Networking & http in flutter
5 mins
5 mins

Ashutosh
Published on Oct 9, 2024
Networking and HTTP in Flutter: An Overview
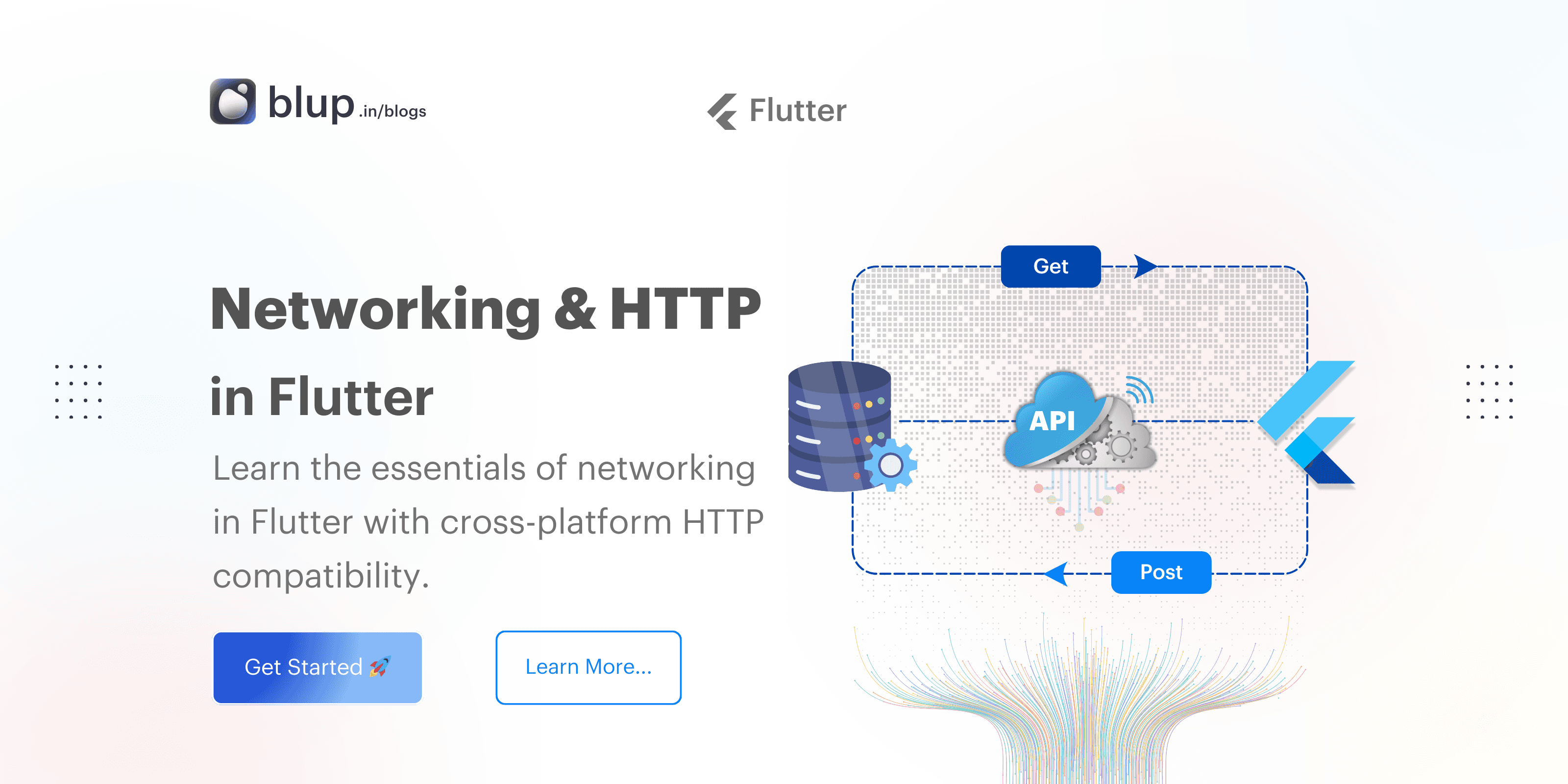
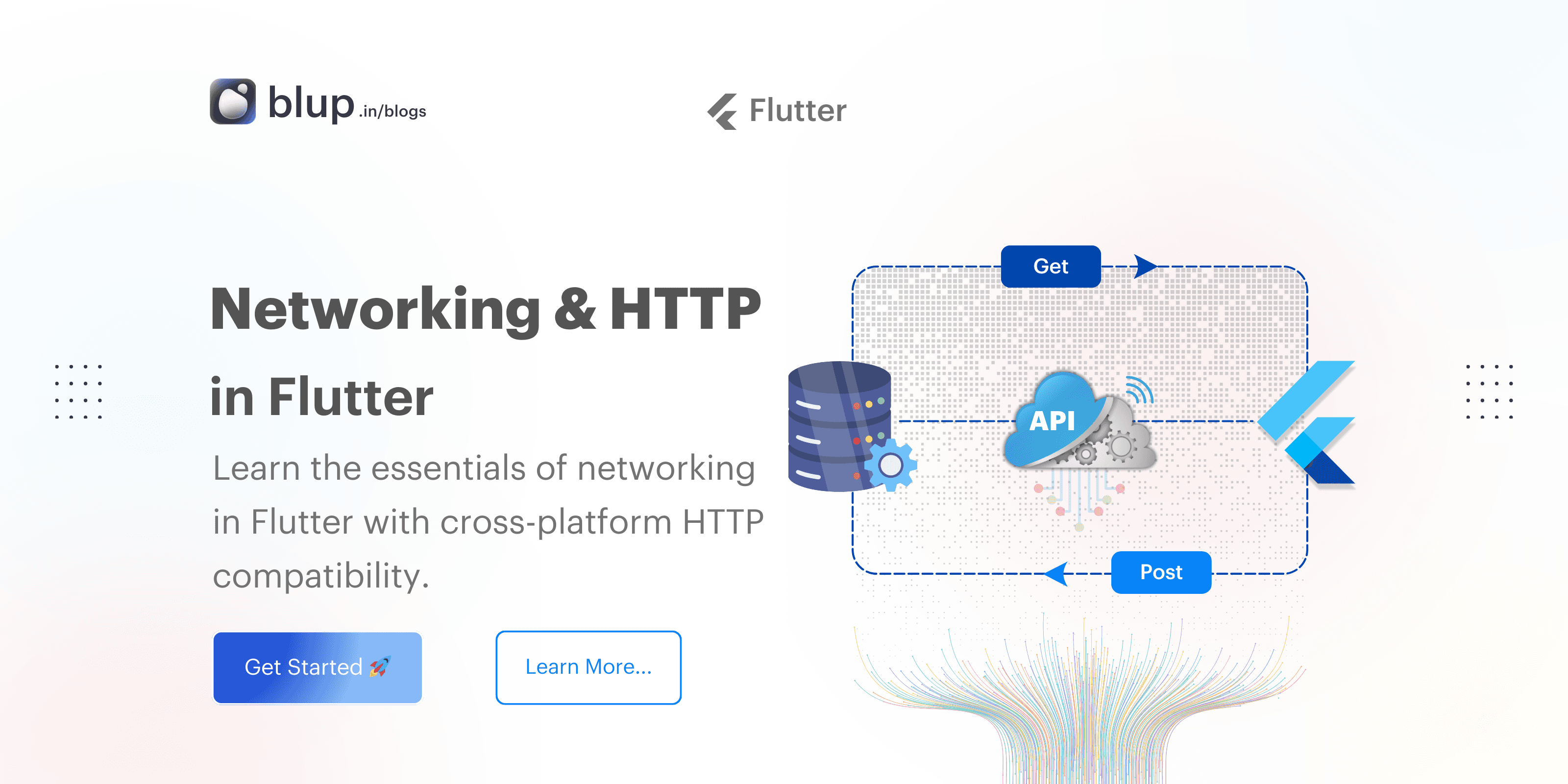
Introduction to Networking in Flutter
Introduction to Networking in Flutter
Introduction to Networking in Flutter
Introduction to Networking in Flutter
Networking is an essential part of modern mobile app development. Whether fetching data from a remote API, posting data to a server, or handling real-time updates, networking is at the core of creating dynamic, data-driven apps.
Flutter, Google’s open-source framework, provides several tools and packages to implement HTTP networking across platforms like Android, iOS, web, macOS, and more. This blog aims to give you a high-level overview of how networking works in Flutter and show you how to make HTTP requests, handle platform-specific networking concerns, and implement best practices.
People Also Ask:
What is networking in Flutter?
Why is networking important for Flutter apps?
Networking is an essential part of modern mobile app development. Whether fetching data from a remote API, posting data to a server, or handling real-time updates, networking is at the core of creating dynamic, data-driven apps.
Flutter, Google’s open-source framework, provides several tools and packages to implement HTTP networking across platforms like Android, iOS, web, macOS, and more. This blog aims to give you a high-level overview of how networking works in Flutter and show you how to make HTTP requests, handle platform-specific networking concerns, and implement best practices.
People Also Ask:
What is networking in Flutter?
Why is networking important for Flutter apps?
Networking is an essential part of modern mobile app development. Whether fetching data from a remote API, posting data to a server, or handling real-time updates, networking is at the core of creating dynamic, data-driven apps.
