Firebase Flutter tutorial
6 mins
6 mins

Ashutosh
Published on Nov 6, 2024
Getting Started with Firebase for Flutter Development
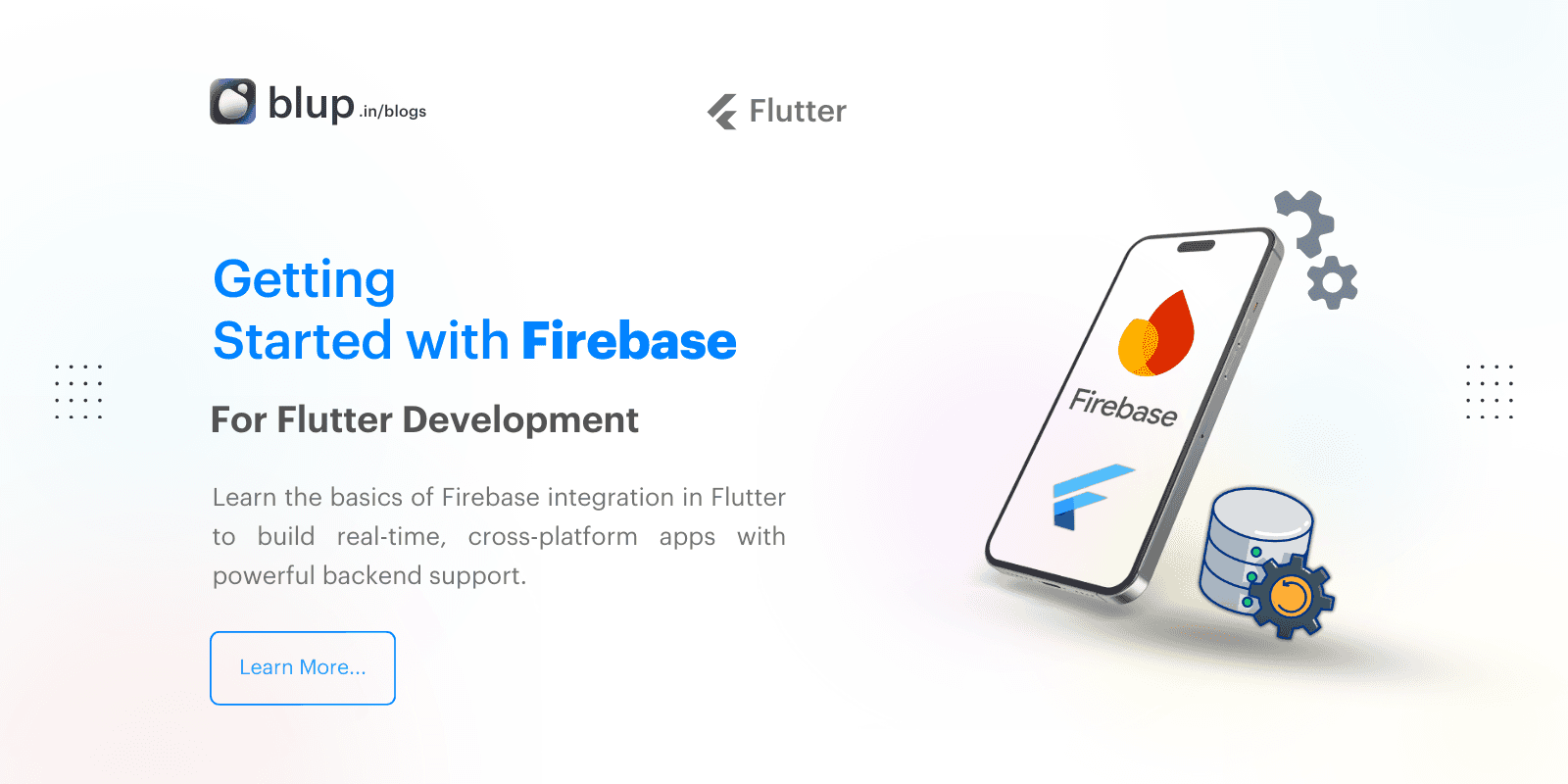
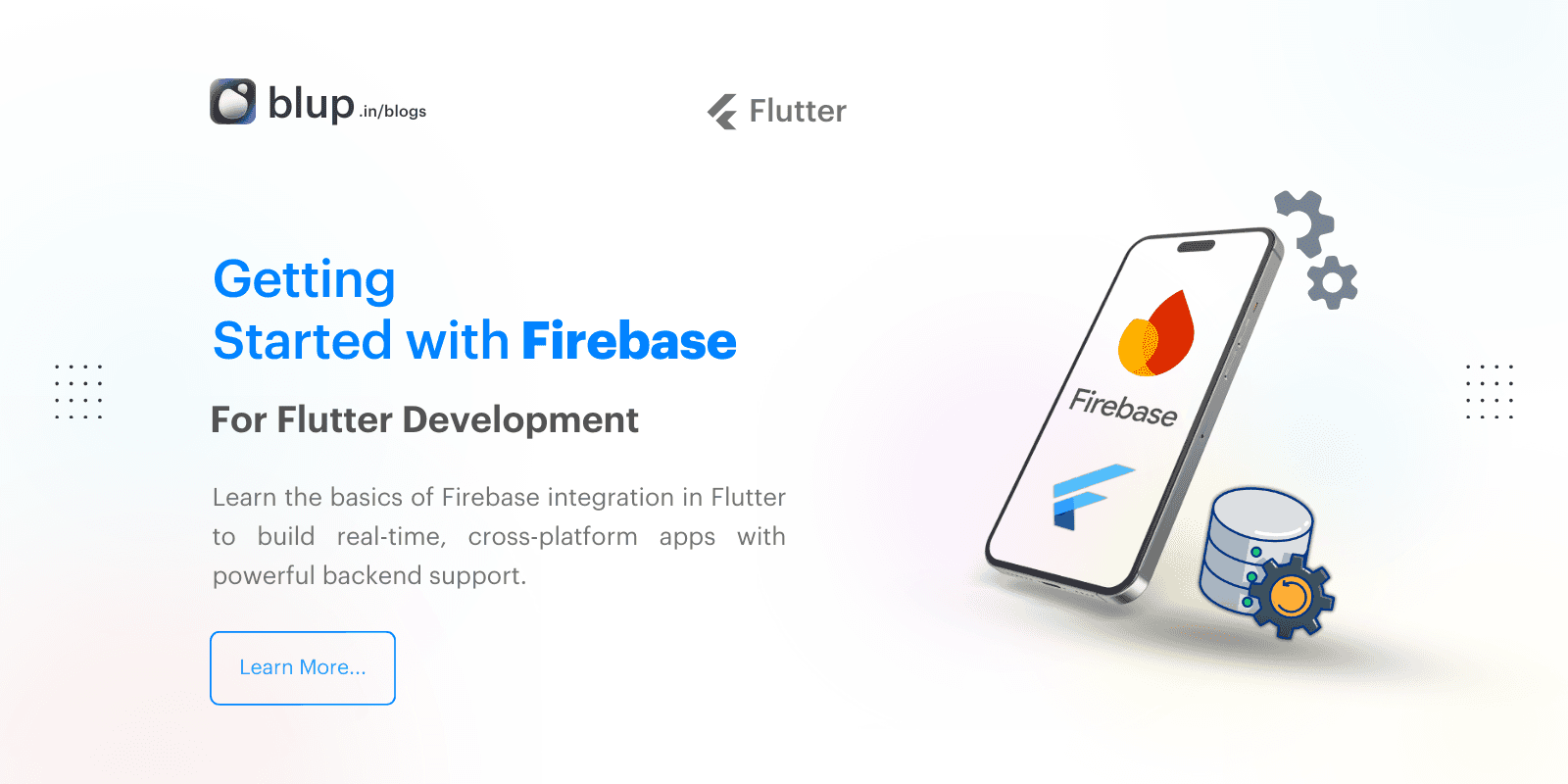
Introduction
Introduction
Introduction
Introduction
Firebase is a platform developed by Google that provides essential tools to create high-quality, scalable mobile and web applications. For Flutter developers, Firebase offers a suite of cloud-based tools to seamlessly manage backend services such as authentication, databases, analytics, and real-time notifications. In this guide, we’ll cover how to integrate Firebase with Flutter, explore its fundamental components, and explain why Firebase can significantly enhance your app’s performance and reliability.
Firebase is a platform developed by Google that provides essential tools to create high-quality, scalable mobile and web applications. For Flutter developers, Firebase offers a suite of cloud-based tools to seamlessly manage backend services such as authentication, databases, analytics, and real-time notifications. In this guide, we’ll cover how to integrate Firebase with Flutter, explore its fundamental components, and explain why Firebase can significantly enhance your app’s performance and reliability.
Firebase is a platform developed by Google that provides essential tools to create high-quality, scalable mobile and web applications. For Flutter developers, Firebase offers a suite of cloud-based tools to seamlessly manage backend services such as authentication, databases, analytics, and real-time notifications. In this guide, we’ll cover how to integrate Firebase with Flutter, explore its fundamental components, and explain why Firebase can significantly enhance your app’s performance and reliability.
Firebase is a platform developed by Google that provides essential tools to create high-quality, scalable mobile and web applications. For Flutter developers, Firebase offers a suite of cloud-based tools to seamlessly manage backend services such as authentication, databases, analytics, and real-time notifications. In this guide, we’ll cover how to integrate Firebase with Flutter, explore its fundamental components, and explain why Firebase can significantly enhance your app’s performance and reliability.
What is Firebase and Why Use It with Flutter?
What is Firebase and Why Use It with Flutter?
What is Firebase and Why Use It with Flutter?
