Riverpod vs Provider: Which is good for Flutter State Management?
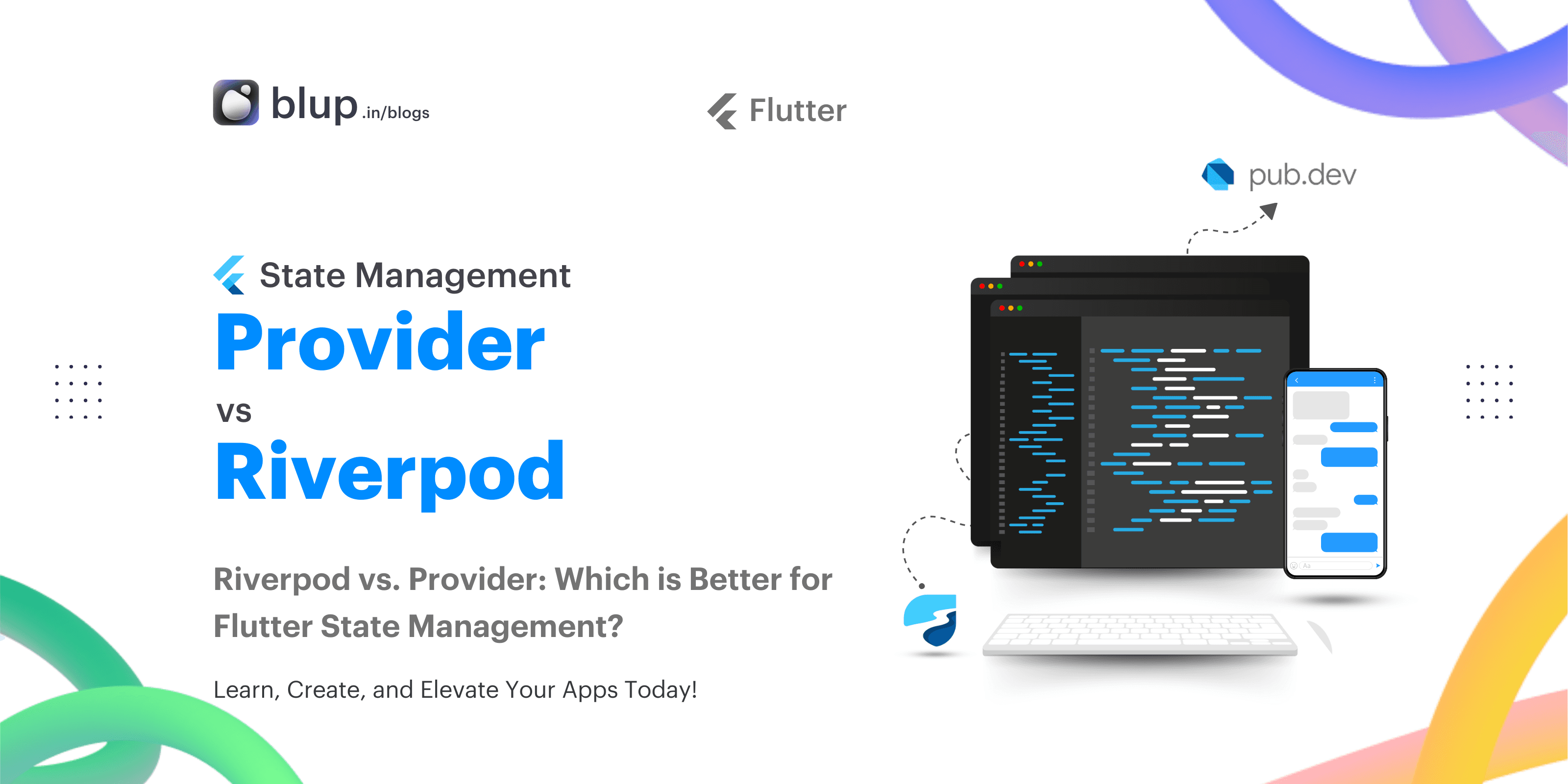
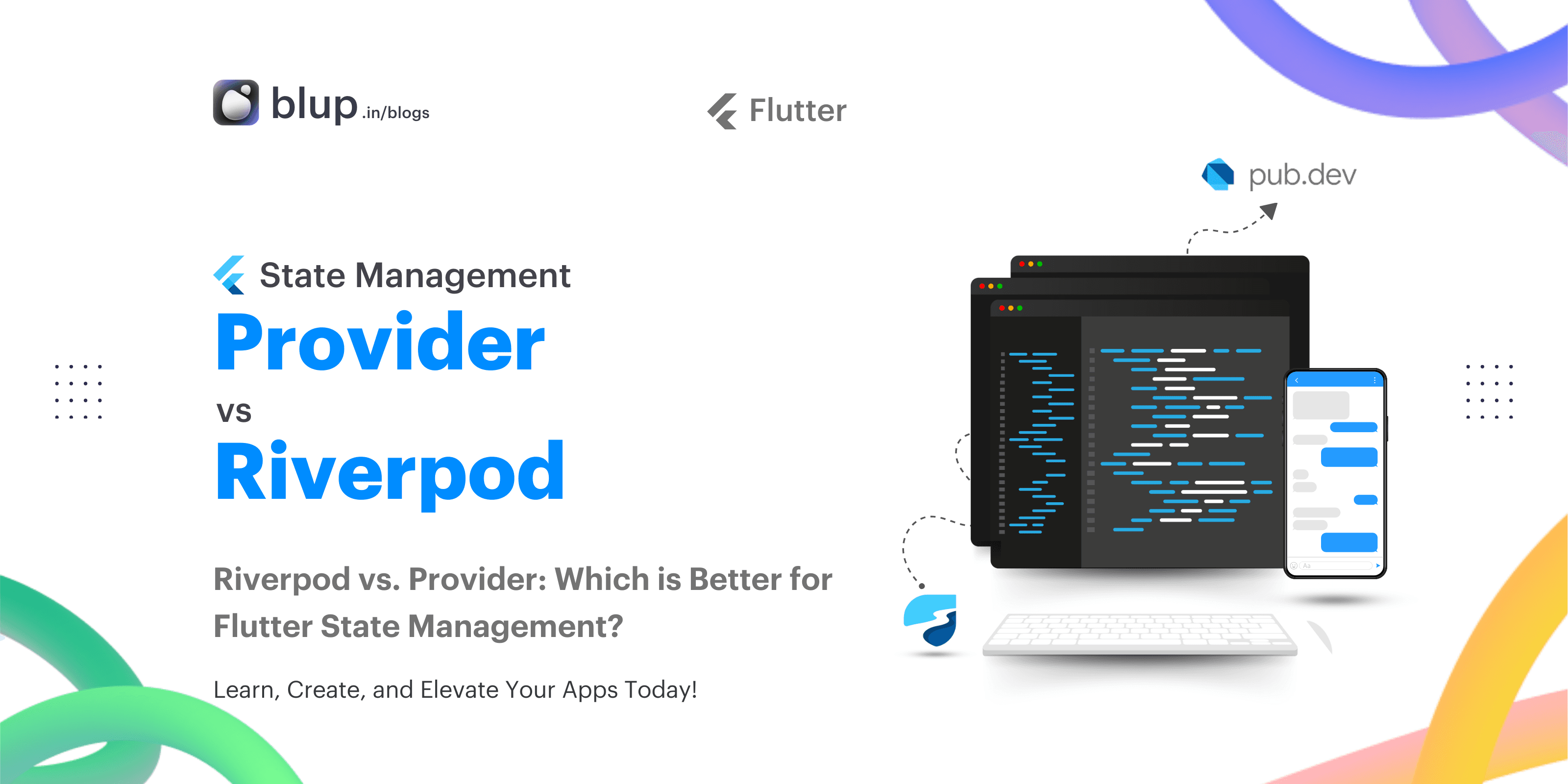
Introduction
Introduction
Introduction
Introduction
State management is a critical aspect of Flutter development, as it determines how data is handled and communicated across the app's components. Among the various state management solutions available, Provider and Riverpod are two popular choices, each offering unique features and benefits.
