Flutter app development
6 min read
6 min read
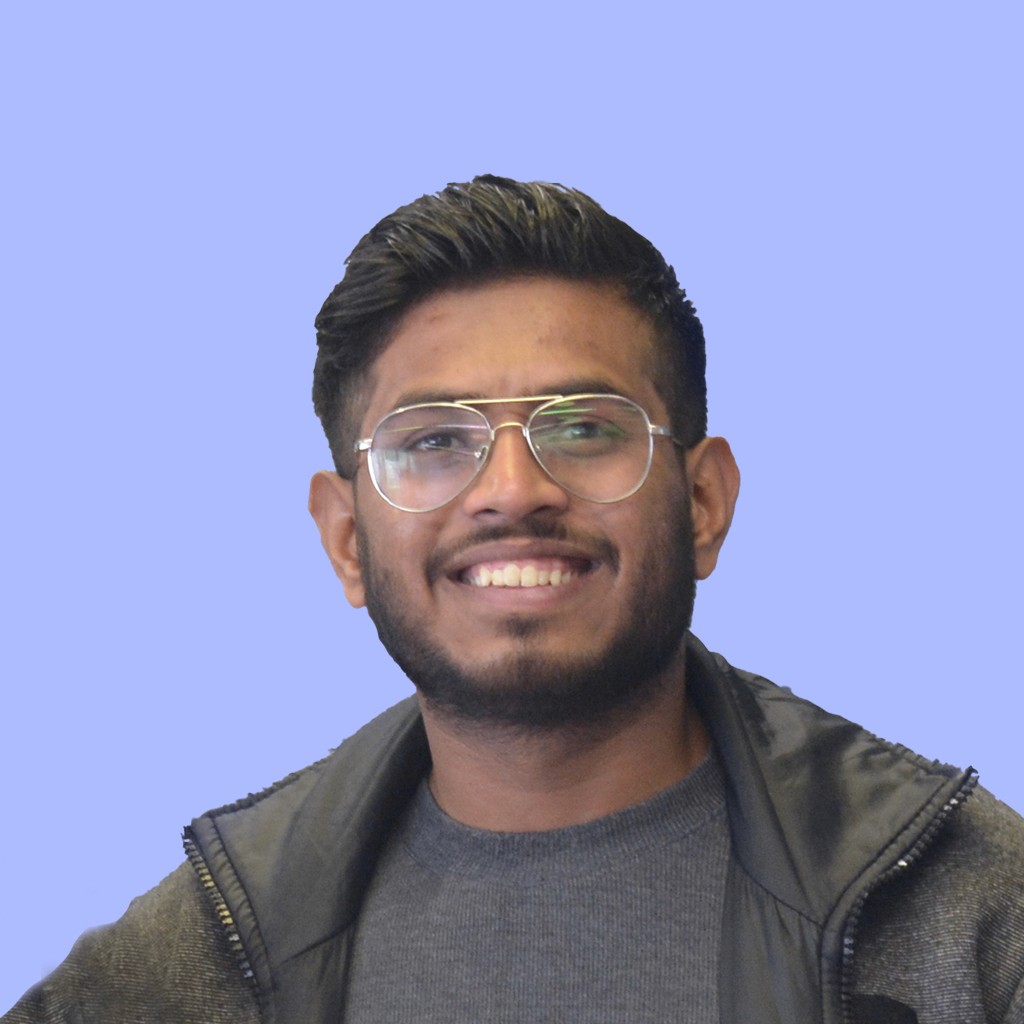
Ashutosh Agarwal
Published on Jul 25, 2023
How to Adjust Flutter Font Size Based on Screen Size? Best 3 ways


Get Started - Adjust Flutter Font Size
Get Started - Adjust Flutter Font Size
Get Started - Adjust Flutter Font Size
Get Started - Adjust Flutter Font Size
In the fast-paced world of mobile app development, creating a seamless user experience across various devices is essential. One critical aspect that significantly impacts user experience is font sizing. Flutter, with its flexible and powerful framework, offers several methods to ensure that fonts adapt gracefully to different screen sizes, providing a visually pleasing and user-friendly interface.
In this blog, we will delve into the world of responsive font sizing in Flutter and explore three effective methods to adjust font size based on screen size. By the end of this article, you'll be equipped with the knowledge to implement the most suitable approach for your app, guaranteeing a delightful experience for every user, no matter the device they use. Let's dive in and unlock the secrets of perfecting font sizing in Flutter!
Understanding the Need for Responsive Font Sizing
In the dynamic world of mobile app development, creating a seamless user experience is paramount to success. One crucial aspect that significantly impacts user satisfaction is font sizing. Responsive font sizing, also known as auto-sizing text, is a technique that ensures fonts adapt fluidly to various screen sizes, providing optimal readability and visual appeal for all users.
Challenges of Fixed Font Sizes:
Inconsistency: Using fixed font sizes can lead to inconsistent text presentation across devices, causing readability issues and a disjointed user experience.
Limited Adaptability: Fixed font sizes may appear too small on larger screens, making the text difficult to read, or too large on smaller screens, resulting in text overflow.
Accessibility Concerns: A lack of responsive font sizing can hinder accessibility for users with visual impairments, as they may struggle to read fixed-size fonts.
Responsive Font Sizing: The Solution
Enhanced Readability: By implementing responsive font sizing, text adjusts intelligently based on screen dimensions, ensuring optimal readability on every device.
Consistent User Experience: With auto-sizing text, you can deliver a cohesive user experience, creating harmony across various screen sizes and resolutions.
Improved Accessibility: Responsive fonts accommodate users with visual impairments, promoting inclusivity and accessibility within your app.
In the following sections, we will explore three effective methods for achieving responsive font sizing in Flutter. Let's delve into these techniques and unlock the power of flexible font sizing for your mobile app.
In the fast-paced world of mobile app development, creating a seamless user experience across various devices is essential. One critical aspect that significantly impacts user experience is font sizing. Flutter, with its flexible and powerful framework, offers several methods to ensure that fonts adapt gracefully to different screen sizes, providing a visually pleasing and user-friendly interface.