What is Firebase and Why Use It with Flutter?
Firebase simplifies backend development by offering various services that Flutter apps can use right out of the box. Here are some of the key features:

Authentication: Streamlines integrating user authentication, including Google, Facebook, and email logins.
Cloud Firestore: A real-time NoSQL database that allows data synchronization and offline support.
Firebase Analytics: Provides deep insights into user behavior, helping developers improve user experience and engagement.
Crashlytics: Monitors app stability and reports crashes for proactive debugging.
For Flutter developers, Firebase provides easy integration, enabling seamless cross-platform support and consistent performance across iOS, Android, and the web.
Firebase simplifies backend development by offering various services that Flutter apps can use right out of the box. Here are some of the key features:

Authentication: Streamlines integrating user authentication, including Google, Facebook, and email logins.
Cloud Firestore: A real-time NoSQL database that allows data synchronization and offline support.
Firebase Analytics: Provides deep insights into user behavior, helping developers improve user experience and engagement.
Crashlytics: Monitors app stability and reports crashes for proactive debugging.
For Flutter developers, Firebase provides easy integration, enabling seamless cross-platform support and consistent performance across iOS, Android, and the web.
Firebase simplifies backend development by offering various services that Flutter apps can use right out of the box. Here are some of the key features:

Authentication: Streamlines integrating user authentication, including Google, Facebook, and email logins.
Cloud Firestore: A real-time NoSQL database that allows data synchronization and offline support.
Firebase Analytics: Provides deep insights into user behavior, helping developers improve user experience and engagement.
Crashlytics: Monitors app stability and reports crashes for proactive debugging.
For Flutter developers, Firebase provides easy integration, enabling seamless cross-platform support and consistent performance across iOS, Android, and the web.
Firebase simplifies backend development by offering various services that Flutter apps can use right out of the box. Here are some of the key features:

Authentication: Streamlines integrating user authentication, including Google, Facebook, and email logins.
