QRScan Flutter: How to scan and generate QR code in Flutter
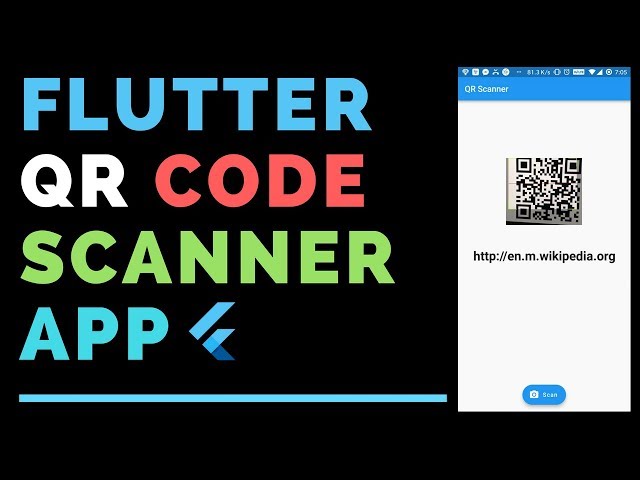
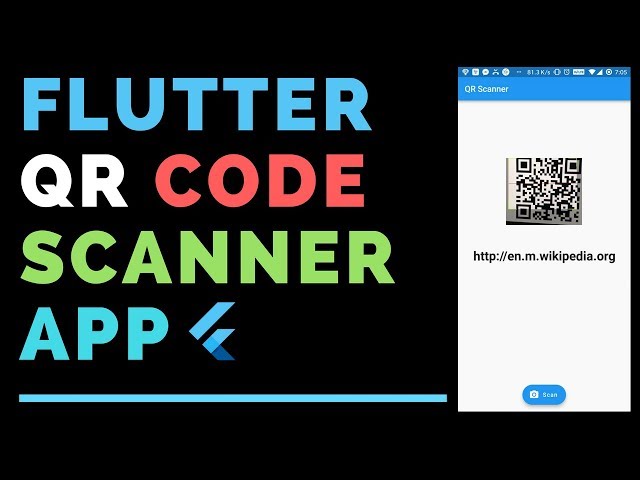
QRScan Flutter is a powerful plugin that enables seamless QR code scanning and barcode recognition within Flutter mobile applications. QR codes have become ubiquitous in modern society, facilitating quick access to information, product details, and app functionalities.
Integrating QRScan Flutter into your app empowers users with the ability to scan QR codes and barcodes effortlessly, enhancing user experiences and opening up new possibilities for interactive and dynamic app functionalities.
In this comprehensive blog, we will delve into the world of QRScan Flutter, exploring its features, implementation, and customization options. We will guide you through the process of integrating QRScan Flutter into your Flutter projects, enabling you to harness the power of QR code scanning in your apps.
We will use the package from https://pub.dev/packages/qr_flutter for this article.
Understanding QRScan Flutte
What is QRScan Flutter?
QRScan Flutter is a powerful Flutter plugin for QR code scanning and barcode recognition.
Key Features: QR code scanning, barcode recognition, customization options, and cross-platform compatibility.
Benefits of QRScan Flutter in Mobile App Development
Mobile scanner apps have revolutionized the way we handle documents and information. Gone are the days of carrying around stacks of papers or relying on bulky scanners. Here are some of the key benefits of using mobile scanner apps:
1. Convenience: Mobile scanner apps provide a convenient solution for digitizing documents on-the-go. With just your smartphone or tablet, you can capture and store important information without the need for additional hardware.
2. Portability: The portability of mobile scanner apps allows you to carry your scanner wherever you go. This means you can quickly capture documents, receipts, or whiteboards, even when you're away from your desk or office.
3. Time-saving: Mobile scanner apps save you valuable time by eliminating the need for manual data entry or searching through physical documents. With advanced OCR technology, these apps can extract text from scanned documents, making it easy to search, edit, and share information.
4. Organization: Mobile scanner apps provide a digital filing system, allowing you to organize and categorize your scanned documents. This makes it easier to find and retrieve important information when you need it.
5. Collaboration: With mobile scanner apps, you can easily share scanned documents with colleagues, clients, or collaborators. This streamlines the collaboration process and eliminates the need for physical document sharing.
6. Security: Mobile scanner apps often offer encryption and password protection features, ensuring the security of your scanned documents. This gives you peace of mind, knowing that your sensitive information is safe.
By leveraging the benefits of mobile scanner apps, you can streamline your workflow, improve productivity, and stay organized, no matter where you are. These apps are a game-changer for individuals and businesses alike.
Getting Started with QRScan Flutter
QRScan Flutter is a powerful Flutter plugin that enables QR code scanning functionality in your mobile app. In this section, we will guide you through the installation process and demonstrate how to initiate the QR code scanning process in your Flutter project.
Installation and Setup
To get started with QRScan Flutter, follow these steps:
Version Compatibility: Before proceeding with the installation, ensure that your Flutter version is compatible with QRScan Flutter. The latest version (4.0.0+) supports null safety and requires a compatible version of Flutter. If your current Flutter version is incompatible, you can use a 3.x version of the library.
Add Dependency to pubspec.yaml: Open your `pubspec.yaml` file and add the QRScan Flutter dependency under the `dependencies` section:
dependencies:
qr_flutter: ^4.1.0
If you're using the Flutter master channel or want to try the latest updates, you can use the master branch directly by specifying the Git URL:
dependencies:
qr_flutter:
git:
url: https://github.com/theyakka/qr.flutter.git
Please note that using the master branch may introduce instability.
Get the Package: After adding the dependency, run `flutter packages get` in the terminal or update your packages using your IDE. This will download and set up the QRScan Flutter package in your project.
Initiating the QR Code Scanning Process
Now that you have QRScan Flutter installed in your project, you can start scanning QR codes. The process is straightforward:
Import Dependency:
In your Dart file where you want to initiate the QR code scanning process, import the QRScan Flutter package:
import 'package:qr_flutter/qr_flutter.dart';
2. Start Scanning:
To initiate the QR code scanning process, use the `scan()` method provided by QRScan Flutter. Here's how to do it:
QRScanner scanner = QRScanner();
void startScanning() async {
String scanResult = await scanner.scan();
// Handle the scan result here
if (scanResult != null) {
print("Scanned QR code: $scanResult");
}
}
In this example, we created a QRScanner instance and called the `scan()` method, which will open the device's camera to scan QR codes. The scanned data will be returned as a string, allowing you to handle it according to your app's requirements.
With these simple steps, you have successfully installed QRScan Flutter in your Flutter project and learned how to initiate the QR code scanning process.
Scanning QR Codes
QRScan Flutter provides robust QR code scanning functionality that allows your Flutter app to scan and retrieve data from QR codes effortlessly. In this section, we will explore how to handle QR code scanning and retrieve the scanned data using QRScan Flutter.
Handling Scan Results and Data Retrieval
When a QR code is successfully scanned, QRScan Flutter returns the scanned data as a string. To handle the scan result, you can use the `scan()` method provided by QRScan Flutter, as shown in the following code snippet:
QRScanner scanner = QRScanner();
void startScanning() async {
String scanResult = await scanner.scan();
// Handle the scan result here
if (scanResult != null) {
print("Scanned QR code: $scanResult");
}
}
In this example, we used the `scan()` method to initiate the scanning process. The scanned data is stored in the `scanResult` variable, and you can perform further actions based on the retrieved data.
