Flutter State Management
5 mins
5 mins

Ashutosh Rawat
Published on Sep 9, 2024
GetX in Flutter: Navigation, State Management & Dependency
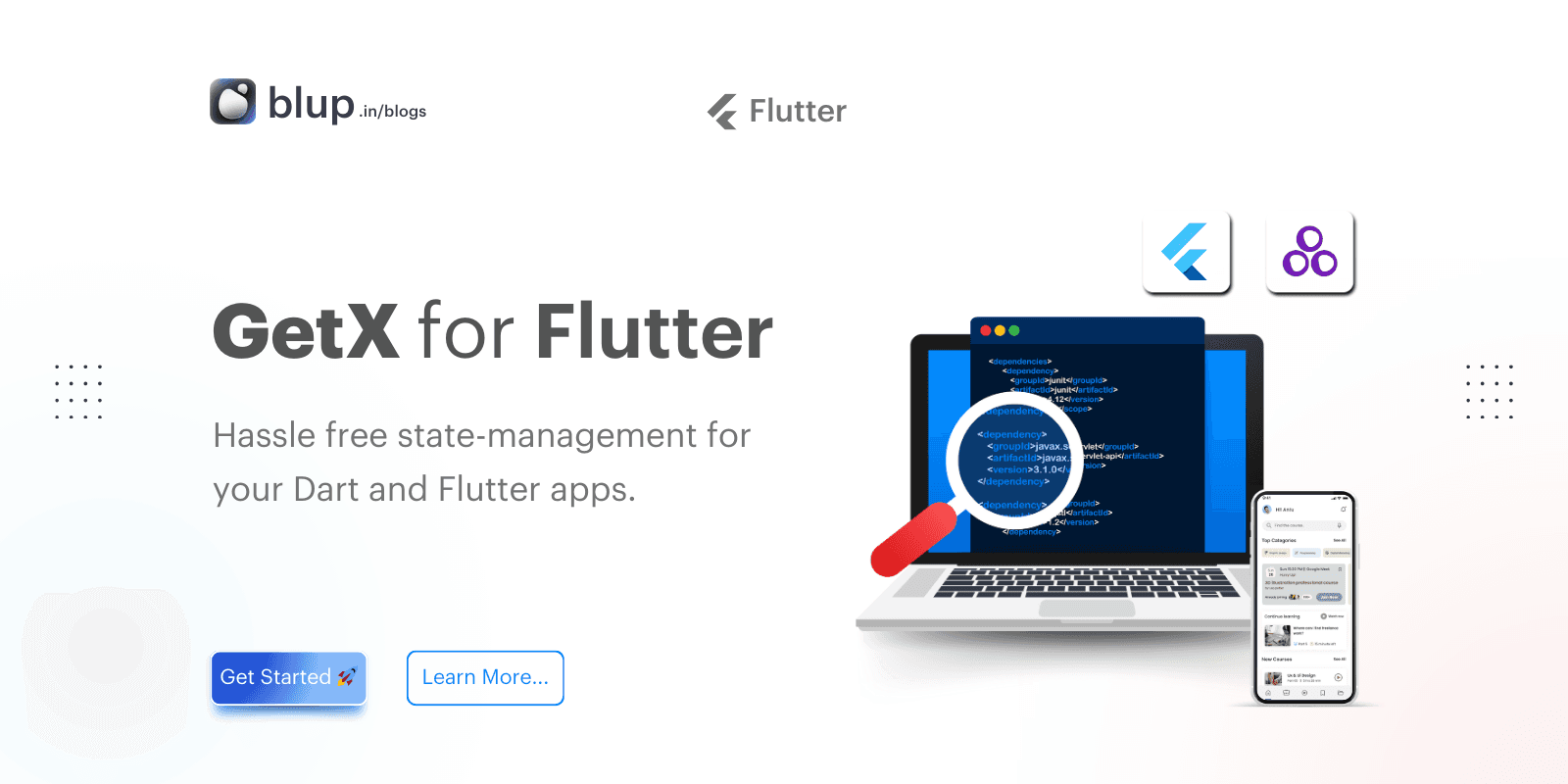
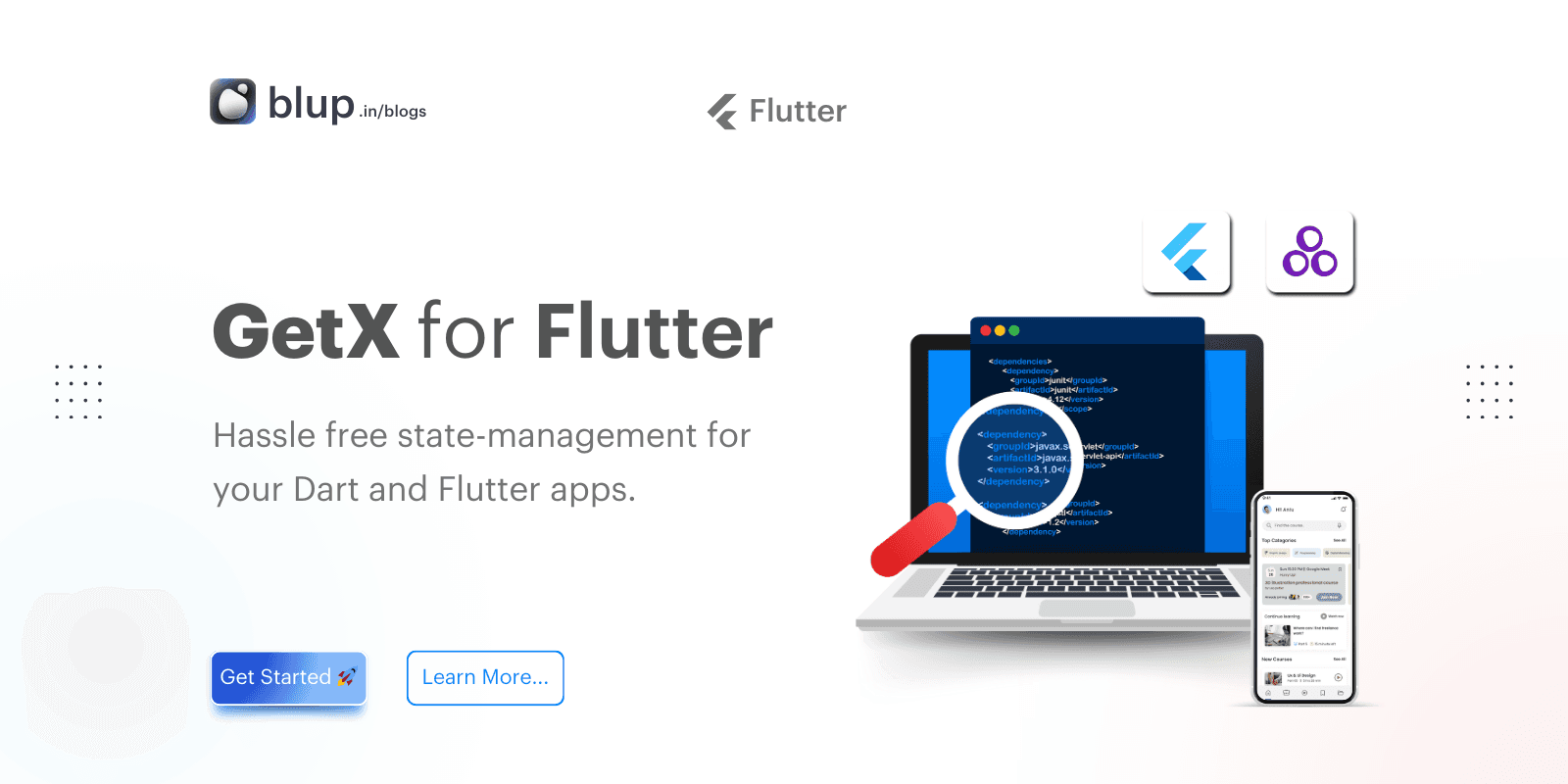
Introduction
Introduction
Introduction
Introduction
Ready to transform your Flutter development? GetX is here to revolutionize your approach with its powerful toolkit for state management, navigation, and dependency injection.
Why GetX?

Lightweight & Efficient: Minimal resource use and high performance, keeping your app responsive.
Unified Approach: Combines state management, routing, and dependency injection into one package.
Ease of Use: Simple APIs and a low learning curve make it ideal for all developers.
In this blog, discover how GetX can elevate your Flutter apps with advanced state management, seamless navigation, and efficient dependency injection. Let's level up your development!
Ready to transform your Flutter development? GetX is here to revolutionize your approach with its powerful toolkit for state management, navigation, and dependency injection.
Why GetX?

Lightweight & Efficient: Minimal resource use and high performance, keeping your app responsive.
Unified Approach: Combines state management, routing, and dependency injection into one package.
Ease of Use: Simple APIs and a low learning curve make it ideal for all developers.
In this blog, discover how GetX can elevate your Flutter apps with advanced state management, seamless navigation, and efficient dependency injection. Let's level up your development!
Ready to transform your Flutter development? GetX is here to revolutionize your approach with its powerful toolkit for state management, navigation, and dependency injection.
Why GetX?

Lightweight & Efficient: Minimal resource use and high performance, keeping your app responsive.
Unified Approach: Combines state management, routing, and dependency injection into one package.
Ease of Use: Simple APIs and a low learning curve make it ideal for all developers.
In this blog, discover how GetX can elevate your Flutter apps with advanced state management, seamless navigation, and efficient dependency injection. Let's level up your development!
Ready to transform your Flutter development? GetX is here to revolutionize your approach with its powerful toolkit for state management, navigation, and dependency injection.
Why GetX?

Lightweight & Efficient: Minimal resource use and high performance, keeping your app responsive.
Unified Approach: Combines state management, routing, and dependency injection into one package.
Ease of Use: Simple APIs and a low learning curve make it ideal for all developers.