In this blog, we'll compare Riverpod and Provider to help you determine which is better suited for your Flutter applications, especially when aiming for scalable, production-ready solutions.
State management is a critical aspect of Flutter development, as it determines how data is handled and communicated across the app's components. Among the various state management solutions available, Provider and Riverpod are two popular choices, each offering unique features and benefits.
In this blog, we'll compare Riverpod and Provider to help you determine which is better suited for your Flutter applications, especially when aiming for scalable, production-ready solutions.
State management is a critical aspect of Flutter development, as it determines how data is handled and communicated across the app's components. Among the various state management solutions available, Provider and Riverpod are two popular choices, each offering unique features and benefits.
In this blog, we'll compare Riverpod and Provider to help you determine which is better suited for your Flutter applications, especially when aiming for scalable, production-ready solutions.
State management is a critical aspect of Flutter development, as it determines how data is handled and communicated across the app's components. Among the various state management solutions available, Provider and Riverpod are two popular choices, each offering unique features and benefits.
In this blog, we'll compare Riverpod and Provider to help you determine which is better suited for your Flutter applications, especially when aiming for scalable, production-ready solutions.
Understanding Provider
Understanding Provider
Understanding Provider
Understanding Provider
What is Provider?
Provider is a state management solution that simplifies the process of managing state in Flutter applications. Developed by the Flutter team, Provider builds on top of Flutter's existing InheritedWidget
to offer a more straightforward and powerful way to pass data down the widget tree. It's designed to be lightweight and easy to use, making it a popular choice among Flutter developers.
How Provider Works
At its core, Provider uses ChangeNotifier
and InheritedWidget
to propagate state changes to widgets. You wrap your widget tree with a Provider
, which exposes the state to its descendants. Widgets that need access to this state use Provider.of<T>(context)
or Consumer<T>
, allowing them to rebuild when the state changes.
Example Code with Provider
import 'package:flutter/material.dart';
import 'package:provider/provider.dart';
// Define a ChangeNotifier
class Counter with ChangeNotifier {
int _count = 0;
int get count => _count;
void increment() {
_count++;
notifyListeners();
}
}
void main() {
runApp(
ChangeNotifierProvider(
create: (context) => Counter(),
child: MyApp(),
),
);
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(title: Text('Provider Example')),
body: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
Text('Count: ${Provider.of<Counter>(context).count}'),
ElevatedButton(
onPressed: () => Provider.of<Counter>(context, listen: false).increment(),
child: Text('Increment'),
),
],
),
),
),
);
}
}
Typical use cases for Provider include managing simple states, such as user preferences or form inputs. It's well-suited for applications where the state management requirements are relatively straightforward and don't involve complex interactions.
Pros and Cons
Advantages:
Ease of Use: Provider's API is simple and integrates seamlessly with Flutter's widget system.
Community Support: Being one of the earliest solutions, it has a large user base and extensive documentation.
Performance: Provider’s approach to rebuilding widgets is efficient, minimizing unnecessary updates.
Disadvantages:
Scalability: As the complexity of the application increases, managing multiple providers can become cumbersome.
Limited Features: Provider lacks some advanced features found in newer solutions, such as scoped providers or improved testability.
What is Provider?
Provider is a state management solution that simplifies the process of managing state in Flutter applications. Developed by the Flutter team, Provider builds on top of Flutter's existing InheritedWidget
to offer a more straightforward and powerful way to pass data down the widget tree. It's designed to be lightweight and easy to use, making it a popular choice among Flutter developers.
How Provider Works
At its core, Provider uses ChangeNotifier
and InheritedWidget
to propagate state changes to widgets. You wrap your widget tree with a Provider
, which exposes the state to its descendants. Widgets that need access to this state use Provider.of<T>(context)
or Consumer<T>
, allowing them to rebuild when the state changes.
Example Code with Provider
import 'package:flutter/material.dart';
import 'package:provider/provider.dart';
// Define a ChangeNotifier
class Counter with ChangeNotifier {
int _count = 0;
int get count => _count;
void increment() {
_count++;
notifyListeners();
}
}
void main() {
runApp(
ChangeNotifierProvider(
create: (context) => Counter(),
child: MyApp(),
),
);
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(title: Text('Provider Example')),
body: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
Text('Count: ${Provider.of<Counter>(context).count}'),
ElevatedButton(
onPressed: () => Provider.of<Counter>(context, listen: false).increment(),
child: Text('Increment'),
),
],
),
),
),
);
}
}
Typical use cases for Provider include managing simple states, such as user preferences or form inputs. It's well-suited for applications where the state management requirements are relatively straightforward and don't involve complex interactions.
Pros and Cons
Advantages:
Ease of Use: Provider's API is simple and integrates seamlessly with Flutter's widget system.
Community Support: Being one of the earliest solutions, it has a large user base and extensive documentation.
Performance: Provider’s approach to rebuilding widgets is efficient, minimizing unnecessary updates.
Disadvantages:
Scalability: As the complexity of the application increases, managing multiple providers can become cumbersome.
Limited Features: Provider lacks some advanced features found in newer solutions, such as scoped providers or improved testability.
What is Provider?
Provider is a state management solution that simplifies the process of managing state in Flutter applications. Developed by the Flutter team, Provider builds on top of Flutter's existing InheritedWidget
to offer a more straightforward and powerful way to pass data down the widget tree. It's designed to be lightweight and easy to use, making it a popular choice among Flutter developers.
How Provider Works
At its core, Provider uses ChangeNotifier
and InheritedWidget
to propagate state changes to widgets. You wrap your widget tree with a Provider
, which exposes the state to its descendants. Widgets that need access to this state use Provider.of<T>(context)
or Consumer<T>
, allowing them to rebuild when the state changes.
Example Code with Provider
import 'package:flutter/material.dart';
import 'package:provider/provider.dart';
// Define a ChangeNotifier
class Counter with ChangeNotifier {
int _count = 0;
int get count => _count;
void increment() {
_count++;
notifyListeners();
}
}
void main() {
runApp(
ChangeNotifierProvider(
create: (context) => Counter(),
child: MyApp(),
),
);
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(title: Text('Provider Example')),
body: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
Text('Count: ${Provider.of<Counter>(context).count}'),
ElevatedButton(
onPressed: () => Provider.of<Counter>(context, listen: false).increment(),
child: Text('Increment'),
),
],
),
),
),
);
}
}
Typical use cases for Provider include managing simple states, such as user preferences or form inputs. It's well-suited for applications where the state management requirements are relatively straightforward and don't involve complex interactions.
Pros and Cons
Advantages:
Ease of Use: Provider's API is simple and integrates seamlessly with Flutter's widget system.
Community Support: Being one of the earliest solutions, it has a large user base and extensive documentation.
Performance: Provider’s approach to rebuilding widgets is efficient, minimizing unnecessary updates.