Flutter, Google’s open-source framework, provides several tools and packages to implement HTTP networking across platforms like Android, iOS, web, macOS, and more. This blog aims to give you a high-level overview of how networking works in Flutter and show you how to make HTTP requests, handle platform-specific networking concerns, and implement best practices.
People Also Ask:
What is networking in Flutter?
Why is networking important for Flutter apps?
Networking is an essential part of modern mobile app development. Whether fetching data from a remote API, posting data to a server, or handling real-time updates, networking is at the core of creating dynamic, data-driven apps.
Flutter, Google’s open-source framework, provides several tools and packages to implement HTTP networking across platforms like Android, iOS, web, macOS, and more. This blog aims to give you a high-level overview of how networking works in Flutter and show you how to make HTTP requests, handle platform-specific networking concerns, and implement best practices.
People Also Ask:
What is networking in Flutter?
Why is networking important for Flutter apps?
Cross-Platform HTTP Networking in Flutter
Cross-Platform HTTP Networking in Flutter
Cross-Platform HTTP Networking in Flutter
Cross-Platform HTTP Networking in Flutter
One of the most compelling features of Flutter is its cross-platform nature. With a single codebase, you can implement HTTP networking for Android, iOS, and the web, without writing separate code for each platform.
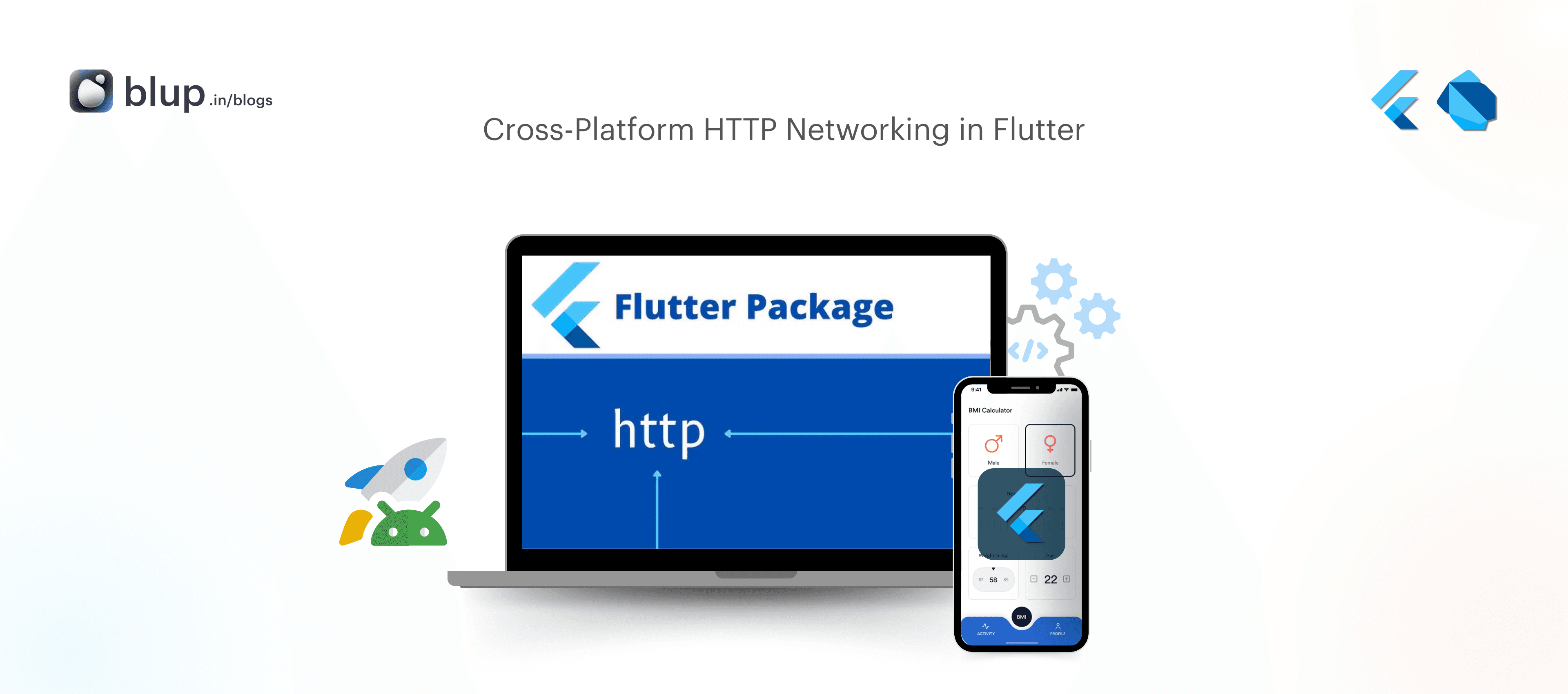
The http
package is the most commonly used package for making HTTP requests in Flutter. It provides methods to send HTTP requests like GET, POST, PUT, and DELETE and simplifies the process of fetching or sending data over the internet.
Here’s how to make a simple GET request in Flutter using the http
package:
import 'package:http/http.dart' as http;
import 'dart:convert';
Future<void> fetchData() async {
final response = await http.get(Uri.parse('https://jsonplaceholder.typicode.com/posts'));
if (response.statusCode == 200) {
var data = jsonDecode(response.body);
print(data);
} else {
throw Exception('Failed to load data');
}
}
In this example, a GET request is sent to fetch data from an API. The http.get()
method makes the request, and if the response status is 200 (success), it decodes and prints the data.
How does Flutter handle HTTP networking?
Can I use the same HTTP code for Android and iOS in Flutter?
One of the most compelling features of Flutter is its cross-platform nature. With a single codebase, you can implement HTTP networking for Android, iOS, and the web, without writing separate code for each platform.
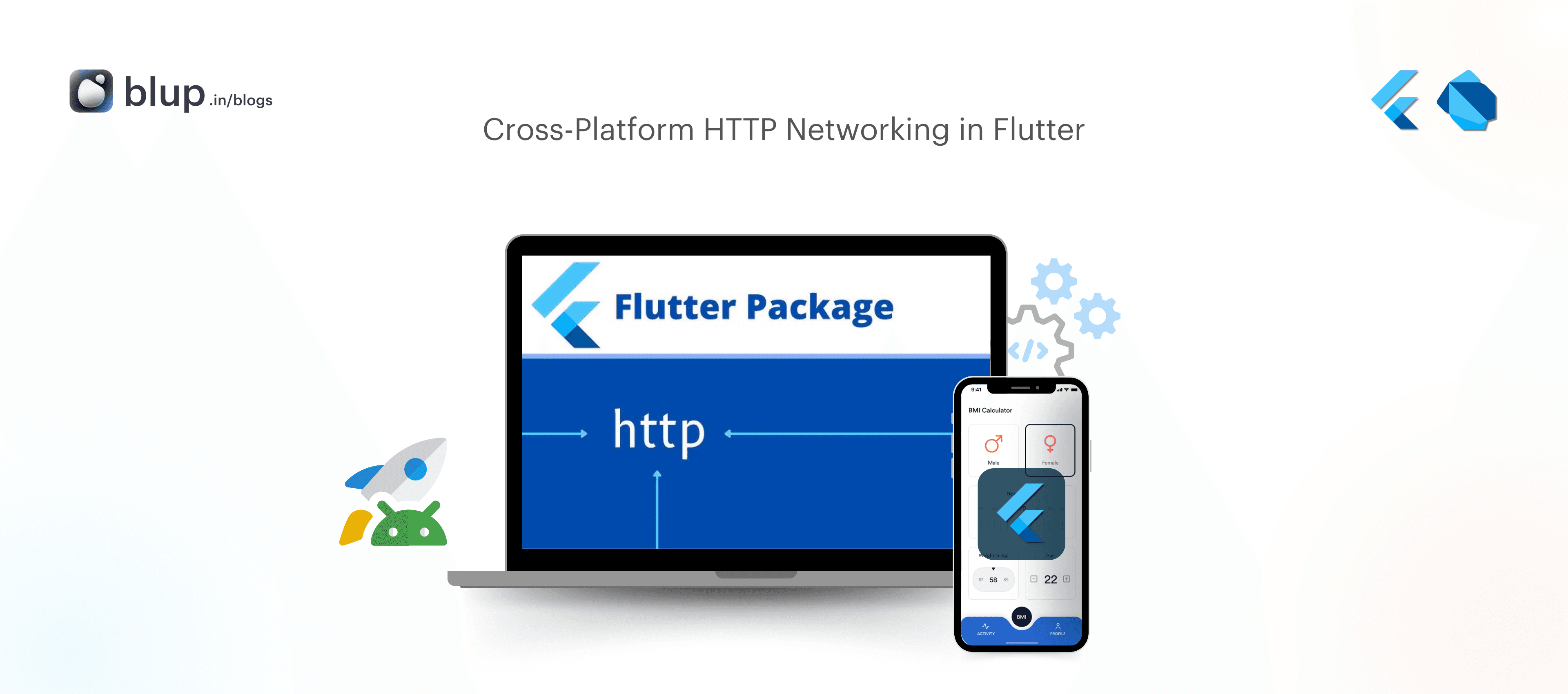
The http
package is the most commonly used package for making HTTP requests in Flutter. It provides methods to send HTTP requests like GET, POST, PUT, and DELETE and simplifies the process of fetching or sending data over the internet.