In this blog, we will delve into the world of responsive font sizing in Flutter and explore three effective methods to adjust font size based on screen size. By the end of this article, you'll be equipped with the knowledge to implement the most suitable approach for your app, guaranteeing a delightful experience for every user, no matter the device they use. Let's dive in and unlock the secrets of perfecting font sizing in Flutter!
Understanding the Need for Responsive Font Sizing
In the dynamic world of mobile app development, creating a seamless user experience is paramount to success. One crucial aspect that significantly impacts user satisfaction is font sizing. Responsive font sizing, also known as auto-sizing text, is a technique that ensures fonts adapt fluidly to various screen sizes, providing optimal readability and visual appeal for all users.
Challenges of Fixed Font Sizes:
Inconsistency: Using fixed font sizes can lead to inconsistent text presentation across devices, causing readability issues and a disjointed user experience.
Limited Adaptability: Fixed font sizes may appear too small on larger screens, making the text difficult to read, or too large on smaller screens, resulting in text overflow.
Accessibility Concerns: A lack of responsive font sizing can hinder accessibility for users with visual impairments, as they may struggle to read fixed-size fonts.
Responsive Font Sizing: The Solution
Enhanced Readability: By implementing responsive font sizing, text adjusts intelligently based on screen dimensions, ensuring optimal readability on every device.
Consistent User Experience: With auto-sizing text, you can deliver a cohesive user experience, creating harmony across various screen sizes and resolutions.
Improved Accessibility: Responsive fonts accommodate users with visual impairments, promoting inclusivity and accessibility within your app.
In the following sections, we will explore three effective methods for achieving responsive font sizing in Flutter. Let's delve into these techniques and unlock the power of flexible font sizing for your mobile app.
In the fast-paced world of mobile app development, creating a seamless user experience across various devices is essential. One critical aspect that significantly impacts user experience is font sizing. Flutter, with its flexible and powerful framework, offers several methods to ensure that fonts adapt gracefully to different screen sizes, providing a visually pleasing and user-friendly interface.
In this blog, we will delve into the world of responsive font sizing in Flutter and explore three effective methods to adjust font size based on screen size. By the end of this article, you'll be equipped with the knowledge to implement the most suitable approach for your app, guaranteeing a delightful experience for every user, no matter the device they use. Let's dive in and unlock the secrets of perfecting font sizing in Flutter!
Understanding the Need for Responsive Font Sizing
In the dynamic world of mobile app development, creating a seamless user experience is paramount to success. One crucial aspect that significantly impacts user satisfaction is font sizing. Responsive font sizing, also known as auto-sizing text, is a technique that ensures fonts adapt fluidly to various screen sizes, providing optimal readability and visual appeal for all users.
Challenges of Fixed Font Sizes:
Inconsistency: Using fixed font sizes can lead to inconsistent text presentation across devices, causing readability issues and a disjointed user experience.
Limited Adaptability: Fixed font sizes may appear too small on larger screens, making the text difficult to read, or too large on smaller screens, resulting in text overflow.
Accessibility Concerns: A lack of responsive font sizing can hinder accessibility for users with visual impairments, as they may struggle to read fixed-size fonts.
Responsive Font Sizing: The Solution
Enhanced Readability: By implementing responsive font sizing, text adjusts intelligently based on screen dimensions, ensuring optimal readability on every device.
Consistent User Experience: With auto-sizing text, you can deliver a cohesive user experience, creating harmony across various screen sizes and resolutions.
Improved Accessibility: Responsive fonts accommodate users with visual impairments, promoting inclusivity and accessibility within your app.
In the following sections, we will explore three effective methods for achieving responsive font sizing in Flutter. Let's delve into these techniques and unlock the power of flexible font sizing for your mobile app.
In the fast-paced world of mobile app development, creating a seamless user experience across various devices is essential. One critical aspect that significantly impacts user experience is font sizing. Flutter, with its flexible and powerful framework, offers several methods to ensure that fonts adapt gracefully to different screen sizes, providing a visually pleasing and user-friendly interface.
In this blog, we will delve into the world of responsive font sizing in Flutter and explore three effective methods to adjust font size based on screen size. By the end of this article, you'll be equipped with the knowledge to implement the most suitable approach for your app, guaranteeing a delightful experience for every user, no matter the device they use. Let's dive in and unlock the secrets of perfecting font sizing in Flutter!
Understanding the Need for Responsive Font Sizing
In the dynamic world of mobile app development, creating a seamless user experience is paramount to success. One crucial aspect that significantly impacts user satisfaction is font sizing. Responsive font sizing, also known as auto-sizing text, is a technique that ensures fonts adapt fluidly to various screen sizes, providing optimal readability and visual appeal for all users.
Challenges of Fixed Font Sizes:
Inconsistency: Using fixed font sizes can lead to inconsistent text presentation across devices, causing readability issues and a disjointed user experience.
Limited Adaptability: Fixed font sizes may appear too small on larger screens, making the text difficult to read, or too large on smaller screens, resulting in text overflow.
Accessibility Concerns: A lack of responsive font sizing can hinder accessibility for users with visual impairments, as they may struggle to read fixed-size fonts.
Responsive Font Sizing: The Solution
Enhanced Readability: By implementing responsive font sizing, text adjusts intelligently based on screen dimensions, ensuring optimal readability on every device.
Consistent User Experience: With auto-sizing text, you can deliver a cohesive user experience, creating harmony across various screen sizes and resolutions.
Improved Accessibility: Responsive fonts accommodate users with visual impairments, promoting inclusivity and accessibility within your app.
In the following sections, we will explore three effective methods for achieving responsive font sizing in Flutter. Let's delve into these techniques and unlock the power of flexible font sizing for your mobile app.
AutoSizeText Widget
AutoSizeText Widget
AutoSizeText Widget
AutoSizeText Widget
Method 1 - Using the AutoSizeText Widget

Flutter offers a convenient solution for responsive font sizing through the `AutoSizeText` widget. This built-in widget automatically adjusts the font size of text based on the available space within its parent widget. It allows developers to set a range of font sizes, and the `AutoSizeText` widget intelligently calculates the ideal font size to fit the available space, ensuring readability on different screen sizes.