Demonstrative Code Snippets
To demonstrate the ease of scanning QR codes with QRScan Flutter, here are some code snippets that showcase different scanning scenarios:
Scanning a QR code and printing the result:
String scanResult = await QRScanner().scan();
print("Scanned QR code: $scanResult");
Handling scan cancellation:
String scanResult = await QRScanner().scan();
if (scanResult == null) {
print("Scan was cancelled.");
} else {
print("Scanned QR code: $scanResult");
}
Performing an action based on the scanned data:
String scanResult = await QRScanner().scan();
if (scanResult != null) {
if (scanResult.startsWith('http://') || scanResult.startsWith('https://')) {
// Open the URL in a web browser
// Implement your code here
} else {
// Handle other data types
// Implement your code here
}
}
With these code snippets, you can easily integrate QR code scanning into your Flutter app and utilize the scanned data for various functionalities. QRScan Flutter simplifies the process of scanning QR codes, making it a valuable addition to your mobile app development toolkit.
Scanning Barcodes with QRScan Flutter
QRScan Flutter not only supports QR code scanning but also provides the ability to scan barcodes effortlessly. In this section, we will explore how to extend QRScan Flutter for barcode scanning and implement the barcode scanning functionality.
Implementation of Barcode Scanning Functionality
To initiate barcode scanning, you can use the `scanBarcode()` method provided by QRScan Flutter. The scanned data will be returned as a string, which you can then process according to your app's requirements.
Code Examples for Scanning Barcodes
Here are some code examples to illustrate how to use QRScan Flutter for barcode scanning:
String scanResult = await QRScanner().scanBarcode();
print("Scanned barcode: $scanResult");
String scanResult = await QRScanner().scanBarcode();
if (scanResult == null) {
print("Scan was cancelled.");
} else {
print("Scanned barcode: $scanResult");
}
String scanResult = await QRScanner().scanBarcode();
if (scanResult != null) {
// Process the barcode data based on its type
// Implement your code here
}
With these examples, you can easily integrate barcode scanning into your Flutter app using QRScan Flutter.
Customizing the QR Scanner
QRScan Flutter offers a range of customization options to tailor the scanner's UI and appearance according to your app's design. In this section, we will explore the various customization options available in QRScan Flutter.
You can modify the appearance of the QR scanner by adjusting parameters such as the size, background color, and padding. Additionally, you can customize the shape and color of the QR code's eyes (corners) and dots.
QRScan Flutter allows you to add overlays to the scanner, providing a visually appealing and informative UI. You can adjust the scan area's size and position to focus on specific parts of the scanned code.
Handling Scan Results
Effectively handling scan results is crucial to ensuring a seamless user experience in your app. In this section, we will delve into the process of processing scan data, interpreting results, and implementing error handling.
Once a QR code or barcode is scanned, you can process the retrieved data to perform various actions. For instance, if the scanned data is a URL, you can open it in a web browser or redirect the user to a specific page within your app.
Error handling is essential when dealing with QR code and barcode scanning. QRScan Flutter provides mechanisms to handle scenarios where the scanned data is invalid or cannot be processed properly.
To provide a practical perspective, we will explore real-world use cases for handling scan results. This will include scenarios such as processing contact information, accessing product details, and authenticating users using QR codes or barcodes.
With a comprehensive understanding of how to handle scan results, you can optimize your app's functionality and user experience when using QRScan Flutter.
QRScan Flutter Use Cases
QRScan Flutter can be used in a wide variety of real-world scenarios, including:
Retail: QRScan Flutter can be used to scan QR codes on product packaging, in-store signage, and marketing materials. This can help retailers to improve customer engagement, track inventory, and provide personalized shopping experiences.
Logistics: QRScan Flutter can be used to scan QR codes on shipping labels, packages, and consignment notes. This can help logistics companies to track shipments, improve efficiency, and reduce errors.
Events: QRScan Flutter can be used to scan QR codes on event tickets, passes, and programs. This can help event organizers to manage attendance, collect data, and provide a more seamless experience for attendees.
Healthcare: QRScan Flutter can be used to scan QR codes on medical records, prescriptions, and other forms of documentation. This can help healthcare providers to improve the efficiency of their operations and provide better care for their patients.
Education: QRScan Flutter can be used to scan QR codes on textbooks, worksheets, and other educational materials.
Comparing QRScan Flutter to Other QR Code Scanning Plugins
QRScan Flutter is a popular QR code scanning plugin for Flutter. It is easy to use, has a wide range of features, and is well-documented. However, there are other QR code scanning plugins available for Flutter. Popular alternatives are:
Barcode Scanner: Barcode Scanner is a simple and easy-to-use QR code scanning plugin. It is not as feature-rich as QRScan Flutter, but it is a good option for basic scanning needs.
ZXing: ZXing is a powerful and versatile QR code scanning plugin. It supports a wide range of features and can be customized to meet specific needs. However, ZXing can be more difficult to use than QRScan Flutter.
ML Kit: ML Kit is a Google-developed plugin that can be used to scan QR codes and other barcodes. It is not specifically designed for Flutter, but it can be used with Flutter apps. ML Kit is a good option for developers who want to use Google's machine learning technology to improve their QR code scanning apps.
Advantages and Unique Features of QRScan Flutter
QRScan Flutter has a number of advantages over other QR code scanning plugins, including:
Easy to use: QRScan Flutter is very easy to use. The documentation is clear and concise, and the code is well-organized.
Wide range of features: QRScan Flutter supports a wide range of features, including scanning of both QR codes and barcodes, customization of the scanner, and handling of scan results.
Well-documented: The documentation for QRScan Flutter is clear and concise. It covers all of the features of the plugin, as well as how to use it in your Flutter apps.
Overall, QRScan Flutter is a powerful and versatile QR code scanning plugin for Flutter. It is easy to use, has a wide range of features, and is well-documented.
Building a QR Code Scanning App with QRScan Flutter
In this section, we will walk through a step-by-step tutorial for creating a basic QR code scanning app using QRScan Flutter. We'll cover the complete Flutter code required to implement the app.
Step-by-Step Tutorial for Creating a Basic QR Code Scanning App
Install QRScan Flutter: Ensure you have QRScan Flutter installed in your Flutter project by adding the following to your `pubspec.yaml` file:
dependencies:
qr_flutter: ^4.1.0
Import Dependencies: Import the necessary dependencies in your Dart file to use QRScan Flutter:
import 'package:flutter/material.dart';
import 'package:flutter/services.dart';
import 'package:qr_flutter/qr_flutter.dart';
Set Up MainScreen Widget: Create a new Dart file named `main_screen.dart` and set up the `MainScreen` widget as the home screen of the app. This widget will contain the QR code scanning functionality.