Cloud Firestore: A real-time NoSQL database that allows data synchronization and offline support.
Firebase Analytics: Provides deep insights into user behavior, helping developers improve user experience and engagement.
Crashlytics: Monitors app stability and reports crashes for proactive debugging.
For Flutter developers, Firebase provides easy integration, enabling seamless cross-platform support and consistent performance across iOS, Android, and the web.
Benefits of Using Firebase with Flutter
Benefits of Using Firebase with Flutter
Benefits of Using Firebase with Flutter
Benefits of Using Firebase with Flutter
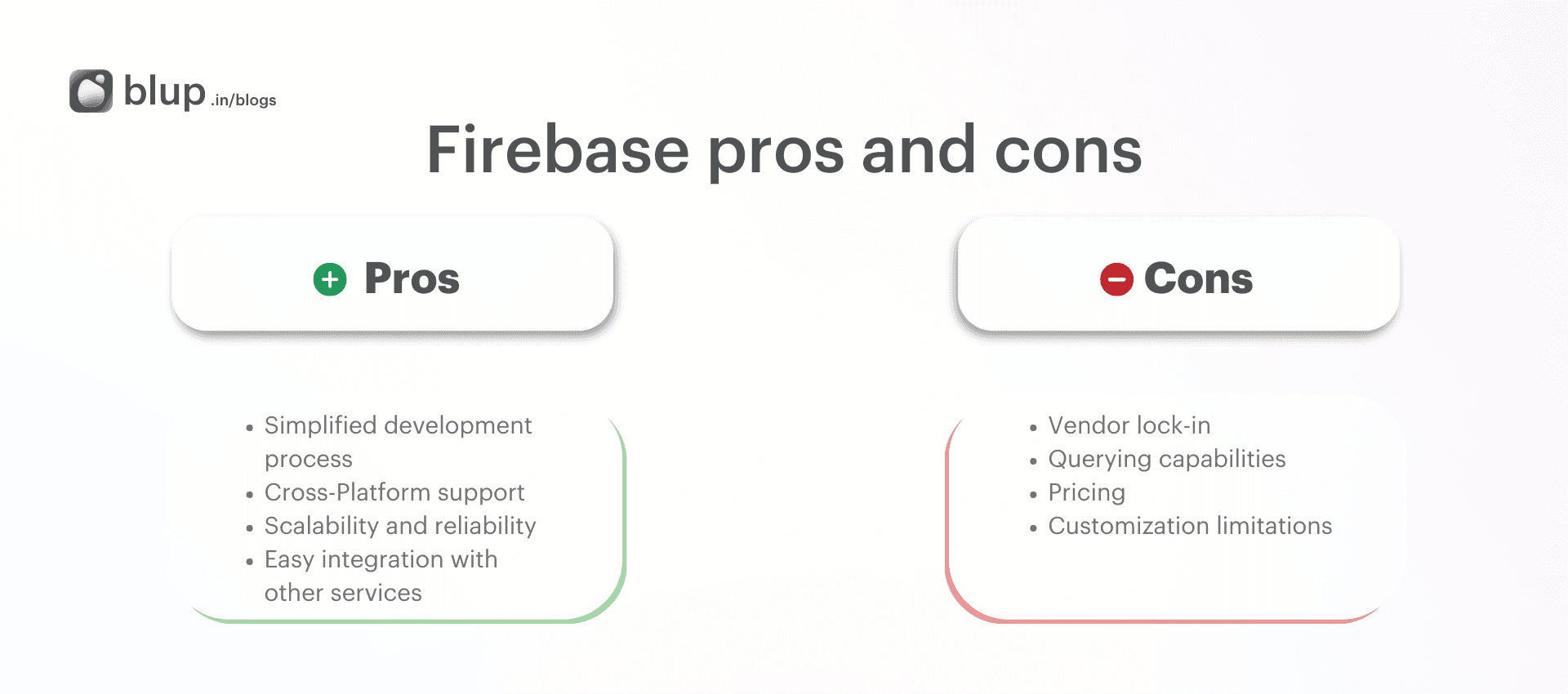
Integrating Firebase with Flutter has several advantages:
Fast Development: Firebase’s pre-built services speed up the development process, allowing developers to focus on core app functionality.
Scalability: Firebase offers the flexibility to scale apps based on user demands.
Cross-Platform Support: Firebase allows you to write code once and deploy it across multiple platforms, making it an ideal backend for Flutter apps.
Real-time Data: Firestore offers real-time data syncing, which is beneficial for apps that rely on live updates.
High Security: Firebase provides built-in security through rules and access control, essential for data protection.