Code Example:
AutoSizeText(
'Sample text',
minFontSize: 12,
maxFontSize: 20,
overflow: TextOverflow.ellipsis,
)
Advantages of Using AutoSizeText:
Dynamic Sizing: The `AutoSizeText` widget adapts the font size dynamically, eliminating the need for manual adjustments for each screen size.
Readability: By automatically fitting the text within the available space, it ensures that the content remains easily readable on any device.
Space Optimization: AutoSizeText optimizes the space, preventing text overflow or excessive white spaces, and creating a clean and professional appearance.
Ideal Scenarios for AutoSizeText:
Text-Heavy Layouts: When dealing with content-rich screens containing various text elements, `AutoSizeText` ensures a harmonious display of all text components.
Multi-Screen Support: In scenarios where your app needs to run on multiple devices with varying screen dimensions, this widget simplifies the font-sizing process.
Localization: For apps with multilingual support, `AutoSizeText` handles different text lengths gracefully, accommodating translations without layout disruptions.
Leveraging the `AutoSizeText` widget empowers developers to create adaptive and user-friendly interfaces, enabling a smooth and consistent user experience across devices. Next, let's explore another method for achieving responsive font sizing in Flutter.
Method 1 - Using the AutoSizeText Widget

Flutter offers a convenient solution for responsive font sizing through the `AutoSizeText` widget. This built-in widget automatically adjusts the font size of text based on the available space within its parent widget. It allows developers to set a range of font sizes, and the `AutoSizeText` widget intelligently calculates the ideal font size to fit the available space, ensuring readability on different screen sizes.
Code Example:
AutoSizeText(
'Sample text',
minFontSize: 12,
maxFontSize: 20,
overflow: TextOverflow.ellipsis,
)
Advantages of Using AutoSizeText:
Dynamic Sizing: The `AutoSizeText` widget adapts the font size dynamically, eliminating the need for manual adjustments for each screen size.
Readability: By automatically fitting the text within the available space, it ensures that the content remains easily readable on any device.
Space Optimization: AutoSizeText optimizes the space, preventing text overflow or excessive white spaces, and creating a clean and professional appearance.
Ideal Scenarios for AutoSizeText:
Text-Heavy Layouts: When dealing with content-rich screens containing various text elements, `AutoSizeText` ensures a harmonious display of all text components.
Multi-Screen Support: In scenarios where your app needs to run on multiple devices with varying screen dimensions, this widget simplifies the font-sizing process.
Localization: For apps with multilingual support, `AutoSizeText` handles different text lengths gracefully, accommodating translations without layout disruptions.
Leveraging the `AutoSizeText` widget empowers developers to create adaptive and user-friendly interfaces, enabling a smooth and consistent user experience across devices. Next, let's explore another method for achieving responsive font sizing in Flutter.
Method 1 - Using the AutoSizeText Widget

Flutter offers a convenient solution for responsive font sizing through the `AutoSizeText` widget. This built-in widget automatically adjusts the font size of text based on the available space within its parent widget. It allows developers to set a range of font sizes, and the `AutoSizeText` widget intelligently calculates the ideal font size to fit the available space, ensuring readability on different screen sizes.
Code Example:
AutoSizeText(
'Sample text',
minFontSize: 12,
maxFontSize: 20,
overflow: TextOverflow.ellipsis,
)
Advantages of Using AutoSizeText:
Dynamic Sizing: The `AutoSizeText` widget adapts the font size dynamically, eliminating the need for manual adjustments for each screen size.
Readability: By automatically fitting the text within the available space, it ensures that the content remains easily readable on any device.
Space Optimization: AutoSizeText optimizes the space, preventing text overflow or excessive white spaces, and creating a clean and professional appearance.
Ideal Scenarios for AutoSizeText:
Text-Heavy Layouts: When dealing with content-rich screens containing various text elements, `AutoSizeText` ensures a harmonious display of all text components.
Multi-Screen Support: In scenarios where your app needs to run on multiple devices with varying screen dimensions, this widget simplifies the font-sizing process.
Localization: For apps with multilingual support, `AutoSizeText` handles different text lengths gracefully, accommodating translations without layout disruptions.
Leveraging the `AutoSizeText` widget empowers developers to create adaptive and user-friendly interfaces, enabling a smooth and consistent user experience across devices. Next, let's explore another method for achieving responsive font sizing in Flutter.
Method 1 - Using the AutoSizeText Widget

Flutter offers a convenient solution for responsive font sizing through the `AutoSizeText` widget. This built-in widget automatically adjusts the font size of text based on the available space within its parent widget. It allows developers to set a range of font sizes, and the `AutoSizeText` widget intelligently calculates the ideal font size to fit the available space, ensuring readability on different screen sizes.
Code Example:
AutoSizeText(
'Sample text',
minFontSize: 12,
maxFontSize: 20,
overflow: TextOverflow.ellipsis,
)
Advantages of Using AutoSizeText:
Dynamic Sizing: The `AutoSizeText` widget adapts the font size dynamically, eliminating the need for manual adjustments for each screen size.
Readability: By automatically fitting the text within the available space, it ensures that the content remains easily readable on any device.
Space Optimization: AutoSizeText optimizes the space, preventing text overflow or excessive white spaces, and creating a clean and professional appearance.
Ideal Scenarios for AutoSizeText:
Text-Heavy Layouts: When dealing with content-rich screens containing various text elements, `AutoSizeText` ensures a harmonious display of all text components.
Multi-Screen Support: In scenarios where your app needs to run on multiple devices with varying screen dimensions, this widget simplifies the font-sizing process.
Localization: For apps with multilingual support, `AutoSizeText` handles different text lengths gracefully, accommodating translations without layout disruptions.
Leveraging the `AutoSizeText` widget empowers developers to create adaptive and user-friendly interfaces, enabling a smooth and consistent user experience across devices. Next, let's explore another method for achieving responsive font sizing in Flutter.