Create QR Code Scanner: Inside the `MainScreen` widget, add a `QrImageView` widget to display the QR code scanner:
class MainScreen extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('QR Code Scanner'),
),
body: Center(
child: QrImageView(
data: 'Scan QR Code',
version: QrVersions.auto,
size: 200.0,
),
),
);
}
}
Handle Scan Results: Implement the functionality to handle the scanned data. You can use the `scanBarcode()` method provided by QRScan Flutter to scan QR codes:
String scannedData = await QRScanner().scanBarcode();
print("Scanned Data: $scannedData");
Complete Sample Flutter Code for the QR Code Scanning App:
// main_screen.dart
import 'package:flutter/material.dart';
import 'package:qr_flutter/qr_flutter.dart';
class MainScreen extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('QR Code Scanner'),
),
body: Center(
child: QrImageView(
data: 'Scan QR Code',
version: QrVersions.auto,
size: 200.0,
),
),
);
}
}
// main.dart
import 'package:flutter/material.dart';
import 'package:flutter/services.dart';
import 'package:qr_flutter/qr_flutter.dart';
import 'main_screen.dart';
void main() => runApp(ExampleApp());
class ExampleApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
SystemChrome.setSystemUIOverlayStyle(
const SystemUiOverlayStyle(
statusBarColor: Colors.white,
statusBarIconBrightness: Brightness.dark,
systemNavigationBarColor: Colors.white,
systemNavigationBarIconBrightness: Brightness.dark,
),
);
return MaterialApp(
title: 'QR Code Scanner App',
theme: ThemeData.light(),
debugShowCheckedModeBanner: false,
home: MainScreen(),
);
}
}
With this code, you have successfully implemented a basic QR code scanning app using QRScan Flutter. Users can scan QR codes using the app, and the scanned data will be printed in the console.
Conclusion
In conclusion, QRScan Flutter is a powerful plugin that enables seamless QR code and barcode scanning functionality in Flutter apps. With its simple setup and customizable options, developers can easily integrate QR code scanning into their mobile applications.
As Flutter continues to evolve, we can anticipate further enhancements and features in QRScan Flutter, making it an even more valuable tool for developers. So, if you're looking to add QR code scanning capabilities to your Flutter app, don't hesitate to explore QRScan Flutter and create innovative solutions for your users. Happy coding!
QRScan Flutter is a powerful plugin that enables seamless QR code scanning and barcode recognition within Flutter mobile applications. QR codes have become ubiquitous in modern society, facilitating quick access to information, product details, and app functionalities.
Integrating QRScan Flutter into your app empowers users with the ability to scan QR codes and barcodes effortlessly, enhancing user experiences and opening up new possibilities for interactive and dynamic app functionalities.
In this comprehensive blog, we will delve into the world of QRScan Flutter, exploring its features, implementation, and customization options. We will guide you through the process of integrating QRScan Flutter into your Flutter projects, enabling you to harness the power of QR code scanning in your apps.
We will use the package from https://pub.dev/packages/qr_flutter for this article.
Understanding QRScan Flutte
What is QRScan Flutter?
QRScan Flutter is a powerful Flutter plugin for QR code scanning and barcode recognition.
Key Features: QR code scanning, barcode recognition, customization options, and cross-platform compatibility.
Benefits of QRScan Flutter in Mobile App Development
Mobile scanner apps have revolutionized the way we handle documents and information. Gone are the days of carrying around stacks of papers or relying on bulky scanners. Here are some of the key benefits of using mobile scanner apps:
1. Convenience: Mobile scanner apps provide a convenient solution for digitizing documents on-the-go. With just your smartphone or tablet, you can capture and store important information without the need for additional hardware.
2. Portability: The portability of mobile scanner apps allows you to carry your scanner wherever you go. This means you can quickly capture documents, receipts, or whiteboards, even when you're away from your desk or office.
3. Time-saving: Mobile scanner apps save you valuable time by eliminating the need for manual data entry or searching through physical documents. With advanced OCR technology, these apps can extract text from scanned documents, making it easy to search, edit, and share information.
4. Organization: Mobile scanner apps provide a digital filing system, allowing you to organize and categorize your scanned documents. This makes it easier to find and retrieve important information when you need it.
5. Collaboration: With mobile scanner apps, you can easily share scanned documents with colleagues, clients, or collaborators. This streamlines the collaboration process and eliminates the need for physical document sharing.
6. Security: Mobile scanner apps often offer encryption and password protection features, ensuring the security of your scanned documents. This gives you peace of mind, knowing that your sensitive information is safe.
By leveraging the benefits of mobile scanner apps, you can streamline your workflow, improve productivity, and stay organized, no matter where you are. These apps are a game-changer for individuals and businesses alike.
Getting Started with QRScan Flutter
QRScan Flutter is a powerful Flutter plugin that enables QR code scanning functionality in your mobile app. In this section, we will guide you through the installation process and demonstrate how to initiate the QR code scanning process in your Flutter project.
Installation and Setup
To get started with QRScan Flutter, follow these steps:
Version Compatibility: Before proceeding with the installation, ensure that your Flutter version is compatible with QRScan Flutter. The latest version (4.0.0+) supports null safety and requires a compatible version of Flutter. If your current Flutter version is incompatible, you can use a 3.x version of the library.
Add Dependency to pubspec.yaml: Open your `pubspec.yaml` file and add the QRScan Flutter dependency under the `dependencies` section:
dependencies:
qr_flutter: ^4.1.0
If you're using the Flutter master channel or want to try the latest updates, you can use the master branch directly by specifying the Git URL:
dependencies:
qr_flutter:
git:
url: https://github.com/theyakka/qr.flutter.git
Please note that using the master branch may introduce instability.
Get the Package: After adding the dependency, run `flutter packages get` in the terminal or update your packages using your IDE. This will download and set up the QRScan Flutter package in your project.
Initiating the QR Code Scanning Process
Now that you have QRScan Flutter installed in your project, you can start scanning QR codes. The process is straightforward:
Import Dependency:
In your Dart file where you want to initiate the QR code scanning process, import the QRScan Flutter package:
import 'package:qr_flutter/qr_flutter.dart';
2. Start Scanning:
To initiate the QR code scanning process, use the `scan()` method provided by QRScan Flutter. Here's how to do it:
QRScanner scanner = QRScanner();
void startScanning() async {
String scanResult = await scanner.scan();
// Handle the scan result here
if (scanResult != null) {
print("Scanned QR code: $scanResult");
}
}
In this example, we created a QRScanner instance and called the `scan()` method, which will open the device's camera to scan QR codes. The scanned data will be returned as a string, allowing you to handle it according to your app's requirements.
With these simple steps, you have successfully installed QRScan Flutter in your Flutter project and learned how to initiate the QR code scanning process.
Scanning QR Codes
QRScan Flutter provides robust QR code scanning functionality that allows your Flutter app to scan and retrieve data from QR codes effortlessly. In this section, we will explore how to handle QR code scanning and retrieve the scanned data using QRScan Flutter.
Handling Scan Results and Data Retrieval
When a QR code is successfully scanned, QRScan Flutter returns the scanned data as a string. To handle the scan result, you can use the `scan()` method provided by QRScan Flutter, as shown in the following code snippet:
QRScanner scanner = QRScanner();
void startScanning() async {
String scanResult = await scanner.scan();
// Handle the scan result here
if (scanResult != null) {
print("Scanned QR code: $scanResult");
}
}
In this example, we used the `scan()` method to initiate the scanning process. The scanned data is stored in the `scanResult` variable, and you can perform further actions based on the retrieved data.
Demonstrative Code Snippets
To demonstrate the ease of scanning QR codes with QRScan Flutter, here are some code snippets that showcase different scanning scenarios:
Scanning a QR code and printing the result:
String scanResult = await QRScanner().scan();
print("Scanned QR code: $scanResult");
Handling scan cancellation:
String scanResult = await QRScanner().scan();
if (scanResult == null) {
print("Scan was cancelled.");
} else {
print("Scanned QR code: $scanResult");
}
Performing an action based on the scanned data:
String scanResult = await QRScanner().scan();
if (scanResult != null) {
if (scanResult.startsWith('http://') || scanResult.startsWith('https://')) {
// Open the URL in a web browser
// Implement your code here
} else {
// Handle other data types
// Implement your code here
}
}
With these code snippets, you can easily integrate QR code scanning into your Flutter app and utilize the scanned data for various functionalities. QRScan Flutter simplifies the process of scanning QR codes, making it a valuable addition to your mobile app development toolkit.
