing and Retrieving Data in Flutter for additional context. Dive into Firestore’s real-time data and offline sync capabilities today to give your Flutter app a robust, modern edge.
Firestore’s real-time and offline capabilities bring exceptional value to Flutter apps, enhancing user experience with instant updates and reliable offline access. By implementing real-time listeners and offline data handling in your app, you can build a resilient, user-friendly experience that keeps users engaged.
Whether you’re building a chat app, social feed, or any dynamic application, these features provide the foundation for an interactive and responsive app. To further explore Firestore in Flutter, revisit our guides on Getting Started with Firebase for Flutter Development and Firestore Basics: Storing and Retrieving Data in Flutter for additional context. Dive into Firestore’s real-time data and offline sync capabilities today to give your Flutter app a robust, modern edge.
Firestore’s real-time and offline capabilities bring exceptional value to Flutter apps, enhancing user experience with instant updates and reliable offline access. By implementing real-time listeners and offline data handling in your app, you can build a resilient, user-friendly experience that keeps users engaged.
Whether you’re building a chat app, social feed, or any dynamic application, these features provide the foundation for an interactive and responsive app. To further explore Firestore in Flutter, revisit our guides on Getting Started with Firebase for Flutter Development and Firestore Basics: Storing and Retrieving Data in Flutter for additional context. Dive into Firestore’s real-time data and offline sync capabilities today to give your Flutter app a robust, modern edge.
Firestore’s real-time and offline capabilities bring exceptional value to Flutter apps, enhancing user experience with instant updates and reliable offline access. By implementing real-time listeners and offline data handling in your app, you can build a resilient, user-friendly experience that keeps users engaged.
Whether you’re building a chat app, social feed, or any dynamic application, these features provide the foundation for an interactive and responsive app. To further explore Firestore in Flutter, revisit our guides on Getting Started with Firebase for Flutter Development and Firestore Basics: Storing and Retrieving Data in Flutter for additional context. Dive into Firestore’s real-time data and offline sync capabilities today to give your Flutter app a robust, modern edge.
Table of content
India(HQ)
9th Floor, Tower C, Logix Cyber Park,
C Block, Phase 2, Industrial Area, Sector 62, Noida, Uttar Pradesh, 201309
USA
2081 Center Street Berkeley,
CA 94704
© 2021-23 Blupx Private Limited.
All rights reserved.
India(HQ)
9th Floor, Tower C, Logix Cyber Park,
C Block, Phase 2, Industrial Area, Sector 62, Noida, Uttar Pradesh, 201309
USA
2081 Center Street Berkeley,
CA 94704
© 2021-23 Blupx Private Limited.
All rights reserved.
India(HQ)
9th Floor, Tower C, Logix Cyber Park,
C Block, Phase 2, Industrial Area, Sector 62, Noida, Uttar Pradesh, 201309
USA
2081 Center Street Berkeley,
CA 94704
© 2021-23 Blupx Private Limited.
All rights reserved.