FittedBox Widget
FittedBox Widget
FittedBox Widget
FittedBox Widget
Method 2 - Using the FittedBox Widget
The `FittedBox` widget in Flutter is another valuable tool for achieving responsive font sizing. It automatically scales the text to fit within the available space without exceeding the bounds of its parent widget. By wrapping the text widget with `FittedBox`, the text will dynamically resize, maintaining its aspect ratio and preventing overflow or text truncation.
Code Example:
FittedBox(
fit: BoxFit.scaleDown,
child: Text(
'This is some text that will resize based on the screen size.',
style: TextStyle(fontSize: 16),
),
)
Advantages of Using FittedBox:
Specific Font Size: Unlike the `AutoSizeText` widget, which automatically determines the font size, `FittedBox` allows developers to set a specific font size for the text while ensuring it scales appropriately.
Responsive Text: The `FittedBox` widget scales the text proportionally to fit the available space, making it a reliable choice for maintaining the text's legibility across different screen sizes.
Use Cases for FittedBox:
Fixed Font Size: When developers prefer to have a constant font size, `FittedBox` ensures that the text adapts smoothly to various screen sizes, providing a consistent user experience.
Text with Background: If the text needs to be displayed on top of a background image or a complex UI component, using `FittedBox` guarantees that the text remains within the desired boundaries without overlapping or becoming illegible.
Buttons and Icons: In scenarios where text is used in conjunction with buttons or icons, `FittedBox` ensures that the text scales appropriately, maintaining visual harmony within the overall design.
By incorporating the `FittedBox` widget into your Flutter app, you can easily manage the font size while preserving the responsiveness of the text. Now that we have explored two effective methods, let's delve into the third approach for responsive font sizing using the `LayoutBuilder` widget.
Method 2 - Using the FittedBox Widget
The `FittedBox` widget in Flutter is another valuable tool for achieving responsive font sizing. It automatically scales the text to fit within the available space without exceeding the bounds of its parent widget. By wrapping the text widget with `FittedBox`, the text will dynamically resize, maintaining its aspect ratio and preventing overflow or text truncation.
Code Example:
FittedBox(
fit: BoxFit.scaleDown,
child: Text(
'This is some text that will resize based on the screen size.',
style: TextStyle(fontSize: 16),
),
)
Advantages of Using FittedBox:
Specific Font Size: Unlike the `AutoSizeText` widget, which automatically determines the font size, `FittedBox` allows developers to set a specific font size for the text while ensuring it scales appropriately.
Responsive Text: The `FittedBox` widget scales the text proportionally to fit the available space, making it a reliable choice for maintaining the text's legibility across different screen sizes.
Use Cases for FittedBox:
Fixed Font Size: When developers prefer to have a constant font size, `FittedBox` ensures that the text adapts smoothly to various screen sizes, providing a consistent user experience.
Text with Background: If the text needs to be displayed on top of a background image or a complex UI component, using `FittedBox` guarantees that the text remains within the desired boundaries without overlapping or becoming illegible.
Buttons and Icons: In scenarios where text is used in conjunction with buttons or icons, `FittedBox` ensures that the text scales appropriately, maintaining visual harmony within the overall design.
By incorporating the `FittedBox` widget into your Flutter app, you can easily manage the font size while preserving the responsiveness of the text. Now that we have explored two effective methods, let's delve into the third approach for responsive font sizing using the `LayoutBuilder` widget.
Method 2 - Using the FittedBox Widget
The `FittedBox` widget in Flutter is another valuable tool for achieving responsive font sizing. It automatically scales the text to fit within the available space without exceeding the bounds of its parent widget. By wrapping the text widget with `FittedBox`, the text will dynamically resize, maintaining its aspect ratio and preventing overflow or text truncation.
Code Example:
FittedBox(
fit: BoxFit.scaleDown,
child: Text(
'This is some text that will resize based on the screen size.',
style: TextStyle(fontSize: 16),
),
)
Advantages of Using FittedBox:
Specific Font Size: Unlike the `AutoSizeText` widget, which automatically determines the font size, `FittedBox` allows developers to set a specific font size for the text while ensuring it scales appropriately.
Responsive Text: The `FittedBox` widget scales the text proportionally to fit the available space, making it a reliable choice for maintaining the text's legibility across different screen sizes.
Use Cases for FittedBox:
Fixed Font Size: When developers prefer to have a constant font size, `FittedBox` ensures that the text adapts smoothly to various screen sizes, providing a consistent user experience.
Text with Background: If the text needs to be displayed on top of a background image or a complex UI component, using `FittedBox` guarantees that the text remains within the desired boundaries without overlapping or becoming illegible.
Buttons and Icons: In scenarios where text is used in conjunction with buttons or icons, `FittedBox` ensures that the text scales appropriately, maintaining visual harmony within the overall design.
By incorporating the `FittedBox` widget into your Flutter app, you can easily manage the font size while preserving the responsiveness of the text. Now that we have explored two effective methods, let's delve into the third approach for responsive font sizing using the `LayoutBuilder` widget.
Method 2 - Using the FittedBox Widget
The `FittedBox` widget in Flutter is another valuable tool for achieving responsive font sizing. It automatically scales the text to fit within the available space without exceeding the bounds of its parent widget. By wrapping the text widget with `FittedBox`, the text will dynamically resize, maintaining its aspect ratio and preventing overflow or text truncation.
Code Example:
FittedBox(
fit: BoxFit.scaleDown,
child: Text(
'This is some text that will resize based on the screen size.',
style: TextStyle(fontSize: 16),
),
)
Advantages of Using FittedBox:
Specific Font Size: Unlike the `AutoSizeText` widget, which automatically determines the font size, `FittedBox` allows developers to set a specific font size for the text while ensuring it scales appropriately.
Responsive Text: The `FittedBox` widget scales the text proportionally to fit the available space, making it a reliable choice for maintaining the text's legibility across different screen sizes.
Use Cases for FittedBox:
Fixed Font Size: When developers prefer to have a constant font size, `FittedBox` ensures that the text adapts smoothly to various screen sizes, providing a consistent user experience.
Text with Background: If the text needs to be displayed on top of a background image or a complex UI component, using `FittedBox` guarantees that the text remains within the desired boundaries without overlapping or becoming illegible.