In this blog, discover how GetX can elevate your Flutter apps with advanced state management, seamless navigation, and efficient dependency injection. Let's level up your development!
What is GetX?
What is GetX?
What is GetX?
What is GetX?
GetX is more than just a state management library for Flutter; it's a microframework that integrates state management, route management, and dependency injection into a single, efficient package. Designed to enhance the development experience, GetX combines high performance with simplicity, offering a powerful solution that's both lightweight and versatile.
Introduction to GetX and Its Capabilities
At its core, GetX operates on three fundamental principles:
Performance: GetX is engineered for optimal resource utilization. It minimizes memory and CPU consumption, ensuring your app runs smoothly even as complexity increases.
Productivity: With its intuitive syntax and efficient tooling, GetX drastically reduces development time. The straightforward approach allows developers to build and maintain applications with less boilerplate code, enhancing overall productivity.
Organization: GetX excels in decoupling business logic from the UI. You no longer need context to manage navigation between routes, and it eliminates the need for stateful widgets, streamlining your codebase.
Comparison with Other State Management Libraries
Unlike alternatives such as MobX or BLoC, GetX offers a unified solution that simplifies complex tasks. While MobX requires additional setup for reactivity and BLoC involves extensive boilerplate, GetX’s integrated approach provides a more straightforward and efficient workflow. This combination of features not only accelerates development but also optimizes app performance.
Certainly! Here’s a comparison table highlighting the key aspects of GetX, MobX, and BLoC for state management in Flutter:
table image
GetX is more than just a state management library for Flutter; it's a microframework that integrates state management, route management, and dependency injection into a single, efficient package. Designed to enhance the development experience, GetX combines high performance with simplicity, offering a powerful solution that's both lightweight and versatile.
Introduction to GetX and Its Capabilities
At its core, GetX operates on three fundamental principles:
Performance: GetX is engineered for optimal resource utilization. It minimizes memory and CPU consumption, ensuring your app runs smoothly even as complexity increases.
Productivity: With its intuitive syntax and efficient tooling, GetX drastically reduces development time. The straightforward approach allows developers to build and maintain applications with less boilerplate code, enhancing overall productivity.