Disadvantages:
Scalability: As the complexity of the application increases, managing multiple providers can become cumbersome.
Limited Features: Provider lacks some advanced features found in newer solutions, such as scoped providers or improved testability.
What is Provider?
Provider is a state management solution that simplifies the process of managing state in Flutter applications. Developed by the Flutter team, Provider builds on top of Flutter's existing InheritedWidget
to offer a more straightforward and powerful way to pass data down the widget tree. It's designed to be lightweight and easy to use, making it a popular choice among Flutter developers.
How Provider Works
At its core, Provider uses ChangeNotifier
and InheritedWidget
to propagate state changes to widgets. You wrap your widget tree with a Provider
, which exposes the state to its descendants. Widgets that need access to this state use Provider.of<T>(context)
or Consumer<T>
, allowing them to rebuild when the state changes.
Example Code with Provider
import 'package:flutter/material.dart';
import 'package:provider/provider.dart';
// Define a ChangeNotifier
class Counter with ChangeNotifier {
int _count = 0;
int get count => _count;
void increment() {
_count++;
notifyListeners();
}
}
void main() {
runApp(
ChangeNotifierProvider(
create: (context) => Counter(),
child: MyApp(),
),
);
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(title: Text('Provider Example')),
body: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
Text('Count: ${Provider.of<Counter>(context).count}'),
ElevatedButton(
onPressed: () => Provider.of<Counter>(context, listen: false).increment(),
child: Text('Increment'),
),
],
),
),
),
);
}
}
Typical use cases for Provider include managing simple states, such as user preferences or form inputs. It's well-suited for applications where the state management requirements are relatively straightforward and don't involve complex interactions.
Pros and Cons
Advantages:
Ease of Use: Provider's API is simple and integrates seamlessly with Flutter's widget system.
Community Support: Being one of the earliest solutions, it has a large user base and extensive documentation.
Performance: Provider’s approach to rebuilding widgets is efficient, minimizing unnecessary updates.
Disadvantages:
Scalability: As the complexity of the application increases, managing multiple providers can become cumbersome.
Limited Features: Provider lacks some advanced features found in newer solutions, such as scoped providers or improved testability.
Exploring Riverpod
Exploring Riverpod
Exploring Riverpod
Exploring Riverpod
What is Riverpod?
Riverpod is a modern state management solution created by the same author as Provider. It addresses some of the limitations of Providers by offering a more flexible and powerful approach to state management. Riverpod introduces the concept of "providers" that are independent of the widget tree, which enhances modularity and scalability.
How Riverpod Works
Riverpod operates with the core concept of "providers" that manage and expose state. Providers are not tied to the widget tree, which means they can be accessed from anywhere in the app. This design promotes a more modular approach, where state management is decoupled from the UI.
Key components include:
Provider: A general-purpose provider that can hold and manage any type of state.
ScopedProvider: Allows for scoped access to state, useful for handling different state instances in various parts of the app.
Riverpod's flexibility allows it to handle complex state management scenarios, such as nested or asynchronous states, more gracefully than Provider.
Example Code with Riverpod
import 'package:flutter/material.dart';
import 'package:flutter_riverpod/flutter_riverpod.dart';
// Define a provider
final counterProvider = StateProvider<int>((ref) => 0);
void main() {
runApp(
ProviderScope(
child: MyApp(),
),
);
}
class MyApp extends ConsumerWidget {
@override
Widget build(BuildContext context, WidgetRef ref) {
final count = ref.watch(counterProvider);
return MaterialApp(
home: Scaffold(
appBar: AppBar(title: Text('Riverpod Example')),
body: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
Text('Count: $count'),
ElevatedButton(
onPressed: () => ref.read(counterProvider.notifier).state++,
child: Text('Increment'),
),
],
),
),
),
);
}
}
Pros and Cons
Advantages:
Enhanced Safety: Riverpod reduces the risk of bugs by enforcing stricter typing and scope management.
Better Testability: Providers can be tested independently of the widget tree, making unit testing more straightforward.
Modularity: The decoupling of providers from the widget tree enhances modularity and scalability.
Disadvantages:
Learning Curve: Riverpod introduces new concepts that may require additional learning for developers familiar with Provider.
Less Mature: Although Riverpod is rapidly gaining popularity, it is newer and may have fewer community resources compared to Provider.
What is Riverpod?
Riverpod is a modern state management solution created by the same author as Provider. It addresses some of the limitations of Providers by offering a more flexible and powerful approach to state management. Riverpod introduces the concept of "providers" that are independent of the widget tree, which enhances modularity and scalability.
How Riverpod Works
Riverpod operates with the core concept of "providers" that manage and expose state. Providers are not tied to the widget tree, which means they can be accessed from anywhere in the app. This design promotes a more modular approach, where state management is decoupled from the UI.
Key components include:
Provider: A general-purpose provider that can hold and manage any type of state.
ScopedProvider: Allows for scoped access to state, useful for handling different state instances in various parts of the app.
Riverpod's flexibility allows it to handle complex state management scenarios, such as nested or asynchronous states, more gracefully than Provider.
Example Code with Riverpod
import 'package:flutter/material.dart';
import 'package:flutter_riverpod/flutter_riverpod.dart';
// Define a provider
final counterProvider = StateProvider<int>((ref) => 0);
void main() {
runApp(
ProviderScope(
child: MyApp(),
),
);
}
class MyApp extends ConsumerWidget {
@override
Widget build(BuildContext context, WidgetRef ref) {
final count = ref.watch(counterProvider);
return MaterialApp(
home: Scaffold(
appBar: AppBar(title: Text('Riverpod Example')),
body: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
Text('Count: $count'),
ElevatedButton(
onPressed: () => ref.read(counterProvider.notifier).state++,
child: Text('Increment'),
),
],
),
),
),
);
}
}
Pros and Cons
Advantages:
Enhanced Safety: Riverpod reduces the risk of bugs by enforcing stricter typing and scope management.
Better Testability: Providers can be tested independently of the widget tree, making unit testing more straightforward.
Modularity: The decoupling of providers from the widget tree enhances modularity and scalability.
Disadvantages:
Learning Curve: Riverpod introduces new concepts that may require additional learning for developers familiar with Provider.
Less Mature: Although Riverpod is rapidly gaining popularity, it is newer and may have fewer community resources compared to Provider.
What is Riverpod?
Riverpod is a modern state management solution created by the same author as Provider. It addresses some of the limitations of Providers by offering a more flexible and powerful approach to state management. Riverpod introduces the concept of "providers" that are independent of the widget tree, which enhances modularity and scalability.
How Riverpod Works
Riverpod operates with the core concept of "providers" that manage and expose state. Providers are not tied to the widget tree, which means they can be accessed from anywhere in the app. This design promotes a more modular approach, where state management is decoupled from the UI.
Key components include:
Provider: A general-purpose provider that can hold and manage any type of state.
ScopedProvider: Allows for scoped access to state, useful for handling different state instances in various parts of the app.
Riverpod's flexibility allows it to handle complex state management scenarios, such as nested or asynchronous states, more gracefully than Provider.