Here’s how to make a simple GET request in Flutter using the http
package:
import 'package:http/http.dart' as http;
import 'dart:convert';
Future<void> fetchData() async {
final response = await http.get(Uri.parse('https://jsonplaceholder.typicode.com/posts'));
if (response.statusCode == 200) {
var data = jsonDecode(response.body);
print(data);
} else {
throw Exception('Failed to load data');
}
}
In this example, a GET request is sent to fetch data from an API. The http.get()
method makes the request, and if the response status is 200 (success), it decodes and prints the data.
How does Flutter handle HTTP networking?
Can I use the same HTTP code for Android and iOS in Flutter?
One of the most compelling features of Flutter is its cross-platform nature. With a single codebase, you can implement HTTP networking for Android, iOS, and the web, without writing separate code for each platform.
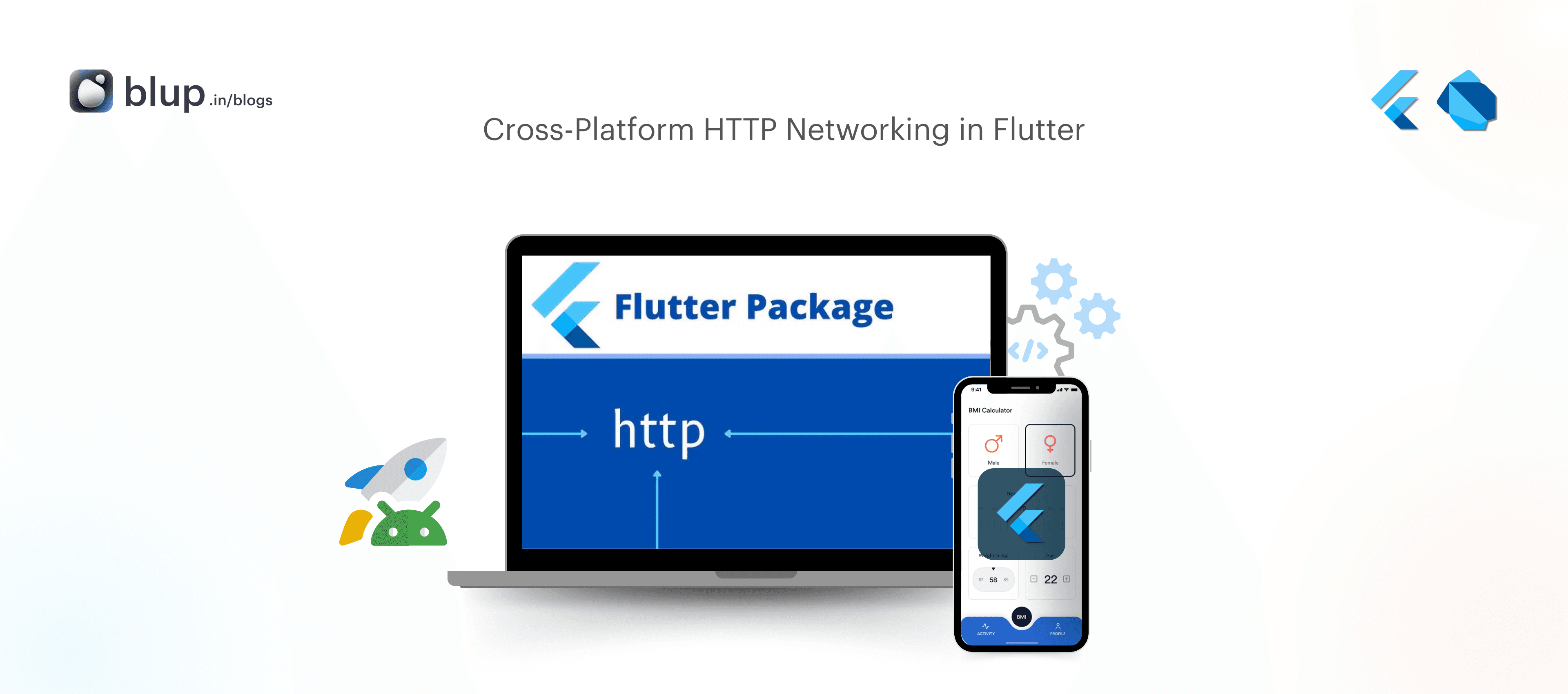
The http
package is the most commonly used package for making HTTP requests in Flutter. It provides methods to send HTTP requests like GET, POST, PUT, and DELETE and simplifies the process of fetching or sending data over the internet.
Here’s how to make a simple GET request in Flutter using the http
package:
import 'package:http/http.dart' as http;
import 'dart:convert';
Future<void> fetchData() async {
final response = await http.get(Uri.parse('https://jsonplaceholder.typicode.com/posts'));
if (response.statusCode == 200) {
var data = jsonDecode(response.body);
print(data);
} else {
throw Exception('Failed to load data');
}
}
In this example, a GET request is sent to fetch data from an API. The http.get()
method makes the request, and if the response status is 200 (success), it decodes and prints the data.
How does Flutter handle HTTP networking?
Can I use the same HTTP code for Android and iOS in Flutter?
One of the most compelling features of Flutter is its cross-platform nature. With a single codebase, you can implement HTTP networking for Android, iOS, and the web, without writing separate code for each platform.
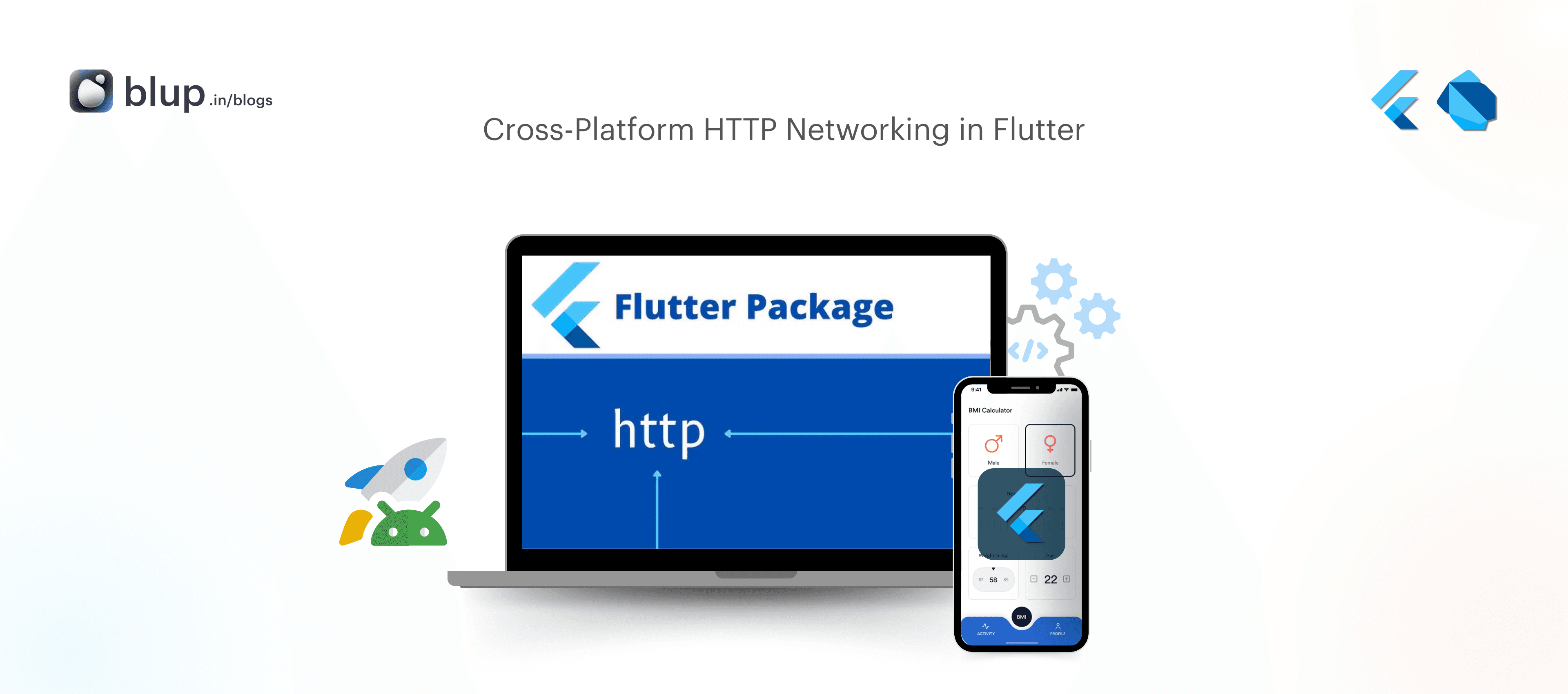
The http
package is the most commonly used package for making HTTP requests in Flutter. It provides methods to send HTTP requests like GET, POST, PUT, and DELETE and simplifies the process of fetching or sending data over the internet.
Here’s how to make a simple GET request in Flutter using the http
package:
import 'package:http/http.dart' as http;
import 'dart:convert';
Future<void> fetchData() async {
final response = await http.get(Uri.parse('https://jsonplaceholder.typicode.com/posts'));
if (response.statusCode == 200) {
var data = jsonDecode(response.body);
print(data);
} else {
throw Exception('Failed to load data');
}
}
In this example, a GET request is sent to fetch data from an API. The http.get()
method makes the request, and if the response status is 200 (success), it decodes and prints the data.
How does Flutter handle HTTP networking?
Can I use the same HTTP code for Android and iOS in Flutter?