Organization: GetX excels in decoupling business logic from the UI. You no longer need context to manage navigation between routes, and it eliminates the need for stateful widgets, streamlining your codebase.
Comparison with Other State Management Libraries
Unlike alternatives such as MobX or BLoC, GetX offers a unified solution that simplifies complex tasks. While MobX requires additional setup for reactivity and BLoC involves extensive boilerplate, GetX’s integrated approach provides a more straightforward and efficient workflow. This combination of features not only accelerates development but also optimizes app performance.
Certainly! Here’s a comparison table highlighting the key aspects of GetX, MobX, and BLoC for state management in Flutter:
table image
GetX is more than just a state management library for Flutter; it's a microframework that integrates state management, route management, and dependency injection into a single, efficient package. Designed to enhance the development experience, GetX combines high performance with simplicity, offering a powerful solution that's both lightweight and versatile.
Introduction to GetX and Its Capabilities
At its core, GetX operates on three fundamental principles:
Performance: GetX is engineered for optimal resource utilization. It minimizes memory and CPU consumption, ensuring your app runs smoothly even as complexity increases.
Productivity: With its intuitive syntax and efficient tooling, GetX drastically reduces development time. The straightforward approach allows developers to build and maintain applications with less boilerplate code, enhancing overall productivity.
Organization: GetX excels in decoupling business logic from the UI. You no longer need context to manage navigation between routes, and it eliminates the need for stateful widgets, streamlining your codebase.
Comparison with Other State Management Libraries
Unlike alternatives such as MobX or BLoC, GetX offers a unified solution that simplifies complex tasks. While MobX requires additional setup for reactivity and BLoC involves extensive boilerplate, GetX’s integrated approach provides a more straightforward and efficient workflow. This combination of features not only accelerates development but also optimizes app performance.
Certainly! Here’s a comparison table highlighting the key aspects of GetX, MobX, and BLoC for state management in Flutter:
table image
GetX is more than just a state management library for Flutter; it's a microframework that integrates state management, route management, and dependency injection into a single, efficient package. Designed to enhance the development experience, GetX combines high performance with simplicity, offering a powerful solution that's both lightweight and versatile.
Introduction to GetX and Its Capabilities
At its core, GetX operates on three fundamental principles:
Performance: GetX is engineered for optimal resource utilization. It minimizes memory and CPU consumption, ensuring your app runs smoothly even as complexity increases.
Productivity: With its intuitive syntax and efficient tooling, GetX drastically reduces development time. The straightforward approach allows developers to build and maintain applications with less boilerplate code, enhancing overall productivity.
Organization: GetX excels in decoupling business logic from the UI. You no longer need context to manage navigation between routes, and it eliminates the need for stateful widgets, streamlining your codebase.
Comparison with Other State Management Libraries
Unlike alternatives such as MobX or BLoC, GetX offers a unified solution that simplifies complex tasks. While MobX requires additional setup for reactivity and BLoC involves extensive boilerplate, GetX’s integrated approach provides a more straightforward and efficient workflow. This combination of features not only accelerates development but also optimizes app performance.
Certainly! Here’s a comparison table highlighting the key aspects of GetX, MobX, and BLoC for state management in Flutter:
table image
The Three Pillars of GetX
The Three Pillars of GetX
The Three Pillars of GetX
The Three Pillars of GetX
Imagine having a Flutter app that handles state, navigation, and dependencies seamlessly—GetX makes this possible with minimal fuss. Ready to elevate your Flutter development experience?
State Management: At the heart of GetX is its powerful state management system. By using
Obx
andGetBuilder
, GetX ensures that only the necessary UI components are updated, leading to a smoother and more responsive user experience. This efficiency not only simplifies your code but also enhances your app’s performance, making it ideal for both small and large applications.
Navigation: Simplifying navigation in Flutter, GetX allows you to manage routes without the cumbersome context handling of traditional methods. With commands like
Get.to()
, you can effortlessly navigate between screens, streamline your routing logic, and keep your codebase clean and maintainable.
Dependency Injection: GetX’s dependency injection system is as efficient as it is straightforward. Register dependencies with
Get.put()
and retrieve them withGet.find()
, eliminating boilerplate code and fostering a more modular and testable application structure.
Imagine having a Flutter app that handles state, navigation, and dependencies seamlessly—GetX makes this possible with minimal fuss. Ready to elevate your Flutter development experience?
State Management: At the heart of GetX is its powerful state management system. By using
Obx
andGetBuilder
, GetX ensures that only the necessary UI components are updated, leading to a smoother and more responsive user experience. This efficiency not only simplifies your code but also enhances your app’s performance, making it ideal for both small and large applications.
Navigation: Simplifying navigation in Flutter, GetX allows you to manage routes without the cumbersome context handling of traditional methods. With commands like
Get.to()
, you can effortlessly navigate between screens, streamline your routing logic, and keep your codebase clean and maintainable.
Dependency Injection: GetX’s dependency injection system is as efficient as it is straightforward. Register dependencies with
Get.put()
and retrieve them withGet.find()
, eliminating boilerplate code and fostering a more modular and testable application structure.
Imagine having a Flutter app that handles state, navigation, and dependencies seamlessly—GetX makes this possible with minimal fuss. Ready to elevate your Flutter development experience?
State Management: At the heart of GetX is its powerful state management system. By using
Obx
andGetBuilder
, GetX ensures that only the necessary UI components are updated, leading to a smoother and more responsive user experience. This efficiency not only simplifies your code but also enhances your app’s performance, making it ideal for both small and large applications.
Navigation: Simplifying navigation in Flutter, GetX allows you to manage routes without the cumbersome context handling of traditional methods. With commands like
Get.to()
, you can effortlessly navigate between screens, streamline your routing logic, and keep your codebase clean and maintainable.
Dependency Injection: GetX’s dependency injection system is as efficient as it is straightforward. Register dependencies with
Get.put()
and retrieve them withGet.find()
, eliminating boilerplate code and fostering a more modular and testable application structure.