Buttons and Icons: In scenarios where text is used in conjunction with buttons or icons, `FittedBox` ensures that the text scales appropriately, maintaining visual harmony within the overall design.
By incorporating the `FittedBox` widget into your Flutter app, you can easily manage the font size while preserving the responsiveness of the text. Now that we have explored two effective methods, let's delve into the third approach for responsive font sizing using the `LayoutBuilder` widget.
TextScaleFactor
TextScaleFactor
TextScaleFactor
TextScaleFactor
Method 3 - Utilizing the textScaleFactor Property
In Flutter, the `textScaleFactor` property is a powerful tool for achieving a consistent font size across the entire app. Unlike the previous two methods, this property is applied globally and automatically scales the font size of all text widgets based on the user's device settings. Users can adjust the text size from their device settings, and the `textScaleFactor` property will dynamically adapt the font size accordingly.
Code Example:
return MaterialApp(
textScaleFactor: 1.2,
// Other app configurations and routes
Benefits of Using textScaleFactor:
Consistent User Experience: By using the `textScaleFactor` property, developers ensure that all text within the app adheres to the user's preferred text size. This promotes inclusivity and provides a seamless experience for users with different visual needs.
Easy Implementation: Unlike the previous methods, which require wrapping specific text widgets, setting the `textScaleFactor` property in the `MaterialApp` widget automatically applies the scaling to all text elements throughout the app.
Accessibility Support: Inclusivity is a critical aspect of app development, and by leveraging the `textScaleFactor`, developers can cater to users with various vision impairments, making their app more accessible.
When to Use textScaleFactor:
Consistent Text Size: If your app's design requires all text elements to have the same font size, the `textScaleFactor` is an ideal choice for maintaining uniformity.
Accessibility-Focused Apps: Apps that prioritize accessibility and aim to accommodate a wide range of users can greatly benefit from the automatic text scaling provided by the `textScaleFactor`.
User-Friendly Interface: When developing apps that prioritize user experience and user preferences, incorporating `textScaleFactor` allows users to customize the text size to their comfort.
By leveraging the `textScaleFactor` property, developers can effortlessly create apps with consistent and adaptable font sizing, ensuring that all users have a delightful experience with their Flutter application. With all three methods explored, let's proceed to the conclusion, summarizing the importance of responsive font sizing and how these techniques can enhance your Flutter app's user interface.
Method 3 - Utilizing the textScaleFactor Property
In Flutter, the `textScaleFactor` property is a powerful tool for achieving a consistent font size across the entire app. Unlike the previous two methods, this property is applied globally and automatically scales the font size of all text widgets based on the user's device settings. Users can adjust the text size from their device settings, and the `textScaleFactor` property will dynamically adapt the font size accordingly.
Code Example:
return MaterialApp(
textScaleFactor: 1.2,
// Other app configurations and routes
Benefits of Using textScaleFactor:
Consistent User Experience: By using the `textScaleFactor` property, developers ensure that all text within the app adheres to the user's preferred text size. This promotes inclusivity and provides a seamless experience for users with different visual needs.
Easy Implementation: Unlike the previous methods, which require wrapping specific text widgets, setting the `textScaleFactor` property in the `MaterialApp` widget automatically applies the scaling to all text elements throughout the app.
Accessibility Support: Inclusivity is a critical aspect of app development, and by leveraging the `textScaleFactor`, developers can cater to users with various vision impairments, making their app more accessible.
When to Use textScaleFactor:
Consistent Text Size: If your app's design requires all text elements to have the same font size, the `textScaleFactor` is an ideal choice for maintaining uniformity.
Accessibility-Focused Apps: Apps that prioritize accessibility and aim to accommodate a wide range of users can greatly benefit from the automatic text scaling provided by the `textScaleFactor`.
User-Friendly Interface: When developing apps that prioritize user experience and user preferences, incorporating `textScaleFactor` allows users to customize the text size to their comfort.
By leveraging the `textScaleFactor` property, developers can effortlessly create apps with consistent and adaptable font sizing, ensuring that all users have a delightful experience with their Flutter application. With all three methods explored, let's proceed to the conclusion, summarizing the importance of responsive font sizing and how these techniques can enhance your Flutter app's user interface.
Method 3 - Utilizing the textScaleFactor Property
In Flutter, the `textScaleFactor` property is a powerful tool for achieving a consistent font size across the entire app. Unlike the previous two methods, this property is applied globally and automatically scales the font size of all text widgets based on the user's device settings. Users can adjust the text size from their device settings, and the `textScaleFactor` property will dynamically adapt the font size accordingly.
Code Example:
return MaterialApp(
textScaleFactor: 1.2,
// Other app configurations and routes
Benefits of Using textScaleFactor:
Consistent User Experience: By using the `textScaleFactor` property, developers ensure that all text within the app adheres to the user's preferred text size. This promotes inclusivity and provides a seamless experience for users with different visual needs.
Easy Implementation: Unlike the previous methods, which require wrapping specific text widgets, setting the `textScaleFactor` property in the `MaterialApp` widget automatically applies the scaling to all text elements throughout the app.
Accessibility Support: Inclusivity is a critical aspect of app development, and by leveraging the `textScaleFactor`, developers can cater to users with various vision impairments, making their app more accessible.
When to Use textScaleFactor:
Consistent Text Size: If your app's design requires all text elements to have the same font size, the `textScaleFactor` is an ideal choice for maintaining uniformity.
Accessibility-Focused Apps: Apps that prioritize accessibility and aim to accommodate a wide range of users can greatly benefit from the automatic text scaling provided by the `textScaleFactor`.
User-Friendly Interface: When developing apps that prioritize user experience and user preferences, incorporating `textScaleFactor` allows users to customize the text size to their comfort.
By leveraging the `textScaleFactor` property, developers can effortlessly create apps with consistent and adaptable font sizing, ensuring that all users have a delightful experience with their Flutter application. With all three methods explored, let's proceed to the conclusion, summarizing the importance of responsive font sizing and how these techniques can enhance your Flutter app's user interface.