Platform-Specific Notes (Android, macOS, Web)
Platform-Specific Notes (Android, macOS, Web)
Platform-Specific Notes (Android, macOS, Web)
Platform-Specific Notes (Android, macOS, Web)
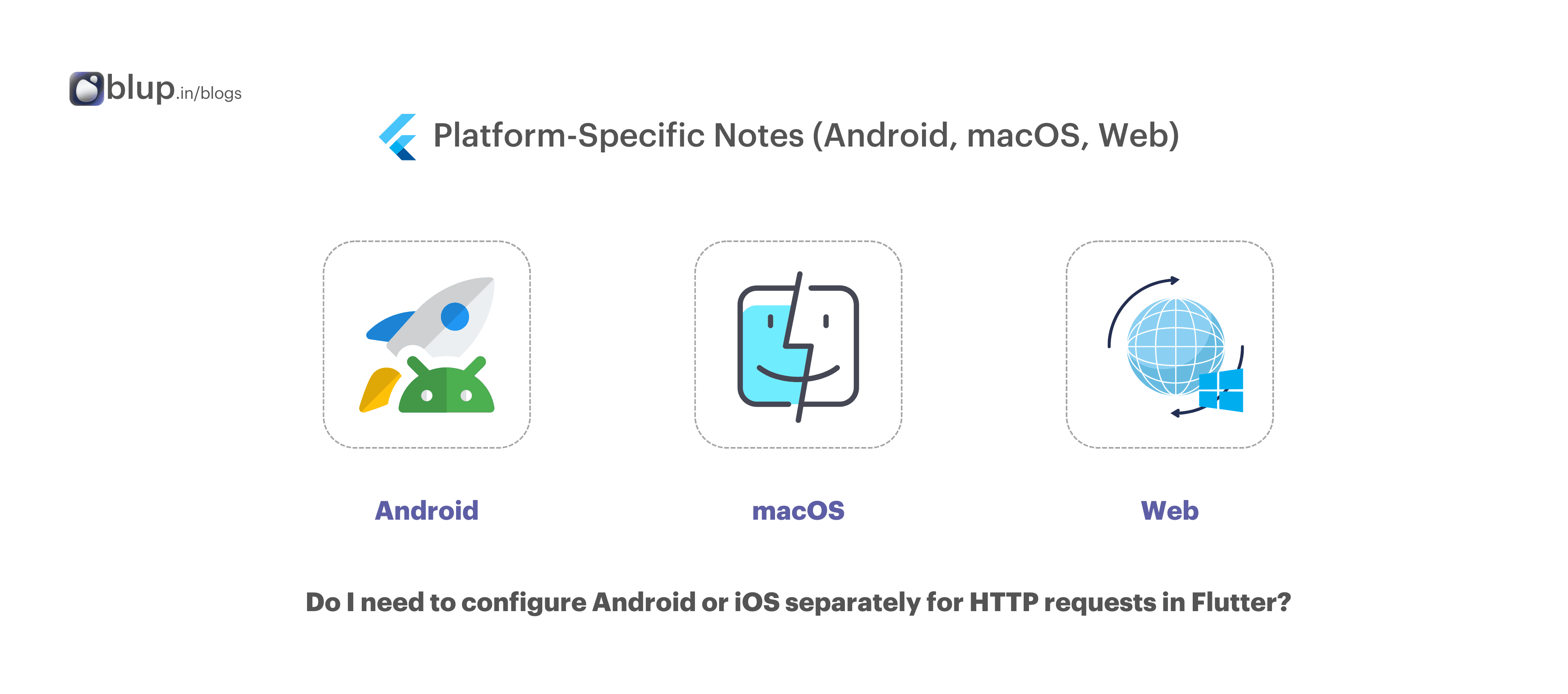
Although Flutter allows cross-platform code reuse, there are certain platform-specific considerations when working with HTTP networking:
1. Android
On Android, you need to declare network access permission in your AndroidManifest.xml
file. Without this permission, your app cannot send or receive data over the network.
Add the following line in AndroidManifest.xml
:
<uses-permission android:name="android.permission.INTERNET" />
<manifest xmlns:android...>
...
<uses-permission android:name="android.permission.INTERNET" />
<application ...
</manifest>
2. macOS
For macOS, you must configure app entitlements to allow network access. Modify the entitlements file (Runner.entitlements
) and ensure your app has the necessary permissions.
<key>com.apple.security.network.client</key>
<true
3. Web
When developing for the web, you may run into CORS (Cross-Origin Resource Sharing) issues. You might need to configure the server to allow cross-origin requests or use third-party services to bypass this restriction.
What are platform-specific networking requirements for Flutter?
Do I need to configure Android or iOS separately for HTTP requests in Flutter?
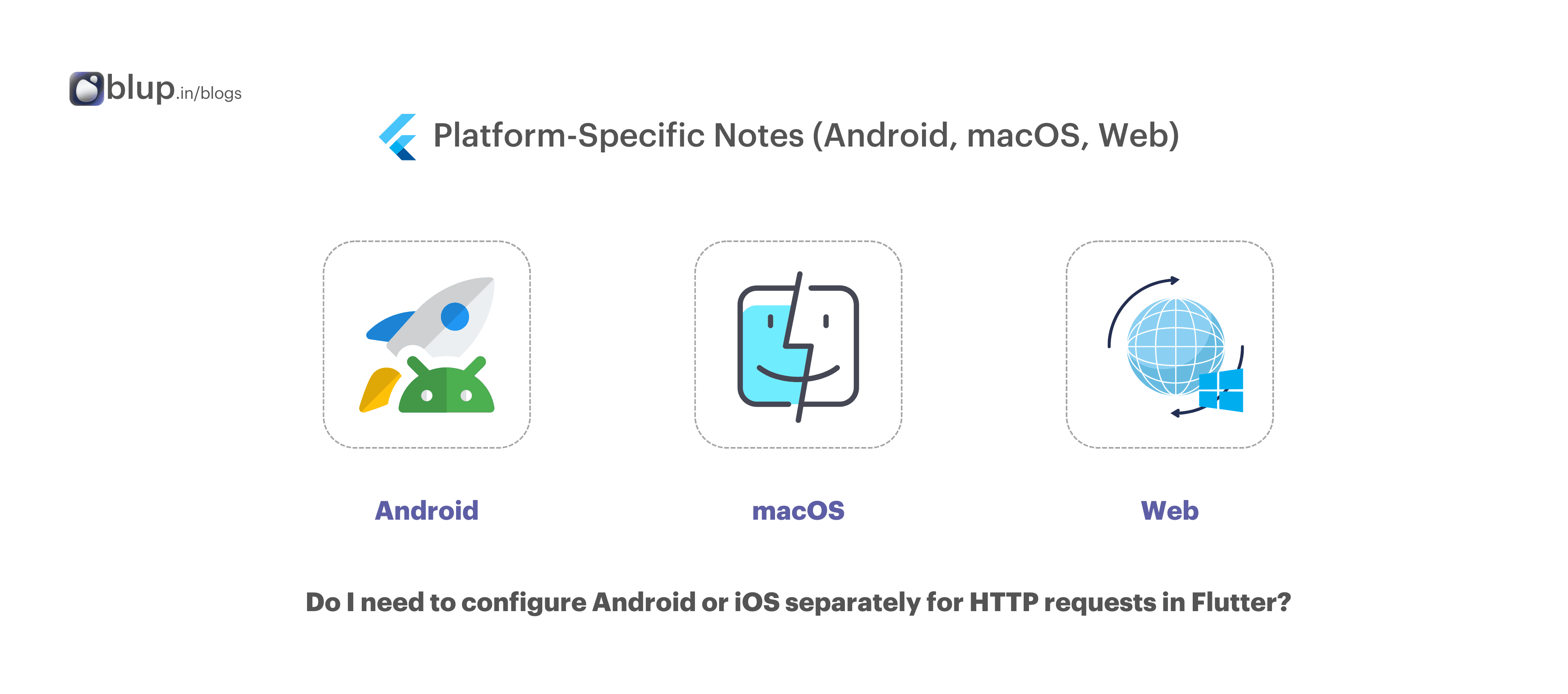
Although Flutter allows cross-platform code reuse, there are certain platform-specific considerations when working with HTTP networking:
1. Android
On Android, you need to declare network access permission in your AndroidManifest.xml
file. Without this permission, your app cannot send or receive data over the network.
Add the following line in AndroidManifest.xml
:
<uses-permission android:name="android.permission.INTERNET" />
<manifest xmlns:android...>
...
<uses-permission android:name="android.permission.INTERNET" />
<application ...
</manifest>
2. macOS
For macOS, you must configure app entitlements to allow network access. Modify the entitlements file (Runner.entitlements
) and ensure your app has the necessary permissions.
<key>com.apple.security.network.client</key>
<true
3. Web
When developing for the web, you may run into CORS (Cross-Origin Resource Sharing) issues. You might need to configure the server to allow cross-origin requests or use third-party services to bypass this restriction.
What are platform-specific networking requirements for Flutter?
Do I need to configure Android or iOS separately for HTTP requests in Flutter?