Imagine having a Flutter app that handles state, navigation, and dependencies seamlessly—GetX makes this possible with minimal fuss. Ready to elevate your Flutter development experience?
State Management: At the heart of GetX is its powerful state management system. By using
Obx
andGetBuilder
, GetX ensures that only the necessary UI components are updated, leading to a smoother and more responsive user experience. This efficiency not only simplifies your code but also enhances your app’s performance, making it ideal for both small and large applications.
Navigation: Simplifying navigation in Flutter, GetX allows you to manage routes without the cumbersome context handling of traditional methods. With commands like
Get.to()
, you can effortlessly navigate between screens, streamline your routing logic, and keep your codebase clean and maintainable.
Dependency Injection: GetX’s dependency injection system is as efficient as it is straightforward. Register dependencies with
Get.put()
and retrieve them withGet.find()
, eliminating boilerplate code and fostering a more modular and testable application structure.
Let’s Get Going with GetX State Management
Let’s Get Going with GetX State Management
Let’s Get Going with GetX State Management
Let’s Get Going with GetX State Management
GetX offers an easier, more efficient way to handle state management, routing, and dependencies all in one place. Let’s dive into setting up GetX to streamline your development process.
Step 1: Create a New Application
To begin, set up a new Flutter project by running this command in your terminal:
flutter create getx_app
This will generate a base Flutter project that you can build on. Open the project in your favorite editor like VSCode or Android Studio.
Step 2: Add Required Dependencies
In your pubspec.yaml
, add GetX to the dependencies list:
dependencies:
flutter:
sdk: flutter
get: ^4.6.5
Next, fetch the package:
flutter pub get
Flutter GetX integrates easily into your project with no additional setup. It’s lightweight but packed with powerful features that streamline complex app functionalities.
Step 3: Update the MaterialApp Widget
To unlock GetX’s full potential, replace MaterialApp
with GetMaterialApp
. This allows for advanced features like navigation without the context
and centralized state management.
import 'package:flutter/material.dart';
import 'package:get/get.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return GetMaterialApp(
title: 'GetX Example App',
theme: ThemeData(primarySwatch: Colors.blue),
home: HomePage(),
);
}
}
By making this change, GetX takes over as your app’s core, providing built-in routing and state management capabilities with minimal configuration.
Step 4: Add GetX Controller
Controllers are essential in GetX for managing the logic and state of your app. Create a controller to handle a simple counter operation.
import 'package:get/get.dart';
class CounterController extends GetxController {
var count = 0.obs;
void increment() {
count++;
}
}
Explanation:
The variable
count
is wrapped in.obs
, which makes it observable. The observable state in GetX is reactive, meaning it can automatically notify the UI when the value changes.GetX Controllers, like
CounterController
, help you separate your business logic from your UI, following the best practices of clean architecture.
Step 5: Dependency Injection
One of the standout features of GetX is the built-in dependency injection in Flutter. You don’t need to rely on external libraries or cumbersome setup. Inject your controller with a single line of code:
void main() {
Get.put(CounterController()); // Injects the controller
runApp(MyApp());
}
This simple command makes you CounterController
globally accessible in your app. You can access it in any widget without recreating or passing it through constructors.
Step 6: Instantiate Controller
Now that your controller is injected, let’s see how you can use it inside your app’s UI. In the HomePage
, retrieve the controller instance using Get.find()
:
class HomePage extends StatelessWidget {
final CounterController controller = Get.find();
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(title: Text('GetX Counter Example')),
body: Center(
child: Obx(() => Text('Count: ${controller.count}')),
),
floatingActionButton: FloatingActionButton(
onPressed: controller.increment,
child: Icon(Icons.add),
),
);
}
}
By calling Get.find()
, you can retrieve the controller and manage its state directly within your widget. This makes the UI cleaner and reduces the need for manual state management.
Step 7: Obx Widget (Observer)
The Obx
widget is GetX’s answer to updating the UI reactively. It listens to changes in observable variables and rebuilds only the part of the UI that depends on them.
Obx(() => Text('Count: ${controller.count}')),
Every time the count
variable is incremented, the text widget inside Obx
will automatically update. There’s no need for setState()
other traditional methods.
GetX offers an easier, more efficient way to handle state management, routing, and dependencies all in one place. Let’s dive into setting up GetX to streamline your development process.
Step 1: Create a New Application
To begin, set up a new Flutter project by running this command in your terminal:
flutter create getx_app
This will generate a base Flutter project that you can build on. Open the project in your favorite editor like VSCode or Android Studio.
Step 2: Add Required Dependencies
In your pubspec.yaml
, add GetX to the dependencies list:
dependencies:
flutter:
sdk: flutter
get: ^4.6.5
Next, fetch the package:
flutter pub get
Flutter GetX integrates easily into your project with no additional setup. It’s lightweight but packed with powerful features that streamline complex app functionalities.
Step 3: Update the MaterialApp Widget
To unlock GetX’s full potential, replace MaterialApp
with GetMaterialApp
. This allows for advanced features like navigation without the context
and centralized state management.
import 'package:flutter/material.dart';
import 'package:get/get.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return GetMaterialApp(
title: 'GetX Example App',
theme: ThemeData(primarySwatch: Colors.blue),
home: HomePage(),
);
}
}
By making this change, GetX takes over as your app’s core, providing built-in routing and state management capabilities with minimal configuration.
Step 4: Add GetX Controller
Controllers are essential in GetX for managing the logic and state of your app. Create a controller to handle a simple counter operation.
import 'package:get/get.dart';
class CounterController extends GetxController {
var count = 0.obs;
void increment() {
count++;
}
}
Explanation:
The variable
count
is wrapped in.obs
, which makes it observable. The observable state in GetX is reactive, meaning it can automatically notify the UI when the value changes.GetX Controllers, like
CounterController
, help you separate your business logic from your UI, following the best practices of clean architecture.
Step 5: Dependency Injection
One of the standout features of GetX is the built-in dependency injection in Flutter. You don’t need to rely on external libraries or cumbersome setup. Inject your controller with a single line of code:
void main() {
Get.put(CounterController()); // Injects the controller
runApp(MyApp());
}
This simple command makes you CounterController
globally accessible in your app. You can access it in any widget without recreating or passing it through constructors.