Method 3 - Utilizing the textScaleFactor Property
In Flutter, the `textScaleFactor` property is a powerful tool for achieving a consistent font size across the entire app. Unlike the previous two methods, this property is applied globally and automatically scales the font size of all text widgets based on the user's device settings. Users can adjust the text size from their device settings, and the `textScaleFactor` property will dynamically adapt the font size accordingly.
Code Example:
return MaterialApp(
textScaleFactor: 1.2,
// Other app configurations and routes
Benefits of Using textScaleFactor:
Consistent User Experience: By using the `textScaleFactor` property, developers ensure that all text within the app adheres to the user's preferred text size. This promotes inclusivity and provides a seamless experience for users with different visual needs.
Easy Implementation: Unlike the previous methods, which require wrapping specific text widgets, setting the `textScaleFactor` property in the `MaterialApp` widget automatically applies the scaling to all text elements throughout the app.
Accessibility Support: Inclusivity is a critical aspect of app development, and by leveraging the `textScaleFactor`, developers can cater to users with various vision impairments, making their app more accessible.
When to Use textScaleFactor:
Consistent Text Size: If your app's design requires all text elements to have the same font size, the `textScaleFactor` is an ideal choice for maintaining uniformity.
Accessibility-Focused Apps: Apps that prioritize accessibility and aim to accommodate a wide range of users can greatly benefit from the automatic text scaling provided by the `textScaleFactor`.
User-Friendly Interface: When developing apps that prioritize user experience and user preferences, incorporating `textScaleFactor` allows users to customize the text size to their comfort.
By leveraging the `textScaleFactor` property, developers can effortlessly create apps with consistent and adaptable font sizing, ensuring that all users have a delightful experience with their Flutter application. With all three methods explored, let's proceed to the conclusion, summarizing the importance of responsive font sizing and how these techniques can enhance your Flutter app's user interface.
Creating responsive apps
Creating responsive apps
Creating responsive apps
Creating responsive apps
Choosing the right method for your app to maintain Responsiveness
In summary, we have explored three effective methods for achieving responsive font sizing in Flutter:
1. AutoSizeText Widget:
Automatically sizes text based on available space.
Ideal for creating text that dynamically adjusts to different screen sizes.
Ensures readability and consistency across devices.
2. FittedBox Widget:
Scales text to fit available space without exceeding container bounds.
Perfect for maintaining a specific font size while making text responsive.
Useful in scenarios where precise control over font size is necessary.
3. textScaleFactor Property:
Globally scales font size based on user device settings.
Ensures a consistent user experience across the entire app.
Enhances accessibility and inclusivity.
Factors Influencing Method Selection:
App Design: Consider the app's overall design and whether a consistent or dynamic font size is more suitable for the user interface.
User Preferences: Prioritize user preferences and accessibility by using the textScaleFactor property to adapt to users' font size choices.
Readability: Choose the method that ensures optimal readability across various screen sizes and resolutions.
Importance of Testing and User Feedback:
Test the chosen method on different devices to verify its effectiveness and responsiveness.
Gather user feedback to identify any readability issues or user preferences regarding font size.
Adapt the method based on user feedback to continually improve the app's font sizing and user experience.
Choosing the right method for your app to maintain Responsiveness
In summary, we have explored three effective methods for achieving responsive font sizing in Flutter:
1. AutoSizeText Widget:
Automatically sizes text based on available space.
Ideal for creating text that dynamically adjusts to different screen sizes.
Ensures readability and consistency across devices.
2. FittedBox Widget:
Scales text to fit available space without exceeding container bounds.
Perfect for maintaining a specific font size while making text responsive.
Useful in scenarios where precise control over font size is necessary.
3. textScaleFactor Property:
Globally scales font size based on user device settings.
Ensures a consistent user experience across the entire app.
Enhances accessibility and inclusivity.
Factors Influencing Method Selection:
App Design: Consider the app's overall design and whether a consistent or dynamic font size is more suitable for the user interface.
User Preferences: Prioritize user preferences and accessibility by using the textScaleFactor property to adapt to users' font size choices.
Readability: Choose the method that ensures optimal readability across various screen sizes and resolutions.
Importance of Testing and User Feedback:
Test the chosen method on different devices to verify its effectiveness and responsiveness.
Gather user feedback to identify any readability issues or user preferences regarding font size.
Adapt the method based on user feedback to continually improve the app's font sizing and user experience.
Choosing the right method for your app to maintain Responsiveness
In summary, we have explored three effective methods for achieving responsive font sizing in Flutter:
1. AutoSizeText Widget:
Automatically sizes text based on available space.
Ideal for creating text that dynamically adjusts to different screen sizes.
Ensures readability and consistency across devices.
2. FittedBox Widget:
Scales text to fit available space without exceeding container bounds.
Perfect for maintaining a specific font size while making text responsive.
Useful in scenarios where precise control over font size is necessary.
3. textScaleFactor Property:
Globally scales font size based on user device settings.
Ensures a consistent user experience across the entire app.
Enhances accessibility and inclusivity.
Factors Influencing Method Selection:
App Design: Consider the app's overall design and whether a consistent or dynamic font size is more suitable for the user interface.
User Preferences: Prioritize user preferences and accessibility by using the textScaleFactor property to adapt to users' font size choices.
Readability: Choose the method that ensures optimal readability across various screen sizes and resolutions.
Importance of Testing and User Feedback:
Test the chosen method on different devices to verify its effectiveness and responsiveness.
Gather user feedback to identify any readability issues or user preferences regarding font size.
Adapt the method based on user feedback to continually improve the app's font sizing and user experience.
Choosing the right method for your app to maintain Responsiveness
In summary, we have explored three effective methods for achieving responsive font sizing in Flutter:
1. AutoSizeText Widget:
Automatically sizes text based on available space.
Ideal for creating text that dynamically adjusts to different screen sizes.
Ensures readability and consistency across devices.
2. FittedBox Widget:
Scales text to fit available space without exceeding container bounds.
Perfect for maintaining a specific font size while making text responsive.
Useful in scenarios where precise control over font size is necessary.
3. textScaleFactor Property:
Globally scales font size based on user device settings.
Ensures a consistent user experience across the entire app.
Enhances accessibility and inclusivity.