Example Code with Riverpod
import 'package:flutter/material.dart';
import 'package:flutter_riverpod/flutter_riverpod.dart';
// Define a provider
final counterProvider = StateProvider<int>((ref) => 0);
void main() {
runApp(
ProviderScope(
child: MyApp(),
),
);
}
class MyApp extends ConsumerWidget {
@override
Widget build(BuildContext context, WidgetRef ref) {
final count = ref.watch(counterProvider);
return MaterialApp(
home: Scaffold(
appBar: AppBar(title: Text('Riverpod Example')),
body: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
Text('Count: $count'),
ElevatedButton(
onPressed: () => ref.read(counterProvider.notifier).state++,
child: Text('Increment'),
),
],
),
),
),
);
}
}
Pros and Cons
Advantages:
Enhanced Safety: Riverpod reduces the risk of bugs by enforcing stricter typing and scope management.
Better Testability: Providers can be tested independently of the widget tree, making unit testing more straightforward.
Modularity: The decoupling of providers from the widget tree enhances modularity and scalability.
Disadvantages:
Learning Curve: Riverpod introduces new concepts that may require additional learning for developers familiar with Provider.
Less Mature: Although Riverpod is rapidly gaining popularity, it is newer and may have fewer community resources compared to Provider.
What is Riverpod?
Riverpod is a modern state management solution created by the same author as Provider. It addresses some of the limitations of Providers by offering a more flexible and powerful approach to state management. Riverpod introduces the concept of "providers" that are independent of the widget tree, which enhances modularity and scalability.
How Riverpod Works
Riverpod operates with the core concept of "providers" that manage and expose state. Providers are not tied to the widget tree, which means they can be accessed from anywhere in the app. This design promotes a more modular approach, where state management is decoupled from the UI.
Key components include:
Provider: A general-purpose provider that can hold and manage any type of state.
ScopedProvider: Allows for scoped access to state, useful for handling different state instances in various parts of the app.
Riverpod's flexibility allows it to handle complex state management scenarios, such as nested or asynchronous states, more gracefully than Provider.
Example Code with Riverpod
import 'package:flutter/material.dart';
import 'package:flutter_riverpod/flutter_riverpod.dart';
// Define a provider
final counterProvider = StateProvider<int>((ref) => 0);
void main() {
runApp(
ProviderScope(
child: MyApp(),
),
);
}
class MyApp extends ConsumerWidget {
@override
Widget build(BuildContext context, WidgetRef ref) {
final count = ref.watch(counterProvider);
return MaterialApp(
home: Scaffold(
appBar: AppBar(title: Text('Riverpod Example')),
body: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
Text('Count: $count'),
ElevatedButton(
onPressed: () => ref.read(counterProvider.notifier).state++,
child: Text('Increment'),
),
],
),
),
),
);
}
}
Pros and Cons
Advantages:
Enhanced Safety: Riverpod reduces the risk of bugs by enforcing stricter typing and scope management.
Better Testability: Providers can be tested independently of the widget tree, making unit testing more straightforward.
Modularity: The decoupling of providers from the widget tree enhances modularity and scalability.
Disadvantages:
Learning Curve: Riverpod introduces new concepts that may require additional learning for developers familiar with Provider.
Less Mature: Although Riverpod is rapidly gaining popularity, it is newer and may have fewer community resources compared to Provider.
Comparison: Riverpod vs. Provider
Comparison: Riverpod vs. Provider
Comparison: Riverpod vs. Provider
Comparison: Riverpod vs. Provider
Riverpod and Provider each offer unique advantages for Flutter state management. Provider integrates seamlessly with Flutter's widget tree, using InheritedWidget
and ChangeNotifier
for straightforward state management. It’s easy to use but can become less efficient in complex applications.
In contrast, Riverpod decouples state management from the widget hierarchy, providing greater flexibility and scalability. It supports advanced features and better modularity, though it comes with a steeper learning curve. Riverpod excels in handling complex scenarios and offers superior testing capabilities, making it ideal for larger, scalable applications. Provider remains effective for simpler use cases.
Here's a comparative table highlighting the key differences between Riverpod and Provider:
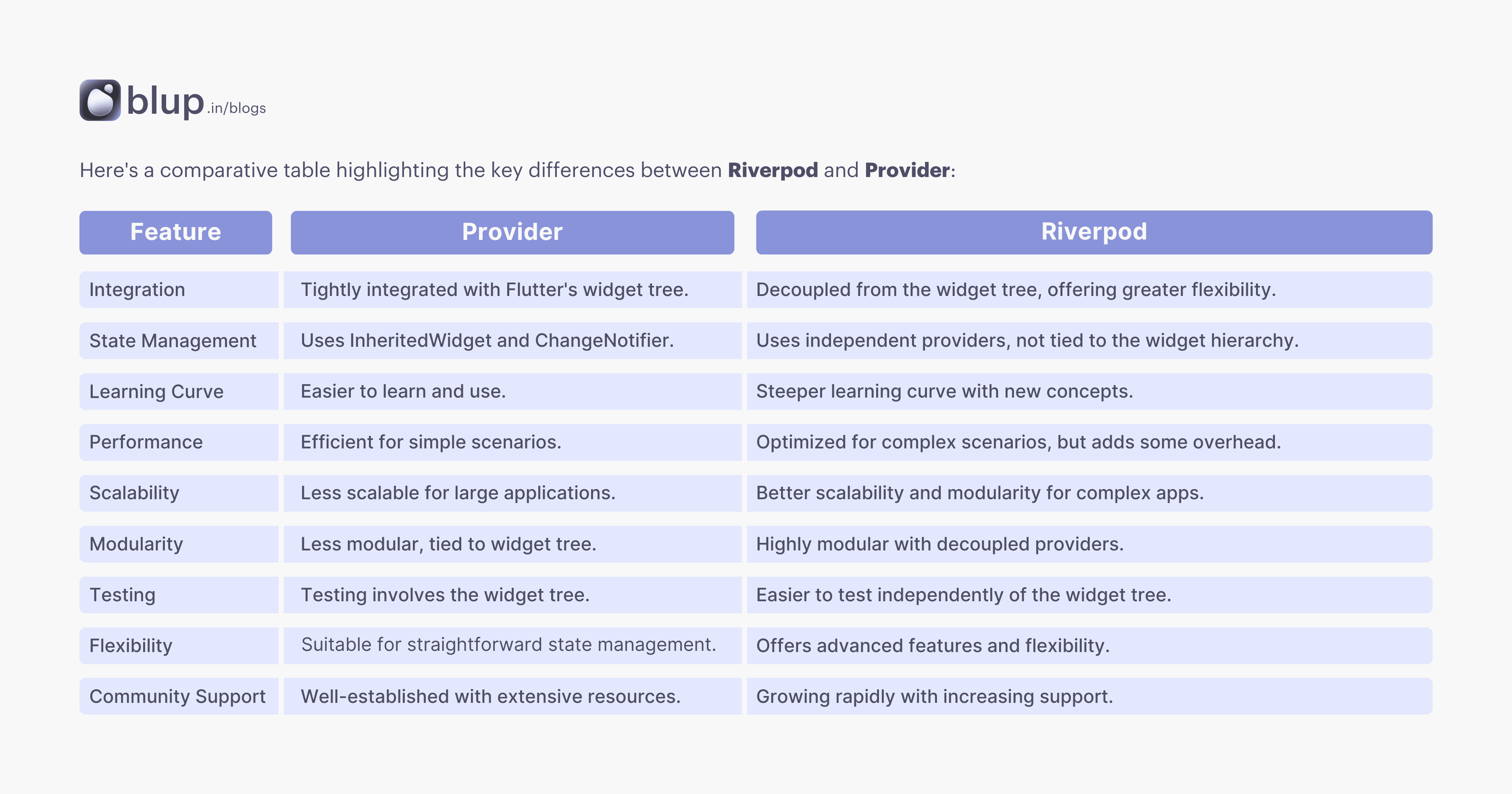
Riverpod and Provider each offer unique advantages for Flutter state management. Provider integrates seamlessly with Flutter's widget tree, using InheritedWidget
and ChangeNotifier
for straightforward state management. It’s easy to use but can become less efficient in complex applications.