Scanning Barcodes with QRScan Flutter
QRScan Flutter not only supports QR code scanning but also provides the ability to scan barcodes effortlessly. In this section, we will explore how to extend QRScan Flutter for barcode scanning and implement the barcode scanning functionality.
Implementation of Barcode Scanning Functionality
To initiate barcode scanning, you can use the `scanBarcode()` method provided by QRScan Flutter. The scanned data will be returned as a string, which you can then process according to your app's requirements.
Code Examples for Scanning Barcodes
Here are some code examples to illustrate how to use QRScan Flutter for barcode scanning:
String scanResult = await QRScanner().scanBarcode();
print("Scanned barcode: $scanResult");
String scanResult = await QRScanner().scanBarcode();
if (scanResult == null) {
print("Scan was cancelled.");
} else {
print("Scanned barcode: $scanResult");
}
String scanResult = await QRScanner().scanBarcode();
if (scanResult != null) {
// Process the barcode data based on its type
// Implement your code here
}
With these examples, you can easily integrate barcode scanning into your Flutter app using QRScan Flutter.
Customizing the QR Scanner
QRScan Flutter offers a range of customization options to tailor the scanner's UI and appearance according to your app's design. In this section, we will explore the various customization options available in QRScan Flutter.
You can modify the appearance of the QR scanner by adjusting parameters such as the size, background color, and padding. Additionally, you can customize the shape and color of the QR code's eyes (corners) and dots.
QRScan Flutter allows you to add overlays to the scanner, providing a visually appealing and informative UI. You can adjust the scan area's size and position to focus on specific parts of the scanned code.
Handling Scan Results
Effectively handling scan results is crucial to ensuring a seamless user experience in your app. In this section, we will delve into the process of processing scan data, interpreting results, and implementing error handling.
Once a QR code or barcode is scanned, you can process the retrieved data to perform various actions. For instance, if the scanned data is a URL, you can open it in a web browser or redirect the user to a specific page within your app.
Error handling is essential when dealing with QR code and barcode scanning. QRScan Flutter provides mechanisms to handle scenarios where the scanned data is invalid or cannot be processed properly.
To provide a practical perspective, we will explore real-world use cases for handling scan results. This will include scenarios such as processing contact information, accessing product details, and authenticating users using QR codes or barcodes.
With a comprehensive understanding of how to handle scan results, you can optimize your app's functionality and user experience when using QRScan Flutter.
QRScan Flutter Use Cases
QRScan Flutter can be used in a wide variety of real-world scenarios, including:
Retail: QRScan Flutter can be used to scan QR codes on product packaging, in-store signage, and marketing materials. This can help retailers to improve customer engagement, track inventory, and provide personalized shopping experiences.
Logistics: QRScan Flutter can be used to scan QR codes on shipping labels, packages, and consignment notes. This can help logistics companies to track shipments, improve efficiency, and reduce errors.
Events: QRScan Flutter can be used to scan QR codes on event tickets, passes, and programs. This can help event organizers to manage attendance, collect data, and provide a more seamless experience for attendees.
Healthcare: QRScan Flutter can be used to scan QR codes on medical records, prescriptions, and other forms of documentation. This can help healthcare providers to improve the efficiency of their operations and provide better care for their patients.
Education: QRScan Flutter can be used to scan QR codes on textbooks, worksheets, and other educational materials.
Comparing QRScan Flutter to Other QR Code Scanning Plugins
QRScan Flutter is a popular QR code scanning plugin for Flutter. It is easy to use, has a wide range of features, and is well-documented. However, there are other QR code scanning plugins available for Flutter. Popular alternatives are:
Barcode Scanner: Barcode Scanner is a simple and easy-to-use QR code scanning plugin. It is not as feature-rich as QRScan Flutter, but it is a good option for basic scanning needs.
ZXing: ZXing is a powerful and versatile QR code scanning plugin. It supports a wide range of features and can be customized to meet specific needs. However, ZXing can be more difficult to use than QRScan Flutter.
ML Kit: ML Kit is a Google-developed plugin that can be used to scan QR codes and other barcodes. It is not specifically designed for Flutter, but it can be used with Flutter apps. ML Kit is a good option for developers who want to use Google's machine learning technology to improve their QR code scanning apps.
Advantages and Unique Features of QRScan Flutter
QRScan Flutter has a number of advantages over other QR code scanning plugins, including:
Easy to use: QRScan Flutter is very easy to use. The documentation is clear and concise, and the code is well-organized.
Wide range of features: QRScan Flutter supports a wide range of features, including scanning of both QR codes and barcodes, customization of the scanner, and handling of scan results.
Well-documented: The documentation for QRScan Flutter is clear and concise. It covers all of the features of the plugin, as well as how to use it in your Flutter apps.
Overall, QRScan Flutter is a powerful and versatile QR code scanning plugin for Flutter. It is easy to use, has a wide range of features, and is well-documented.
Building a QR Code Scanning App with QRScan Flutter
In this section, we will walk through a step-by-step tutorial for creating a basic QR code scanning app using QRScan Flutter. We'll cover the complete Flutter code required to implement the app.
Step-by-Step Tutorial for Creating a Basic QR Code Scanning App
Install QRScan Flutter: Ensure you have QRScan Flutter installed in your Flutter project by adding the following to your `pubspec.yaml` file:
dependencies:
qr_flutter: ^4.1.0
Import Dependencies: Import the necessary dependencies in your Dart file to use QRScan Flutter:
import 'package:flutter/material.dart';
import 'package:flutter/services.dart';
import 'package:qr_flutter/qr_flutter.dart';
Set Up MainScreen Widget: Create a new Dart file named `main_screen.dart` and set up the `MainScreen` widget as the home screen of the app. This widget will contain the QR code scanning functionality.
Create QR Code Scanner: Inside the `MainScreen` widget, add a `QrImageView` widget to display the QR code scanner:
class MainScreen extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('QR Code Scanner'),
),
body: Center(
child: QrImageView(
data: 'Scan QR Code',
version: QrVersions.auto,
size: 200.0,
),
),
);
}
}
Handle Scan Results: Implement the functionality to handle the scanned data. You can use the `scanBarcode()` method provided by QRScan Flutter to scan QR codes:
String scannedData = await QRScanner().scanBarcode();
print("Scanned Data: $scannedData");
Complete Sample Flutter Code for the QR Code Scanning App:
// main_screen.dart
import 'package:flutter/material.dart';
import 'package:qr_flutter/qr_flutter.dart';
class MainScreen extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('QR Code Scanner'),
),
body: Center(
child: QrImageView(
data: 'Scan QR Code',
version: QrVersions.auto,
size: 200.0,
),
),
);
}
}
// main.dart
import 'package:flutter/material.dart';
import 'package:flutter/services.dart';
import 'package:qr_flutter/qr_flutter.dart';
import 'main_screen.dart';
void main() => runApp(ExampleApp());
class ExampleApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
SystemChrome.setSystemUIOverlayStyle(
const SystemUiOverlayStyle(
statusBarColor: Colors.white,
statusBarIconBrightness: Brightness.dark,
systemNavigationBarColor: Colors.white,
systemNavigationBarIconBrightness: Brightness.dark,
),
);
return MaterialApp(
title: 'QR Code Scanner App',
theme: ThemeData.light(),
debugShowCheckedModeBanner: false,
home: MainScreen(),
);
}
}
With this code, you have successfully implemented a basic QR code scanning app using QRScan Flutter. Users can scan QR codes using the app, and the scanned data will be printed in the console.