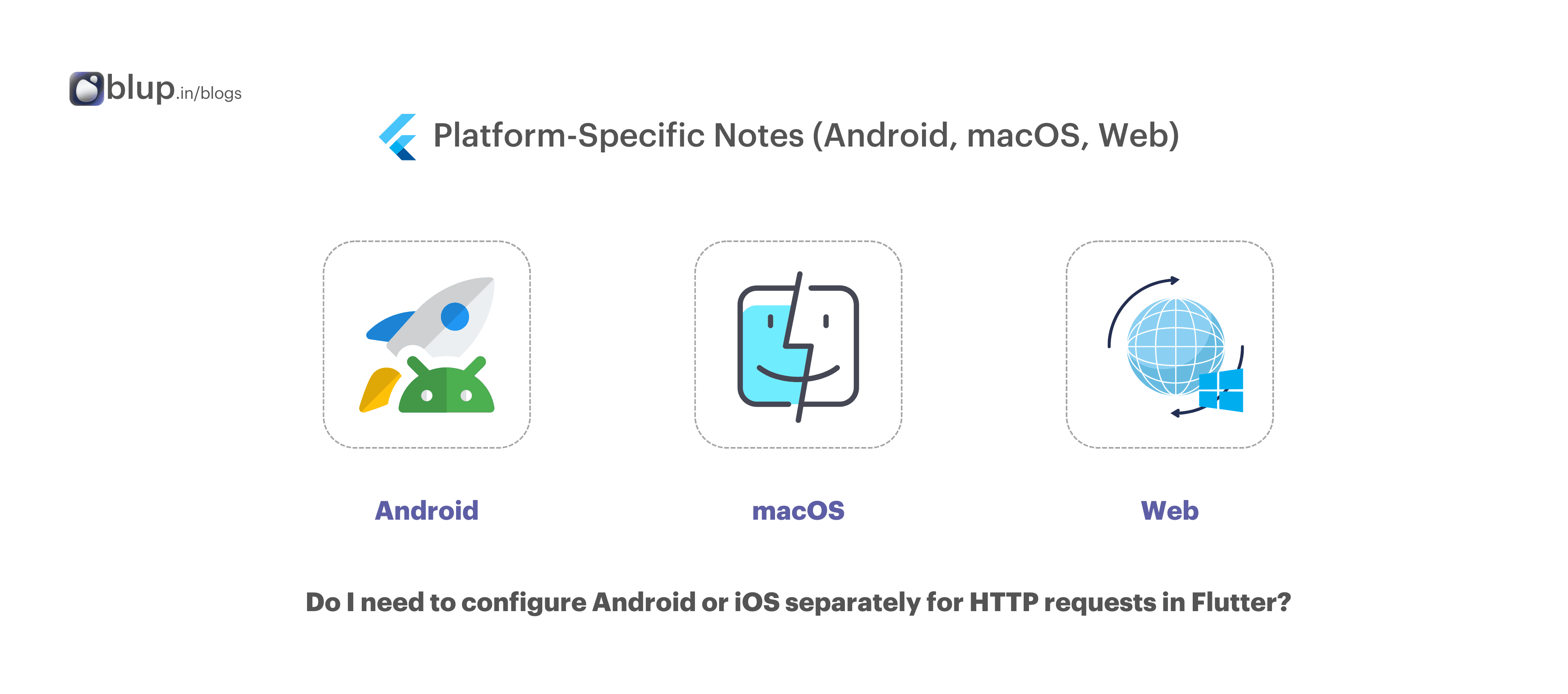
Although Flutter allows cross-platform code reuse, there are certain platform-specific considerations when working with HTTP networking:
1. Android
On Android, you need to declare network access permission in your AndroidManifest.xml
file. Without this permission, your app cannot send or receive data over the network.
Add the following line in AndroidManifest.xml
:
<uses-permission android:name="android.permission.INTERNET" />
<manifest xmlns:android...>
...
<uses-permission android:name="android.permission.INTERNET" />
<application ...
</manifest>
2. macOS
For macOS, you must configure app entitlements to allow network access. Modify the entitlements file (Runner.entitlements
) and ensure your app has the necessary permissions.
<key>com.apple.security.network.client</key>
<true
3. Web
When developing for the web, you may run into CORS (Cross-Origin Resource Sharing) issues. You might need to configure the server to allow cross-origin requests or use third-party services to bypass this restriction.
What are platform-specific networking requirements for Flutter?
Do I need to configure Android or iOS separately for HTTP requests in Flutter?
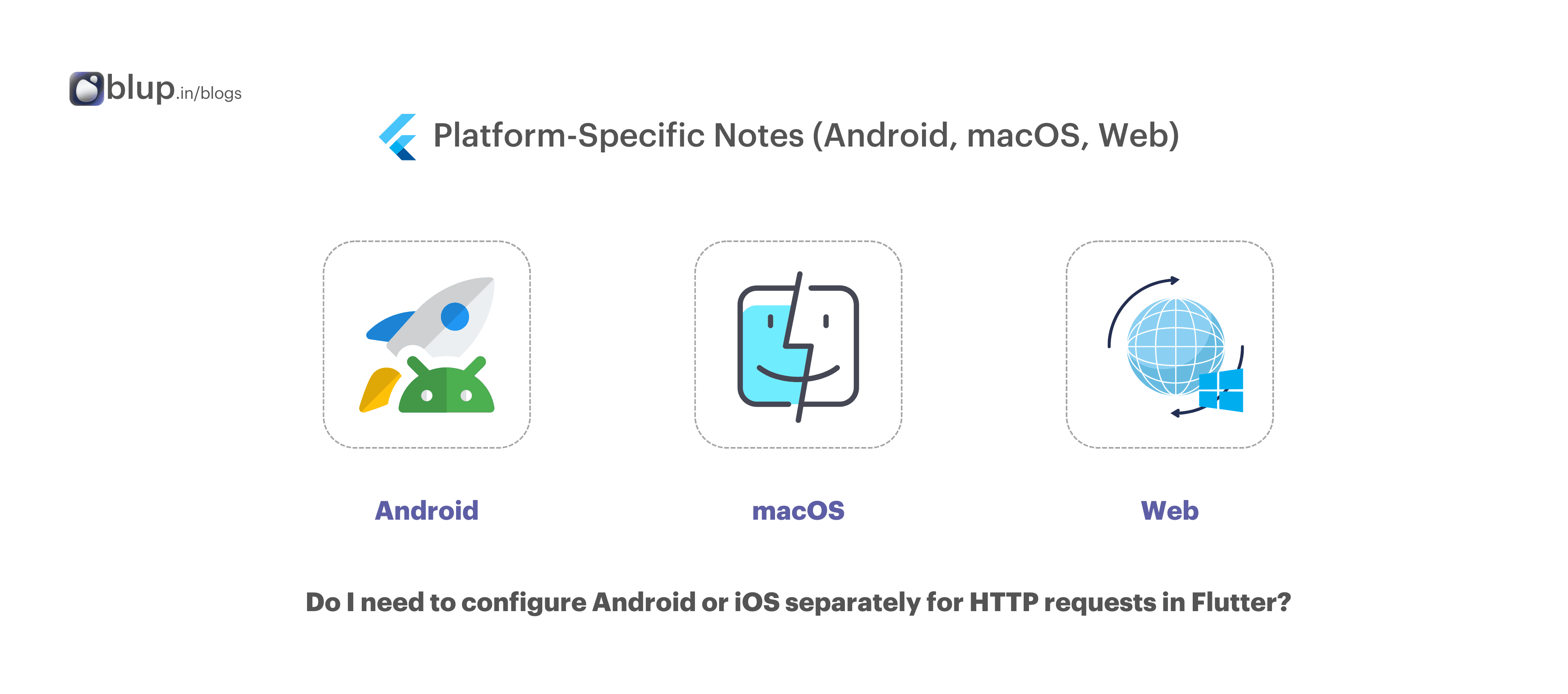
Although Flutter allows cross-platform code reuse, there are certain platform-specific considerations when working with HTTP networking:
1. Android
On Android, you need to declare network access permission in your AndroidManifest.xml
file. Without this permission, your app cannot send or receive data over the network.
Add the following line in AndroidManifest.xml
:
<uses-permission android:name="android.permission.INTERNET" />
<manifest xmlns:android...>
...
<uses-permission android:name="android.permission.INTERNET" />
<application ...
</manifest>
2. macOS
For macOS, you must configure app entitlements to allow network access. Modify the entitlements file (Runner.entitlements
) and ensure your app has the necessary permissions.
<key>com.apple.security.network.client</key>
<true
3. Web
When developing for the web, you may run into CORS (Cross-Origin Resource Sharing) issues. You might need to configure the server to allow cross-origin requests or use third-party services to bypass this restriction.
What are platform-specific networking requirements for Flutter?
Do I need to configure Android or iOS separately for HTTP requests in Flutter?
Example Use Cases for Networking in Flutter
Example Use Cases for Networking in Flutter
Example Use Cases for Networking in Flutter
Example Use Cases for Networking in Flutter
Networking is useful in several scenarios. Here are some common use cases for HTTP networking in Flutter:
1. Fetching Data from an API
One of the most common uses of networking is fetching data from a REST API. Whether you're developing a social media app, an e-commerce app, or a weather app, you’ll likely need to retrieve data from a server.
2. Sending Data to a Server (POST Requests)
Sometimes you need to send data from your app to a server. For example, when a user fills out a form or submits information, the data needs to be sent to a backend server. Here's how you can perform an HTTP POST request in Flutter:
How do I fetch data from a REST API in Flutter?
How do I send data using HTTP POST in Flutter?