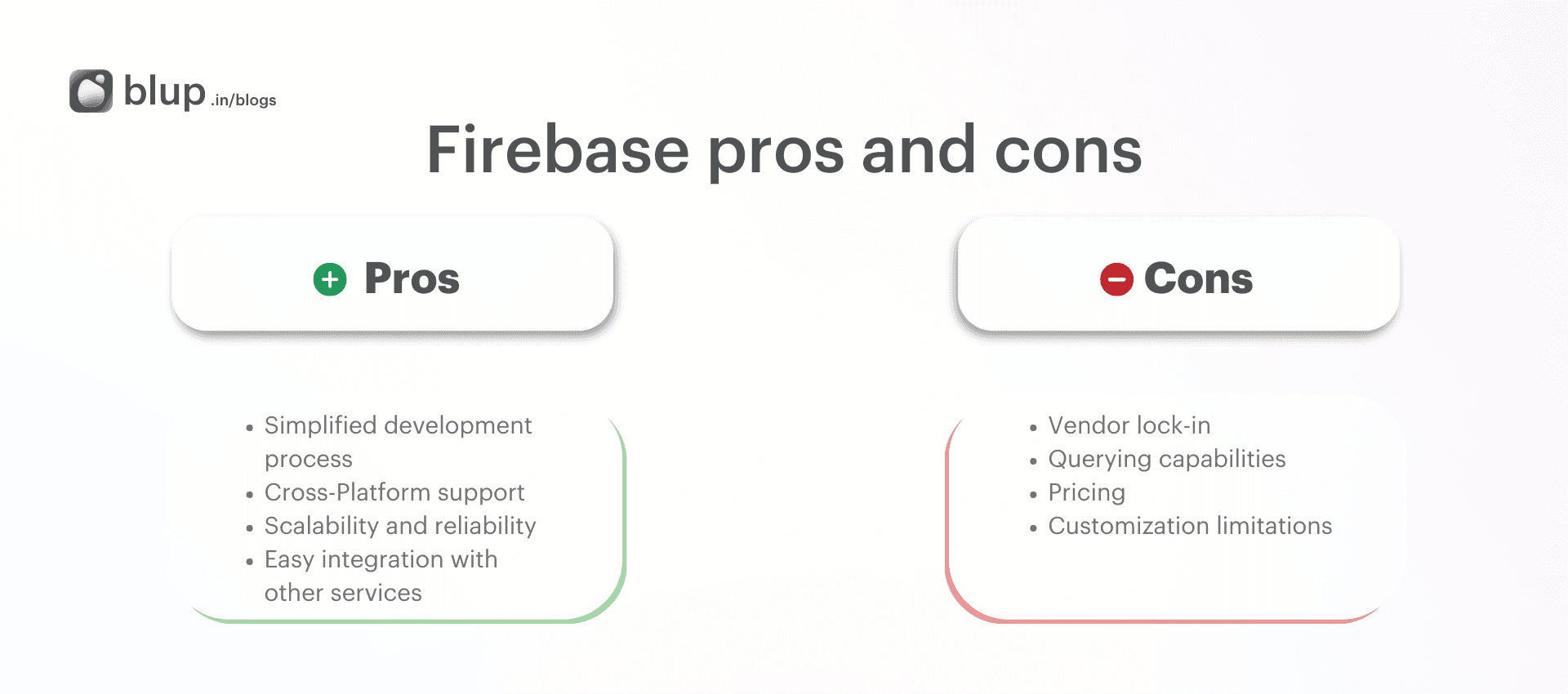
Integrating Firebase with Flutter has several advantages:
Fast Development: Firebase’s pre-built services speed up the development process, allowing developers to focus on core app functionality.
Scalability: Firebase offers the flexibility to scale apps based on user demands.
Cross-Platform Support: Firebase allows you to write code once and deploy it across multiple platforms, making it an ideal backend for Flutter apps.
Real-time Data: Firestore offers real-time data syncing, which is beneficial for apps that rely on live updates.
High Security: Firebase provides built-in security through rules and access control, essential for data protection.
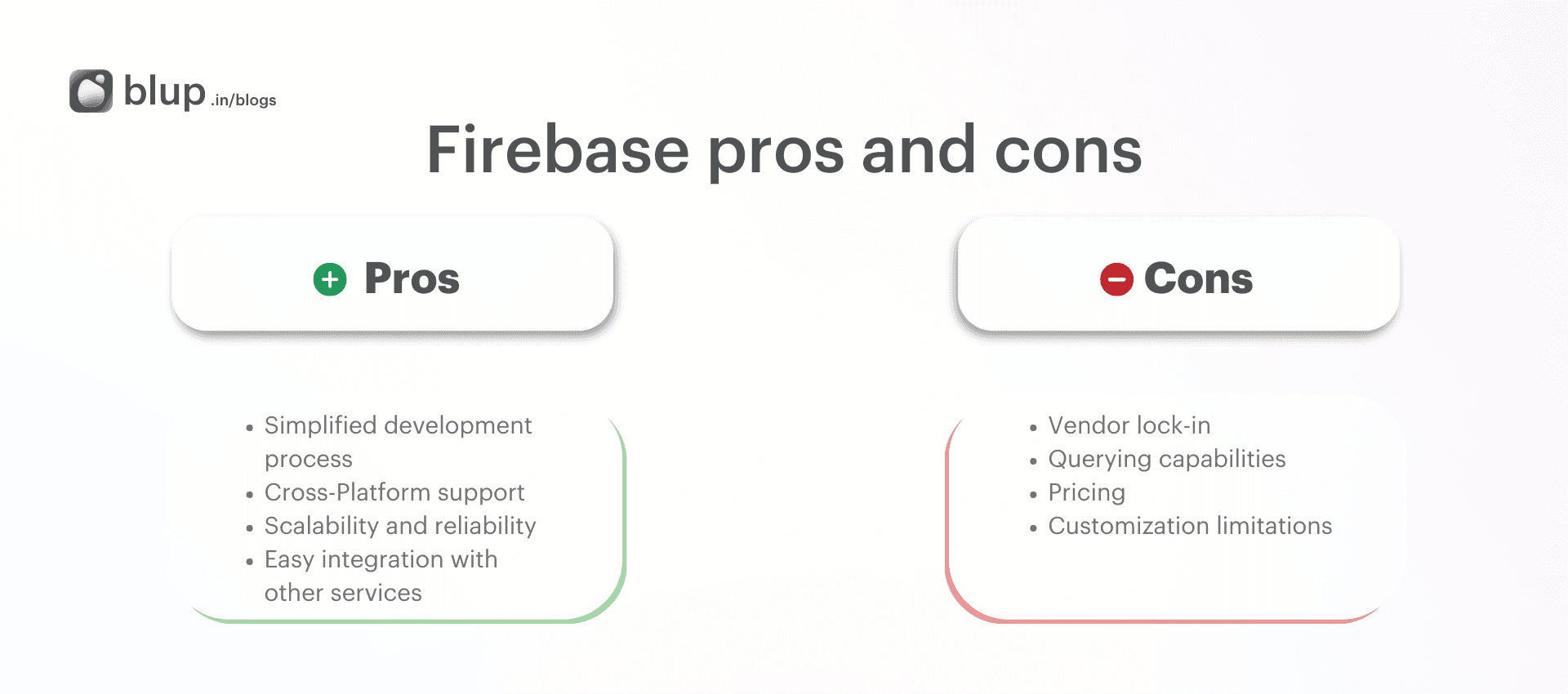
Integrating Firebase with Flutter has several advantages:
Fast Development: Firebase’s pre-built services speed up the development process, allowing developers to focus on core app functionality.