In contrast, Riverpod decouples state management from the widget hierarchy, providing greater flexibility and scalability. It supports advanced features and better modularity, though it comes with a steeper learning curve. Riverpod excels in handling complex scenarios and offers superior testing capabilities, making it ideal for larger, scalable applications. Provider remains effective for simpler use cases.
Here's a comparative table highlighting the key differences between Riverpod and Provider:
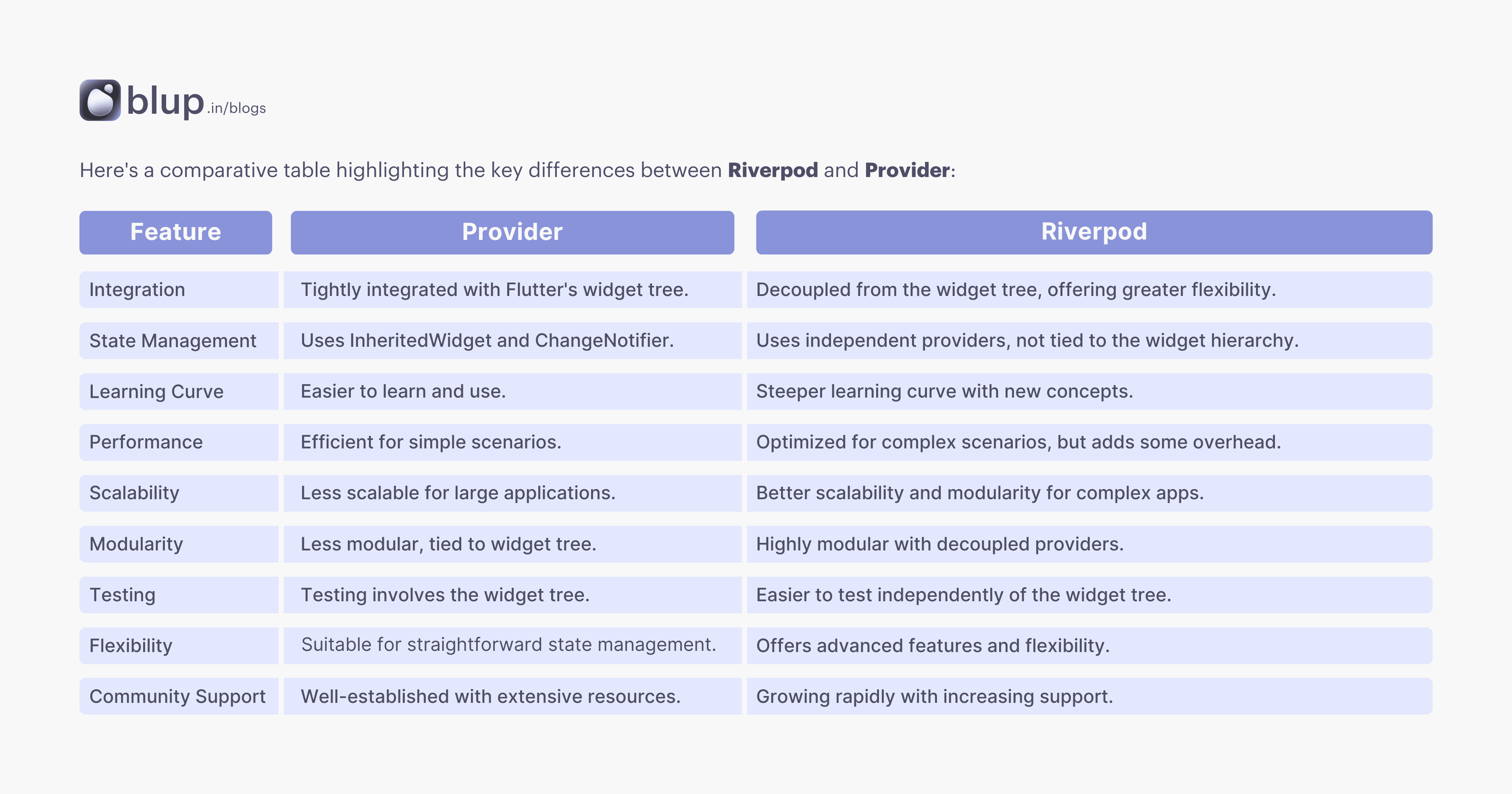
Riverpod and Provider each offer unique advantages for Flutter state management. Provider integrates seamlessly with Flutter's widget tree, using InheritedWidget
and ChangeNotifier
for straightforward state management. It’s easy to use but can become less efficient in complex applications.
In contrast, Riverpod decouples state management from the widget hierarchy, providing greater flexibility and scalability. It supports advanced features and better modularity, though it comes with a steeper learning curve. Riverpod excels in handling complex scenarios and offers superior testing capabilities, making it ideal for larger, scalable applications. Provider remains effective for simpler use cases.
Here's a comparative table highlighting the key differences between Riverpod and Provider:
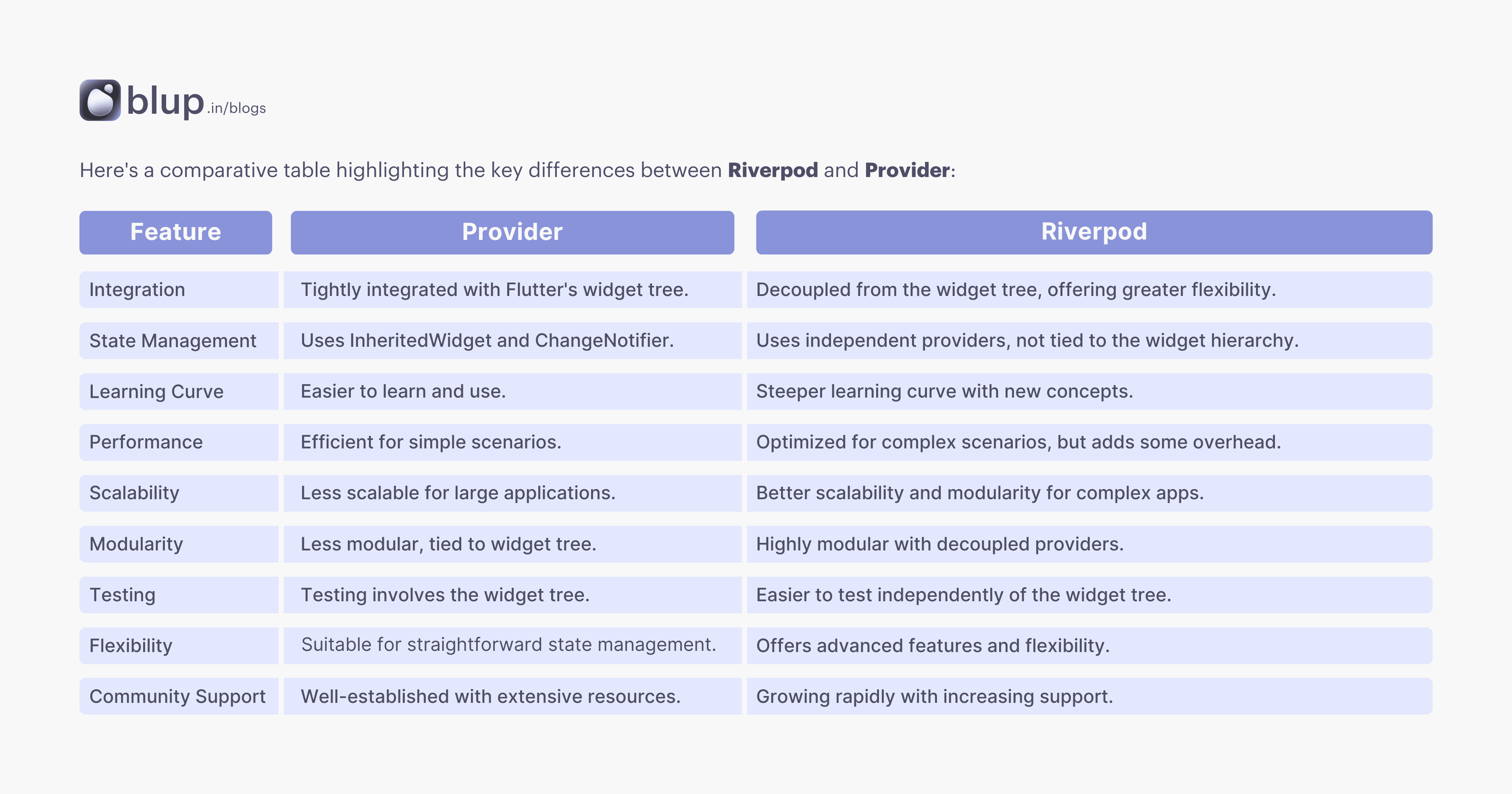
Riverpod and Provider each offer unique advantages for Flutter state management. Provider integrates seamlessly with Flutter's widget tree, using InheritedWidget
and ChangeNotifier
for straightforward state management. It’s easy to use but can become less efficient in complex applications.