Conclusion
In conclusion, QRScan Flutter is a powerful plugin that enables seamless QR code and barcode scanning functionality in Flutter apps. With its simple setup and customizable options, developers can easily integrate QR code scanning into their mobile applications.
As Flutter continues to evolve, we can anticipate further enhancements and features in QRScan Flutter, making it an even more valuable tool for developers. So, if you're looking to add QR code scanning capabilities to your Flutter app, don't hesitate to explore QRScan Flutter and create innovative solutions for your users. Happy coding!
QRScan Flutter is a powerful plugin that enables seamless QR code scanning and barcode recognition within Flutter mobile applications. QR codes have become ubiquitous in modern society, facilitating quick access to information, product details, and app functionalities.
Integrating QRScan Flutter into your app empowers users with the ability to scan QR codes and barcodes effortlessly, enhancing user experiences and opening up new possibilities for interactive and dynamic app functionalities.
In this comprehensive blog, we will delve into the world of QRScan Flutter, exploring its features, implementation, and customization options. We will guide you through the process of integrating QRScan Flutter into your Flutter projects, enabling you to harness the power of QR code scanning in your apps.
We will use the package from https://pub.dev/packages/qr_flutter for this article.
Understanding QRScan Flutte
What is QRScan Flutter?
QRScan Flutter is a powerful Flutter plugin for QR code scanning and barcode recognition.
Key Features: QR code scanning, barcode recognition, customization options, and cross-platform compatibility.
Benefits of QRScan Flutter in Mobile App Development
Mobile scanner apps have revolutionized the way we handle documents and information. Gone are the days of carrying around stacks of papers or relying on bulky scanners. Here are some of the key benefits of using mobile scanner apps:
1. Convenience: Mobile scanner apps provide a convenient solution for digitizing documents on-the-go. With just your smartphone or tablet, you can capture and store important information without the need for additional hardware.
2. Portability: The portability of mobile scanner apps allows you to carry your scanner wherever you go. This means you can quickly capture documents, receipts, or whiteboards, even when you're away from your desk or office.
3. Time-saving: Mobile scanner apps save you valuable time by eliminating the need for manual data entry or searching through physical documents. With advanced OCR technology, these apps can extract text from scanned documents, making it easy to search, edit, and share information.
4. Organization: Mobile scanner apps provide a digital filing system, allowing you to organize and categorize your scanned documents. This makes it easier to find and retrieve important information when you need it.
5. Collaboration: With mobile scanner apps, you can easily share scanned documents with colleagues, clients, or collaborators. This streamlines the collaboration process and eliminates the need for physical document sharing.
6. Security: Mobile scanner apps often offer encryption and password protection features, ensuring the security of your scanned documents. This gives you peace of mind, knowing that your sensitive information is safe.
By leveraging the benefits of mobile scanner apps, you can streamline your workflow, improve productivity, and stay organized, no matter where you are. These apps are a game-changer for individuals and businesses alike.
Getting Started with QRScan Flutter
QRScan Flutter is a powerful Flutter plugin that enables QR code scanning functionality in your mobile app. In this section, we will guide you through the installation process and demonstrate how to initiate the QR code scanning process in your Flutter project.
Installation and Setup
To get started with QRScan Flutter, follow these steps:
Version Compatibility: Before proceeding with the installation, ensure that your Flutter version is compatible with QRScan Flutter. The latest version (4.0.0+) supports null safety and requires a compatible version of Flutter. If your current Flutter version is incompatible, you can use a 3.x version of the library.
Add Dependency to pubspec.yaml: Open your `pubspec.yaml` file and add the QRScan Flutter dependency under the `dependencies` section:
dependencies:
qr_flutter: ^4.1.0
If you're using the Flutter master channel or want to try the latest updates, you can use the master branch directly by specifying the Git URL:
dependencies:
qr_flutter:
git:
url: https://github.com/theyakka/qr.flutter.git
Please note that using the master branch may introduce instability.
Get the Package: After adding the dependency, run `flutter packages get` in the terminal or update your packages using your IDE. This will download and set up the QRScan Flutter package in your project.
Initiating the QR Code Scanning Process
Now that you have QRScan Flutter installed in your project, you can start scanning QR codes. The process is straightforward:
Import Dependency:
In your Dart file where you want to initiate the QR code scanning process, import the QRScan Flutter package:
import 'package:qr_flutter/qr_flutter.dart';
2. Start Scanning:
To initiate the QR code scanning process, use the `scan()` method provided by QRScan Flutter. Here's how to do it:
QRScanner scanner = QRScanner();
void startScanning() async {
String scanResult = await scanner.scan();
// Handle the scan result here
if (scanResult != null) {
print("Scanned QR code: $scanResult");
}
}
In this example, we created a QRScanner instance and called the `scan()` method, which will open the device's camera to scan QR codes. The scanned data will be returned as a string, allowing you to handle it according to your app's requirements.
With these simple steps, you have successfully installed QRScan Flutter in your Flutter project and learned how to initiate the QR code scanning process.
Scanning QR Codes
QRScan Flutter provides robust QR code scanning functionality that allows your Flutter app to scan and retrieve data from QR codes effortlessly. In this section, we will explore how to handle QR code scanning and retrieve the scanned data using QRScan Flutter.
Handling Scan Results and Data Retrieval
When a QR code is successfully scanned, QRScan Flutter returns the scanned data as a string. To handle the scan result, you can use the `scan()` method provided by QRScan Flutter, as shown in the following code snippet:
QRScanner scanner = QRScanner();
void startScanning() async {
String scanResult = await scanner.scan();
// Handle the scan result here
if (scanResult != null) {
print("Scanned QR code: $scanResult");
}
}
In this example, we used the `scan()` method to initiate the scanning process. The scanned data is stored in the `scanResult` variable, and you can perform further actions based on the retrieved data.
Demonstrative Code Snippets
To demonstrate the ease of scanning QR codes with QRScan Flutter, here are some code snippets that showcase different scanning scenarios:
Scanning a QR code and printing the result:
String scanResult = await QRScanner().scan();
print("Scanned QR code: $scanResult");
Handling scan cancellation:
String scanResult = await QRScanner().scan();
if (scanResult == null) {
print("Scan was cancelled.");
} else {
print("Scanned QR code: $scanResult");
}
Performing an action based on the scanned data:
String scanResult = await QRScanner().scan();
if (scanResult != null) {
if (scanResult.startsWith('http://') || scanResult.startsWith('https://')) {
// Open the URL in a web browser
// Implement your code here
} else {
// Handle other data types
// Implement your code here
}
}
With these code snippets, you can easily integrate QR code scanning into your Flutter app and utilize the scanned data for various functionalities. QRScan Flutter simplifies the process of scanning QR codes, making it a valuable addition to your mobile app development toolkit.
Scanning Barcodes with QRScan Flutter
QRScan Flutter not only supports QR code scanning but also provides the ability to scan barcodes effortlessly. In this section, we will explore how to extend QRScan Flutter for barcode scanning and implement the barcode scanning functionality.