Scalability: Firebase offers the flexibility to scale apps based on user demands.
Cross-Platform Support: Firebase allows you to write code once and deploy it across multiple platforms, making it an ideal backend for Flutter apps.
Real-time Data: Firestore offers real-time data syncing, which is beneficial for apps that rely on live updates.
High Security: Firebase provides built-in security through rules and access control, essential for data protection.
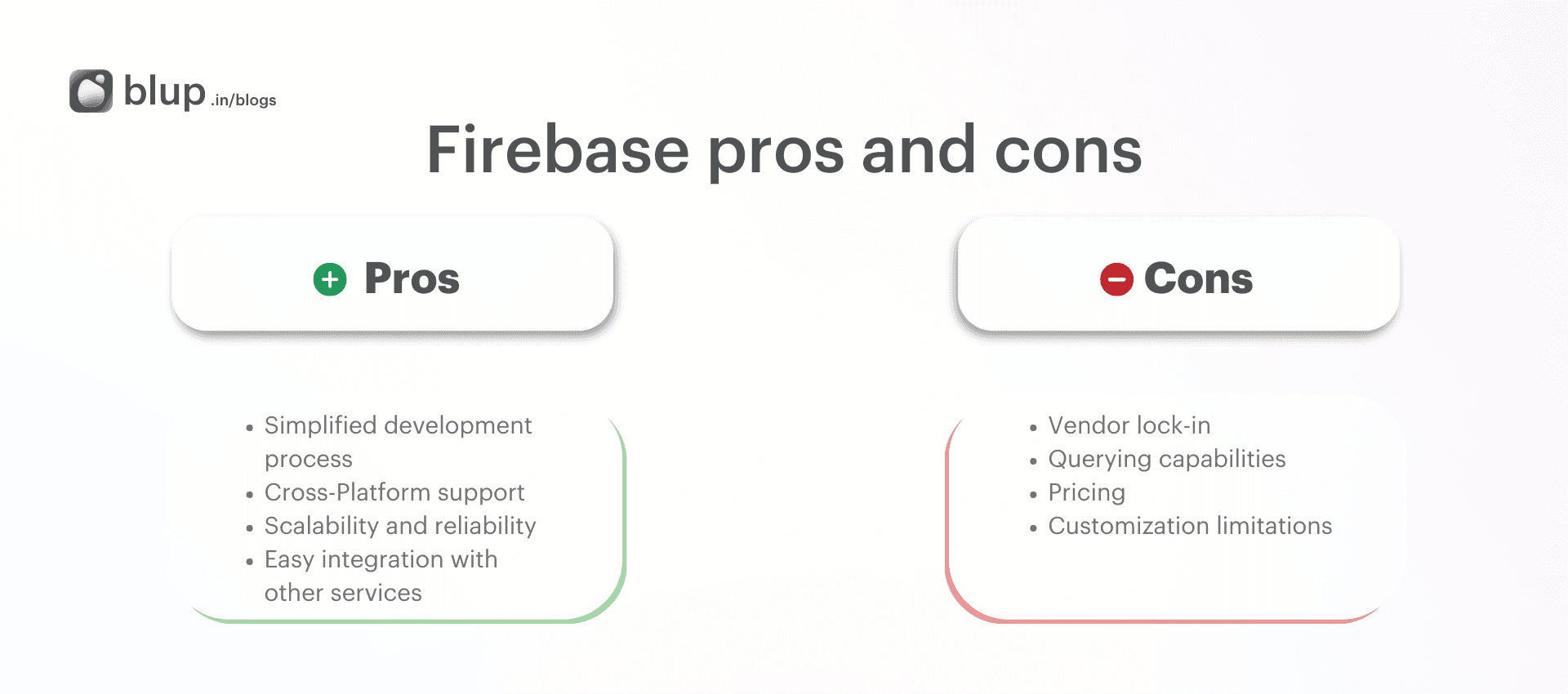
Integrating Firebase with Flutter has several advantages:
Fast Development: Firebase’s pre-built services speed up the development process, allowing developers to focus on core app functionality.
Scalability: Firebase offers the flexibility to scale apps based on user demands.
Cross-Platform Support: Firebase allows you to write code once and deploy it across multiple platforms, making it an ideal backend for Flutter apps.
Real-time Data: Firestore offers real-time data syncing, which is beneficial for apps that rely on live updates.
High Security: Firebase provides built-in security through rules and access control, essential for data protection.
Setting Up Firebase for Flutter
Setting Up Firebase for Flutter
Setting Up Firebase for Flutter
Setting Up Firebase for Flutter
Connect Your App to Firebase
Install Firebase SDKs for Flutter
Make sure you’ve installed and initialized the Firebase SDKs for Flutter.Add Firebase Authentication
In the root of your Flutter project, run:After installation, rebuild your app:
Import Firebase Authentication in Dart
Add the following import in your Dart code:Enable Authentication Providers
In the Firebase Console, go to Authentication > Sign-in Method and enable Email/Password or any other providers you want.
Step 1: Create a Firebase Project
Visit the Firebase Console and sign in with a Google account.
Click on “Add Project” and provide a project name.
Accept the terms, set up Google Analytics (optional), and click on “Create Project.”
Step 2: Register Your Flutter App with Firebase
In your Firebase project console, click on “Add App.”