Future<void> sendData() async {
final response = await http.post(
Uri.parse('https://jsonplaceholder.typicode.com/posts'),
headers: <String, String>{
'Content-Type': 'application/json; charset=UTF-8',
},
body: jsonEncode(<String, String>{
'title': 'Flutter Networking',
'body': 'This is an example of POST request.',
'userId': '1',
}),
);
if (response.statusCode == 201) {
print('Data posted successfully');
} else {
throw Exception('Failed to post data');
}
}
This example demonstrates how to send data to a server using a POST request. It includes JSON encoding to properly format the data.
Networking is useful in several scenarios. Here are some common use cases for HTTP networking in Flutter:
1. Fetching Data from an API
One of the most common uses of networking is fetching data from a REST API. Whether you're developing a social media app, an e-commerce app, or a weather app, you’ll likely need to retrieve data from a server.
2. Sending Data to a Server (POST Requests)
Sometimes you need to send data from your app to a server. For example, when a user fills out a form or submits information, the data needs to be sent to a backend server. Here's how you can perform an HTTP POST request in Flutter:
How do I fetch data from a REST API in Flutter?
How do I send data using HTTP POST in Flutter?
Future<void> sendData() async {
final response = await http.post(
Uri.parse('https://jsonplaceholder.typicode.com/posts'),
headers: <String, String>{
'Content-Type': 'application/json; charset=UTF-8',
},
body: jsonEncode(<String, String>{
'title': 'Flutter Networking',
'body': 'This is an example of POST request.',
'userId': '1',
}),
);
if (response.statusCode == 201) {
print('Data posted successfully');
} else {
throw Exception('Failed to post data');
}
}
This example demonstrates how to send data to a server using a POST request. It includes JSON encoding to properly format the data.
Networking is useful in several scenarios. Here are some common use cases for HTTP networking in Flutter:
1. Fetching Data from an API
One of the most common uses of networking is fetching data from a REST API. Whether you're developing a social media app, an e-commerce app, or a weather app, you’ll likely need to retrieve data from a server.
2. Sending Data to a Server (POST Requests)
Sometimes you need to send data from your app to a server. For example, when a user fills out a form or submits information, the data needs to be sent to a backend server. Here's how you can perform an HTTP POST request in Flutter:
How do I fetch data from a REST API in Flutter?
How do I send data using HTTP POST in Flutter?
Future<void> sendData() async {
final response = await http.post(
Uri.parse('https://jsonplaceholder.typicode.com/posts'),
headers: <String, String>{
'Content-Type': 'application/json; charset=UTF-8',
},
body: jsonEncode(<String, String>{
'title': 'Flutter Networking',
'body': 'This is an example of POST request.',
'userId': '1',
}),
);
if (response.statusCode == 201) {
print('Data posted successfully');
} else {
throw Exception('Failed to post data');
}
}
This example demonstrates how to send data to a server using a POST request. It includes JSON encoding to properly format the data.
Networking is useful in several scenarios. Here are some common use cases for HTTP networking in Flutter:
1. Fetching Data from an API
One of the most common uses of networking is fetching data from a REST API. Whether you're developing a social media app, an e-commerce app, or a weather app, you’ll likely need to retrieve data from a server.
2. Sending Data to a Server (POST Requests)
Sometimes you need to send data from your app to a server. For example, when a user fills out a form or submits information, the data needs to be sent to a backend server. Here's how you can perform an HTTP POST request in Flutter:
How do I fetch data from a REST API in Flutter?
How do I send data using HTTP POST in Flutter?
Future<void> sendData() async {
final response = await http.post(
Uri.parse('https://jsonplaceholder.typicode.com/posts'),
headers: <String, String>{
'Content-Type': 'application/json; charset=UTF-8',
},
body: jsonEncode(<String, String>{
'title': 'Flutter Networking',
'body': 'This is an example of POST request.',
'userId': '1',
}),
);
if (response.statusCode == 201) {
print('Data posted successfully');
} else {
throw Exception('Failed to post data');
}
}
This example demonstrates how to send data to a server using a POST request. It includes JSON encoding to properly format the data.
Code Samples for HTTP Networking in Flutter
Code Samples for HTTP Networking in Flutter
Code Samples for HTTP Networking in Flutter
Code Samples for HTTP Networking in Flutter
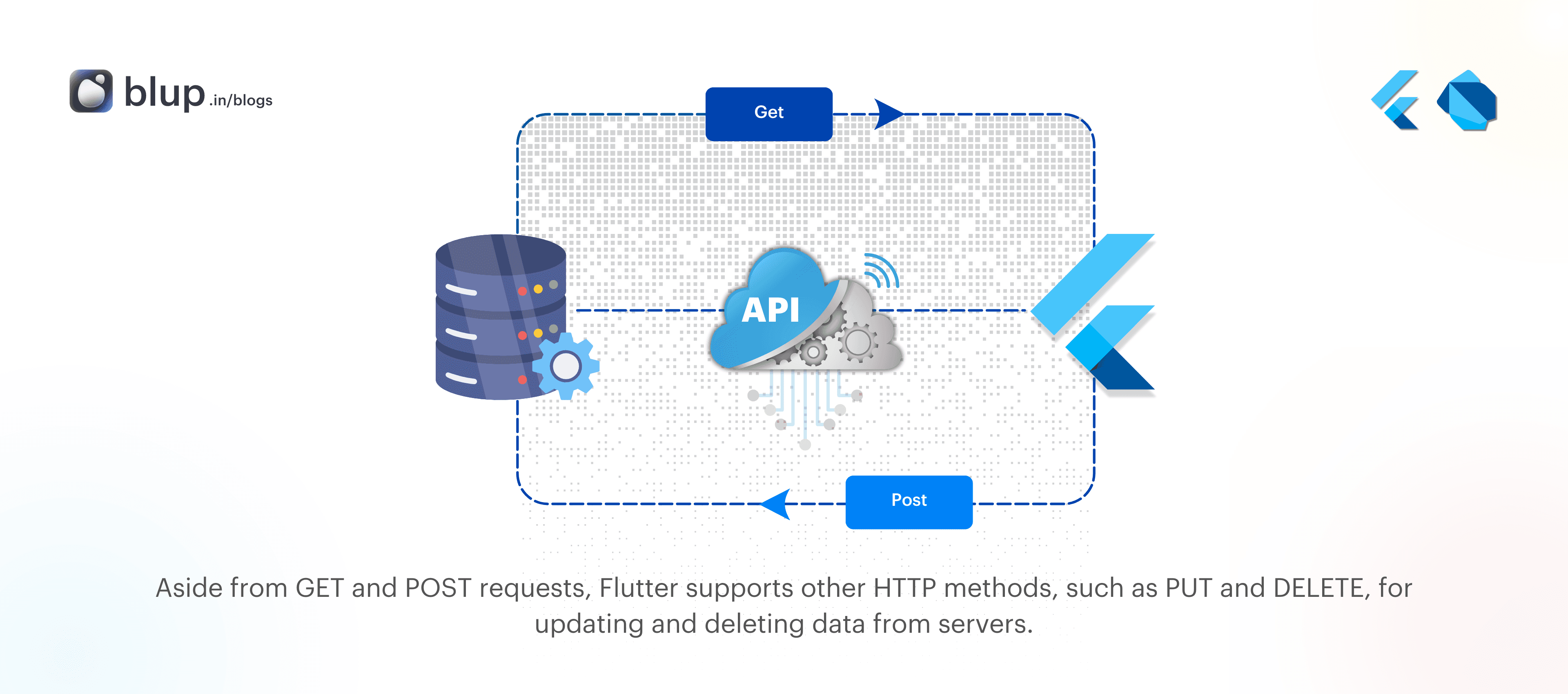
Aside from GET and POST requests, Flutter supports other HTTP methods, such as PUT and DELETE, for updating and deleting data from servers.
HTTP PUT Request
A PUT request updates existing data on the server. Here’s a sample code:
How do I update data with HTTP PUT in Flutter?
Future<void> updateData() async {
final response = await http.put(
Uri.parse('https://jsonplaceholder.typicode.com/posts/1'),
headers: <String, String>{
'Content-Type': 'application/json; charset=UTF-8',
},
body: jsonEncode(<String, String>{
'title': 'Updated Title',
}),
);
if (response.statusCode == 200) {
print('Data updated successfully');
} else {
throw Exception('Failed to update data');
}
}
HTTP DELETE Request
To delete data from a server, you can use the DELETE method:
How do I delete data using HTTP DELETE in Flutter?
Future<void> deleteData() async {
final response = await http.delete(
Uri.parse('https://jsonplaceholder.typicode.com/posts/1'));
if (response.statusCode == 200) {
print('Data deleted successfully');
} else {
throw Exception('Failed to delete data');
}
}
These code samples demonstrate how easy it is to integrate different HTTP methods in your Flutter app.