Implementation of Barcode Scanning Functionality
To initiate barcode scanning, you can use the `scanBarcode()` method provided by QRScan Flutter. The scanned data will be returned as a string, which you can then process according to your app's requirements.
Code Examples for Scanning Barcodes
Here are some code examples to illustrate how to use QRScan Flutter for barcode scanning:
String scanResult = await QRScanner().scanBarcode();
print("Scanned barcode: $scanResult");
String scanResult = await QRScanner().scanBarcode();
if (scanResult == null) {
print("Scan was cancelled.");
} else {
print("Scanned barcode: $scanResult");
}
String scanResult = await QRScanner().scanBarcode();
if (scanResult != null) {
// Process the barcode data based on its type
// Implement your code here
}
With these examples, you can easily integrate barcode scanning into your Flutter app using QRScan Flutter.
Customizing the QR Scanner
QRScan Flutter offers a range of customization options to tailor the scanner's UI and appearance according to your app's design. In this section, we will explore the various customization options available in QRScan Flutter.
You can modify the appearance of the QR scanner by adjusting parameters such as the size, background color, and padding. Additionally, you can customize the shape and color of the QR code's eyes (corners) and dots.
QRScan Flutter allows you to add overlays to the scanner, providing a visually appealing and informative UI. You can adjust the scan area's size and position to focus on specific parts of the scanned code.
Handling Scan Results
Effectively handling scan results is crucial to ensuring a seamless user experience in your app. In this section, we will delve into the process of processing scan data, interpreting results, and implementing error handling.
Once a QR code or barcode is scanned, you can process the retrieved data to perform various actions. For instance, if the scanned data is a URL, you can open it in a web browser or redirect the user to a specific page within your app.
Error handling is essential when dealing with QR code and barcode scanning. QRScan Flutter provides mechanisms to handle scenarios where the scanned data is invalid or cannot be processed properly.
To provide a practical perspective, we will explore real-world use cases for handling scan results. This will include scenarios such as processing contact information, accessing product details, and authenticating users using QR codes or barcodes.
With a comprehensive understanding of how to handle scan results, you can optimize your app's functionality and user experience when using QRScan Flutter.
QRScan Flutter Use Cases
QRScan Flutter can be used in a wide variety of real-world scenarios, including:
Retail: QRScan Flutter can be used to scan QR codes on product packaging, in-store signage, and marketing materials. This can help retailers to improve customer engagement, track inventory, and provide personalized shopping experiences.
Logistics: QRScan Flutter can be used to scan QR codes on shipping labels, packages, and consignment notes. This can help logistics companies to track shipments, improve efficiency, and reduce errors.
Events: QRScan Flutter can be used to scan QR codes on event tickets, passes, and programs. This can help event organizers to manage attendance, collect data, and provide a more seamless experience for attendees.
Healthcare: QRScan Flutter can be used to scan QR codes on medical records, prescriptions, and other forms of documentation. This can help healthcare providers to improve the efficiency of their operations and provide better care for their patients.
Education: QRScan Flutter can be used to scan QR codes on textbooks, worksheets, and other educational materials.
Comparing QRScan Flutter to Other QR Code Scanning Plugins
QRScan Flutter is a popular QR code scanning plugin for Flutter. It is easy to use, has a wide range of features, and is well-documented. However, there are other QR code scanning plugins available for Flutter. Popular alternatives are:
Barcode Scanner: Barcode Scanner is a simple and easy-to-use QR code scanning plugin. It is not as feature-rich as QRScan Flutter, but it is a good option for basic scanning needs.
ZXing: ZXing is a powerful and versatile QR code scanning plugin. It supports a wide range of features and can be customized to meet specific needs. However, ZXing can be more difficult to use than QRScan Flutter.
ML Kit: ML Kit is a Google-developed plugin that can be used to scan QR codes and other barcodes. It is not specifically designed for Flutter, but it can be used with Flutter apps. ML Kit is a good option for developers who want to use Google's machine learning technology to improve their QR code scanning apps.
Advantages and Unique Features of QRScan Flutter
QRScan Flutter has a number of advantages over other QR code scanning plugins, including:
Easy to use: QRScan Flutter is very easy to use. The documentation is clear and concise, and the code is well-organized.
Wide range of features: QRScan Flutter supports a wide range of features, including scanning of both QR codes and barcodes, customization of the scanner, and handling of scan results.
Well-documented: The documentation for QRScan Flutter is clear and concise. It covers all of the features of the plugin, as well as how to use it in your Flutter apps.
Overall, QRScan Flutter is a powerful and versatile QR code scanning plugin for Flutter. It is easy to use, has a wide range of features, and is well-documented.
Building a QR Code Scanning App with QRScan Flutter
In this section, we will walk through a step-by-step tutorial for creating a basic QR code scanning app using QRScan Flutter. We'll cover the complete Flutter code required to implement the app.
Step-by-Step Tutorial for Creating a Basic QR Code Scanning App
Install QRScan Flutter: Ensure you have QRScan Flutter installed in your Flutter project by adding the following to your `pubspec.yaml` file:
dependencies:
qr_flutter: ^4.1.0
Import Dependencies: Import the necessary dependencies in your Dart file to use QRScan Flutter:
import 'package:flutter/material.dart';
import 'package:flutter/services.dart';
import 'package:qr_flutter/qr_flutter.dart';
Set Up MainScreen Widget: Create a new Dart file named `main_screen.dart` and set up the `MainScreen` widget as the home screen of the app. This widget will contain the QR code scanning functionality.
Create QR Code Scanner: Inside the `MainScreen` widget, add a `QrImageView` widget to display the QR code scanner:
class MainScreen extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('QR Code Scanner'),
),
body: Center(
child: QrImageView(
data: 'Scan QR Code',
version: QrVersions.auto,
size: 200.0,
),
),
);
}
}
Handle Scan Results: Implement the functionality to handle the scanned data. You can use the `scanBarcode()` method provided by QRScan Flutter to scan QR codes:
String scannedData = await QRScanner().scanBarcode();
print("Scanned Data: $scannedData");
Complete Sample Flutter Code for the QR Code Scanning App:
// main_screen.dart
import 'package:flutter/material.dart';
import 'package:qr_flutter/qr_flutter.dart';
class MainScreen extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('QR Code Scanner'),
),
body: Center(
child: QrImageView(
data: 'Scan QR Code',
version: QrVersions.auto,
size: 200.0,
),
),
);
}
}
// main.dart
import 'package:flutter/material.dart';
import 'package:flutter/services.dart';
import 'package:qr_flutter/qr_flutter.dart';
import 'main_screen.dart';
void main() => runApp(ExampleApp());
class ExampleApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
SystemChrome.setSystemUIOverlayStyle(
const SystemUiOverlayStyle(
statusBarColor: Colors.white,
statusBarIconBrightness: Brightness.dark,
systemNavigationBarColor: Colors.white,
systemNavigationBarIconBrightness: Brightness.dark,
),
);
return MaterialApp(
title: 'QR Code Scanner App',
theme: ThemeData.light(),
debugShowCheckedModeBanner: false,
home: MainScreen(),
);
}
}
With this code, you have successfully implemented a basic QR code scanning app using QRScan Flutter. Users can scan QR codes using the app, and the scanned data will be printed in the console.