In contrast, Riverpod decouples state management from the widget hierarchy, providing greater flexibility and scalability. It supports advanced features and better modularity, though it comes with a steeper learning curve. Riverpod excels in handling complex scenarios and offers superior testing capabilities, making it ideal for larger, scalable applications. Provider remains effective for simpler use cases.
Here's a comparative table highlighting the key differences between Riverpod and Provider:
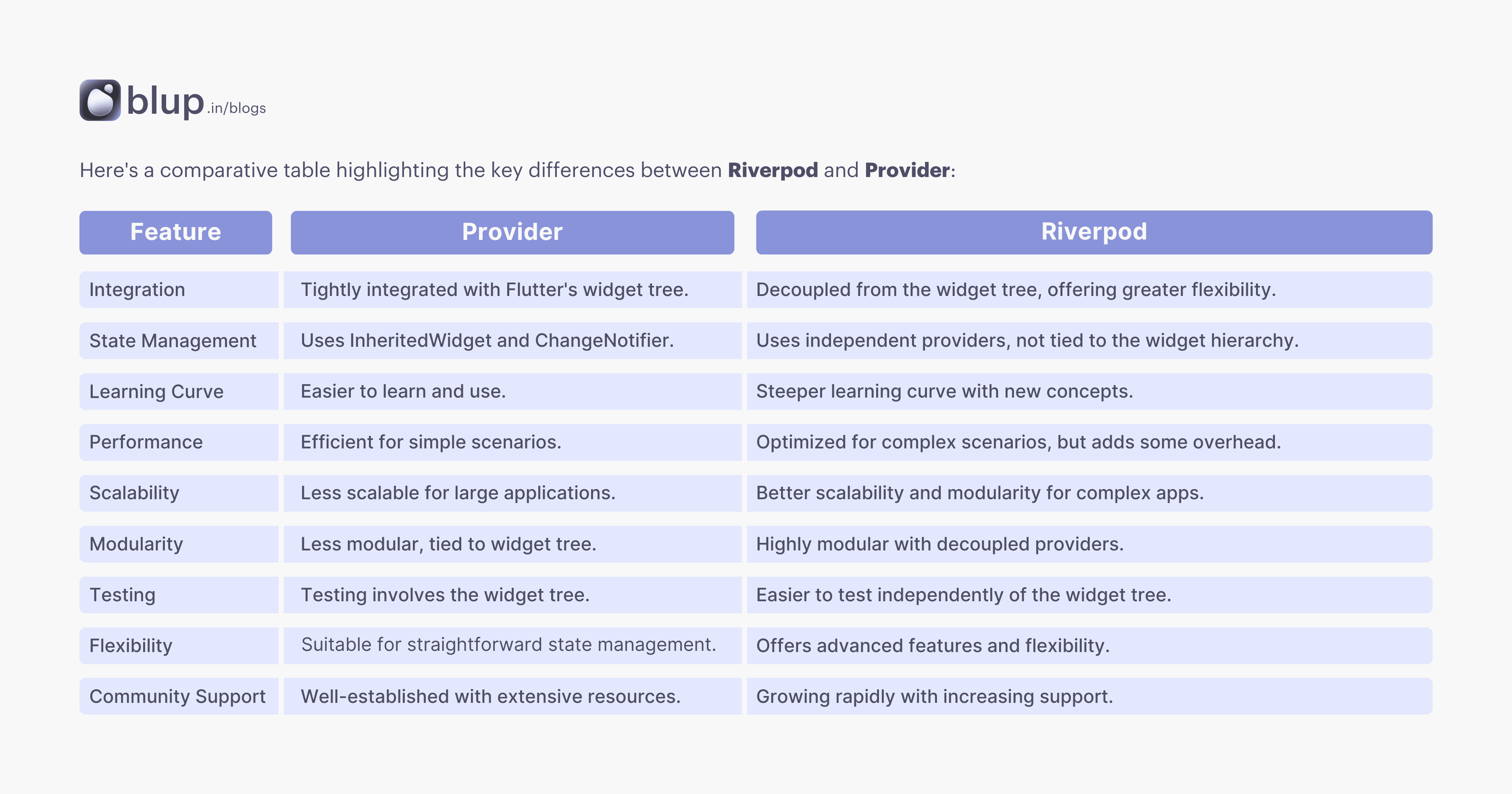
Testing and Debugging
Testing and Debugging
Testing and Debugging
Testing and Debugging
Riverpod offers superior testing and debugging capabilities compared to Provider. The decoupling of state from the widget tree allows for easier unit testing of individual providers without needing to build the entire widget tree. Provider’s approach, while functional, can make testing more challenging, as it involves interactions with the widget tree and InheritedWidget
.
Riverpod offers superior testing and debugging capabilities compared to Provider. The decoupling of state from the widget tree allows for easier unit testing of individual providers without needing to build the entire widget tree. Provider’s approach, while functional, can make testing more challenging, as it involves interactions with the widget tree and InheritedWidget
.
Riverpod offers superior testing and debugging capabilities compared to Provider. The decoupling of state from the widget tree allows for easier unit testing of individual providers without needing to build the entire widget tree. Provider’s approach, while functional, can make testing more challenging, as it involves interactions with the widget tree and InheritedWidget
.
Riverpod offers superior testing and debugging capabilities compared to Provider. The decoupling of state from the widget tree allows for easier unit testing of individual providers without needing to build the entire widget tree. Provider’s approach, while functional, can make testing more challenging, as it involves interactions with the widget tree and InheritedWidget
.