Factors Influencing Method Selection:
App Design: Consider the app's overall design and whether a consistent or dynamic font size is more suitable for the user interface.
User Preferences: Prioritize user preferences and accessibility by using the textScaleFactor property to adapt to users' font size choices.
Readability: Choose the method that ensures optimal readability across various screen sizes and resolutions.
Importance of Testing and User Feedback:
Test the chosen method on different devices to verify its effectiveness and responsiveness.
Gather user feedback to identify any readability issues or user preferences regarding font size.
Adapt the method based on user feedback to continually improve the app's font sizing and user experience.
Frequently asked questions (FAQ)
Frequently asked questions (FAQ)
Frequently asked questions (FAQ)
Frequently asked questions (FAQ)
Adjust Flutter Font Size
Q: How do I auto-resize text in Flutter?
A: You can use the AutoSizeText
widget in Flutter to automatically resize text based on available space. Here's a basic example:
AutoSizeText(
'Sample text',
minFontSize: 12,
maxFontSize: 20,
overflow: TextOverflow.ellipsis,
)
Q: How do you make text smaller in Flutter?
A: One way to make text smaller in Flutter is by adjusting the font size property of the Text
widget. Alternatively, you can use the AutoSizeText
widget to automatically resize text based on available space.
Q: How do I make text font size responsive in Flutter?
A: To make text font size responsive in Flutter, you can use techniques such as the AutoSizeText
widget, FittedBox
widget, or setting the textScaleFactor
property in the MaterialApp
widget.
Q: What is the text scale factor in Flutter?
A: The text scale factor in Flutter is a property that globally adjusts the font size of all text elements within the app based on the specified factor. It is typically set in the MaterialApp
widget.
Flutter Change Font Size Dynamically
Q: How do I change font size dynamically in Flutter?
A: You can change font size dynamically in Flutter by updating the font-size property of the Text
widget or by using widgets like AutoSizeText
or FittedBox
to adjust font size based on available space.
Q: How do you use AutoSizeText in Flutter?
A: To use AutoSizeText
in Flutter, simply wrap your text within the AutoSizeText
widget and specify the desired range for font sizes using the minFontSize
and maxFontSize
parameters.
Q: What does the FittedBox widget do in Flutter?
A: The FittedBox
widget in Flutter scales its child widget to fit within its constraints without exceeding its original size. It's commonly used to adjust text size dynamically based on available space.
Q: What is the minimum font size for the screen?
A: The minimum font size for the screen can vary depending on factors such as screen resolution and user preferences. However, you can specify a minimum font size using the minFontSize
parameter in the AutoSizeText
widget to ensure readability.
Adjust Flutter Font Size
Q: How do I auto-resize text in Flutter?
A: You can use the AutoSizeText
widget in Flutter to automatically resize text based on available space. Here's a basic example:
AutoSizeText(
'Sample text',
minFontSize: 12,
maxFontSize: 20,
overflow: TextOverflow.ellipsis,
)
Q: How do you make text smaller in Flutter?
A: One way to make text smaller in Flutter is by adjusting the font size property of the Text
widget. Alternatively, you can use the AutoSizeText
widget to automatically resize text based on available space.
Q: How do I make text font size responsive in Flutter?
A: To make text font size responsive in Flutter, you can use techniques such as the AutoSizeText
widget, FittedBox
widget, or setting the textScaleFactor
property in the MaterialApp
widget.
Q: What is the text scale factor in Flutter?
A: The text scale factor in Flutter is a property that globally adjusts the font size of all text elements within the app based on the specified factor. It is typically set in the MaterialApp
widget.
Flutter Change Font Size Dynamically
Q: How do I change font size dynamically in Flutter?
A: You can change font size dynamically in Flutter by updating the font-size property of the Text
widget or by using widgets like AutoSizeText
or FittedBox
to adjust font size based on available space.
Q: How do you use AutoSizeText in Flutter?
A: To use AutoSizeText
in Flutter, simply wrap your text within the AutoSizeText
widget and specify the desired range for font sizes using the minFontSize
and maxFontSize
parameters.
Q: What does the FittedBox widget do in Flutter?
A: The FittedBox
widget in Flutter scales its child widget to fit within its constraints without exceeding its original size. It's commonly used to adjust text size dynamically based on available space.
Q: What is the minimum font size for the screen?
A: The minimum font size for the screen can vary depending on factors such as screen resolution and user preferences. However, you can specify a minimum font size using the minFontSize
parameter in the AutoSizeText
widget to ensure readability.
Adjust Flutter Font Size
Q: How do I auto-resize text in Flutter?
A: You can use the AutoSizeText
widget in Flutter to automatically resize text based on available space. Here's a basic example:
AutoSizeText(
'Sample text',
minFontSize: 12,
maxFontSize: 20,
overflow: TextOverflow.ellipsis,
)
Q: How do you make text smaller in Flutter?
A: One way to make text smaller in Flutter is by adjusting the font size property of the Text
widget. Alternatively, you can use the AutoSizeText
widget to automatically resize text based on available space.
Q: How do I make text font size responsive in Flutter?
A: To make text font size responsive in Flutter, you can use techniques such as the AutoSizeText
widget, FittedBox
widget, or setting the textScaleFactor
property in the MaterialApp
widget.
Q: What is the text scale factor in Flutter?
A: The text scale factor in Flutter is a property that globally adjusts the font size of all text elements within the app based on the specified factor. It is typically set in the MaterialApp
widget.
Flutter Change Font Size Dynamically
Q: How do I change font size dynamically in Flutter?
A: You can change font size dynamically in Flutter by updating the font-size property of the Text
widget or by using widgets like AutoSizeText
or FittedBox
to adjust font size based on available space.
Q: How do you use AutoSizeText in Flutter?
A: To use AutoSizeText
in Flutter, simply wrap your text within the AutoSizeText
widget and specify the desired range for font sizes using the minFontSize
and maxFontSize
parameters.
Q: What does the FittedBox widget do in Flutter?
A: The FittedBox
widget in Flutter scales its child widget to fit within its constraints without exceeding its original size. It's commonly used to adjust text size dynamically based on available space.