Conclusion
In conclusion, QRScan Flutter is a powerful plugin that enables seamless QR code and barcode scanning functionality in Flutter apps. With its simple setup and customizable options, developers can easily integrate QR code scanning into their mobile applications.
As Flutter continues to evolve, we can anticipate further enhancements and features in QRScan Flutter, making it an even more valuable tool for developers. So, if you're looking to add QR code scanning capabilities to your Flutter app, don't hesitate to explore QRScan Flutter and create innovative solutions for your users. Happy coding!
QRScan Flutter is a powerful plugin that enables seamless QR code scanning and barcode recognition within Flutter mobile applications. QR codes have become ubiquitous in modern society, facilitating quick access to information, product details, and app functionalities.
Integrating QRScan Flutter into your app empowers users with the ability to scan QR codes and barcodes effortlessly, enhancing user experiences and opening up new possibilities for interactive and dynamic app functionalities.
In this comprehensive blog, we will delve into the world of QRScan Flutter, exploring its features, implementation, and customization options. We will guide you through the process of integrating QRScan Flutter into your Flutter projects, enabling you to harness the power of QR code scanning in your apps.
We will use the package from https://pub.dev/packages/qr_flutter for this article.
Understanding QRScan Flutte
What is QRScan Flutter?
QRScan Flutter is a powerful Flutter plugin for QR code scanning and barcode recognition.
Key Features: QR code scanning, barcode recognition, customization options, and cross-platform compatibility.
Benefits of QRScan Flutter in Mobile App Development
Mobile scanner apps have revolutionized the way we handle documents and information. Gone are the days of carrying around stacks of papers or relying on bulky scanners. Here are some of the key benefits of using mobile scanner apps:
1. Convenience: Mobile scanner apps provide a convenient solution for digitizing documents on-the-go. With just your smartphone or tablet, you can capture and store important information without the need for additional hardware.
2. Portability: The portability of mobile scanner apps allows you to carry your scanner wherever you go. This means you can quickly capture documents, receipts, or whiteboards, even when you're away from your desk or office.
3. Time-saving: Mobile scanner apps save you valuable time by eliminating the need for manual data entry or searching through physical documents. With advanced OCR technology, these apps can extract text from scanned documents, making it easy to search, edit, and share information.
4. Organization: Mobile scanner apps provide a digital filing system, allowing you to organize and categorize your scanned documents. This makes it easier to find and retrieve important information when you need it.
5. Collaboration: With mobile scanner apps, you can easily share scanned documents with colleagues, clients, or collaborators. This streamlines the collaboration process and eliminates the need for physical document sharing.
6. Security: Mobile scanner apps often offer encryption and password protection features, ensuring the security of your scanned documents. This gives you peace of mind, knowing that your sensitive information is safe.
By leveraging the benefits of mobile scanner apps, you can streamline your workflow, improve productivity, and stay organized, no matter where you are. These apps are a game-changer for individuals and businesses alike.
Getting Started with QRScan Flutter
QRScan Flutter is a powerful Flutter plugin that enables QR code scanning functionality in your mobile app. In this section, we will guide you through the installation process and demonstrate how to initiate the QR code scanning process in your Flutter project.
Installation and Setup
To get started with QRScan Flutter, follow these steps:
Version Compatibility: Before proceeding with the installation, ensure that your Flutter version is compatible with QRScan Flutter. The latest version (4.0.0+) supports null safety and requires a compatible version of Flutter. If your current Flutter version is incompatible, you can use a 3.x version of the library.
Add Dependency to pubspec.yaml: Open your `pubspec.yaml` file and add the QRScan Flutter dependency under the `dependencies` section:
dependencies:
qr_flutter: ^4.1.0
If you're using the Flutter master channel or want to try the latest updates, you can use the master branch directly by specifying the Git URL:
dependencies:
qr_flutter:
git:
url: https://github.com/theyakka/qr.flutter.git
Please note that using the master branch may introduce instability.
Get the Package: After adding the dependency, run `flutter packages get` in the terminal or update your packages using your IDE. This will download and set up the QRScan Flutter package in your project.
Initiating the QR Code Scanning Process
Now that you have QRScan Flutter installed in your project, you can start scanning QR codes. The process is straightforward:
Import Dependency:
In your Dart file where you want to initiate the QR code scanning process, import the QRScan Flutter package:
import 'package:qr_flutter/qr_flutter.dart';
2. Start Scanning:
To initiate the QR code scanning process, use the `scan()` method provided by QRScan Flutter. Here's how to do it:
QRScanner scanner = QRScanner();
void startScanning() async {
String scanResult = await scanner.scan();
// Handle the scan result here
if (scanResult != null) {
print("Scanned QR code: $scanResult");
}
}
In this example, we created a QRScanner instance and called the `scan()` method, which will open the device's camera to scan QR codes. The scanned data will be returned as a string, allowing you to handle it according to your app's requirements.
With these simple steps, you have successfully installed QRScan Flutter in your Flutter project and learned how to initiate the QR code scanning process.
Scanning QR Codes
QRScan Flutter provides robust QR code scanning functionality that allows your Flutter app to scan and retrieve data from QR codes effortlessly. In this section, we will explore how to handle QR code scanning and retrieve the scanned data using QRScan Flutter.
Handling Scan Results and Data Retrieval
When a QR code is successfully scanned, QRScan Flutter returns the scanned data as a string. To handle the scan result, you can use the `scan()` method provided by QRScan Flutter, as shown in the following code snippet:
QRScanner scanner = QRScanner();
void startScanning() async {
String scanResult = await scanner.scan();
// Handle the scan result here
if (scanResult != null) {
print("Scanned QR code: $scanResult");
}
}
In this example, we used the `scan()` method to initiate the scanning process. The scanned data is stored in the `scanResult` variable, and you can perform further actions based on the retrieved data.
Demonstrative Code Snippets
To demonstrate the ease of scanning QR codes with QRScan Flutter, here are some code snippets that showcase different scanning scenarios:
Scanning a QR code and printing the result:
String scanResult = await QRScanner().scan();
print("Scanned QR code: $scanResult");
Handling scan cancellation:
String scanResult = await QRScanner().scan();
if (scanResult == null) {
print("Scan was cancelled.");
} else {
print("Scanned QR code: $scanResult");
}
Performing an action based on the scanned data:
String scanResult = await QRScanner().scan();
if (scanResult != null) {
if (scanResult.startsWith('http://') || scanResult.startsWith('https://')) {
// Open the URL in a web browser
// Implement your code here
} else {
// Handle other data types
// Implement your code here
}
}
With these code snippets, you can easily integrate QR code scanning into your Flutter app and utilize the scanned data for various functionalities. QRScan Flutter simplifies the process of scanning QR codes, making it a valuable addition to your mobile app development toolkit.
Scanning Barcodes with QRScan Flutter
QRScan Flutter not only supports QR code scanning but also provides the ability to scan barcodes effortlessly. In this section, we will explore how to extend QRScan Flutter for barcode scanning and implement the barcode scanning functionality.
Implementation of Barcode Scanning Functionality
To initiate barcode scanning, you can use the `scanBarcode()` method provided by QRScan Flutter. The scanned data will be returned as a string, which you can then process according to your app's requirements.
Code Examples for Scanning Barcodes
Here are some code examples to illustrate how to use QRScan Flutter for barcode scanning:
String scanResult = await QRScanner().scanBarcode();
print("Scanned barcode: $scanResult");
String scanResult = await QRScanner().scanBarcode();
if (scanResult == null) {
print("Scan was cancelled.");
} else {
print("Scanned barcode: $scanResult");
}
String scanResult = await QRScanner().scanBarcode();
if (scanResult != null) {
// Process the barcode data based on its type
// Implement your code here
}
With these examples, you can easily integrate barcode scanning into your Flutter app using QRScan Flutter.
