Firebase Authentication in Flutter: Secure User Access
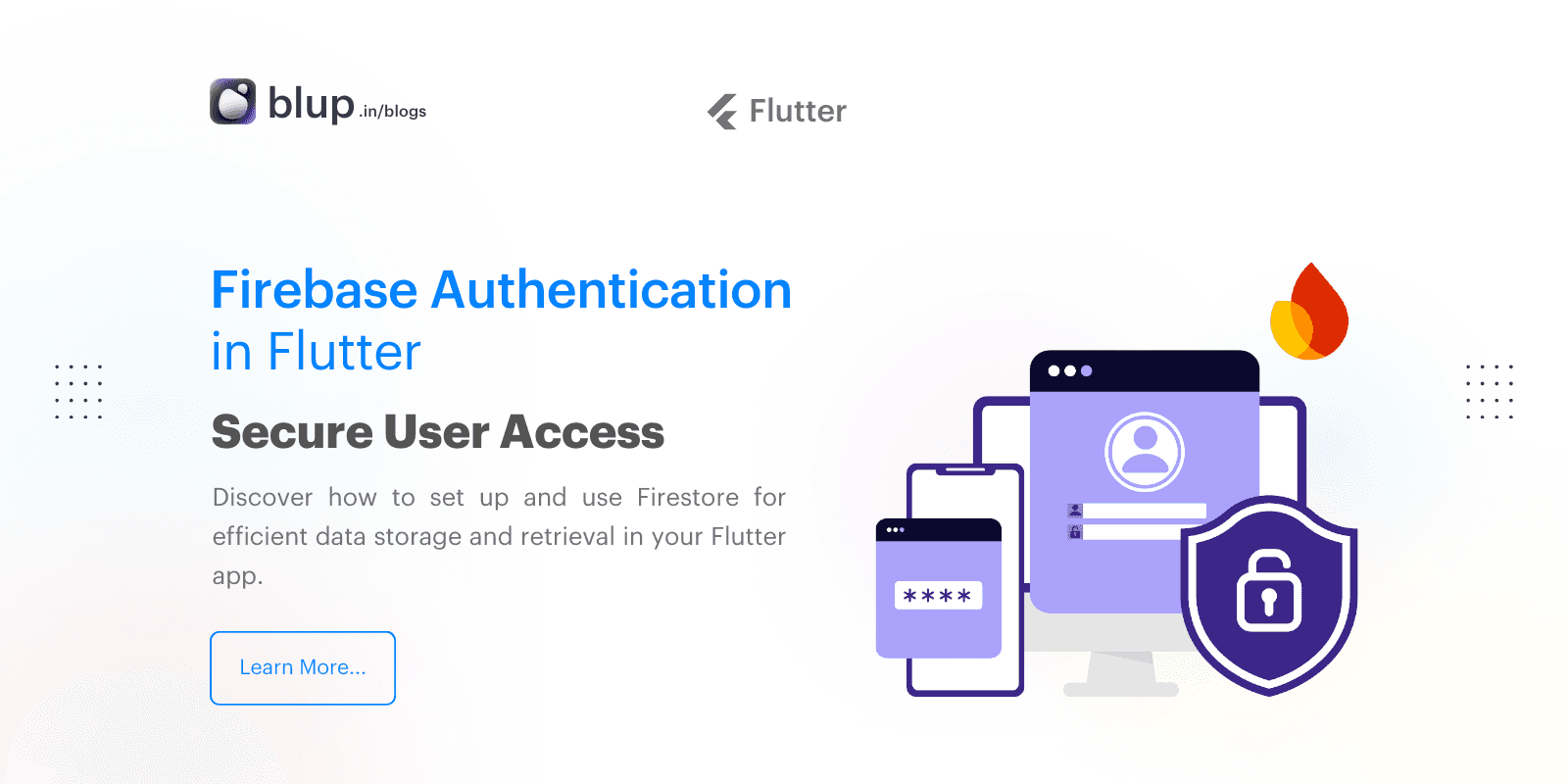
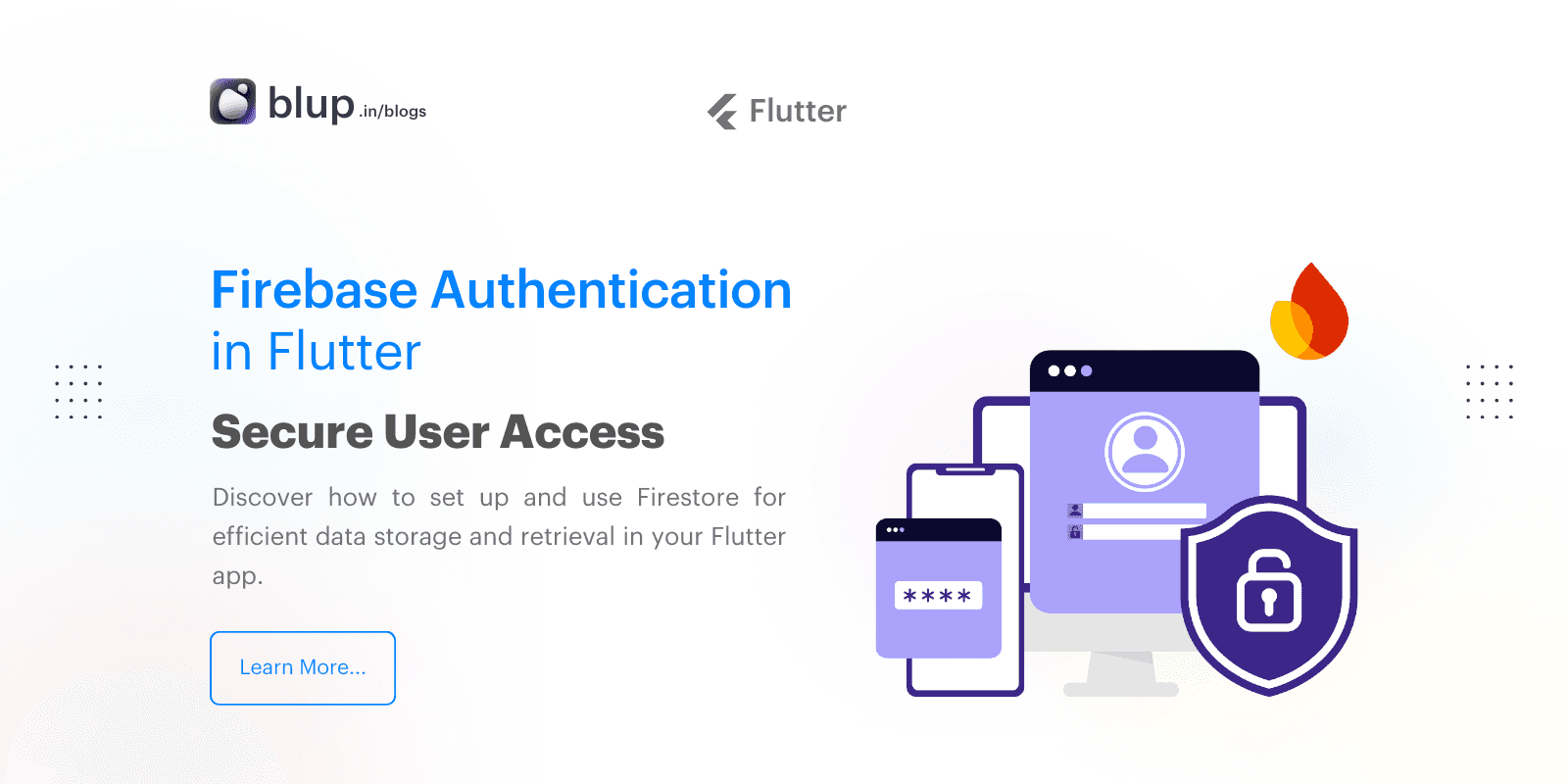
Introduction
Introduction
Introduction
Introduction
In our previous blogs on Firebase for Flutter, we explored how to set up Firebase and manage Firestore data in your Flutter apps. If you haven’t read them yet, check out Getting Started with Firebase for Flutter Development and Firestore Basics: Storing and Retrieving Data in Flutter to get the foundation you need. Building on that knowledge, this blog dives into Firebase Authentication, a critical tool for securing your Flutter apps and providing users with safe and reliable access options.