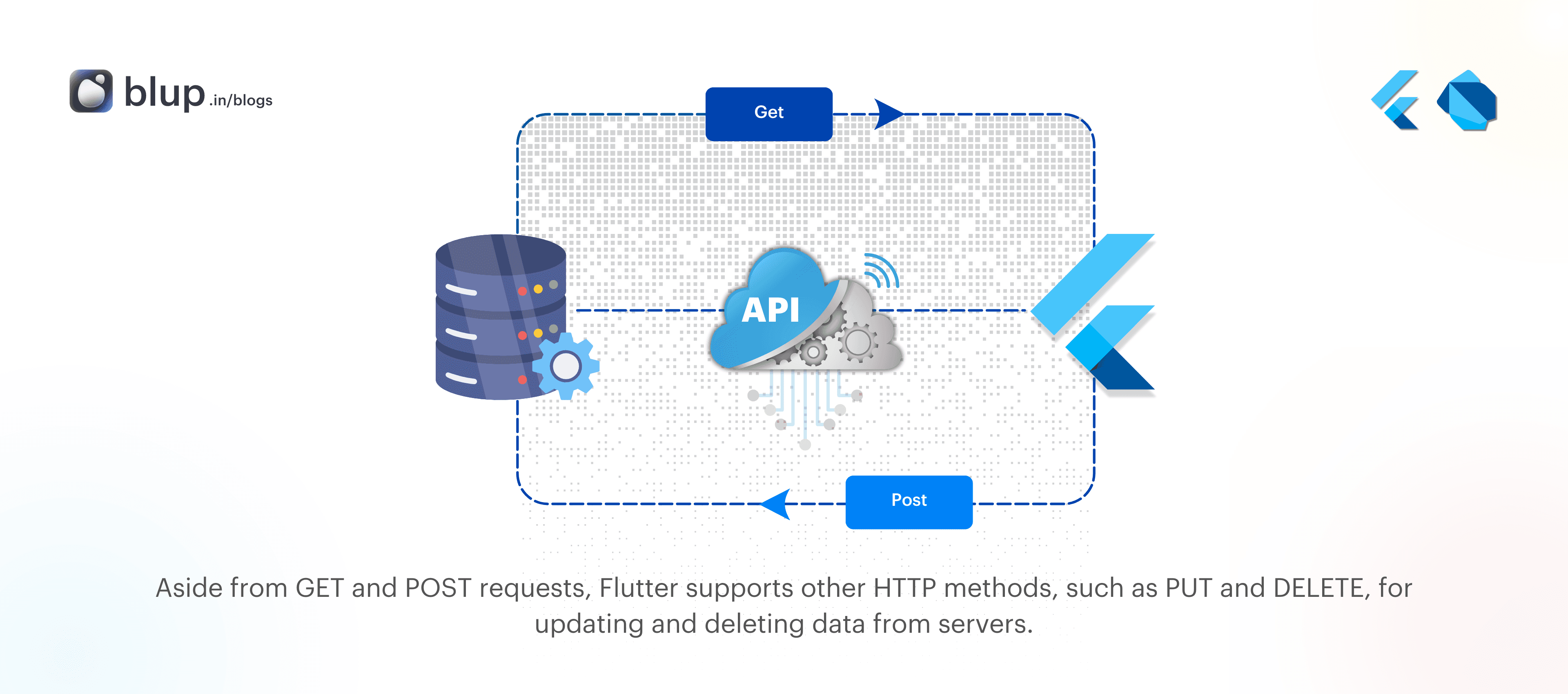
Aside from GET and POST requests, Flutter supports other HTTP methods, such as PUT and DELETE, for updating and deleting data from servers.
HTTP PUT Request
A PUT request updates existing data on the server. Here’s a sample code:
How do I update data with HTTP PUT in Flutter?
Future<void> updateData() async {
final response = await http.put(
Uri.parse('https://jsonplaceholder.typicode.com/posts/1'),
headers: <String, String>{
'Content-Type': 'application/json; charset=UTF-8',
},
body: jsonEncode(<String, String>{
'title': 'Updated Title',
}),
);
if (response.statusCode == 200) {
print('Data updated successfully');
} else {
throw Exception('Failed to update data');
}
}
HTTP DELETE Request
To delete data from a server, you can use the DELETE method:
How do I delete data using HTTP DELETE in Flutter?
Future<void> deleteData() async {
final response = await http.delete(
Uri.parse('https://jsonplaceholder.typicode.com/posts/1'));
if (response.statusCode == 200) {
print('Data deleted successfully');
} else {
throw Exception('Failed to delete data');
}
}
These code samples demonstrate how easy it is to integrate different HTTP methods in your Flutter app.
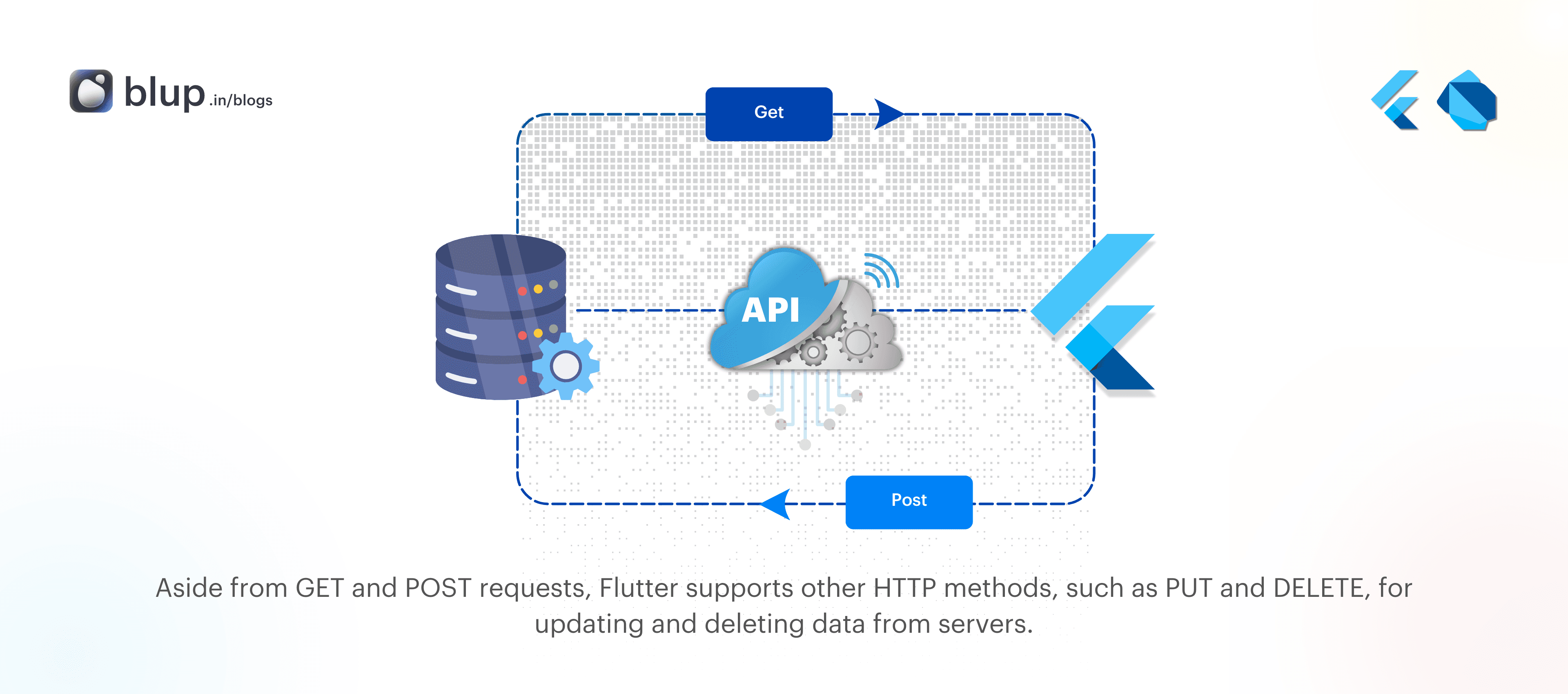
Aside from GET and POST requests, Flutter supports other HTTP methods, such as PUT and DELETE, for updating and deleting data from servers.
HTTP PUT Request
A PUT request updates existing data on the server. Here’s a sample code:
How do I update data with HTTP PUT in Flutter?
Future<void> updateData() async {
final response = await http.put(
Uri.parse('https://jsonplaceholder.typicode.com/posts/1'),
headers: <String, String>{
'Content-Type': 'application/json; charset=UTF-8',
},
body: jsonEncode(<String, String>{
'title': 'Updated Title',
}),
);
if (response.statusCode == 200) {
print('Data updated successfully');
} else {
throw Exception('Failed to update data');
}
}
HTTP DELETE Request
To delete data from a server, you can use the DELETE method:
How do I delete data using HTTP DELETE in Flutter?
Future<void> deleteData() async {
final response = await http.delete(
Uri.parse('https://jsonplaceholder.typicode.com/posts/1'));
if (response.statusCode == 200) {
print('Data deleted successfully');
} else {
throw Exception('Failed to delete data');
}
}
These code samples demonstrate how easy it is to integrate different HTTP methods in your Flutter app.