Step 6: Instantiate Controller
Now that your controller is injected, let’s see how you can use it inside your app’s UI. In the HomePage
, retrieve the controller instance using Get.find()
:
class HomePage extends StatelessWidget {
final CounterController controller = Get.find();
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(title: Text('GetX Counter Example')),
body: Center(
child: Obx(() => Text('Count: ${controller.count}')),
),
floatingActionButton: FloatingActionButton(
onPressed: controller.increment,
child: Icon(Icons.add),
),
);
}
}
By calling Get.find()
, you can retrieve the controller and manage its state directly within your widget. This makes the UI cleaner and reduces the need for manual state management.
Step 7: Obx Widget (Observer)
The Obx
widget is GetX’s answer to updating the UI reactively. It listens to changes in observable variables and rebuilds only the part of the UI that depends on them.
Obx(() => Text('Count: ${controller.count}')),
Every time the count
variable is incremented, the text widget inside Obx
will automatically update. There’s no need for setState()
other traditional methods.
GetX offers an easier, more efficient way to handle state management, routing, and dependencies all in one place. Let’s dive into setting up GetX to streamline your development process.
Step 1: Create a New Application
To begin, set up a new Flutter project by running this command in your terminal:
flutter create getx_app
This will generate a base Flutter project that you can build on. Open the project in your favorite editor like VSCode or Android Studio.
Step 2: Add Required Dependencies
In your pubspec.yaml
, add GetX to the dependencies list:
dependencies:
flutter:
sdk: flutter
get: ^4.6.5
Next, fetch the package:
flutter pub get
Flutter GetX integrates easily into your project with no additional setup. It’s lightweight but packed with powerful features that streamline complex app functionalities.
Step 3: Update the MaterialApp Widget
To unlock GetX’s full potential, replace MaterialApp
with GetMaterialApp
. This allows for advanced features like navigation without the context
and centralized state management.
import 'package:flutter/material.dart';
import 'package:get/get.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return GetMaterialApp(
title: 'GetX Example App',
theme: ThemeData(primarySwatch: Colors.blue),
home: HomePage(),
);
}
}
By making this change, GetX takes over as your app’s core, providing built-in routing and state management capabilities with minimal configuration.
Step 4: Add GetX Controller
Controllers are essential in GetX for managing the logic and state of your app. Create a controller to handle a simple counter operation.
import 'package:get/get.dart';
class CounterController extends GetxController {
var count = 0.obs;
void increment() {
count++;
}
}
Explanation:
The variable
count
is wrapped in.obs
, which makes it observable. The observable state in GetX is reactive, meaning it can automatically notify the UI when the value changes.GetX Controllers, like
CounterController
, help you separate your business logic from your UI, following the best practices of clean architecture.
Step 5: Dependency Injection
One of the standout features of GetX is the built-in dependency injection in Flutter. You don’t need to rely on external libraries or cumbersome setup. Inject your controller with a single line of code:
void main() {
Get.put(CounterController()); // Injects the controller
runApp(MyApp());
}
This simple command makes you CounterController
globally accessible in your app. You can access it in any widget without recreating or passing it through constructors.
Step 6: Instantiate Controller
Now that your controller is injected, let’s see how you can use it inside your app’s UI. In the HomePage
, retrieve the controller instance using Get.find()
:
class HomePage extends StatelessWidget {
final CounterController controller = Get.find();
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(title: Text('GetX Counter Example')),
body: Center(
child: Obx(() => Text('Count: ${controller.count}')),
),
floatingActionButton: FloatingActionButton(
onPressed: controller.increment,
child: Icon(Icons.add),
),
);
}
}
By calling Get.find()
, you can retrieve the controller and manage its state directly within your widget. This makes the UI cleaner and reduces the need for manual state management.
Step 7: Obx Widget (Observer)
The Obx
widget is GetX’s answer to updating the UI reactively. It listens to changes in observable variables and rebuilds only the part of the UI that depends on them.
Obx(() => Text('Count: ${controller.count}')),
Every time the count
variable is incremented, the text widget inside Obx
will automatically update. There’s no need for setState()
other traditional methods.
GetX offers an easier, more efficient way to handle state management, routing, and dependencies all in one place. Let’s dive into setting up GetX to streamline your development process.
Step 1: Create a New Application
To begin, set up a new Flutter project by running this command in your terminal:
flutter create getx_app
This will generate a base Flutter project that you can build on. Open the project in your favorite editor like VSCode or Android Studio.
Step 2: Add Required Dependencies
In your pubspec.yaml
, add GetX to the dependencies list:
dependencies:
flutter:
sdk: flutter
get: ^4.6.5
Next, fetch the package:
flutter pub get
Flutter GetX integrates easily into your project with no additional setup. It’s lightweight but packed with powerful features that streamline complex app functionalities.
Step 3: Update the MaterialApp Widget
To unlock GetX’s full potential, replace MaterialApp
with GetMaterialApp
. This allows for advanced features like navigation without the context
and centralized state management.
import 'package:flutter/material.dart';
import 'package:get/get.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return GetMaterialApp(
title: 'GetX Example App',
theme: ThemeData(primarySwatch: Colors.blue),
home: HomePage(),
);
}
}
By making this change, GetX takes over as your app’s core, providing built-in routing and state management capabilities with minimal configuration.
Step 4: Add GetX Controller
Controllers are essential in GetX for managing the logic and state of your app. Create a controller to handle a simple counter operation.
import 'package:get/get.dart';
class CounterController extends GetxController {
var count = 0.obs;
void increment() {
count++;
}
}
Explanation:
The variable
count
is wrapped in.obs
, which makes it observable. The observable state in GetX is reactive, meaning it can automatically notify the UI when the value changes.GetX Controllers, like
CounterController
, help you separate your business logic from your UI, following the best practices of clean architecture.