Q: What is the minimum font size for the screen?
A: The minimum font size for the screen can vary depending on factors such as screen resolution and user preferences. However, you can specify a minimum font size using the minFontSize
parameter in the AutoSizeText
widget to ensure readability.
Adjust Flutter Font Size
Q: How do I auto-resize text in Flutter?
A: You can use the AutoSizeText
widget in Flutter to automatically resize text based on available space. Here's a basic example:
AutoSizeText(
'Sample text',
minFontSize: 12,
maxFontSize: 20,
overflow: TextOverflow.ellipsis,
)
Q: How do you make text smaller in Flutter?
A: One way to make text smaller in Flutter is by adjusting the font size property of the Text
widget. Alternatively, you can use the AutoSizeText
widget to automatically resize text based on available space.
Q: How do I make text font size responsive in Flutter?
A: To make text font size responsive in Flutter, you can use techniques such as the AutoSizeText
widget, FittedBox
widget, or setting the textScaleFactor
property in the MaterialApp
widget.
Q: What is the text scale factor in Flutter?
A: The text scale factor in Flutter is a property that globally adjusts the font size of all text elements within the app based on the specified factor. It is typically set in the MaterialApp
widget.
Flutter Change Font Size Dynamically
Q: How do I change font size dynamically in Flutter?
A: You can change font size dynamically in Flutter by updating the font-size property of the Text
widget or by using widgets like AutoSizeText
or FittedBox
to adjust font size based on available space.
Q: How do you use AutoSizeText in Flutter?
A: To use AutoSizeText
in Flutter, simply wrap your text within the AutoSizeText
widget and specify the desired range for font sizes using the minFontSize
and maxFontSize
parameters.
Q: What does the FittedBox widget do in Flutter?
A: The FittedBox
widget in Flutter scales its child widget to fit within its constraints without exceeding its original size. It's commonly used to adjust text size dynamically based on available space.
Q: What is the minimum font size for the screen?
A: The minimum font size for the screen can vary depending on factors such as screen resolution and user preferences. However, you can specify a minimum font size using the minFontSize
parameter in the AutoSizeText
widget to ensure readability.
Conclusion
Conclusion
Conclusion
Conclusion
Conclusion on maintaining responsiveness in Flutter apps
In conclusion, responsive font sizing is a crucial aspect of creating a user-friendly and visually appealing Flutter app. By leveraging the AutoSizeText widget, FittedBox widget, or textScaleFactor property, developers can ensure that text elements dynamically adjust to different screen sizes, providing an enhanced user experience across various devices.
We encourage all Flutter developers to implement these techniques in their apps to achieve a consistent and adaptive font sizing approach. Flutter's versatility in handling responsive design allows developers to create mobile applications that not only look visually appealing but also cater to the diverse preferences and needs of users.
With these powerful methods at your disposal, you can confidently develop Flutter apps that deliver exceptional user experiences on every device, making your app stand out in today's competitive mobile landscape. Happy coding and building!
Conclusion on maintaining responsiveness in Flutter apps
In conclusion, responsive font sizing is a crucial aspect of creating a user-friendly and visually appealing Flutter app. By leveraging the AutoSizeText widget, FittedBox widget, or textScaleFactor property, developers can ensure that text elements dynamically adjust to different screen sizes, providing an enhanced user experience across various devices.
We encourage all Flutter developers to implement these techniques in their apps to achieve a consistent and adaptive font sizing approach. Flutter's versatility in handling responsive design allows developers to create mobile applications that not only look visually appealing but also cater to the diverse preferences and needs of users.
With these powerful methods at your disposal, you can confidently develop Flutter apps that deliver exceptional user experiences on every device, making your app stand out in today's competitive mobile landscape. Happy coding and building!
Conclusion on maintaining responsiveness in Flutter apps
In conclusion, responsive font sizing is a crucial aspect of creating a user-friendly and visually appealing Flutter app. By leveraging the AutoSizeText widget, FittedBox widget, or textScaleFactor property, developers can ensure that text elements dynamically adjust to different screen sizes, providing an enhanced user experience across various devices.
We encourage all Flutter developers to implement these techniques in their apps to achieve a consistent and adaptive font sizing approach. Flutter's versatility in handling responsive design allows developers to create mobile applications that not only look visually appealing but also cater to the diverse preferences and needs of users.
With these powerful methods at your disposal, you can confidently develop Flutter apps that deliver exceptional user experiences on every device, making your app stand out in today's competitive mobile landscape. Happy coding and building!
Conclusion on maintaining responsiveness in Flutter apps
In conclusion, responsive font sizing is a crucial aspect of creating a user-friendly and visually appealing Flutter app. By leveraging the AutoSizeText widget, FittedBox widget, or textScaleFactor property, developers can ensure that text elements dynamically adjust to different screen sizes, providing an enhanced user experience across various devices.
We encourage all Flutter developers to implement these techniques in their apps to achieve a consistent and adaptive font sizing approach. Flutter's versatility in handling responsive design allows developers to create mobile applications that not only look visually appealing but also cater to the diverse preferences and needs of users.
With these powerful methods at your disposal, you can confidently develop Flutter apps that deliver exceptional user experiences on every device, making your app stand out in today's competitive mobile landscape. Happy coding and building!
Table of content
India(HQ)
9th Floor, Tower C, Logix Cyber Park,
C Block, Phase 2, Industrial Area, Sector 62, Noida, Uttar Pradesh, 201309
USA
2081 Center Street Berkeley,
CA 94704
© 2021-23 Blupx Private Limited.
All rights reserved.
India(HQ)
9th Floor, Tower C, Logix Cyber Park,
C Block, Phase 2, Industrial Area, Sector 62, Noida, Uttar Pradesh, 201309
USA
2081 Center Street Berkeley,
CA 94704
© 2021-23 Blupx Private Limited.
All rights reserved.
India(HQ)
9th Floor, Tower C, Logix Cyber Park,
C Block, Phase 2, Industrial Area, Sector 62, Noida, Uttar Pradesh, 201309
USA
2081 Center Street Berkeley,
CA 94704
© 2021-23 Blupx Private Limited.
All rights reserved.