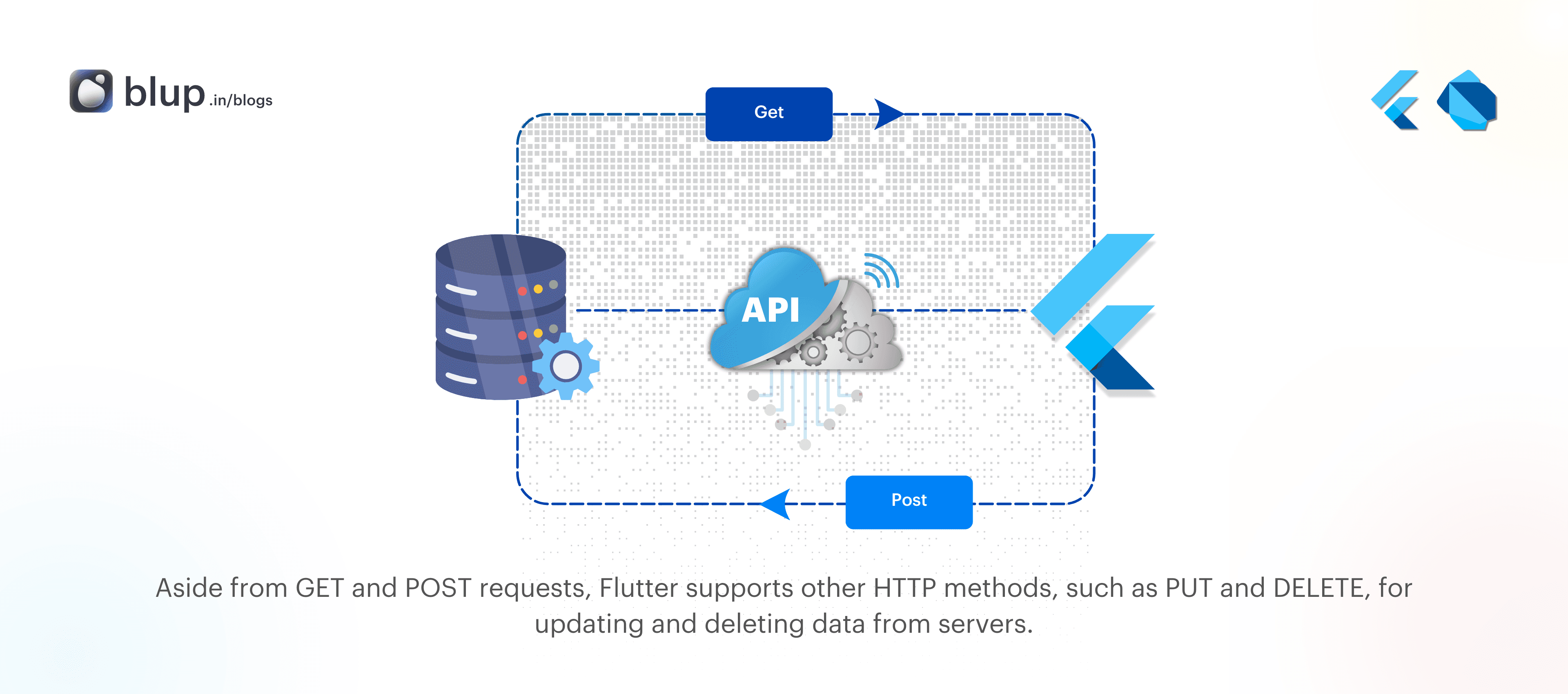
Aside from GET and POST requests, Flutter supports other HTTP methods, such as PUT and DELETE, for updating and deleting data from servers.
HTTP PUT Request
A PUT request updates existing data on the server. Here’s a sample code:
How do I update data with HTTP PUT in Flutter?
Future<void> updateData() async {
final response = await http.put(
Uri.parse('https://jsonplaceholder.typicode.com/posts/1'),
headers: <String, String>{
'Content-Type': 'application/json; charset=UTF-8',
},
body: jsonEncode(<String, String>{
'title': 'Updated Title',
}),
);
if (response.statusCode == 200) {
print('Data updated successfully');
} else {
throw Exception('Failed to update data');
}
}
HTTP DELETE Request
To delete data from a server, you can use the DELETE method:
How do I delete data using HTTP DELETE in Flutter?
Future<void> deleteData() async {
final response = await http.delete(
Uri.parse('https://jsonplaceholder.typicode.com/posts/1'));
if (response.statusCode == 200) {
print('Data deleted successfully');
} else {
throw Exception('Failed to delete data');
}
}
These code samples demonstrate how easy it is to integrate different HTTP methods in your Flutter app.
Best Practices for Cross-Platform Networking in Flutter
Best Practices for Cross-Platform Networking in Flutter
Best Practices for Cross-Platform Networking in Flutter
Best Practices for Cross-Platform Networking in Flutter
While implementing HTTP networking in Flutter is straightforward, there are several best practices you should follow to ensure optimal performance and security.
What are the best practices for HTTP networking in Flutter?
How can I optimize HTTP requests in Flutter apps?
1. Handle Errors Gracefully
Network failures are inevitable, so it's important to handle them appropriately. Use try-catch blocks to manage exceptions and provide meaningful error messages to users.
2. Optimize API Calls
Reduce the number of unnecessary API calls by caching data when possible. You can use Flutter’s shared_preferences
package to store data locally and avoid making duplicate network requests.
3. Secure Your Requests
Always use HTTPS to encrypt your data and secure your network communication. Avoid sending sensitive data in plain text and use appropriate headers and authentication methods.
While implementing HTTP networking in Flutter is straightforward, there are several best practices you should follow to ensure optimal performance and security.
What are the best practices for HTTP networking in Flutter?
How can I optimize HTTP requests in Flutter apps?
1. Handle Errors Gracefully
Network failures are inevitable, so it's important to handle them appropriately. Use try-catch blocks to manage exceptions and provide meaningful error messages to users.
2. Optimize API Calls
Reduce the number of unnecessary API calls by caching data when possible. You can use Flutter’s shared_preferences
package to store data locally and avoid making duplicate network requests.
3. Secure Your Requests
Always use HTTPS to encrypt your data and secure your network communication. Avoid sending sensitive data in plain text and use appropriate headers and authentication methods.
While implementing HTTP networking in Flutter is straightforward, there are several best practices you should follow to ensure optimal performance and security.
What are the best practices for HTTP networking in Flutter?
How can I optimize HTTP requests in Flutter apps?
1. Handle Errors Gracefully
Network failures are inevitable, so it's important to handle them appropriately. Use try-catch blocks to manage exceptions and provide meaningful error messages to users.
2. Optimize API Calls
Reduce the number of unnecessary API calls by caching data when possible. You can use Flutter’s shared_preferences
package to store data locally and avoid making duplicate network requests.
3. Secure Your Requests
Always use HTTPS to encrypt your data and secure your network communication. Avoid sending sensitive data in plain text and use appropriate headers and authentication methods.
While implementing HTTP networking in Flutter is straightforward, there are several best practices you should follow to ensure optimal performance and security.
What are the best practices for HTTP networking in Flutter?
How can I optimize HTTP requests in Flutter apps?
1. Handle Errors Gracefully
Network failures are inevitable, so it's important to handle them appropriately. Use try-catch blocks to manage exceptions and provide meaningful error messages to users.
2. Optimize API Calls
Reduce the number of unnecessary API calls by caching data when possible. You can use Flutter’s shared_preferences
package to store data locally and avoid making duplicate network requests.
3. Secure Your Requests
Always use HTTPS to encrypt your data and secure your network communication. Avoid sending sensitive data in plain text and use appropriate headers and authentication methods.
Conclusion
Conclusion
Conclusion
Conclusion
Networking is a crucial part of developing any mobile app, and Flutter makes it easy to implement HTTP networking across platforms. Whether you’re fetching data from an API, sending data to a server, or handling platform-specific issues, Flutter’s tools make the process simple and efficient.
By following best practices for handling errors, optimizing requests, and securing data, you can ensure that your app’s networking functionality is both robust and reliable. With the right code structure and cross-platform support, Flutter gives developers the flexibility they need to create dynamic and responsive apps.
Networking is a crucial part of developing any mobile app, and Flutter makes it easy to implement HTTP networking across platforms. Whether you’re fetching data from an API, sending data to a server, or handling platform-specific issues, Flutter’s tools make the process simple and efficient.
By following best practices for handling errors, optimizing requests, and securing data, you can ensure that your app’s networking functionality is both robust and reliable. With the right code structure and cross-platform support, Flutter gives developers the flexibility they need to create dynamic and responsive apps.
Networking is a crucial part of developing any mobile app, and Flutter makes it easy to implement HTTP networking across platforms. Whether you’re fetching data from an API, sending data to a server, or handling platform-specific issues, Flutter’s tools make the process simple and efficient.
By following best practices for handling errors, optimizing requests, and securing data, you can ensure that your app’s networking functionality is both robust and reliable. With the right code structure and cross-platform support, Flutter gives developers the flexibility they need to create dynamic and responsive apps.
Networking is a crucial part of developing any mobile app, and Flutter makes it easy to implement HTTP networking across platforms. Whether you’re fetching data from an API, sending data to a server, or handling platform-specific issues, Flutter’s tools make the process simple and efficient.
By following best practices for handling errors, optimizing requests, and securing data, you can ensure that your app’s networking functionality is both robust and reliable. With the right code structure and cross-platform support, Flutter gives developers the flexibility they need to create dynamic and responsive apps.
Table of content
India(HQ)
9th Floor, Tower C, Logix Cyber Park,
C Block, Phase 2, Industrial Area, Sector 62, Noida, Uttar Pradesh, 201309
USA
2081 Center Street Berkeley,
CA 94704
© 2021-23 Blupx Private Limited.
All rights reserved.
India(HQ)
9th Floor, Tower C, Logix Cyber Park,
C Block, Phase 2, Industrial Area, Sector 62, Noida, Uttar Pradesh, 201309
USA
2081 Center Street Berkeley,
CA 94704
© 2021-23 Blupx Private Limited.
All rights reserved.
India(HQ)
9th Floor, Tower C, Logix Cyber Park,
C Block, Phase 2, Industrial Area, Sector 62, Noida, Uttar Pradesh, 201309
USA
2081 Center Street Berkeley,
CA 94704
© 2021-23 Blupx Private Limited.
All rights reserved.