Firebase Authentication offers several login methods, from classic email/password to social sign-ins like Google, and even phone-based authentication. We’ll go over these methods in detail and offer practical steps for setting up Firebase Authentication in your Flutter app, creating a secure and seamless login experience for users.
In our previous blogs on Firebase for Flutter, we explored how to set up Firebase and manage Firestore data in your Flutter apps. If you haven’t read them yet, check out Getting Started with Firebase for Flutter Development and Firestore Basics: Storing and Retrieving Data in Flutter to get the foundation you need. Building on that knowledge, this blog dives into Firebase Authentication, a critical tool for securing your Flutter apps and providing users with safe and reliable access options.
Firebase Authentication offers several login methods, from classic email/password to social sign-ins like Google, and even phone-based authentication. We’ll go over these methods in detail and offer practical steps for setting up Firebase Authentication in your Flutter app, creating a secure and seamless login experience for users.
In our previous blogs on Firebase for Flutter, we explored how to set up Firebase and manage Firestore data in your Flutter apps. If you haven’t read them yet, check out Getting Started with Firebase for Flutter Development and Firestore Basics: Storing and Retrieving Data in Flutter to get the foundation you need. Building on that knowledge, this blog dives into Firebase Authentication, a critical tool for securing your Flutter apps and providing users with safe and reliable access options.
Firebase Authentication offers several login methods, from classic email/password to social sign-ins like Google, and even phone-based authentication. We’ll go over these methods in detail and offer practical steps for setting up Firebase Authentication in your Flutter app, creating a secure and seamless login experience for users.
In our previous blogs on Firebase for Flutter, we explored how to set up Firebase and manage Firestore data in your Flutter apps. If you haven’t read them yet, check out Getting Started with Firebase for Flutter Development and Firestore Basics: Storing and Retrieving Data in Flutter to get the foundation you need. Building on that knowledge, this blog dives into Firebase Authentication, a critical tool for securing your Flutter apps and providing users with safe and reliable access options.
Firebase Authentication offers several login methods, from classic email/password to social sign-ins like Google, and even phone-based authentication. We’ll go over these methods in detail and offer practical steps for setting up Firebase Authentication in your Flutter app, creating a secure and seamless login experience for users.
Why Use Firebase Authentication?
Why Use Firebase Authentication?
Why Use Firebase Authentication?
Why Use Firebase Authentication?
Firebase Authentication is a flexible and secure solution designed for developers. It abstracts away the complexities of implementing secure logins by handling many of the critical security aspects. Here's why Firebase Authentication stands out:
Multiple Sign-in Options: Support for email/password, Google, phone authentication, and other providers like Facebook and Twitter.
High-Level Security: Firebase complies with standards like OAuth 2.0, OpenID Connect, and offers token-based authentication.
Scalability and Reliability: Firebase Authentication is built on Google's infrastructure, ensuring it scales with your app’s growth.
User Management: Firebase makes it easy to handle user sessions, password resets, and account linking.
Firebase Authentication is a flexible and secure solution designed for developers. It abstracts away the complexities of implementing secure logins by handling many of the critical security aspects. Here's why Firebase Authentication stands out:
Multiple Sign-in Options: Support for email/password, Google, phone authentication, and other providers like Facebook and Twitter.
High-Level Security: Firebase complies with standards like OAuth 2.0, OpenID Connect, and offers token-based authentication.
Scalability and Reliability: Firebase Authentication is built on Google's infrastructure, ensuring it scales with your app’s growth.
User Management: Firebase makes it easy to handle user sessions, password resets, and account linking.
Firebase Authentication is a flexible and secure solution designed for developers. It abstracts away the complexities of implementing secure logins by handling many of the critical security aspects. Here's why Firebase Authentication stands out:
Multiple Sign-in Options: Support for email/password, Google, phone authentication, and other providers like Facebook and Twitter.
High-Level Security: Firebase complies with standards like OAuth 2.0, OpenID Connect, and offers token-based authentication.
Scalability and Reliability: Firebase Authentication is built on Google's infrastructure, ensuring it scales with your app’s growth.
User Management: Firebase makes it easy to handle user sessions, password resets, and account linking.