Step 5: Dependency Injection
One of the standout features of GetX is the built-in dependency injection in Flutter. You don’t need to rely on external libraries or cumbersome setup. Inject your controller with a single line of code:
void main() {
Get.put(CounterController()); // Injects the controller
runApp(MyApp());
}
This simple command makes you CounterController
globally accessible in your app. You can access it in any widget without recreating or passing it through constructors.
Step 6: Instantiate Controller
Now that your controller is injected, let’s see how you can use it inside your app’s UI. In the HomePage
, retrieve the controller instance using Get.find()
:
class HomePage extends StatelessWidget {
final CounterController controller = Get.find();
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(title: Text('GetX Counter Example')),
body: Center(
child: Obx(() => Text('Count: ${controller.count}')),
),
floatingActionButton: FloatingActionButton(
onPressed: controller.increment,
child: Icon(Icons.add),
),
);
}
}
By calling Get.find()
, you can retrieve the controller and manage its state directly within your widget. This makes the UI cleaner and reduces the need for manual state management.
Step 7: Obx Widget (Observer)
The Obx
widget is GetX’s answer to updating the UI reactively. It listens to changes in observable variables and rebuilds only the part of the UI that depends on them.
Obx(() => Text('Count: ${controller.count}')),
Every time the count
variable is incremented, the text widget inside Obx
will automatically update. There’s no need for setState()
other traditional methods.
Value-Added Features of GetX
Value-Added Features of GetX
Value-Added Features of GetX
Value-Added Features of GetX
GetX stands out as a top Flutter state management solution due to its performance, ease of use, and strong community support.
Performance Benefits
GetX optimizes app performance by selectively rebuilding only the necessary UI components. Its lightweight nature ensures smooth performance, making it ideal for small to large-scale applications. By reducing unnecessary rebuilds, GetX ensures efficient resource usage.
Ease of Use
GetX is designed for simplicity. With minimal boilerplate and intuitive APIs, developers can easily integrate state management, navigation, and dependency injection. The unified approach speeds up development, making GetX beginner-friendly and efficient for experienced developers alike.
Community Support
GetX boasts an active and engaged community. With frequent updates, bug fixes, and extensive documentation, developers have access to a vast range of resources to help them overcome challenges and learn advanced techniques.
GetX stands out as a top Flutter state management solution due to its performance, ease of use, and strong community support.
Performance Benefits
GetX optimizes app performance by selectively rebuilding only the necessary UI components. Its lightweight nature ensures smooth performance, making it ideal for small to large-scale applications. By reducing unnecessary rebuilds, GetX ensures efficient resource usage.
Ease of Use
GetX is designed for simplicity. With minimal boilerplate and intuitive APIs, developers can easily integrate state management, navigation, and dependency injection. The unified approach speeds up development, making GetX beginner-friendly and efficient for experienced developers alike.
Community Support
GetX boasts an active and engaged community. With frequent updates, bug fixes, and extensive documentation, developers have access to a vast range of resources to help them overcome challenges and learn advanced techniques.
GetX stands out as a top Flutter state management solution due to its performance, ease of use, and strong community support.
Performance Benefits
GetX optimizes app performance by selectively rebuilding only the necessary UI components. Its lightweight nature ensures smooth performance, making it ideal for small to large-scale applications. By reducing unnecessary rebuilds, GetX ensures efficient resource usage.
Ease of Use
GetX is designed for simplicity. With minimal boilerplate and intuitive APIs, developers can easily integrate state management, navigation, and dependency injection. The unified approach speeds up development, making GetX beginner-friendly and efficient for experienced developers alike.
Community Support
GetX boasts an active and engaged community. With frequent updates, bug fixes, and extensive documentation, developers have access to a vast range of resources to help them overcome challenges and learn advanced techniques.
GetX stands out as a top Flutter state management solution due to its performance, ease of use, and strong community support.
Performance Benefits
GetX optimizes app performance by selectively rebuilding only the necessary UI components. Its lightweight nature ensures smooth performance, making it ideal for small to large-scale applications. By reducing unnecessary rebuilds, GetX ensures efficient resource usage.
Ease of Use
GetX is designed for simplicity. With minimal boilerplate and intuitive APIs, developers can easily integrate state management, navigation, and dependency injection. The unified approach speeds up development, making GetX beginner-friendly and efficient for experienced developers alike.
Community Support
GetX boasts an active and engaged community. With frequent updates, bug fixes, and extensive documentation, developers have access to a vast range of resources to help them overcome challenges and learn advanced techniques.
Other GetX Features
Other GetX Features
Other GetX Features
Other GetX Features
While state management is a core feature of GetX, its capabilities extend to much more, making it a comprehensive tool for Flutter developers.
Route Management
GetX streamlines route management in Flutter, making it much simpler and more efficient. Typically, navigating between screens involves writing verbose code like this:
Navigator.push(
context,
MaterialPageRoute(builder: (context) => Home())
);
With GetX, you can achieve the same result with just two words:
Get.to(Home());
Going back to the previous screen is just as easy. Instead of using:
Navigator.pop(context);
You can use:
Get.back();
Additionally, GetX simplifies navigating to named routes while closing dialogs or drawers. You can use the following command to close any open views and navigate:
Get.offAndToNamed('/second');
Switching Between Themes
GetX also excels in theme management. Switching between light and dark modes becomes effortless, allowing for a more dynamic user experience. For example, to change the app’s theme to dark mode, all you need is:
Get.changeTheme(ThemeData.dark());
This feature is especially beneficial for apps that offer users a choice between different themes, making theme switching quick and seamless.
While state management is a core feature of GetX, its capabilities extend to much more, making it a comprehensive tool for Flutter developers.
Route Management
GetX streamlines route management in Flutter, making it much simpler and more efficient. Typically, navigating between screens involves writing verbose code like this:
Navigator.push(
context,
MaterialPageRoute(builder: (context) => Home())
);
With GetX, you can achieve the same result with just two words:
Get.to(Home());
Going back to the previous screen is just as easy. Instead of using:
Navigator.pop(context);
You can use:
Get.back();
Additionally, GetX simplifies navigating to named routes while closing dialogs or drawers. You can use the following command to close any open views and navigate:
Get.offAndToNamed('/second');
Switching Between Themes
GetX also excels in theme management. Switching between light and dark modes becomes effortless, allowing for a more dynamic user experience. For example, to change the app’s theme to dark mode, all you need is:
Get.changeTheme(ThemeData.dark());
This feature is especially beneficial for apps that offer users a choice between different themes, making theme switching quick and seamless.