Case Studies and Real-World Use Cases
Case Studies and Real-World Use Cases
Case Studies and Real-World Use Cases
Case Studies and Real-World Use Cases
Provider in Action
Provider has been widely adopted in various applications due to its simplicity and effectiveness. For instance, many small to medium-sized apps use Provider to manage user settings, form state, or simple data synchronization. Its ease of integration with Flutter makes it a go-to choice for developers looking for a reliable yet straightforward solution.
Riverpod in Action
Riverpod is increasingly being adopted in applications that require more advanced state management features. For example, apps with complex data flow, multiple state instances, or extensive asynchronous operations benefit from Riverpod’s modular design. Large-scale projects or applications with intricate state requirements often find Riverpod’s features advantageous for maintaining clean and manageable codebases.
Provider in Action
Provider has been widely adopted in various applications due to its simplicity and effectiveness. For instance, many small to medium-sized apps use Provider to manage user settings, form state, or simple data synchronization. Its ease of integration with Flutter makes it a go-to choice for developers looking for a reliable yet straightforward solution.
Riverpod in Action
Riverpod is increasingly being adopted in applications that require more advanced state management features. For example, apps with complex data flow, multiple state instances, or extensive asynchronous operations benefit from Riverpod’s modular design. Large-scale projects or applications with intricate state requirements often find Riverpod’s features advantageous for maintaining clean and manageable codebases.
Provider in Action
Provider has been widely adopted in various applications due to its simplicity and effectiveness. For instance, many small to medium-sized apps use Provider to manage user settings, form state, or simple data synchronization. Its ease of integration with Flutter makes it a go-to choice for developers looking for a reliable yet straightforward solution.
Riverpod in Action
Riverpod is increasingly being adopted in applications that require more advanced state management features. For example, apps with complex data flow, multiple state instances, or extensive asynchronous operations benefit from Riverpod’s modular design. Large-scale projects or applications with intricate state requirements often find Riverpod’s features advantageous for maintaining clean and manageable codebases.
Provider in Action
Provider has been widely adopted in various applications due to its simplicity and effectiveness. For instance, many small to medium-sized apps use Provider to manage user settings, form state, or simple data synchronization. Its ease of integration with Flutter makes it a go-to choice for developers looking for a reliable yet straightforward solution.
Riverpod in Action
Riverpod is increasingly being adopted in applications that require more advanced state management features. For example, apps with complex data flow, multiple state instances, or extensive asynchronous operations benefit from Riverpod’s modular design. Large-scale projects or applications with intricate state requirements often find Riverpod’s features advantageous for maintaining clean and manageable codebases.
Conclusion
Conclusion
Conclusion
Conclusion
In summary, both Riverpod and Provider are effective state management solutions for Flutter, each with its strengths and weaknesses. Provider excels in ease of use and integration with Flutter’s widget system, making it suitable for simpler applications. Riverpod, with its advanced features and modular design, is better suited for complex and scalable applications.
Choosing between Riverpod and Provider depends on your specific needs and the complexity of your application. For those just starting with state management in Flutter, Provider offers a gentle introduction, while Riverpod provides a more robust solution for developers tackling more intricate state management challenges.
To make an informed decision, consider experimenting with both solutions in smaller projects to understand their benefits and trade-offs. Dive into their documentation, engage with the community, and explore real-world use cases to find the best fit for your application.
In summary, both Riverpod and Provider are effective state management solutions for Flutter, each with its strengths and weaknesses. Provider excels in ease of use and integration with Flutter’s widget system, making it suitable for simpler applications. Riverpod, with its advanced features and modular design, is better suited for complex and scalable applications.
Choosing between Riverpod and Provider depends on your specific needs and the complexity of your application. For those just starting with state management in Flutter, Provider offers a gentle introduction, while Riverpod provides a more robust solution for developers tackling more intricate state management challenges.
To make an informed decision, consider experimenting with both solutions in smaller projects to understand their benefits and trade-offs. Dive into their documentation, engage with the community, and explore real-world use cases to find the best fit for your application.
In summary, both Riverpod and Provider are effective state management solutions for Flutter, each with its strengths and weaknesses. Provider excels in ease of use and integration with Flutter’s widget system, making it suitable for simpler applications. Riverpod, with its advanced features and modular design, is better suited for complex and scalable applications.
Choosing between Riverpod and Provider depends on your specific needs and the complexity of your application. For those just starting with state management in Flutter, Provider offers a gentle introduction, while Riverpod provides a more robust solution for developers tackling more intricate state management challenges.
To make an informed decision, consider experimenting with both solutions in smaller projects to understand their benefits and trade-offs. Dive into their documentation, engage with the community, and explore real-world use cases to find the best fit for your application.
In summary, both Riverpod and Provider are effective state management solutions for Flutter, each with its strengths and weaknesses. Provider excels in ease of use and integration with Flutter’s widget system, making it suitable for simpler applications. Riverpod, with its advanced features and modular design, is better suited for complex and scalable applications.
Choosing between Riverpod and Provider depends on your specific needs and the complexity of your application. For those just starting with state management in Flutter, Provider offers a gentle introduction, while Riverpod provides a more robust solution for developers tackling more intricate state management challenges.
To make an informed decision, consider experimenting with both solutions in smaller projects to understand their benefits and trade-offs. Dive into their documentation, engage with the community, and explore real-world use cases to find the best fit for your application.
FAQs: Riverpod vs. Provider
FAQs: Riverpod vs. Provider
FAQs: Riverpod vs. Provider
FAQs: Riverpod vs. Provider
For further exploration, check out the official documentation for Provider and Riverpod. Share your experiences or ask questions in the comments to connect with other developers and learn from their insights.

Here are 10 frequently asked questions (FAQs) about Riverpod and Provider in Flutter:
FAQs
What are the main differences between Riverpod and Provider?
The provider is tightly integrated with Flutter's widget tree and is used
InheritedWidget
for state management. Riverpod decouples state management from the widget tree, offering more flexibility and modularity with providers not dependent on the widget hierarchy.
Which is easier to learn, Riverpod or Provider?
Provider is generally easier to learn due to its straightforward API and direct integration with Flutter's widget system. Riverpod introduces new concepts and requires a deeper understanding, which might present a steeper learning curve.
Can I use Riverpod and Provider for the same project?
Yes, you can use both Riverpod and Provider in the same project, but it’s typically advisable to stick with one solution to maintain consistency and avoid confusion.
How does performance compare between Riverpod and Provider?
Both Riverpod and Provider are performant for managing state, but Riverpod introduces additional layers of abstraction that might impact performance in specific scenarios. Provider is generally simpler and can be more efficient for straightforward use cases.
Is Riverpod more scalable than Provider?
Yes, Riverpod is designed to handle complex state management scenarios and large applications better than Provider. Its modular design and decoupling from the widget tree enhance scalability.
How does testing differ between Riverpod and Provider?
Riverpod offers better testability because its providers are independent of the widget tree, allowing for easier unit testing. Provider requires testing with the widget tree, which can be more challenging.
What are the main advantages of using Provider?
Provider is known for its simplicity, ease of use, and integration with Flutter's widget system. It has a large community and extensive documentation, making it a good choice for simpler state management needs.
What are the key benefits of using Riverpod?
Riverpod provides enhanced safety with stricter typing and scope management, better modularity, and improved testability. It’s suitable for complex state management requirements and large-scale applications.
Can Riverpod replace Provider in existing projects?
Yes, Riverpod can replace Provider in existing projects, but this would require refactoring the state management logic. Riverpod’s advanced features may offer benefits for new projects or those requiring more sophisticated state management.