Firebase Authentication is a flexible and secure solution designed for developers. It abstracts away the complexities of implementing secure logins by handling many of the critical security aspects. Here's why Firebase Authentication stands out:
Multiple Sign-in Options: Support for email/password, Google, phone authentication, and other providers like Facebook and Twitter.
High-Level Security: Firebase complies with standards like OAuth 2.0, OpenID Connect, and offers token-based authentication.
Scalability and Reliability: Firebase Authentication is built on Google's infrastructure, ensuring it scales with your app’s growth.
User Management: Firebase makes it easy to handle user sessions, password resets, and account linking.
Getting Started with Firebase Authentication in Flutter
Getting Started with Firebase Authentication in Flutter
Getting Started with Firebase Authentication in Flutter
Getting Started with Firebase Authentication in Flutter
To begin using Firebase Authentication, you'll need to set up Firebase in your Flutter project. This setup process includes enabling authentication methods in the Firebase Console and configuring your Flutter app to use Firebase.
Step 1: Set Up Firebase Authentication
Create a Firebase Project: Go to the Firebase Console, and create a new project for your app if you haven’t already.
Enable Authentication Methods: In your Firebase Console, navigate to Authentication > Sign-in method. Enable the authentication providers you wish to use, such as Email/Password, Google, or Phone.
Add Firebase to Your Flutter App: Follow Firebase’s instructions to add your app to the Firebase project and update your
android
,ios
, andlib
directories with the appropriate Firebase configurations.
Step 2: Install Firebase Authentication in Your Flutter Project
Add the firebase_auth
package to your pubspec.yaml
:
Run flutter pub get
to install the package.
Step 3: Initialize Firebase in Your Flutter App
In your app’s main file, import Firebase and initialize it:
To begin using Firebase Authentication, you'll need to set up Firebase in your Flutter project. This setup process includes enabling authentication methods in the Firebase Console and configuring your Flutter app to use Firebase.
Step 1: Set Up Firebase Authentication
Create a Firebase Project: Go to the Firebase Console, and create a new project for your app if you haven’t already.
Enable Authentication Methods: In your Firebase Console, navigate to Authentication > Sign-in method. Enable the authentication providers you wish to use, such as Email/Password, Google, or Phone.
Add Firebase to Your Flutter App: Follow Firebase’s instructions to add your app to the Firebase project and update your
android
,ios
, andlib
directories with the appropriate Firebase configurations.
Step 2: Install Firebase Authentication in Your Flutter Project
Add the firebase_auth
package to your pubspec.yaml
:
Run flutter pub get
to install the package.
Step 3: Initialize Firebase in Your Flutter App
In your app’s main file, import Firebase and initialize it:
To begin using Firebase Authentication, you'll need to set up Firebase in your Flutter project. This setup process includes enabling authentication methods in the Firebase Console and configuring your Flutter app to use Firebase.
Step 1: Set Up Firebase Authentication
Create a Firebase Project: Go to the Firebase Console, and create a new project for your app if you haven’t already.
Enable Authentication Methods: In your Firebase Console, navigate to Authentication > Sign-in method. Enable the authentication providers you wish to use, such as Email/Password, Google, or Phone.
Add Firebase to Your Flutter App: Follow Firebase’s instructions to add your app to the Firebase project and update your
android
,ios
, andlib
directories with the appropriate Firebase configurations.
Step 2: Install Firebase Authentication in Your Flutter Project
Add the firebase_auth
package to your pubspec.yaml
:
Run flutter pub get
to install the package.
Step 3: Initialize Firebase in Your Flutter App
In your app’s main file, import Firebase and initialize it:
To begin using Firebase Authentication, you'll need to set up Firebase in your Flutter project. This setup process includes enabling authentication methods in the Firebase Console and configuring your Flutter app to use Firebase.
Step 1: Set Up Firebase Authentication
Create a Firebase Project: Go to the Firebase Console, and create a new project for your app if you haven’t already.
Enable Authentication Methods: In your Firebase Console, navigate to Authentication > Sign-in method. Enable the authentication providers you wish to use, such as Email/Password, Google, or Phone.
Add Firebase to Your Flutter App: Follow Firebase’s instructions to add your app to the Firebase project and update your
android
,ios
, andlib
directories with the appropriate Firebase configurations.