While state management is a core feature of GetX, its capabilities extend to much more, making it a comprehensive tool for Flutter developers.
Route Management
GetX streamlines route management in Flutter, making it much simpler and more efficient. Typically, navigating between screens involves writing verbose code like this:
Navigator.push(
context,
MaterialPageRoute(builder: (context) => Home())
);
With GetX, you can achieve the same result with just two words:
Get.to(Home());
Going back to the previous screen is just as easy. Instead of using:
Navigator.pop(context);
You can use:
Get.back();
Additionally, GetX simplifies navigating to named routes while closing dialogs or drawers. You can use the following command to close any open views and navigate:
Get.offAndToNamed('/second');
Switching Between Themes
GetX also excels in theme management. Switching between light and dark modes becomes effortless, allowing for a more dynamic user experience. For example, to change the app’s theme to dark mode, all you need is:
Get.changeTheme(ThemeData.dark());
This feature is especially beneficial for apps that offer users a choice between different themes, making theme switching quick and seamless.
While state management is a core feature of GetX, its capabilities extend to much more, making it a comprehensive tool for Flutter developers.
Route Management
GetX streamlines route management in Flutter, making it much simpler and more efficient. Typically, navigating between screens involves writing verbose code like this:
Navigator.push(
context,
MaterialPageRoute(builder: (context) => Home())
);
With GetX, you can achieve the same result with just two words:
Get.to(Home());
Going back to the previous screen is just as easy. Instead of using:
Navigator.pop(context);
You can use:
Get.back();
Additionally, GetX simplifies navigating to named routes while closing dialogs or drawers. You can use the following command to close any open views and navigate:
Get.offAndToNamed('/second');
Switching Between Themes
GetX also excels in theme management. Switching between light and dark modes becomes effortless, allowing for a more dynamic user experience. For example, to change the app’s theme to dark mode, all you need is:
Get.changeTheme(ThemeData.dark());
This feature is especially beneficial for apps that offer users a choice between different themes, making theme switching quick and seamless.
FAQs: GetX for Dart and Flutter
FAQs: GetX for Dart and Flutter
FAQs: GetX for Dart and Flutter
FAQs: GetX for Dart and Flutter

Frequently Asked Questions (FAQs)
What is GetX in Flutter?
GetX is a lightweight and powerful state management library for Flutter. It provides efficient state management, routing, and dependency injection with minimal boilerplate code.How do I install GetX in my Flutter project?
Add the following to yourpubspec.yaml
file and runflutter pub get
:yamlCopy code
dependencies: get: ^4.1.4
What are the main features of GetX?
GetX offers state management, route management, dependency injection, and theme management, all designed to simplify development and enhance performance.How does GetX improve state management?
GetX uses reactive programming to update only the parts of the UI that need to change, leading to better performance and simpler code compared to other state management solutions.Can GetX handle complex navigation scenarios?
Yes, GetX simplifies navigation by allowing you to navigate between screens without needing context, and it also supports named routes and route transitions.What is dependency injection in GetX?
Dependency injection in GetX allows you to manage and inject dependencies easily throughout your application, facilitating cleaner and more modular code.How do I use GetX for theme management?
You can switch themes dynamically with GetX by callingGet.changeTheme(ThemeData.dark())
for dark mode orThemeData.light()
for light mode.What is the advantage of using GetX over other state management solutions?
GetX is known for its simplicity, minimal boilerplate, and performance efficiency. It combines state management, routing, and dependency injection into a single framework.How do I update the UI reactively with GetX?
Use theObx
widget to automatically rebuild parts of the UI when observable variables change.Can GetX be used with other state management libraries?
While GetX is a complete solution on its own, it can be used alongside other libraries if needed, though it is designed to be a standalone solution.How do I test GetX controllers?
Write unit tests for GetX controllers by creating mock dependencies and asserting expected behavior using standard Dart testing frameworks.Where can I find more resources and documentation for GetX?
Visit the official GetX documentation for detailed guides, examples, and community support.

Frequently Asked Questions (FAQs)
What is GetX in Flutter?
GetX is a lightweight and powerful state management library for Flutter. It provides efficient state management, routing, and dependency injection with minimal boilerplate code.How do I install GetX in my Flutter project?
Add the following to yourpubspec.yaml
file and runflutter pub get
:yamlCopy code
dependencies: get: ^4.1.4
What are the main features of GetX?
GetX offers state management, route management, dependency injection, and theme management, all designed to simplify development and enhance performance.How does GetX improve state management?
GetX uses reactive programming to update only the parts of the UI that need to change, leading to better performance and simpler code compared to other state management solutions.Can GetX handle complex navigation scenarios?
Yes, GetX simplifies navigation by allowing you to navigate between screens without needing context, and it also supports named routes and route transitions.What is dependency injection in GetX?
Dependency injection in GetX allows you to manage and inject dependencies easily throughout your application, facilitating cleaner and more modular code.How do I use GetX for theme management?
You can switch themes dynamically with GetX by callingGet.changeTheme(ThemeData.dark())
for dark mode orThemeData.light()
for light mode.What is the advantage of using GetX over other state management solutions?
GetX is known for its simplicity, minimal boilerplate, and performance efficiency. It combines state management, routing, and dependency injection into a single framework.How do I update the UI reactively with GetX?
Use theObx
widget to automatically rebuild parts of the UI when observable variables change.Can GetX be used with other state management libraries?
While GetX is a complete solution on its own, it can be used alongside other libraries if needed, though it is designed to be a standalone solution.How do I test GetX controllers?
Write unit tests for GetX controllers by creating mock dependencies and asserting expected behavior using standard Dart testing frameworks.Where can I find more resources and documentation for GetX?