Where can I find resources to learn more about Riverpod and Provider?
These FAQs should help clarify common concerns and guide developers in choosing the best state management solution for their Flutter applications. Here are 10 frequently asked questions (FAQs) about Riverpod and Provider in Flutter:
For further exploration, check out the official documentation for Provider and Riverpod. Share your experiences or ask questions in the comments to connect with other developers and learn from their insights.

Here are 10 frequently asked questions (FAQs) about Riverpod and Provider in Flutter:
FAQs
What are the main differences between Riverpod and Provider?
The provider is tightly integrated with Flutter's widget tree and is used
InheritedWidget
for state management. Riverpod decouples state management from the widget tree, offering more flexibility and modularity with providers not dependent on the widget hierarchy.
Which is easier to learn, Riverpod or Provider?
Provider is generally easier to learn due to its straightforward API and direct integration with Flutter's widget system. Riverpod introduces new concepts and requires a deeper understanding, which might present a steeper learning curve.
Can I use Riverpod and Provider for the same project?
Yes, you can use both Riverpod and Provider in the same project, but it’s typically advisable to stick with one solution to maintain consistency and avoid confusion.
How does performance compare between Riverpod and Provider?
Both Riverpod and Provider are performant for managing state, but Riverpod introduces additional layers of abstraction that might impact performance in specific scenarios. Provider is generally simpler and can be more efficient for straightforward use cases.
Is Riverpod more scalable than Provider?
Yes, Riverpod is designed to handle complex state management scenarios and large applications better than Provider. Its modular design and decoupling from the widget tree enhance scalability.
How does testing differ between Riverpod and Provider?
Riverpod offers better testability because its providers are independent of the widget tree, allowing for easier unit testing. Provider requires testing with the widget tree, which can be more challenging.
What are the main advantages of using Provider?
Provider is known for its simplicity, ease of use, and integration with Flutter's widget system. It has a large community and extensive documentation, making it a good choice for simpler state management needs.
What are the key benefits of using Riverpod?
Riverpod provides enhanced safety with stricter typing and scope management, better modularity, and improved testability. It’s suitable for complex state management requirements and large-scale applications.
Can Riverpod replace Provider in existing projects?
Yes, Riverpod can replace Provider in existing projects, but this would require refactoring the state management logic. Riverpod’s advanced features may offer benefits for new projects or those requiring more sophisticated state management.
Where can I find resources to learn more about Riverpod and Provider?
These FAQs should help clarify common concerns and guide developers in choosing the best state management solution for their Flutter applications. Here are 10 frequently asked questions (FAQs) about Riverpod and Provider in Flutter:
For further exploration, check out the official documentation for Provider and Riverpod. Share your experiences or ask questions in the comments to connect with other developers and learn from their insights.

Here are 10 frequently asked questions (FAQs) about Riverpod and Provider in Flutter:
FAQs
What are the main differences between Riverpod and Provider?
The provider is tightly integrated with Flutter's widget tree and is used
InheritedWidget
for state management. Riverpod decouples state management from the widget tree, offering more flexibility and modularity with providers not dependent on the widget hierarchy.
Which is easier to learn, Riverpod or Provider?
Provider is generally easier to learn due to its straightforward API and direct integration with Flutter's widget system. Riverpod introduces new concepts and requires a deeper understanding, which might present a steeper learning curve.
Can I use Riverpod and Provider for the same project?
Yes, you can use both Riverpod and Provider in the same project, but it’s typically advisable to stick with one solution to maintain consistency and avoid confusion.
How does performance compare between Riverpod and Provider?
Both Riverpod and Provider are performant for managing state, but Riverpod introduces additional layers of abstraction that might impact performance in specific scenarios. Provider is generally simpler and can be more efficient for straightforward use cases.
Is Riverpod more scalable than Provider?
Yes, Riverpod is designed to handle complex state management scenarios and large applications better than Provider. Its modular design and decoupling from the widget tree enhance scalability.
How does testing differ between Riverpod and Provider?
Riverpod offers better testability because its providers are independent of the widget tree, allowing for easier unit testing. Provider requires testing with the widget tree, which can be more challenging.
What are the main advantages of using Provider?
Provider is known for its simplicity, ease of use, and integration with Flutter's widget system. It has a large community and extensive documentation, making it a good choice for simpler state management needs.
What are the key benefits of using Riverpod?
Riverpod provides enhanced safety with stricter typing and scope management, better modularity, and improved testability. It’s suitable for complex state management requirements and large-scale applications.
Can Riverpod replace Provider in existing projects?
Yes, Riverpod can replace Provider in existing projects, but this would require refactoring the state management logic. Riverpod’s advanced features may offer benefits for new projects or those requiring more sophisticated state management.
Where can I find resources to learn more about Riverpod and Provider?
These FAQs should help clarify common concerns and guide developers in choosing the best state management solution for their Flutter applications. Here are 10 frequently asked questions (FAQs) about Riverpod and Provider in Flutter:
For further exploration, check out the official documentation for Provider and Riverpod. Share your experiences or ask questions in the comments to connect with other developers and learn from their insights.

Here are 10 frequently asked questions (FAQs) about Riverpod and Provider in Flutter:
FAQs
What are the main differences between Riverpod and Provider?
The provider is tightly integrated with Flutter's widget tree and is used
InheritedWidget
for state management. Riverpod decouples state management from the widget tree, offering more flexibility and modularity with providers not dependent on the widget hierarchy.
Which is easier to learn, Riverpod or Provider?
Provider is generally easier to learn due to its straightforward API and direct integration with Flutter's widget system. Riverpod introduces new concepts and requires a deeper understanding, which might present a steeper learning curve.
Can I use Riverpod and Provider for the same project?
Yes, you can use both Riverpod and Provider in the same project, but it’s typically advisable to stick with one solution to maintain consistency and avoid confusion.
How does performance compare between Riverpod and Provider?
Both Riverpod and Provider are performant for managing state, but Riverpod introduces additional layers of abstraction that might impact performance in specific scenarios. Provider is generally simpler and can be more efficient for straightforward use cases.
Is Riverpod more scalable than Provider?
Yes, Riverpod is designed to handle complex state management scenarios and large applications better than Provider. Its modular design and decoupling from the widget tree enhance scalability.
How does testing differ between Riverpod and Provider?
Riverpod offers better testability because its providers are independent of the widget tree, allowing for easier unit testing. Provider requires testing with the widget tree, which can be more challenging.
What are the main advantages of using Provider?
Provider is known for its simplicity, ease of use, and integration with Flutter's widget system. It has a large community and extensive documentation, making it a good choice for simpler state management needs.
What are the key benefits of using Riverpod?
Riverpod provides enhanced safety with stricter typing and scope management, better modularity, and improved testability. It’s suitable for complex state management requirements and large-scale applications.
Can Riverpod replace Provider in existing projects?
Yes, Riverpod can replace Provider in existing projects, but this would require refactoring the state management logic. Riverpod’s advanced features may offer benefits for new projects or those requiring more sophisticated state management.
Where can I find resources to learn more about Riverpod and Provider?
These FAQs should help clarify common concerns and guide developers in choosing the best state management solution for their Flutter applications. Here are 10 frequently asked questions (FAQs) about Riverpod and Provider in Flutter:
Table of content
© 2021-25 Blupx Private Limited.
All rights reserved.
© 2021-25 Blupx Private Limited.
All rights reserved.
© 2021-25 Blupx Private Limited.
All rights reserved.