Step 2: Install Firebase Authentication in Your Flutter Project
Add the firebase_auth
package to your pubspec.yaml
:
Run flutter pub get
to install the package.
Step 3: Initialize Firebase in Your Flutter App
In your app’s main file, import Firebase and initialize it:
Implementing Email and Password Authentication
Implementing Email and Password Authentication
Implementing Email and Password Authentication
Implementing Email and Password Authentication
Email and password authentication is one of the simplest and most common ways to secure access to an app. Here’s how to add this feature to your Flutter app.
1. Registering a New User with Email and Password
To create a new account, use Firebase Authentication's createUserWithEmailAndPassword
method:
Future<void>
2. Logging In with Email and Password
For an existing user to log in, use the signInWithEmailAndPassword
method:
Future<void>
3. Logging Out
To log a user out, use the signOut
method:
dartCopy codeFuture<void>
Email and password authentication is one of the simplest and most common ways to secure access to an app. Here’s how to add this feature to your Flutter app.
1. Registering a New User with Email and Password
To create a new account, use Firebase Authentication's createUserWithEmailAndPassword
method:
Future<void>
2. Logging In with Email and Password
For an existing user to log in, use the signInWithEmailAndPassword
method:
Future<void>
3. Logging Out
To log a user out, use the signOut
method:
dartCopy codeFuture<void>
Email and password authentication is one of the simplest and most common ways to secure access to an app. Here’s how to add this feature to your Flutter app.
1. Registering a New User with Email and Password
To create a new account, use Firebase Authentication's createUserWithEmailAndPassword
method:
Future<void>
2. Logging In with Email and Password
For an existing user to log in, use the signInWithEmailAndPassword
method:
Future<void>
3. Logging Out
To log a user out, use the signOut
method:
dartCopy codeFuture<void>
Email and password authentication is one of the simplest and most common ways to secure access to an app. Here’s how to add this feature to your Flutter app.
1. Registering a New User with Email and Password
To create a new account, use Firebase Authentication's createUserWithEmailAndPassword
method:
Future<void>
2. Logging In with Email and Password
For an existing user to log in, use the signInWithEmailAndPassword
method:
Future<void>
3. Logging Out
To log a user out, use the signOut
method:
dartCopy codeFuture<void>
Google Sign-In Authentication
Google Sign-In Authentication
Google Sign-In Authentication
Google Sign-In Authentication
Google Sign-In is popular due to its ease of use and security. Here’s how to integrate it with Firebase Authentication in Flutter.
1. Configure Google Sign-In on Firebase Console
Go to Authentication > Sign-in method in the Firebase Console, and enable Google.
2. Add Google Sign-In Package
Add google_sign_in
to your pubspec.yaml
:
3. Implement Google Sign-In in Flutter
Import the necessary packages and set up a function to handle the Google sign-in process:
import 'package:google_sign_in/google_sign_in.dart';
Future<void>
Google Sign-In is popular due to its ease of use and security. Here’s how to integrate it with Firebase Authentication in Flutter.
1. Configure Google Sign-In on Firebase Console
Go to Authentication > Sign-in method in the Firebase Console, and enable Google.
2. Add Google Sign-In Package
Add google_sign_in
to your pubspec.yaml
:
3. Implement Google Sign-In in Flutter
Import the necessary packages and set up a function to handle the Google sign-in process:
import 'package:google_sign_in/google_sign_in.dart';
Future<void>
Google Sign-In is popular due to its ease of use and security. Here’s how to integrate it with Firebase Authentication in Flutter.
1. Configure Google Sign-In on Firebase Console
Go to Authentication > Sign-in method in the Firebase Console, and enable Google.
2. Add Google Sign-In Package
Add google_sign_in
to your pubspec.yaml
:
3. Implement Google Sign-In in Flutter
Import the necessary packages and set up a function to handle the Google sign-in process:
import 'package:google_sign_in/google_sign_in.dart';
Future<void>
Google Sign-In is popular due to its ease of use and security. Here’s how to integrate it with Firebase Authentication in Flutter.
1. Configure Google Sign-In on Firebase Console
Go to Authentication > Sign-in method in the Firebase Console, and enable Google.