Visit the official GetX documentation for detailed guides, examples, and community support.

Frequently Asked Questions (FAQs)
What is GetX in Flutter?
GetX is a lightweight and powerful state management library for Flutter. It provides efficient state management, routing, and dependency injection with minimal boilerplate code.How do I install GetX in my Flutter project?
Add the following to yourpubspec.yaml
file and runflutter pub get
:yamlCopy code
dependencies: get: ^4.1.4
What are the main features of GetX?
GetX offers state management, route management, dependency injection, and theme management, all designed to simplify development and enhance performance.How does GetX improve state management?
GetX uses reactive programming to update only the parts of the UI that need to change, leading to better performance and simpler code compared to other state management solutions.Can GetX handle complex navigation scenarios?
Yes, GetX simplifies navigation by allowing you to navigate between screens without needing context, and it also supports named routes and route transitions.What is dependency injection in GetX?
Dependency injection in GetX allows you to manage and inject dependencies easily throughout your application, facilitating cleaner and more modular code.How do I use GetX for theme management?
You can switch themes dynamically with GetX by callingGet.changeTheme(ThemeData.dark())
for dark mode orThemeData.light()
for light mode.What is the advantage of using GetX over other state management solutions?
GetX is known for its simplicity, minimal boilerplate, and performance efficiency. It combines state management, routing, and dependency injection into a single framework.How do I update the UI reactively with GetX?
Use theObx
widget to automatically rebuild parts of the UI when observable variables change.Can GetX be used with other state management libraries?
While GetX is a complete solution on its own, it can be used alongside other libraries if needed, though it is designed to be a standalone solution.How do I test GetX controllers?
Write unit tests for GetX controllers by creating mock dependencies and asserting expected behavior using standard Dart testing frameworks.Where can I find more resources and documentation for GetX?
Visit the official GetX documentation for detailed guides, examples, and community support.

Frequently Asked Questions (FAQs)
What is GetX in Flutter?
GetX is a lightweight and powerful state management library for Flutter. It provides efficient state management, routing, and dependency injection with minimal boilerplate code.How do I install GetX in my Flutter project?
Add the following to yourpubspec.yaml
file and runflutter pub get
:yamlCopy code
dependencies: get: ^4.1.4
What are the main features of GetX?
GetX offers state management, route management, dependency injection, and theme management, all designed to simplify development and enhance performance.How does GetX improve state management?
GetX uses reactive programming to update only the parts of the UI that need to change, leading to better performance and simpler code compared to other state management solutions.Can GetX handle complex navigation scenarios?
Yes, GetX simplifies navigation by allowing you to navigate between screens without needing context, and it also supports named routes and route transitions.What is dependency injection in GetX?
Dependency injection in GetX allows you to manage and inject dependencies easily throughout your application, facilitating cleaner and more modular code.How do I use GetX for theme management?
You can switch themes dynamically with GetX by callingGet.changeTheme(ThemeData.dark())
for dark mode orThemeData.light()
for light mode.What is the advantage of using GetX over other state management solutions?
GetX is known for its simplicity, minimal boilerplate, and performance efficiency. It combines state management, routing, and dependency injection into a single framework.How do I update the UI reactively with GetX?
Use theObx
widget to automatically rebuild parts of the UI when observable variables change.Can GetX be used with other state management libraries?
While GetX is a complete solution on its own, it can be used alongside other libraries if needed, though it is designed to be a standalone solution.How do I test GetX controllers?
Write unit tests for GetX controllers by creating mock dependencies and asserting expected behavior using standard Dart testing frameworks.Where can I find more resources and documentation for GetX?
Visit the official GetX documentation for detailed guides, examples, and community support.
Conclusion
Conclusion
Conclusion
Conclusion
GetX revolutionizes Flutter development by integrating state management, routing, and dependency injection into one efficient framework. Its lightweight design enhances app performance and simplifies complex tasks, making development faster and easier. Explore GetX to elevate your projects and streamline your coding process.
For additional resources and insights on Flutter, visit Blup's Flutter Resources. Unlock the full potential of Flutter with GetX today!
GetX revolutionizes Flutter development by integrating state management, routing, and dependency injection into one efficient framework. Its lightweight design enhances app performance and simplifies complex tasks, making development faster and easier. Explore GetX to elevate your projects and streamline your coding process.
For additional resources and insights on Flutter, visit Blup's Flutter Resources. Unlock the full potential of Flutter with GetX today!
GetX revolutionizes Flutter development by integrating state management, routing, and dependency injection into one efficient framework. Its lightweight design enhances app performance and simplifies complex tasks, making development faster and easier. Explore GetX to elevate your projects and streamline your coding process.
For additional resources and insights on Flutter, visit Blup's Flutter Resources. Unlock the full potential of Flutter with GetX today!
GetX revolutionizes Flutter development by integrating state management, routing, and dependency injection into one efficient framework. Its lightweight design enhances app performance and simplifies complex tasks, making development faster and easier. Explore GetX to elevate your projects and streamline your coding process.
For additional resources and insights on Flutter, visit Blup's Flutter Resources. Unlock the full potential of Flutter with GetX today!
Table of content
India(HQ)
9th Floor, Tower C, Logix Cyber Park,
C Block, Phase 2, Industrial Area, Sector 62, Noida, Uttar Pradesh, 201309
USA
2081 Center Street Berkeley,
CA 94704
© 2021-23 Blupx Private Limited.
All rights reserved.
India(HQ)
9th Floor, Tower C, Logix Cyber Park,
C Block, Phase 2, Industrial Area, Sector 62, Noida, Uttar Pradesh, 201309
USA
2081 Center Street Berkeley,
CA 94704
© 2021-23 Blupx Private Limited.
All rights reserved.
India(HQ)
9th Floor, Tower C, Logix Cyber Park,
C Block, Phase 2, Industrial Area, Sector 62, Noida, Uttar Pradesh, 201309
USA
2081 Center Street Berkeley,
CA 94704
© 2021-23 Blupx Private Limited.
All rights reserved.