Select the platform(s) for your Flutter app (iOS, Android, or Web).
Follow the instructions to download the
google-services.json
(for Android) orGoogleService-Info.plist
(for iOS) configuration files.Place these files in the correct directories of your Flutter project (
android/app
for Android andios/Runner
iOS).
Step 3: Install Firebase Plugins in Flutter
Add Firebase dependencies to your Flutter project. Open your pubspec.yaml
file and add the required Firebase packages. Some common Firebase packages include:
dependencies:
firebase_core: latest_version
firebase_auth: latest_version
cloud_firestore: latest_version
firebase_analytics: latest_version
Then, run:
Step 4: Initialize Firebase in Your Flutter App
Initialize Firebase in your Flutter app by updating the main file, main.dart
, as shown below:
Connect Your App to Firebase
Install Firebase SDKs for Flutter
Make sure you’ve installed and initialized the Firebase SDKs for Flutter.Add Firebase Authentication
In the root of your Flutter project, run:After installation, rebuild your app:
Import Firebase Authentication in Dart
Add the following import in your Dart code:Enable Authentication Providers
In the Firebase Console, go to Authentication > Sign-in Method and enable Email/Password or any other providers you want.
Step 1: Create a Firebase Project
Visit the Firebase Console and sign in with a Google account.
Click on “Add Project” and provide a project name.
Accept the terms, set up Google Analytics (optional), and click on “Create Project.”
Step 2: Register Your Flutter App with Firebase
In your Firebase project console, click on “Add App.”
Select the platform(s) for your Flutter app (iOS, Android, or Web).
Follow the instructions to download the
google-services.json
(for Android) orGoogleService-Info.plist
(for iOS) configuration files.Place these files in the correct directories of your Flutter project (
android/app
for Android andios/Runner
iOS).
Step 3: Install Firebase Plugins in Flutter
Add Firebase dependencies to your Flutter project. Open your pubspec.yaml
file and add the required Firebase packages. Some common Firebase packages include:
dependencies:
firebase_core: latest_version
firebase_auth: latest_version
cloud_firestore: latest_version
firebase_analytics: latest_version
Then, run:
Step 4: Initialize Firebase in Your Flutter App
Initialize Firebase in your Flutter app by updating the main file, main.dart
, as shown below:
Connect Your App to Firebase
Install Firebase SDKs for Flutter
Make sure you’ve installed and initialized the Firebase SDKs for Flutter.Add Firebase Authentication
In the root of your Flutter project, run:After installation, rebuild your app:
Import Firebase Authentication in Dart
Add the following import in your Dart code:Enable Authentication Providers
In the Firebase Console, go to Authentication > Sign-in Method and enable Email/Password or any other providers you want.
Step 1: Create a Firebase Project
Visit the Firebase Console and sign in with a Google account.
Click on “Add Project” and provide a project name.
Accept the terms, set up Google Analytics (optional), and click on “Create Project.”
Step 2: Register Your Flutter App with Firebase
In your Firebase project console, click on “Add App.”
Select the platform(s) for your Flutter app (iOS, Android, or Web).
Follow the instructions to download the
google-services.json
(for Android) orGoogleService-Info.plist
(for iOS) configuration files.Place these files in the correct directories of your Flutter project (
android/app
for Android andios/Runner
iOS).
Step 3: Install Firebase Plugins in Flutter
Add Firebase dependencies to your Flutter project. Open your pubspec.yaml
file and add the required Firebase packages. Some common Firebase packages include:
dependencies:
firebase_core: latest_version
firebase_auth: latest_version
cloud_firestore: latest_version
firebase_analytics: latest_version
Then, run:
Step 4: Initialize Firebase in Your Flutter App
Initialize Firebase in your Flutter app by updating the main file, main.dart
, as shown below:
Connect Your App to Firebase
Install Firebase SDKs for Flutter
Make sure you’ve installed and initialized the Firebase SDKs for Flutter.Add Firebase Authentication
In the root of your Flutter project, run:After installation, rebuild your app:
Import Firebase Authentication in Dart
Add the following import in your Dart code:Enable Authentication Providers
In the Firebase Console, go to Authentication > Sign-in Method and enable Email/Password or any other providers you want.
Step 1: Create a Firebase Project
Visit the Firebase Console and sign in with a Google account.
Click on “Add Project” and provide a project name.
Accept the terms, set up Google Analytics (optional), and click on “Create Project.”
Step 2: Register Your Flutter App with Firebase
In your Firebase project console, click on “Add App.”
Select the platform(s) for your Flutter app (iOS, Android, or Web).
Follow the instructions to download the
google-services.json
(for Android) orGoogleService-Info.plist
(for iOS) configuration files.Place these files in the correct directories of your Flutter project (
android/app
for Android andios/Runner
iOS).
Step 3: Install Firebase Plugins in Flutter
Add Firebase dependencies to your Flutter project. Open your pubspec.yaml
file and add the required Firebase packages. Some common Firebase packages include:
dependencies:
firebase_core: latest_version
firebase_auth: latest_version
cloud_firestore: latest_version
firebase_analytics: latest_version
Then, run:
Step 4: Initialize Firebase in Your Flutter App
Initialize Firebase in your Flutter app by updating the main file, main.dart
, as shown below:
Firebase Features for Flutter
Firebase Features for Flutter
Firebase Features for Flutter
Firebase Features for Flutter
Firebase Authentication
Firebase Authentication allows users to sign in using various methods, including email/password, Google, Facebook, and phone numbers. Here’s an example of setting up email and password login:
Cloud Firestore
Firestore is Firebase’s NoSQL cloud database that supports real-time data syncing. Here’s a quick example of adding and retrieving data from Firestore:
import 'package:cloud_firestore/cloud_firestore.dart';
final FirebaseFirestore firestore = FirebaseFirestore.instance;
// Add Data
void addData() async {
await firestore.collection('users').add({
'name': 'John Doe',
'age': 30,
});
}
// Retrieve Data
void retrieveData() {
firestore.collection('users').get().then((QuerySnapshot querySnapshot) {
querySnapshot.docs.forEach((doc) {
print(doc["name"]
Firebase Analytics
Firebase Analytics provides insights into how users interact with your app, helping you understand user behavior. Initialize Analytics and log custom events to track user actions:
Crashlytics for Stability Monitoring
Firebase Crashlytics provides real-time crash reporting, helping developers debug issues quickly. To use it, add firebase_crashlytics
to your pubspec.yaml
and set up custom error handling.