2. Add Google Sign-In Package
Add google_sign_in
to your pubspec.yaml
:
3. Implement Google Sign-In in Flutter
Import the necessary packages and set up a function to handle the Google sign-in process:
import 'package:google_sign_in/google_sign_in.dart';
Future<void>
Phone Authentication
Phone Authentication
Phone Authentication
Phone Authentication
Phone number authentication is another widely used method, particularly in mobile apps where SMS-based verification is preferred.
1. Enable Phone Sign-In in Firebase Console
In the Firebase Console, navigate to Authentication > Sign-in method, and enable Phone.
2. Request Phone Verification Code
Future<void>
3. Sign In with the Verification Code
Future<void>
Phone number authentication is another widely used method, particularly in mobile apps where SMS-based verification is preferred.
1. Enable Phone Sign-In in Firebase Console
In the Firebase Console, navigate to Authentication > Sign-in method, and enable Phone.
2. Request Phone Verification Code
Future<void>
3. Sign In with the Verification Code
Future<void>
Phone number authentication is another widely used method, particularly in mobile apps where SMS-based verification is preferred.
1. Enable Phone Sign-In in Firebase Console
In the Firebase Console, navigate to Authentication > Sign-in method, and enable Phone.
2. Request Phone Verification Code
Future<void>
3. Sign In with the Verification Code
Future<void>
Phone number authentication is another widely used method, particularly in mobile apps where SMS-based verification is preferred.
1. Enable Phone Sign-In in Firebase Console
In the Firebase Console, navigate to Authentication > Sign-in method, and enable Phone.
2. Request Phone Verification Code
Future<void>
3. Sign In with the Verification Code
Future<void>
User Authentication Management
User Authentication Management
User Authentication Management
User Authentication Management
Firebase Authentication offers powerful features for managing user sessions, such as tracking the current user, password reset, and account deletion.
1. Check If User Is Signed In
2. Password Reset
Future<void>
3. Account Deletion
Future<void>
Firebase Authentication offers powerful features for managing user sessions, such as tracking the current user, password reset, and account deletion.
1. Check If User Is Signed In
2. Password Reset
Future<void>
3. Account Deletion
Future<void>
Firebase Authentication offers powerful features for managing user sessions, such as tracking the current user, password reset, and account deletion.
1. Check If User Is Signed In
2. Password Reset
Future<void>
3. Account Deletion
Future<void>
Firebase Authentication offers powerful features for managing user sessions, such as tracking the current user, password reset, and account deletion.
1. Check If User Is Signed In
2. Password Reset
Future<void>
3. Account Deletion
Future<void>
FAQs
FAQs
FAQs
FAQs
Q1: Can I use multiple authentication methods with Firebase in one app?
Yes, Firebase Authentication allows you to use multiple sign-in providers. You can enable email, Google, and phone authentication simultaneously to provide users with more choices.
Q2: Is Firebase Authentication free to use?
Firebase Authentication is free within generous limits. Beyond a certain usage, costs may apply, particularly if you use phone authentication, which incurs a cost per SMS sent.
Q3: How can I ensure data security with Firebase Authentication?
Firebase Authentication uses industry-standard protocols like OAuth 2.0 and token-based authentication to secure user data. Ensure you’re using Firebase Security Rules to restrict access to sensitive information in Firestore or Realtime Database.
Q4: Can I customize the UI of Firebase Authentication screens?
Firebase Authentication does not provide a UI by default. You have the flexibility to create custom login forms or integrate Firebase’s UI packages like firebase_ui_auth
for predefined screens.
Q1: Can I use multiple authentication methods with Firebase in one app?
Yes, Firebase Authentication allows you to use multiple sign-in providers. You can enable email, Google, and phone authentication simultaneously to provide users with more choices.
Q2: Is Firebase Authentication free to use?
Firebase Authentication is free within generous limits. Beyond a certain usage, costs may apply, particularly if you use phone authentication, which incurs a cost per SMS sent.
Q3: How can I ensure data security with Firebase Authentication?
Firebase Authentication uses industry-standard protocols like OAuth 2.0 and token-based authentication to secure user data. Ensure you’re using Firebase Security Rules to restrict access to sensitive information in Firestore or Realtime Database.