Customizing the QR Scanner
QRScan Flutter offers a range of customization options to tailor the scanner's UI and appearance according to your app's design. In this section, we will explore the various customization options available in QRScan Flutter.
You can modify the appearance of the QR scanner by adjusting parameters such as the size, background color, and padding. Additionally, you can customize the shape and color of the QR code's eyes (corners) and dots.
QRScan Flutter allows you to add overlays to the scanner, providing a visually appealing and informative UI. You can adjust the scan area's size and position to focus on specific parts of the scanned code.
Handling Scan Results
Effectively handling scan results is crucial to ensuring a seamless user experience in your app. In this section, we will delve into the process of processing scan data, interpreting results, and implementing error handling.
Once a QR code or barcode is scanned, you can process the retrieved data to perform various actions. For instance, if the scanned data is a URL, you can open it in a web browser or redirect the user to a specific page within your app.
Error handling is essential when dealing with QR code and barcode scanning. QRScan Flutter provides mechanisms to handle scenarios where the scanned data is invalid or cannot be processed properly.
To provide a practical perspective, we will explore real-world use cases for handling scan results. This will include scenarios such as processing contact information, accessing product details, and authenticating users using QR codes or barcodes.
With a comprehensive understanding of how to handle scan results, you can optimize your app's functionality and user experience when using QRScan Flutter.
QRScan Flutter Use Cases
QRScan Flutter can be used in a wide variety of real-world scenarios, including:
Retail: QRScan Flutter can be used to scan QR codes on product packaging, in-store signage, and marketing materials. This can help retailers to improve customer engagement, track inventory, and provide personalized shopping experiences.
Logistics: QRScan Flutter can be used to scan QR codes on shipping labels, packages, and consignment notes. This can help logistics companies to track shipments, improve efficiency, and reduce errors.
Events: QRScan Flutter can be used to scan QR codes on event tickets, passes, and programs. This can help event organizers to manage attendance, collect data, and provide a more seamless experience for attendees.
Healthcare: QRScan Flutter can be used to scan QR codes on medical records, prescriptions, and other forms of documentation. This can help healthcare providers to improve the efficiency of their operations and provide better care for their patients.
Education: QRScan Flutter can be used to scan QR codes on textbooks, worksheets, and other educational materials.
Comparing QRScan Flutter to Other QR Code Scanning Plugins
QRScan Flutter is a popular QR code scanning plugin for Flutter. It is easy to use, has a wide range of features, and is well-documented. However, there are other QR code scanning plugins available for Flutter. Popular alternatives are:
Barcode Scanner: Barcode Scanner is a simple and easy-to-use QR code scanning plugin. It is not as feature-rich as QRScan Flutter, but it is a good option for basic scanning needs.
ZXing: ZXing is a powerful and versatile QR code scanning plugin. It supports a wide range of features and can be customized to meet specific needs. However, ZXing can be more difficult to use than QRScan Flutter.
ML Kit: ML Kit is a Google-developed plugin that can be used to scan QR codes and other barcodes. It is not specifically designed for Flutter, but it can be used with Flutter apps. ML Kit is a good option for developers who want to use Google's machine learning technology to improve their QR code scanning apps.
Advantages and Unique Features of QRScan Flutter
QRScan Flutter has a number of advantages over other QR code scanning plugins, including:
Easy to use: QRScan Flutter is very easy to use. The documentation is clear and concise, and the code is well-organized.
Wide range of features: QRScan Flutter supports a wide range of features, including scanning of both QR codes and barcodes, customization of the scanner, and handling of scan results.
Well-documented: The documentation for QRScan Flutter is clear and concise. It covers all of the features of the plugin, as well as how to use it in your Flutter apps.
Overall, QRScan Flutter is a powerful and versatile QR code scanning plugin for Flutter. It is easy to use, has a wide range of features, and is well-documented.
Building a QR Code Scanning App with QRScan Flutter
In this section, we will walk through a step-by-step tutorial for creating a basic QR code scanning app using QRScan Flutter. We'll cover the complete Flutter code required to implement the app.
Step-by-Step Tutorial for Creating a Basic QR Code Scanning App
Install QRScan Flutter: Ensure you have QRScan Flutter installed in your Flutter project by adding the following to your `pubspec.yaml` file:
dependencies:
qr_flutter: ^4.1.0
Import Dependencies: Import the necessary dependencies in your Dart file to use QRScan Flutter:
import 'package:flutter/material.dart';
import 'package:flutter/services.dart';
import 'package:qr_flutter/qr_flutter.dart';
Set Up MainScreen Widget: Create a new Dart file named `main_screen.dart` and set up the `MainScreen` widget as the home screen of the app. This widget will contain the QR code scanning functionality.
Create QR Code Scanner: Inside the `MainScreen` widget, add a `QrImageView` widget to display the QR code scanner:
class MainScreen extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('QR Code Scanner'),
),
body: Center(
child: QrImageView(
data: 'Scan QR Code',
version: QrVersions.auto,
size: 200.0,
),
),
);
}
}
Handle Scan Results: Implement the functionality to handle the scanned data. You can use the `scanBarcode()` method provided by QRScan Flutter to scan QR codes:
String scannedData = await QRScanner().scanBarcode();
print("Scanned Data: $scannedData");
Complete Sample Flutter Code for the QR Code Scanning App:
// main_screen.dart
import 'package:flutter/material.dart';
import 'package:qr_flutter/qr_flutter.dart';
class MainScreen extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('QR Code Scanner'),
),
body: Center(
child: QrImageView(
data: 'Scan QR Code',
version: QrVersions.auto,
size: 200.0,
),
),
);
}
}
// main.dart
import 'package:flutter/material.dart';
import 'package:flutter/services.dart';
import 'package:qr_flutter/qr_flutter.dart';
import 'main_screen.dart';
void main() => runApp(ExampleApp());
class ExampleApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
SystemChrome.setSystemUIOverlayStyle(
const SystemUiOverlayStyle(
statusBarColor: Colors.white,
statusBarIconBrightness: Brightness.dark,
systemNavigationBarColor: Colors.white,
systemNavigationBarIconBrightness: Brightness.dark,
),
);
return MaterialApp(
title: 'QR Code Scanner App',
theme: ThemeData.light(),
debugShowCheckedModeBanner: false,
home: MainScreen(),
);
}
}
With this code, you have successfully implemented a basic QR code scanning app using QRScan Flutter. Users can scan QR codes using the app, and the scanned data will be printed in the console.
Conclusion
In conclusion, QRScan Flutter is a powerful plugin that enables seamless QR code and barcode scanning functionality in Flutter apps. With its simple setup and customizable options, developers can easily integrate QR code scanning into their mobile applications.
As Flutter continues to evolve, we can anticipate further enhancements and features in QRScan Flutter, making it an even more valuable tool for developers. So, if you're looking to add QR code scanning capabilities to your Flutter app, don't hesitate to explore QRScan Flutter and create innovative solutions for your users. Happy coding!
© 2021-25 Blupx Private Limited.
All rights reserved.
© 2021-25 Blupx Private Limited.
All rights reserved.
© 2021-25 Blupx Private Limited.
All rights reserved.