Firebase Authentication
Firebase Authentication allows users to sign in using various methods, including email/password, Google, Facebook, and phone numbers. Here’s an example of setting up email and password login:
Cloud Firestore
Firestore is Firebase’s NoSQL cloud database that supports real-time data syncing. Here’s a quick example of adding and retrieving data from Firestore:
import 'package:cloud_firestore/cloud_firestore.dart';
final FirebaseFirestore firestore = FirebaseFirestore.instance;
// Add Data
void addData() async {
await firestore.collection('users').add({
'name': 'John Doe',
'age': 30,
});
}
// Retrieve Data
void retrieveData() {
firestore.collection('users').get().then((QuerySnapshot querySnapshot) {
querySnapshot.docs.forEach((doc) {
print(doc["name"]
Firebase Analytics
Firebase Analytics provides insights into how users interact with your app, helping you understand user behavior. Initialize Analytics and log custom events to track user actions:
Crashlytics for Stability Monitoring
Firebase Crashlytics provides real-time crash reporting, helping developers debug issues quickly. To use it, add firebase_crashlytics
to your pubspec.yaml
and set up custom error handling.
Firebase Authentication
Firebase Authentication allows users to sign in using various methods, including email/password, Google, Facebook, and phone numbers. Here’s an example of setting up email and password login:
Cloud Firestore
Firestore is Firebase’s NoSQL cloud database that supports real-time data syncing. Here’s a quick example of adding and retrieving data from Firestore:
import 'package:cloud_firestore/cloud_firestore.dart';
final FirebaseFirestore firestore = FirebaseFirestore.instance;
// Add Data
void addData() async {
await firestore.collection('users').add({
'name': 'John Doe',
'age': 30,
});
}
// Retrieve Data
void retrieveData() {
firestore.collection('users').get().then((QuerySnapshot querySnapshot) {
querySnapshot.docs.forEach((doc) {
print(doc["name"]
Firebase Analytics
Firebase Analytics provides insights into how users interact with your app, helping you understand user behavior. Initialize Analytics and log custom events to track user actions:
Crashlytics for Stability Monitoring
Firebase Crashlytics provides real-time crash reporting, helping developers debug issues quickly. To use it, add firebase_crashlytics
to your pubspec.yaml
and set up custom error handling.
Firebase Authentication
Firebase Authentication allows users to sign in using various methods, including email/password, Google, Facebook, and phone numbers. Here’s an example of setting up email and password login:
Cloud Firestore
Firestore is Firebase’s NoSQL cloud database that supports real-time data syncing. Here’s a quick example of adding and retrieving data from Firestore:
import 'package:cloud_firestore/cloud_firestore.dart';
final FirebaseFirestore firestore = FirebaseFirestore.instance;
// Add Data
void addData() async {
await firestore.collection('users').add({
'name': 'John Doe',
'age': 30,
});
}
// Retrieve Data
void retrieveData() {
firestore.collection('users').get().then((QuerySnapshot querySnapshot) {
querySnapshot.docs.forEach((doc) {
print(doc["name"]
Firebase Analytics
Firebase Analytics provides insights into how users interact with your app, helping you understand user behavior. Initialize Analytics and log custom events to track user actions:
Crashlytics for Stability Monitoring
Firebase Crashlytics provides real-time crash reporting, helping developers debug issues quickly. To use it, add firebase_crashlytics
to your pubspec.yaml
and set up custom error handling.
Testing and Deploying Firebase in Flutter
Testing and Deploying Firebase in Flutter
Testing and Deploying Firebase in Flutter
Testing and Deploying Firebase in Flutter
Using Firebase Emulator Suite for Local Testing
The Firebase Emulator Suite allows you to run and test Firebase features locally. To set it up, install the Firebase CLI and run firebase emulators:start
from your project directory.
Deploying Firebase Functions (Advanced)
Firebase Functions allow you to run serverless code to automate tasks or interact with other Firebase services. To create a Firebase Function:
Initialize Firebase Functions by running
firebase init functions
.Write your functions in
index.js
orindex.ts
.Deploy functions with
firebase deploy --only functions
.
Using Firebase Emulator Suite for Local Testing
The Firebase Emulator Suite allows you to run and test Firebase features locally. To set it up, install the Firebase CLI and run firebase emulators:start
from your project directory.
Deploying Firebase Functions (Advanced)
Firebase Functions allow you to run serverless code to automate tasks or interact with other Firebase services. To create a Firebase Function:
Initialize Firebase Functions by running
firebase init functions
.Write your functions in
index.js
orindex.ts
.Deploy functions with
firebase deploy --only functions
.
Using Firebase Emulator Suite for Local Testing
The Firebase Emulator Suite allows you to run and test Firebase features locally. To set it up, install the Firebase CLI and run firebase emulators:start
from your project directory.
Deploying Firebase Functions (Advanced)
Firebase Functions allow you to run serverless code to automate tasks or interact with other Firebase services. To create a Firebase Function:
Initialize Firebase Functions by running
firebase init functions
.Write your functions in
index.js
orindex.ts
.Deploy functions with
firebase deploy --only functions
.