Q4: Can I customize the UI of Firebase Authentication screens?
Firebase Authentication does not provide a UI by default. You have the flexibility to create custom login forms or integrate Firebase’s UI packages like firebase_ui_auth
for predefined screens.
Q1: Can I use multiple authentication methods with Firebase in one app?
Yes, Firebase Authentication allows you to use multiple sign-in providers. You can enable email, Google, and phone authentication simultaneously to provide users with more choices.
Q2: Is Firebase Authentication free to use?
Firebase Authentication is free within generous limits. Beyond a certain usage, costs may apply, particularly if you use phone authentication, which incurs a cost per SMS sent.
Q3: How can I ensure data security with Firebase Authentication?
Firebase Authentication uses industry-standard protocols like OAuth 2.0 and token-based authentication to secure user data. Ensure you’re using Firebase Security Rules to restrict access to sensitive information in Firestore or Realtime Database.
Q4: Can I customize the UI of Firebase Authentication screens?
Firebase Authentication does not provide a UI by default. You have the flexibility to create custom login forms or integrate Firebase’s UI packages like firebase_ui_auth
for predefined screens.
Q1: Can I use multiple authentication methods with Firebase in one app?
Yes, Firebase Authentication allows you to use multiple sign-in providers. You can enable email, Google, and phone authentication simultaneously to provide users with more choices.
Q2: Is Firebase Authentication free to use?
Firebase Authentication is free within generous limits. Beyond a certain usage, costs may apply, particularly if you use phone authentication, which incurs a cost per SMS sent.
Q3: How can I ensure data security with Firebase Authentication?
Firebase Authentication uses industry-standard protocols like OAuth 2.0 and token-based authentication to secure user data. Ensure you’re using Firebase Security Rules to restrict access to sensitive information in Firestore or Realtime Database.
Q4: Can I customize the UI of Firebase Authentication screens?
Firebase Authentication does not provide a UI by default. You have the flexibility to create custom login forms or integrate Firebase’s UI packages like firebase_ui_auth
for predefined screens.
Conclusion
Conclusion
Conclusion
Conclusion
Implementing Firebase Authentication in your Flutter app is an effective way to secure user access while providing flexibility with various login options. Firebase’s robust authentication system makes it easier to manage user sessions, handle login flows, and ensure secure user data management. Integrate Firebase Authentication today and elevate the security and user experience of your Flutter app.
If you’re interested in learning more about Firebase features, check out our previous posts on Getting Started with Firebase for Flutter Development and Firestore Basics: Storing and Retrieving Data in Flutter.
Implementing Firebase Authentication in your Flutter app is an effective way to secure user access while providing flexibility with various login options. Firebase’s robust authentication system makes it easier to manage user sessions, handle login flows, and ensure secure user data management. Integrate Firebase Authentication today and elevate the security and user experience of your Flutter app.
If you’re interested in learning more about Firebase features, check out our previous posts on Getting Started with Firebase for Flutter Development and Firestore Basics: Storing and Retrieving Data in Flutter.
Implementing Firebase Authentication in your Flutter app is an effective way to secure user access while providing flexibility with various login options. Firebase’s robust authentication system makes it easier to manage user sessions, handle login flows, and ensure secure user data management. Integrate Firebase Authentication today and elevate the security and user experience of your Flutter app.
If you’re interested in learning more about Firebase features, check out our previous posts on Getting Started with Firebase for Flutter Development and Firestore Basics: Storing and Retrieving Data in Flutter.
Implementing Firebase Authentication in your Flutter app is an effective way to secure user access while providing flexibility with various login options. Firebase’s robust authentication system makes it easier to manage user sessions, handle login flows, and ensure secure user data management. Integrate Firebase Authentication today and elevate the security and user experience of your Flutter app.
If you’re interested in learning more about Firebase features, check out our previous posts on Getting Started with Firebase for Flutter Development and Firestore Basics: Storing and Retrieving Data in Flutter.
Table of content
© 2021-25 Blupx Private Limited.
All rights reserved.
© 2021-25 Blupx Private Limited.
All rights reserved.
© 2021-25 Blupx Private Limited.
All rights reserved.