Using Firebase Emulator Suite for Local Testing
The Firebase Emulator Suite allows you to run and test Firebase features locally. To set it up, install the Firebase CLI and run firebase emulators:start
from your project directory.
Deploying Firebase Functions (Advanced)
Firebase Functions allow you to run serverless code to automate tasks or interact with other Firebase services. To create a Firebase Function:
Initialize Firebase Functions by running
firebase init functions
.Write your functions in
index.js
orindex.ts
.Deploy functions with
firebase deploy --only functions
.
FAQs
FAQs
FAQs
FAQs
Why should I use Firebase with Flutter?
Firebase offers easy-to-use, scalable backend services that work seamlessly with Flutter, making it ideal for real-time apps and cross-platform development.How do I secure my Firebase database in Flutter?
Use Firestore security rules to control access to your database. You can specify rules based on authentication status, user roles, or data ownership.Can I test Firebase locally in Flutter?
Yes, Firebase Emulator Suite enables local testing for Firestore, authentication, and functions, which helps test without affecting live data.What are the costs associated with Firebase?
Firebase has a free tier, but costs can vary depending on the services you use and app traffic. Monitor usage and refer to Firebase’s pricing page to avoid unexpected costs.How do I integrate Firebase with multiple platforms in a single Flutter app?
You can integrate Firebase with Android, iOS, and the web by following Firebase's setup guides for each platform and adding the appropriate configuration files to your Flutter project.
Why should I use Firebase with Flutter?
Firebase offers easy-to-use, scalable backend services that work seamlessly with Flutter, making it ideal for real-time apps and cross-platform development.How do I secure my Firebase database in Flutter?
Use Firestore security rules to control access to your database. You can specify rules based on authentication status, user roles, or data ownership.Can I test Firebase locally in Flutter?
Yes, Firebase Emulator Suite enables local testing for Firestore, authentication, and functions, which helps test without affecting live data.What are the costs associated with Firebase?
Firebase has a free tier, but costs can vary depending on the services you use and app traffic. Monitor usage and refer to Firebase’s pricing page to avoid unexpected costs.How do I integrate Firebase with multiple platforms in a single Flutter app?
You can integrate Firebase with Android, iOS, and the web by following Firebase's setup guides for each platform and adding the appropriate configuration files to your Flutter project.
Why should I use Firebase with Flutter?
Firebase offers easy-to-use, scalable backend services that work seamlessly with Flutter, making it ideal for real-time apps and cross-platform development.How do I secure my Firebase database in Flutter?
Use Firestore security rules to control access to your database. You can specify rules based on authentication status, user roles, or data ownership.Can I test Firebase locally in Flutter?
Yes, Firebase Emulator Suite enables local testing for Firestore, authentication, and functions, which helps test without affecting live data.What are the costs associated with Firebase?
Firebase has a free tier, but costs can vary depending on the services you use and app traffic. Monitor usage and refer to Firebase’s pricing page to avoid unexpected costs.How do I integrate Firebase with multiple platforms in a single Flutter app?
You can integrate Firebase with Android, iOS, and the web by following Firebase's setup guides for each platform and adding the appropriate configuration files to your Flutter project.
Why should I use Firebase with Flutter?
Firebase offers easy-to-use, scalable backend services that work seamlessly with Flutter, making it ideal for real-time apps and cross-platform development.How do I secure my Firebase database in Flutter?
Use Firestore security rules to control access to your database. You can specify rules based on authentication status, user roles, or data ownership.Can I test Firebase locally in Flutter?
Yes, Firebase Emulator Suite enables local testing for Firestore, authentication, and functions, which helps test without affecting live data.What are the costs associated with Firebase?
Firebase has a free tier, but costs can vary depending on the services you use and app traffic. Monitor usage and refer to Firebase’s pricing page to avoid unexpected costs.How do I integrate Firebase with multiple platforms in a single Flutter app?
You can integrate Firebase with Android, iOS, and the web by following Firebase's setup guides for each platform and adding the appropriate configuration files to your Flutter project.
Conclusion
Conclusion
Conclusion
Conclusion
Firebase offers a powerful backend infrastructure for Flutter developers, making it easier to build scalable, feature-rich applications. By leveraging Firebase’s suite of services, from real-time databases to robust analytics and crash reporting, you can streamline your development process and create a seamless user experience.
Firebase offers a powerful backend infrastructure for Flutter developers, making it easier to build scalable, feature-rich applications. By leveraging Firebase’s suite of services, from real-time databases to robust analytics and crash reporting, you can streamline your development process and create a seamless user experience.
Firebase offers a powerful backend infrastructure for Flutter developers, making it easier to build scalable, feature-rich applications. By leveraging Firebase’s suite of services, from real-time databases to robust analytics and crash reporting, you can streamline your development process and create a seamless user experience.
Firebase offers a powerful backend infrastructure for Flutter developers, making it easier to build scalable, feature-rich applications. By leveraging Firebase’s suite of services, from real-time databases to robust analytics and crash reporting, you can streamline your development process and create a seamless user experience.
Table of content
India(HQ)
9th Floor, Tower C, Logix Cyber Park,
C Block, Phase 2, Industrial Area, Sector 62, Noida, Uttar Pradesh, 201309
USA
2081 Center Street Berkeley,
CA 94704
© 2021-23 Blupx Private Limited.
All rights reserved.
India(HQ)
9th Floor, Tower C, Logix Cyber Park,
C Block, Phase 2, Industrial Area, Sector 62, Noida, Uttar Pradesh, 201309
USA
2081 Center Street Berkeley,
CA 94704
© 2021-23 Blupx Private Limited.
All rights reserved.
India(HQ)
9th Floor, Tower C, Logix Cyber Park,
C Block, Phase 2, Industrial Area, Sector 62, Noida, Uttar Pradesh, 201309
USA
2081 Center Street Berkeley,
CA 94704
© 2021-23 Blupx Private Limited.
All rights reserved.